The `isalnum` function in C++ checks whether a character is an alphanumeric character (either a digit or a letter).
Here's a simple example:
#include <iostream>
#include <cctype>
int main() {
char ch = 'A';
if (isalnum(ch)) {
std::cout << ch << " is alphanumeric." << std::endl;
} else {
std::cout << ch << " is not alphanumeric." << std::endl;
}
return 0;
}
Understanding `isalnum`
What is `isalnum`?
`isalnum` is a function provided by the C++ Standard Library that is used to determine whether a given character is alphanumeric. In C++, alphanumeric characters refer to both letters (uppercase and lowercase) and digits (0-9). This function plays a crucial role in character classification, assisting in input validation, data processing, and more. Understanding `isalnum` is essential for developers who want to ensure their applications handle character data correctly.
Syntax of `isalnum`
The syntax for using `isalnum` is straightforward:
#include <cctype>
int isalnum(int c);
To utilize `isalnum`, you must include the header `<cctype>`, which contains declarations for functions that classify characters.

How `isalnum` Works
Character Classification
`isalnum` checks its input character and returns a non-zero value (true) if the character is either a letter (A-Z, a-z) or a digit (0-9). If the character does not fulfill either condition, the function returns `0` (false). This simple classification helps in significantly determining the format and content of character data during runtime.
Return Value of `isalnum`
The return value of `isalnum` is vital for decision-making in your programs. A non-zero return signifies that the character is alphanumeric, while a `0` return indicates it is not. This can be effectively used in `if` statements, allowing you to branch your code based on the character classification.

Usage of `isalnum` in C++
Basic Example of Using `isalnum`
To get started, here's a simple use case of the `isalnum` function:
#include <iostream>
#include <cctype>
int main() {
char c = 'A';
if (isalnum(c)) {
std::cout << c << " is alphanumeric." << std::endl;
} else {
std::cout << c << " is not alphanumeric." << std::endl;
}
return 0;
}
In this example, the character `A` is checked. Since it is an uppercase letter, the output will confirm that it is indeed alphanumeric. This example demonstrates how to use `isalnum` in a basic form.
Checking Strings with `isalnum`
One of the powerful features of `isalnum` is the ability to check each character within a string. This can be useful in various scenarios, such as input validation within applications.
Iterating Over Characters
Here’s how you can iterate through each character in a string:
#include <iostream>
#include <cctype>
#include <string>
int main() {
std::string str = "Hello123!";
for (char c : str) {
if (isalnum(c)) {
std::cout << c << " is alphanumeric." << std::endl;
} else {
std::cout << c << " is not alphanumeric." << std::endl;
}
}
return 0;
}
In this example, the program iterates over each character in the string `"Hello123!"`, checking if each character is alphanumeric. The output will clearly indicate which characters pass the test and which do not, providing valuable feedback for data validation.
Practical Applications of `isalnum`
Input Validation
One of the most common applications of `isalnum` is to validate user input. By restricting allowable characters to alphanumeric values, you can enhance user experience and code robustness.
Here's a sample function that validates usernames:
#include <iostream>
#include <cctype>
#include <string>
bool isValidUsername(const std::string& username) {
for (char c : username) {
if (!isalnum(c)) {
return false; // Invalid character found
}
}
return true; // All characters are valid
}
int main() {
std::string username;
std::cout << "Enter a username: ";
std::cin >> username;
if (isValidUsername(username)) {
std::cout << "Valid username!" << std::endl;
} else {
std::cout << "Invalid username! Only alphanumeric characters are allowed." << std::endl;
}
return 0;
}
In this code, the `isValidUsername` function checks if the entire username consists only of alphanumeric characters. This ensures that user inputs meet your application's requirements, improving security and data integrity.
Handling Edge Cases
While `isalnum` is quite effective, it’s important to be aware of edge cases that might arise. For example, characters like null characters or various special symbols can impact program behavior.
Null Characters and Special Inputs
Consider this example:
#include <iostream>
#include <cctype>
int main() {
char specialChar = '#';
if (isalnum(specialChar)) {
std::cout << specialChar << " is alphanumeric." << std::endl;
} else {
std::cout << specialChar << " is not alphanumeric." << std::endl;
}
return 0;
}
In this case, the character `'#'` is checked, and since it's not alphanumeric, the output will indicate that it is not alphanumeric. Recognizing and handling such edge cases is key to writing robust C++ applications.

Common Mistakes with `isalnum`
Misinterpreting the Alphanumeric Check
One common error when using `isalnum` is misunderstanding what constitutes an alphanumeric character. Some programmers may mistakenly believe that other characters, including special symbols, might qualify. It’s crucial to recognize that `isalnum` only classifies letters and digits as valid.
Platform Differences
Another aspect to consider is that character classification might behave differently across various platforms or locales. To ensure consistent behavior in different environments, developers must be mindful of locale settings. Use the standard locale settings or adjust character sets to avoid unexpected results.
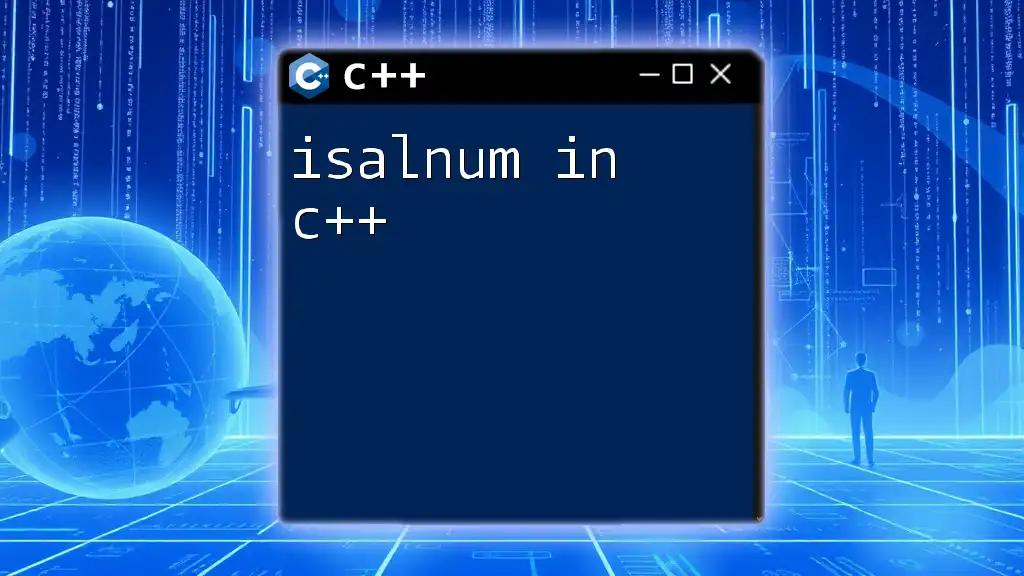
Summary
Recap of `isalnum`
In summary, the `isalnum` function is a fundamental tool in C++ for checking if a character is alphanumeric. Understanding its syntax, return values, and appropriate use cases is essential for any developer who wants to handle character data effectively.
Further Reading and Resources
For those interested in deepening their knowledge of character classification in C++, it is recommended to explore additional resources and documentation, such as the official C++ Standard Library documentation, tutorials on character manipulation, and best practices for handling user input.
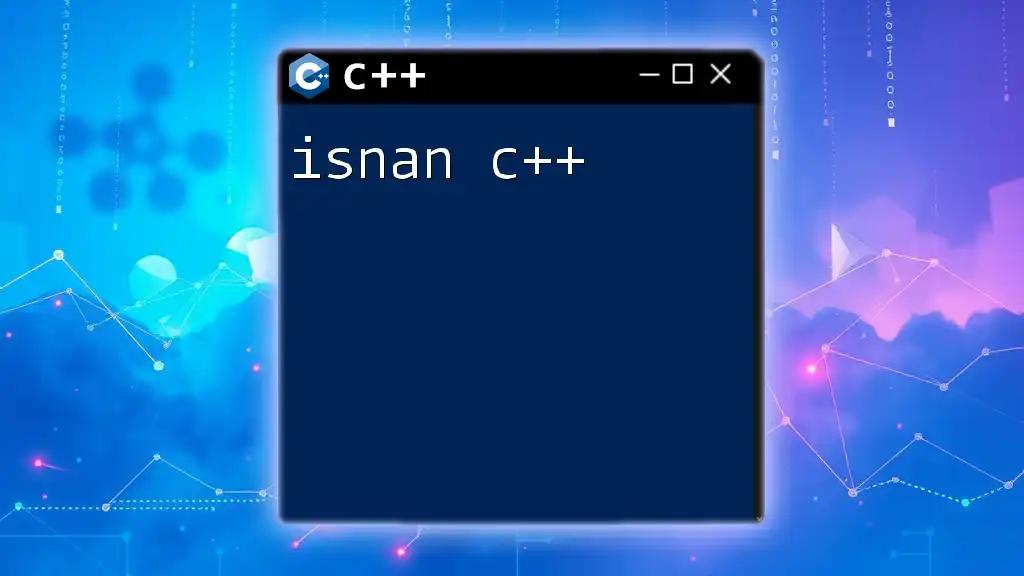
Conclusion
Mastering `isalnum` not only improves your programming skills in C++ but also enhances the reliability of your code. As you practice and integrate `isalnum` into your applications, you will find it invaluable for creating robust and user-friendly software solutions.