In C++, an index is used to access individual elements in an array or a vector, allowing you to retrieve or modify data at a specific position.
Here's a simple example demonstrating how to access elements in an array:
#include <iostream>
using namespace std;
int main() {
int arr[5] = {10, 20, 30, 40, 50};
cout << "The third element is: " << arr[2] << endl; // Indexing starts at 0
return 0;
}
Understanding Indices
What are Indices?
In C++, an index is a numerical representation that allows you to access elements within data structures like arrays, strings, and various containers. Each element in these structures is assigned a unique index, which you can use to manipulate or retrieve data.
Zero-based Indexing
C++ employs zero-based indexing, which means that the first element of an array or string is accessed using the index 0. This approach is common in many programming languages and contributes to efficient memory management and computations.
Example: Here's a simple illustration of zero-based indexing:
int arr[] = {10, 20, 30, 40, 50};
cout << arr[0]; // Outputs: 10
Here, `arr[0]` refers to the first element of the array, which is `10`. Understanding this concept is pivotal as it lays the foundation for how you interact with data in C++.
One-based Indexing
While C++ uses zero-based indexing, some other programming languages, such as Fortran and MATLAB, utilize one-based indexing. In this system, the first element is accessed with index 1. Recognizing this difference is key, particularly when transitioning between languages or interpreting code from other programming paradigms.
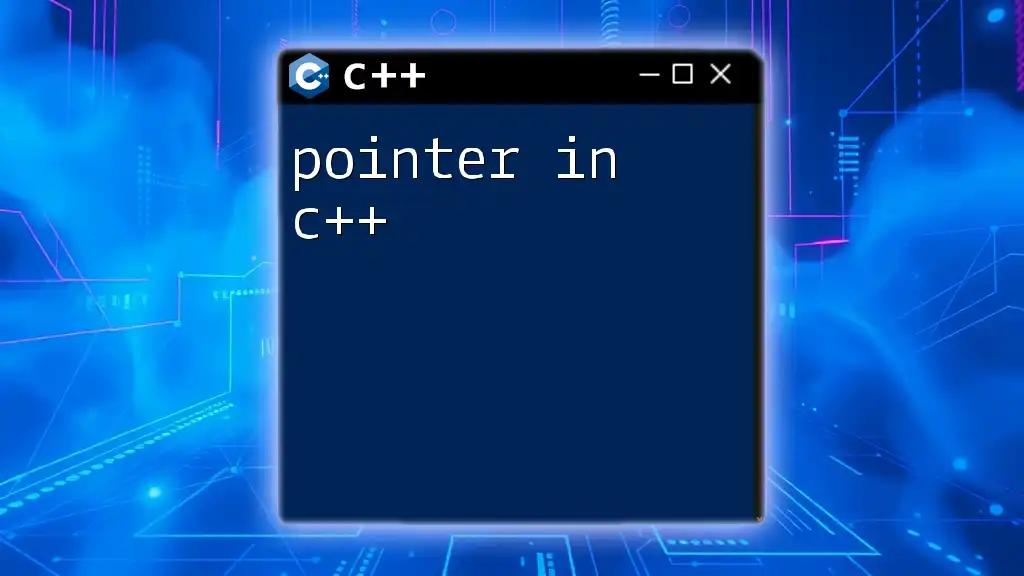
Arrays and Indices
Declaring and Initializing Arrays
C++ provides a straightforward syntax to declare arrays. An array is a collection of elements of the same type stored in contiguous memory locations. The syntax generally follows this pattern:
type arrayName[size];
Example: Here’s how to declare and initialize an array:
int myArray[5] = {1, 2, 3, 4, 5};
This line creates an integer array named `myArray` that holds five elements.
Accessing Array Elements
Once an array is declared, you can access and modify its elements using their indices. This is a fundamental operation in C++ programming.
Example: If you want to change the value of the third element in the `myArray` array to `10`, you can do so with:
myArray[2] = 10; // Changes the third element to 10
Looping Through Arrays
Indices play a critical role when you need to iterate over arrays. Using loops, particularly `for` loops, you can navigate through the elements efficiently.
Example: Here’s how to loop through an array and print its elements:
for (int i = 0; i < 5; i++) {
cout << myArray[i] << " ";
}
This code snippet will output each element of the array sequentially.
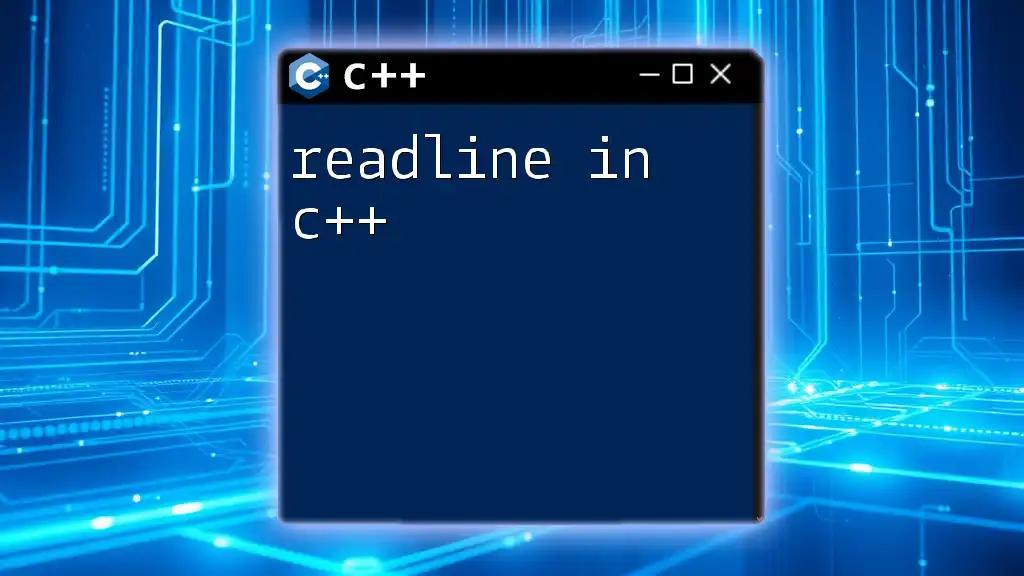
Strings and Indices
Understanding Strings in C++
In C++, a string is essentially an array of characters. Recognizing strings as character arrays is important, especially when dealing with indices.
Accessing Characters in Strings
You can access individual characters in a string just like you would with an array. C++ strings support indexing through the bracket notation.
Example:
string myString = "Hello";
cout << myString[1]; // Outputs: e
You can also modify specific characters:
myString[1] = 'a'; // Changes string to "Hallo"
Common String Operations with Indices
Functions such as `length()` and `substr()` utilize indices for various operations. For instance, `substr()` allows you to extract a portion of a string using starting index and length.
Example: Using the `substr()` function:
string sub = myString.substr(1, 3); // Outputs: "all"
In this instance, `substr(1, 3)` starts from index `1` and grabs the next three characters.
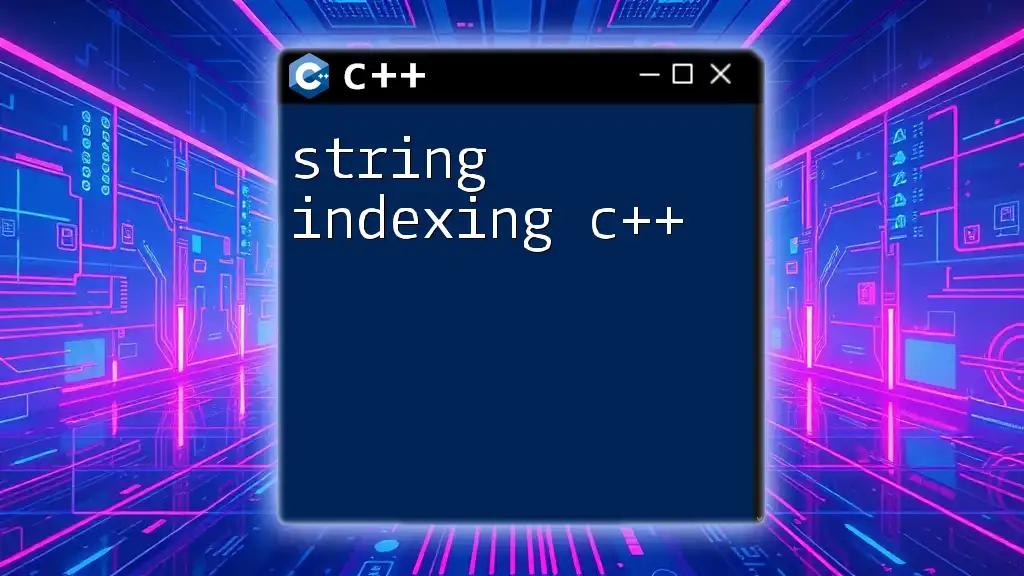
C++ Standard Library Containers and Indices
Introduction to STL (Standard Template Library)
C++’s Standard Template Library (STL) includes several containers, primarily vectors, lists, and maps. Each container has specific rules for indexing that are essential to understand.
Using Indices with Vectors
Vectors are dynamic arrays that can resize themselves automatically. To access elements in a vector, you can use indices similarly to arrays.
Example: Here’s a demonstration:
vector<int> vec = {1, 2, 3, 4};
cout << vec[2]; // Outputs: 3
vec[2] = 10; // Changes the third element to 10
Differences in Indexing for Other Containers
When using other STL containers, the concept of indexing can vary. For example, a `list` uses iterators to traverse elements, while a `map` uses keys as indices.
Code Snippet: Iterating through a `map` involves the following approach:
map<string, int> m;
m["one"] = 1;
m["two"] = 2;
for (auto& pair : m) {
cout << pair.first << " : " << pair.second << endl;
}
In this case, the keys (`"one"` and `"two"`) act as the index for accessing values.
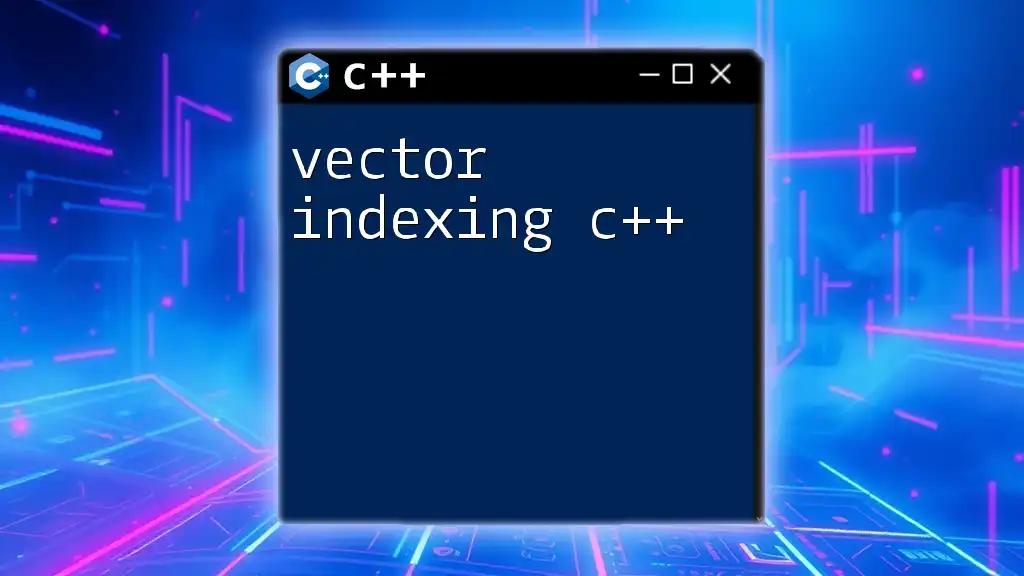
Advanced Indexing Techniques
Multi-dimensional Arrays
C++ supports multi-dimensional arrays, allowing you to create complex data structures. These arrays can be accessed using multiple indices.
Example: Here’s how you declare and access a two-dimensional array:
int matrix[2][3] = {{1, 2, 3}, {4, 5, 6}};
cout << matrix[1][2]; // Outputs: 6
In this snippet, the code accesses the element located in the second row and third column.
Using Indices with Pointers
Pointers in C++ can enhance your ability to work with arrays, as they reference the base address of the array. You can access array elements using pointer arithmetic or indexing.
Code Snippet:
int* p = myArray;
cout << p[1]; // Outputs: 2
This example shows how to use a pointer to navigate through an array, emphasizing the flexible control pointers provide.
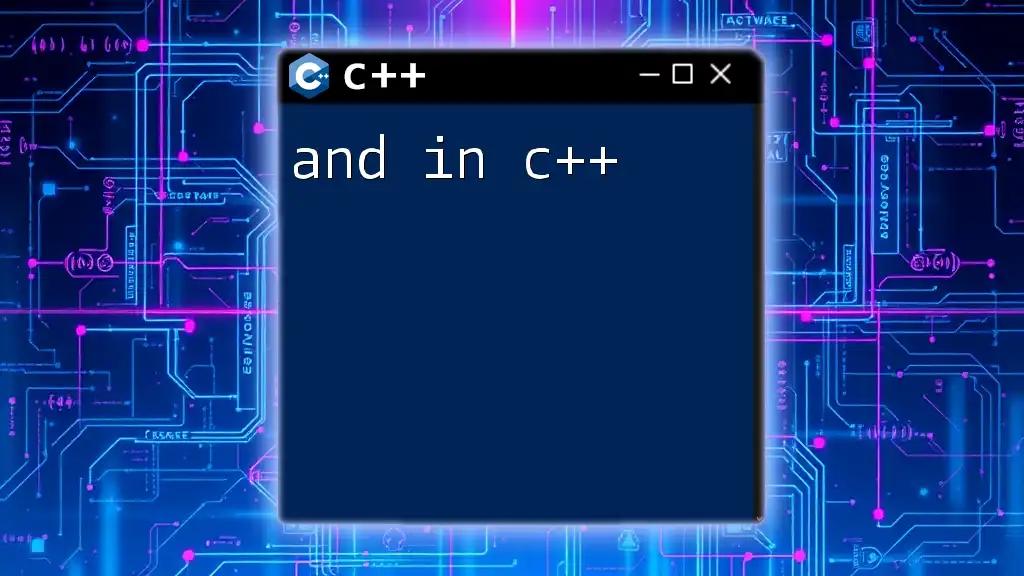
Conclusion
Understanding the index in C++ is crucial for effective programming. Indices allow you to access and manipulate data structures such as arrays and strings with ease. Mastery of indices facilitates better coding practices and promotes efficient algorithms.
By exploring elements through code snippets and practical examples, you can build a solid foundation to handle more complex scenarios involving indices in your C++ programming journey.