Drogon is a high-performance C++ web application framework designed to simplify the development of web services with asynchronous support and an easy-to-use interface.
Here's a basic example of a Drogon application that handles a simple HTTP GET request:
#include <drogon/drogon.h>
int main() {
drogon::app().registerHandler("/hello", [](const drogon::HttpRequestPtr &req,
std::function<void (const drogon::HttpResponsePtr &)> &&callback) {
auto resp = drogon::HttpResponse::newHttpResponse();
resp->setBody("Hello, Drogon!");
callback(resp);
}).setThreadNum(4).run();
return 0;
}
What is Drogon?
Drogon is a modern web application framework for C++, specifically designed to facilitate the development of high-performance web applications. Leveraging an asynchronous I/O model, Drogon provides a robust environment for building scalable web services. What truly sets Drogon apart is its focus on performance, making it an ideal choice for applications that require quick and efficient handling of requests.
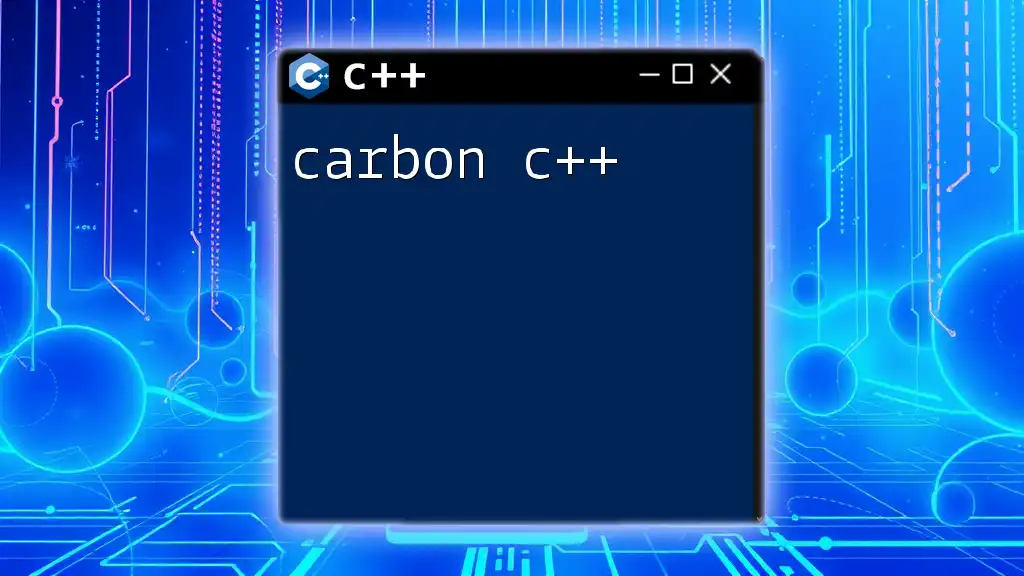
Why Choose Drogon?
When choosing a framework, performance and functionality are paramount. With Drogon, you benefit from:
- Performance Advantages: The asynchronous I/O model not only enhances performance but also the ability to handle thousands of simultaneous connections without blocking.
- C++ Standards Compliance: It adopts modern C++ features, allowing developers to write clean, efficient, and maintainable code.
- Active Community and Support: Drogon's dedicated user community and comprehensive documentation provide a solid foundation for learning and troubleshooting.
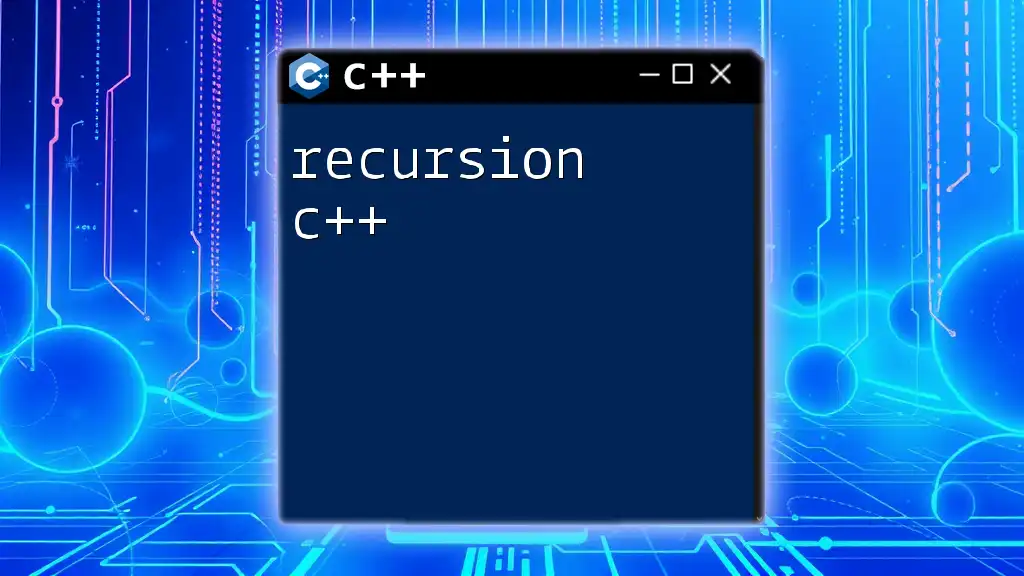
Getting Started with Drogon
Installation
Setting up Drogon is straightforward but requires a few dependencies. For a successful installation, you will need to install the following:
- libuv: A multi-platform support library with a focus on asynchronous I/O.
- OpenSSL: For secure communications over the web.
To install Drogon, follow these steps for your operating system:
Ubuntu Example:
# Installing required dependencies
sudo apt install libuv1-dev libssl-dev
# Cloning the Drogon repository
git clone https://github.com/drogonframework/drogon.git
Once installed, navigate to the Drogon folder and build the project using `cmake`.
Project Structure
A typical Drogon project has a well-defined structure that maximizes clarity and organization. Here's a brief overview of the primary components:
- Controllers: Where you define the logic for handling HTTP requests.
- Models: Data structures typically used for interacting with databases.
- Views: Templates for rendering responses.
This organized layout allows developers to manage their code efficiently, making it easier to collaborate and maintain.
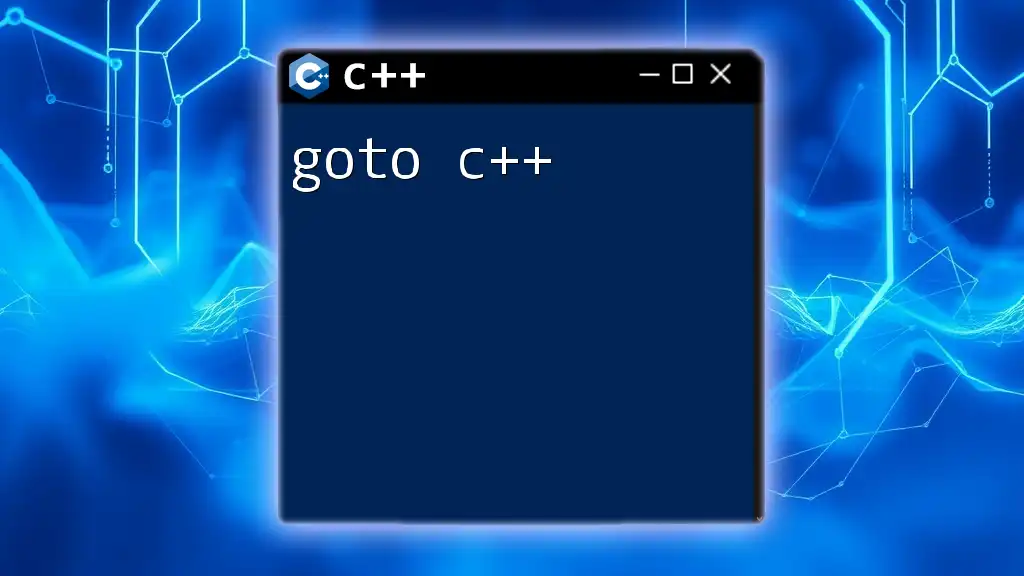
Core Concepts in Drogon
Routing
Routing is a fundamental aspect of any web application, determining how the application responds to specific client requests. In Drogon, routing is defined using easily readable syntax.
Here's an example of how to set up a simple route:
// Example of defining a route in a Drogon application
app().registerHandler("/hello", [](const HttpRequestPtr &req, std::function<void(const HttpResponsePtr &)> &&callback) {
auto resp = HttpResponse::newHttpResponse();
resp->setBody("Hello, World!");
callback(resp);
});
In this snippet, when a user accesses the `/hello` endpoint, they will receive a "Hello, World!" response. Drogon's routing system can handle various types of requests, including GET, POST, and more, making it versatile for different web applications.
Controllers
Controllers in Drogon serve as the backbone of request handling. They encapsulate the logic necessary to process incoming requests and produce a suitable response.
Creating a controller is intuitive. Below is a simple example:
class HelloController : public drogon::HttpController<HelloController> {
public:
void hello(const HttpRequestPtr &req, std::function<void(const HttpResponsePtr &)> &&callback) {
auto resp = HttpResponse::newHttpResponse();
resp->setBody("Hello from HelloController");
callback(resp);
}
};
In this example, `HelloController` manages the `/hello` endpoint and returns a response when this endpoint is accessed.
Middleware
Middleware provides a mechanism to modify requests and responses globally, allowing developers to implement additional functionalities such as logging, authentication, or request validation.
Here's how you can create a middleware in Drogon:
class ExampleMiddleware {
public:
void operator()(const HttpRequestPtr &req, std::function<void(const HttpResponsePtr &)> &&callback) {
// You can perform operations here, such as logging
std::cout << "Received request for: " << req->getPath() << std::endl;
callback(req);
}
};
When applied, this middleware will log every incoming request, enhancing your application's observability.
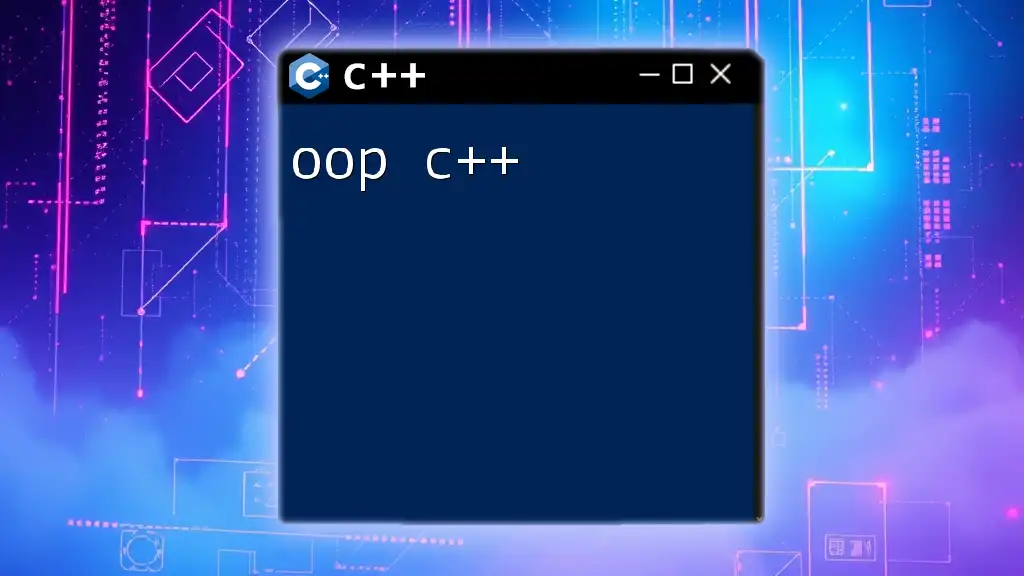
Advanced Features
Asynchronous Programming
Asynchronous programming is a critical feature of Drogon, allowing your application to handle multiple requests concurrently. This is especially important for applications experiencing heavy traffic.
Consider this simple example of an asynchronous route handler:
app().registerHandler("/async", drogon::async<std::string>([](const HttpRequestPtr &req) -> std::string {
return "This is an asynchronous response";
}));
This route will return an asynchronous response, significantly improving performance under load.
Template Rendering
Drogon integrates a template engine that allows developers to create dynamic web pages easily. Rendering templates helps separate your application’s logic from its presentation.
Here’s a brief example of how to render a response using JSON:
auto response = HttpResponse::newHttpJsonResponse(json::object({{"key", "value"}}));
response->setContentTypeCode(CT_JSON);
callback(response);
This snippet constructs a JSON response, showcasing Drogon’s capability to handle different response formats seamlessly.
Database Integration
Connecting to a database is crucial for most web applications. Drogon provides built-in support for popular databases, such as PostgreSQL and MySQL, allowing you to perform CRUD operations effectively.
To set up a PostgreSQL connection, for example, you might use the following:
// Example of connecting to a PostgreSQL database
orm::DbClient::newDbClient("host=localhost user=yourusername password=yourpassword dbname=yourdb");
Building on this connection, you can execute queries and handle results through Drogon's ORM capabilities, streamlining your database interactions.
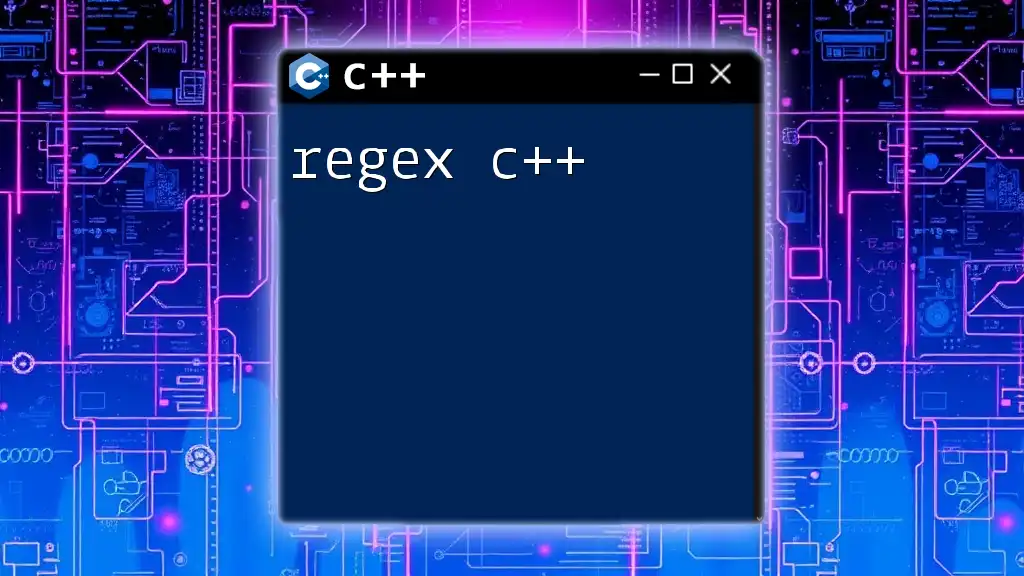
Testing and Deployment
Unit Testing
Testing is key to ensuring your web application functions as expected. Drogon integrates well with popular testing libraries like Google Test or Catch2.
Here’s a simple unit test example for a controller:
TEST(HelloControllerTest, TestHello) {
// Example test case for HelloController
ASSERT_EQ(1, 1); // A basic assertion, could be improved with actual logic
}
Creating unit tests helps maintain code quality and ensures that new changes do not introduce bugs into your application.
Deployment Strategies
Once development is complete, deploying your Drogon application involves selecting appropriate hosting options. Common choices include dedicated servers, containers, and cloud providers.
Best practices for deployment involve:
- Configuration Management: Keep your configurations separated from your codebase.
- Monitoring: Implement logging and monitoring to track application performance and catch issues early.
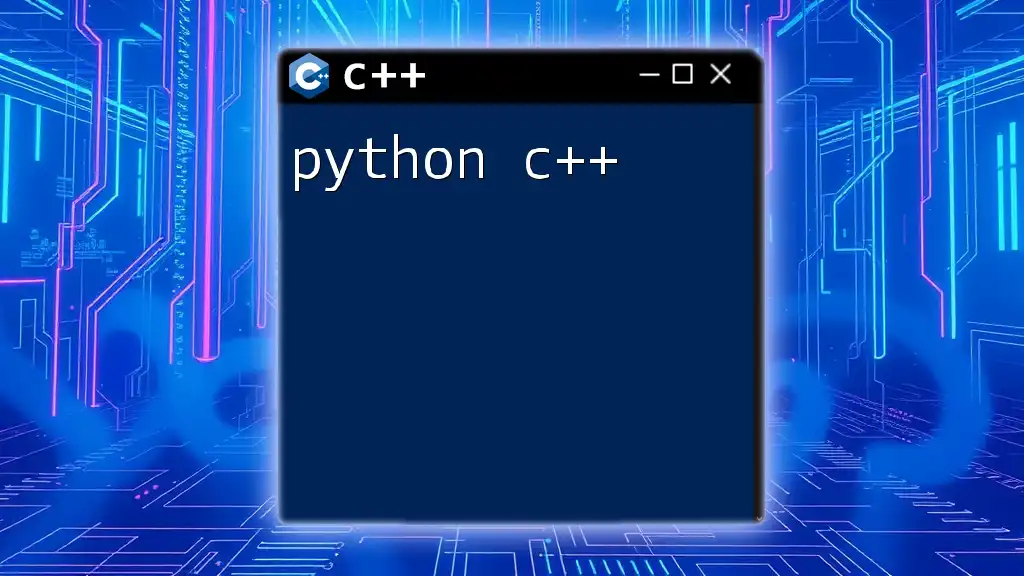
Final Thoughts
In summary, Drogon C++ offers a powerful, efficient framework for building web applications. With its focus on performance, modern C++ features, and a supportive community, Drogon is worth exploring for developers looking to create robust web services.
Don’t hesitate to dive deeper into these topics and experiment with different features of Drogon. Your journey in mastering this framework starts here, and the possibilities are endless!
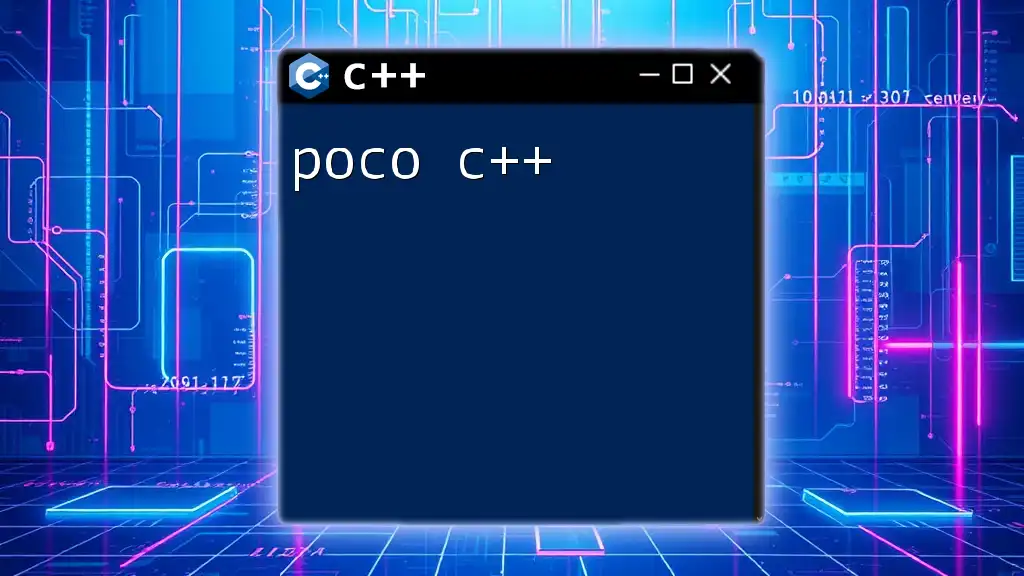
Additional Resources
For further exploration, visit the official Drogon documentation and GitHub repository. These resources, alongside recommended books and tutorials, will help you deepen your understanding of this powerful web application framework.