Doxygen is a documentation generator that helps C++ developers create easy-to-read documentation from annotated source code, allowing for efficient maintenance and understanding of the codebase.
Here’s how you can use Doxygen comments in your C++ code:
/**
* \brief Adds two integers.
*
* This function takes two integers as input and returns their sum.
*
* \param a First integer to add.
* \param b Second integer to add.
* \return The sum of a and b.
*/
int add(int a, int b) {
return a + b;
}
What is Doxygen?
Doxygen is a powerful documentation generation tool designed primarily for C++, although it also supports other programming languages such as C, Java, Python, and more. It transforms annotated source code into comprehensive documentation in various formats—including HTML, LaTeX, and RTF—making it easier for developers to maintain high-quality codebases. Utilizing Doxygen effectively enhances code readability and facilitates collaborative development by providing clear, structured, and updated documentation.
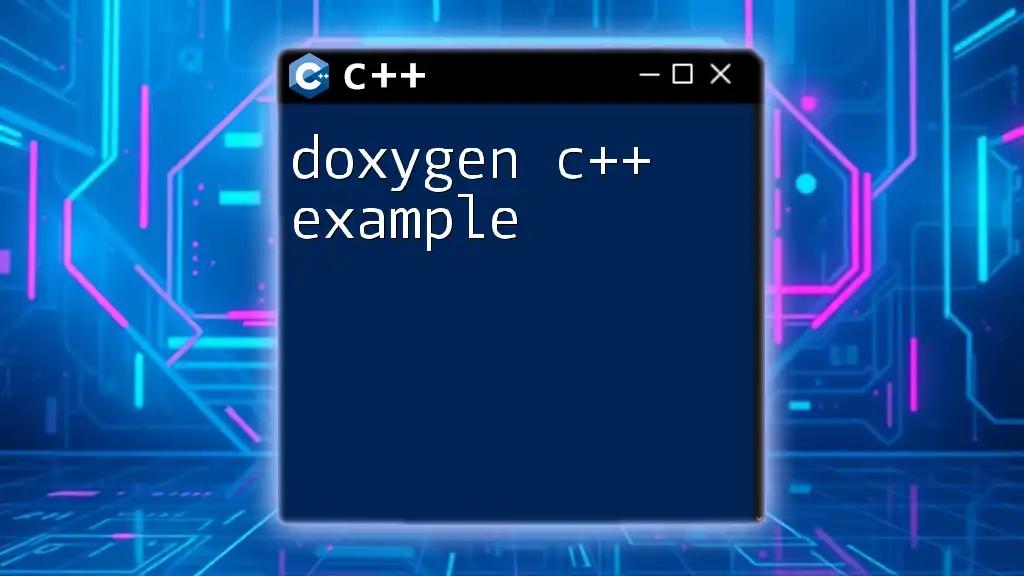
Benefits of Using Doxygen
Using Doxygen offers numerous benefits for developers and teams.
Improved Documentation: By integrating Doxygen comments into the code, developers can automatically generate documentation that accurately reflects the current state of the codebase. This minimizes the risk of outdated documentation, which can lead to confusion and bugs.
Enhanced Code Readability: Well-documented code is easier to navigate, making onboarding new team members simpler and faster. Clear comments help developers understand the purpose and functionality of each function and class.
Facilitates Collaboration Among Development Teams: Doxygen promotes consistent documentation practices across teams, ensuring everyone is on the same page and reducing misunderstandings.

Setting Up Doxygen
Installation Procedures
To get started, you need to install Doxygen on your preferred operating system.
Installing Doxygen on Different Operating Systems:
- Windows: Download the installer from the official Doxygen website and follow the prompts to complete the installation.
- macOS: Use Homebrew to install Doxygen by executing the command:
brew install doxygen
- Linux: Most distributions include Doxygen in their package management system. For example, on Ubuntu, you can install it using:
sudo apt-get install doxygen
Configuring Doxygen for a C++ Project
Once installed, the next step is to configure Doxygen for your C++ project. This is done through a configuration file called Doxyfile.
Introduction to the Doxyfile: The Doxyfile contains settings that control how Doxygen processes your code and generates documentation. You can create a default Doxyfile by running:
doxygen -g Doxyfile
Basic Configuration Options:
- PROJECT_NAME: Set the name of your project.
- OUTPUT_DIRECTORY: Specify where you want Doxygen to generate the output documentation.
- INPUT: Define the source files or directories that Doxygen should scan for documentation comments.

Documenting C++ Code with Doxygen
Adding Doxygen Comments
To leverage Doxygen fully, you need to add comments in your C++ code that Doxygen can parse. There are different styles of comments you can use, including C-style and C++-style comments.
Practical Example:
/**
* @brief Brief description of the function
* @param a An integer parameter
* @return The result of the operation
*/
int exampleFunction(int a);
In this example, the `@brief` tag provides a concise summary of the function, while the `@param` and `@return` tags describe the parameters and return type, respectively.
Special Doxygen Commands
Doxygen supports several commands that allow for detailed and structured documentation.
- @param: Use this to document function parameters.
- @return: Use this for documenting return values.
- @brief: Provides a short summary explaining what a function does.
- @details: Adds in-depth explanations.
- @note: For any extra information that could be helpful to the developer.
- @warning: To highlight critical issues or side effects.

Advanced Doxygen Features
Organizing Code Documentation
For larger projects, you might want to organize your documentation effectively. Doxygen allows you to create modules and groups through specific commands:
- @defgroup: This command helps you define a group of related functions or other elements.
- @ingroup: Allows you to associate a function or member with a previously defined group.
Example:
/**
* @defgroup MathFunctions Mathematical Functions
* @{
*/
/**
* This function adds two integers.
* @ingroup MathFunctions
*/
int add(int a, int b);
/** @} */
In this snippet, we define a group called `MathFunctions` and associate the `add` function with it. This helps keep related functions together in the generated documentation.
Generating Class Diagrams
Doxygen also supports the generation of class diagrams using Graphviz. To enable this feature, set the `HAVE_DOT` option to `YES` in the Doxyfile. This helps visualize the relationships between classes, which can be crucial for understanding complex systems.
Customizing Output Styles
Doxygen provides various formatting options for the generated documents. You can customize HTML output styles, create LaTeX documents, and even modify templates to fit your preferred look and feel. Understanding how to adjust these settings will allow your documentation to reflect your project's branding.

Running Doxygen
Generating Documentation
Once you've added comments and configured your project, the next step is to generate the documentation.
Step-by-step Guide on How to Run Doxygen:
- Open a terminal or command prompt.
- Navigate to the directory containing your Doxyfile.
- Run the command:
doxygen Doxyfile
Interpreting Doxygen Outputs
After running Doxygen, check the specified OUTPUT_DIRECTORY for generated documentation files. The typical structure includes an index page summarizing the documentation, class hierarchy graphs, and individual pages for each documented element. Understanding this structure helps in navigating the documentation effectively.

Best Practices for Doxygen Comments
Writing Clear and Concise Comments
When documenting your code, clarity is paramount. Comments should be straightforward to read and free of jargon.
Tips for Non-Native Speakers: Use simple vocabulary and structure sentences directly. Keeping comments consistent across your codebase is equally important.
Maintaining Documentation
Documentation should be treated as a living part of your code. Regular updates are essential, especially whenever code changes occur. Consider integrating the documentation updating process into your code review practices to ensure it remains current.
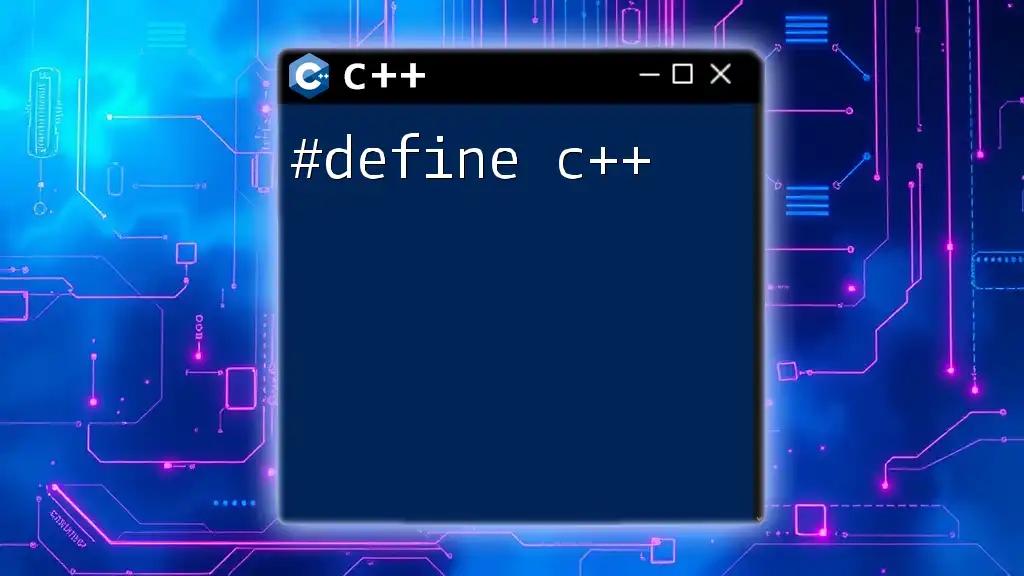
Troubleshooting Common Doxygen Issues
Common Configuration Errors
During the use of Doxygen, you may encounter some common configuration errors. These typically include missing or improperly set paths in the Doxyfile or unrecognized commands.
Debugging Tips
If you run into issues, enabling verbose output can provide additional information that can be helpful in diagnosing problems. Additionally, check any log files generated by Doxygen, as they often contain valuable error messages that can guide you in resolving issues.

Conclusion
Doxygen is an essential tool for any C++ developer serious about maintaining high-quality documentation. By taking the time to integrate Doxygen comments into your code, you not only improve your project's documentation but also enhance its overall maintainability and collaboration potential. Start using Doxygen today and elevate your code quality!

Additional Resources
To further your understanding of Doxygen and its applications in C++, refer to the official Doxygen [documentation](http://www.doxygen.nl/manual/index.html). Additionally, consider exploring recommended books and online courses that provide in-depth tutorials on effective code documentation. Don’t hesitate to engage with community forums and user groups for support, tips, and best practices!