In C++, division can be performed using the division operator `/`, which operates on two numerical values to yield their quotient.
Here's a simple code snippet demonstrating division in C++:
#include <iostream>
int main() {
int a = 10;
int b = 2;
float result = static_cast<float>(a) / b; // Perform division
std::cout << "Result of division: " << result << std::endl;
return 0;
}
C++ Division Operators
Understanding Arithmetic Operators
In C++, arithmetic operations are fundamental for performing calculations. Among these operators, the division operator is crucial for splitting quantities into equal parts.
Using the Division Operator
The primary division operator in C++ is represented by the `/` symbol. This operator allows you to divide one number by another.
Syntax:
result = dividend / divisor;
Example of Basic Division Operation:
int a = 10;
int b = 2;
int result = a / b; // result will be 5
In this example, the integer `10` is divided by `2`, resulting in `5`. Here, both the dividend and divisor are integers.
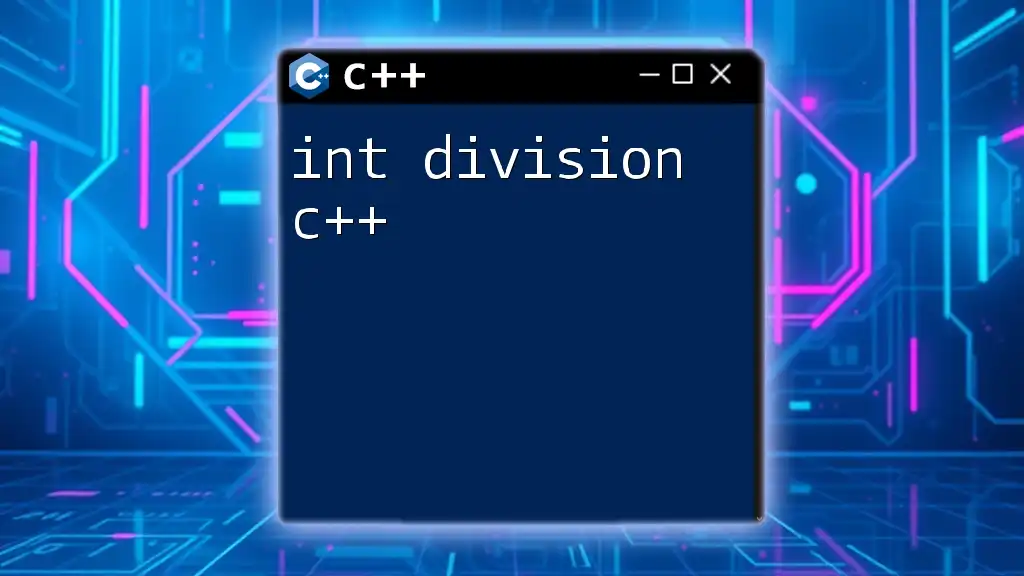
Different Types of Division
Integer Division
Integer division plays a significant role in C++. When both the dividend and divisor are integers, the result is also an integer. Importantly, any decimal component is truncated, not rounded.
Example of Integer Division:
int a = 7;
int b = 3;
int result = a / b; // result will be 2 (not 2.3333)
In the above code, dividing `7` by `3` produces `2`, trimming away the `.3333`.
Floating-Point Division
Conversely, when you involve floating-point numbers (i.e., numbers that contain a decimal point), C++ retains the decimal representation. This method is vital for applications requiring precision.
Example of Floating-Point Division:
float a = 7.0;
float b = 3.0;
float result = a / b; // result will be approximately 2.3333
This operation leads to a more precise result because both numbers are treated as floating-point numbers.
Mixed Division
Often in programming, you'll deal with mixed data types, such as an integer divided by a floating-point number. In this case, C++ promotes the integer to a floating-point type.
Example of Mixed-Type Division:
int a = 7;
float b = 2.0;
float result = a / b; // result will be 3.5
Here, the integer `7` is divided by the floating-point `2.0`, resulting in `3.5`, demonstrating how C++ handles mixed types seamlessly.
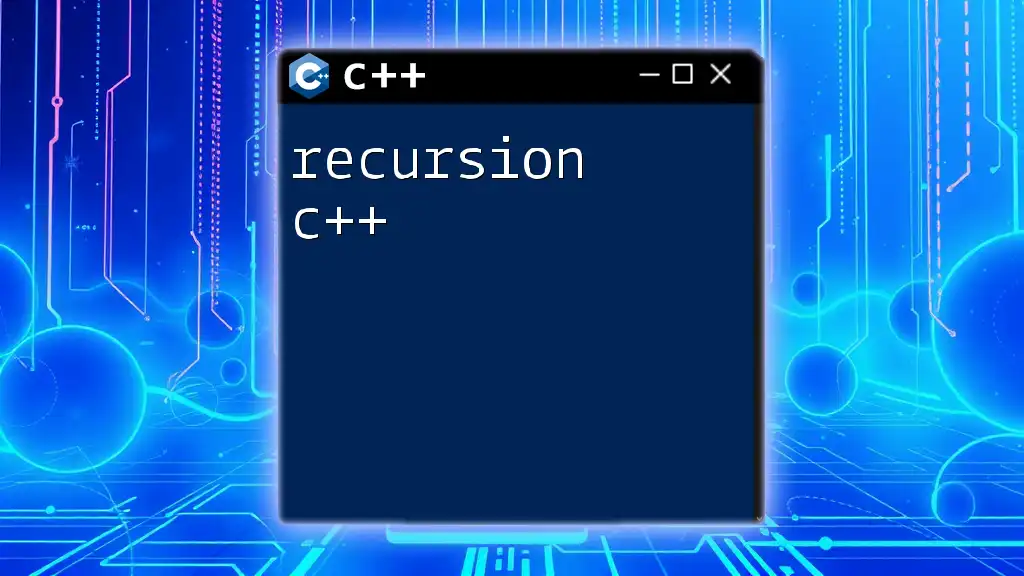
Common Division Pitfalls
Division by Zero
One of the most critical errors to guard against in C++ is division by zero. Attempting to divide a number by zero leads to undefined behavior, often causing runtime errors that can crash your program.
Preventive Measures: Always validate the divisor before performing a division operation.
int a = 10, b = 0;
if (b != 0) {
int result = a / b;
} else {
// Handle division by zero
std::cout << "Cannot divide by zero!" << std::endl;
}
This example demonstrates a simple check to prevent division by zero, ensuring your program remains stable.
Rounding Errors
With floating-point arithmetic, rounding errors can occur due to the limitations of representing decimal numbers in binary. This issue can lead to a loss of precision.
Example Demonstration of Potential Inaccuracies:
float a = 0.1;
float b = 0.2;
float result = a + b; // result may not equal 0.3 due to precision issues
In this scenario, the sum of `0.1` and `0.2` may not precisely equal `0.3`, showcasing how small discrepancies can emerge when dealing with floating-point numbers.
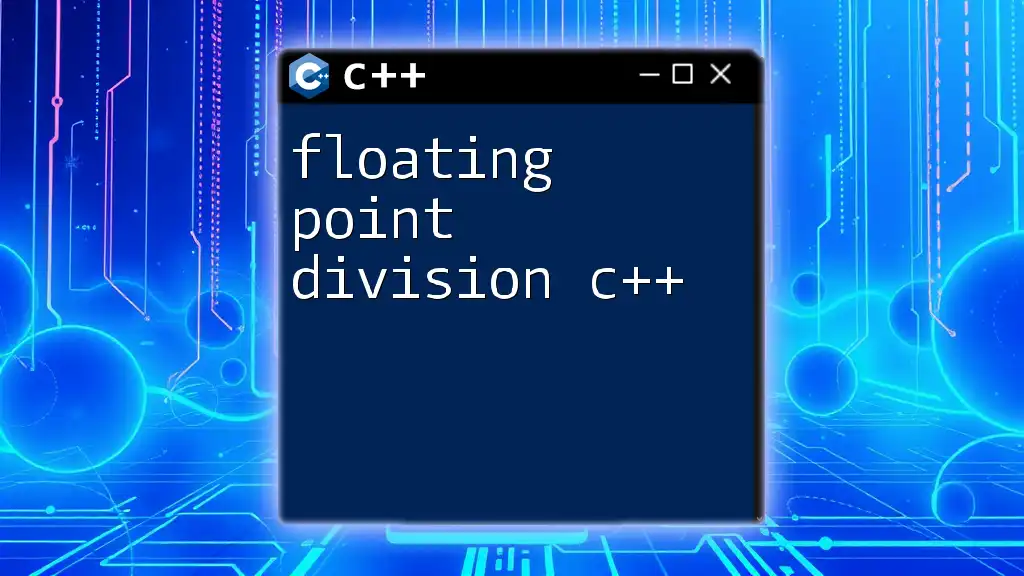
Advanced Division Techniques
The Modulus Operator
Another relevant operator in C++ is the modulus operator `%`, which provides the remainder of a division operation. This is beneficial when you need to identify whether a number is even or odd, among other applications.
Example Showing How Modulus Can Be Useful:
int a = 10;
int b = 3;
int quotient = a / b;
int remainder = a % b; // remainder will be 1
In this case, using the modulus operator with `10` and `3` gives you a remainder of `1`, as `10` divided by `3` leaves a leftover of `1`.
Custom Division Functions
In some cases, it may be more appropriate to write your custom division function, especially when you want to manage errors or special cases more effectively.
Example of a Simple Division Function Handling Edge Cases:
float divide(int numerator, int denominator) {
if (denominator == 0) {
throw std::runtime_error("Division by zero");
}
return static_cast<float>(numerator) / denominator;
}
This custom function checks for division by zero and throws an exception if the denominator is zero, improving the safety and reliability of your code.
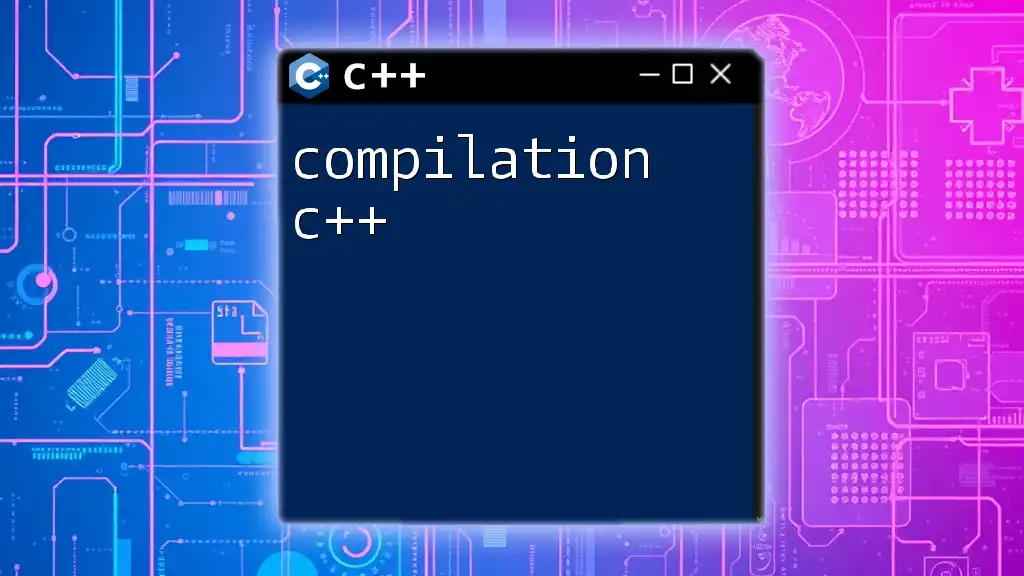
Conclusion
Mastering division in C++ is fundamental to any programmer’s skill set. Understanding the nuances between integer and floating-point division, as well as pitfalls to avoid, will make your coding more robust and reduce bugs significantly. Exploring division further through custom functions and operators like modulus can open avenues for more advanced programming tasks. Dive deeper into real-world applications, and let your understanding of "division c++" elevate your coding proficiency!
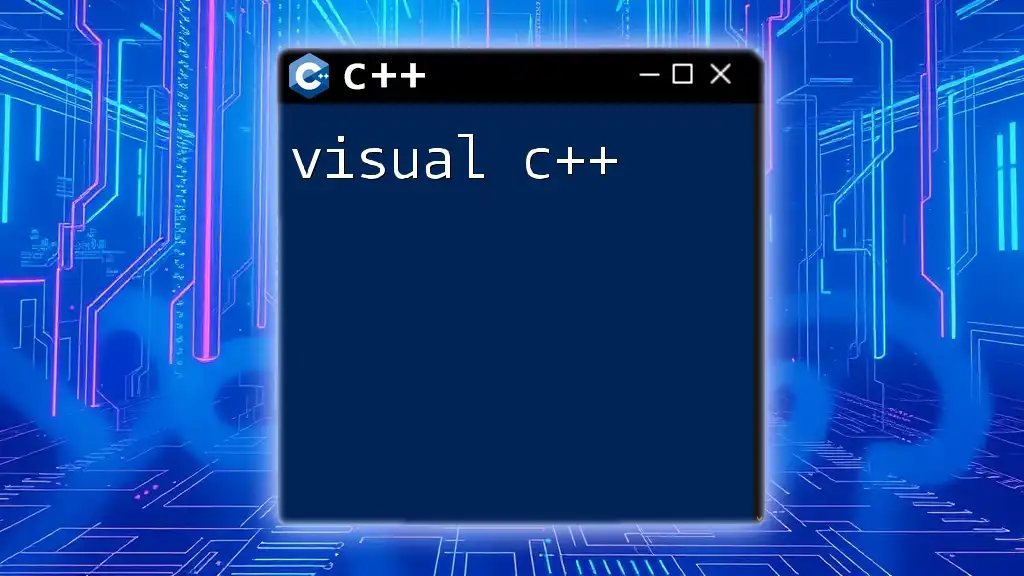
Additional Resources
For further reading, you can refer to the official C++ documentation on arithmetic operators and explore various tutorials that delve deeper into programming in C++. Engaging with community forums and platforms can also enrich your learning experience as you discuss challenges and solutions with fellow learners.