The `final` keyword in C++ is used to indicate that a class cannot be inherited from or that a virtual method cannot be overridden in derived classes.
class Base {
public:
virtual void show() final { // This method cannot be overridden
std::cout << "Base class show function called." << std::endl;
}
};
class Derived : public Base {
public:
// void show() override { // Uncommenting this will cause a compile error
// std::cout << "Derived class show function called." << std::endl;
// }
};
What is the `final` Keyword?
The `final` keyword in C++ serves as a powerful tool to manage class behavior and inheritance. It was introduced in C++11 and enables developers to impose restrictions on class inheritance and method overriding. By marking a class or method as `final`, you convey to both the compiler and other developers that certain behaviors should not be altered, enhancing both safety and clarity in your design.
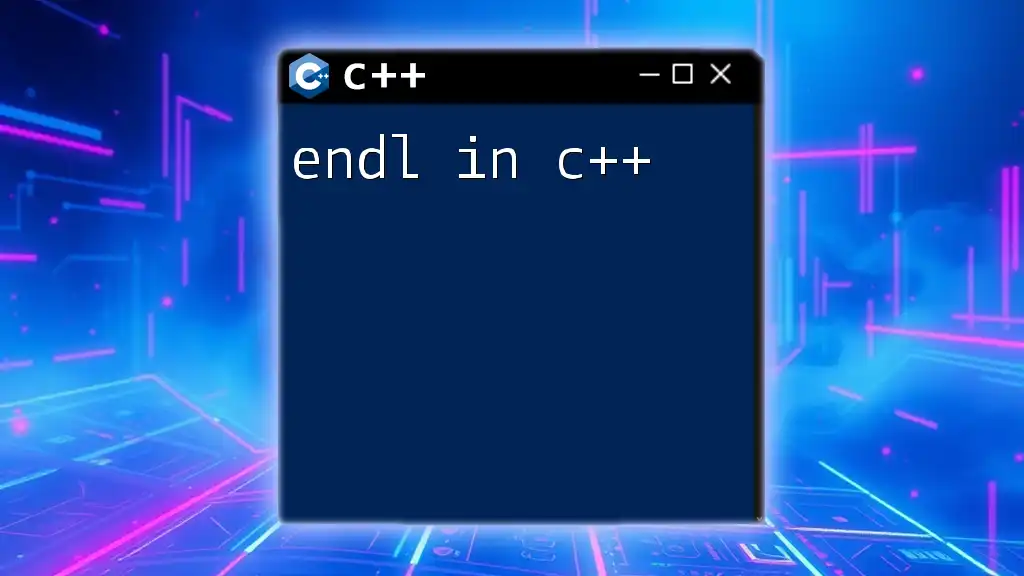
Why Use `final`?
Utilizing the `final` keyword presents several benefits, particularly in situations where control over class behavior is paramount:
-
Preventing Further Inheritance: When a class is marked as `final`, no other class can inherit from it. This restriction helps avoid unintended modifications or misuse of class functionality.
-
Avoiding Unintended Method Overrides: A method declared as `final` cannot be overridden in derived classes, ensuring that the intended functionality remains intact.
Consider a scenario where you have a class with critical business logic. By marking it as `final`, you prevent future developers from creating subclasses that could inadvertently alter its core functionality.
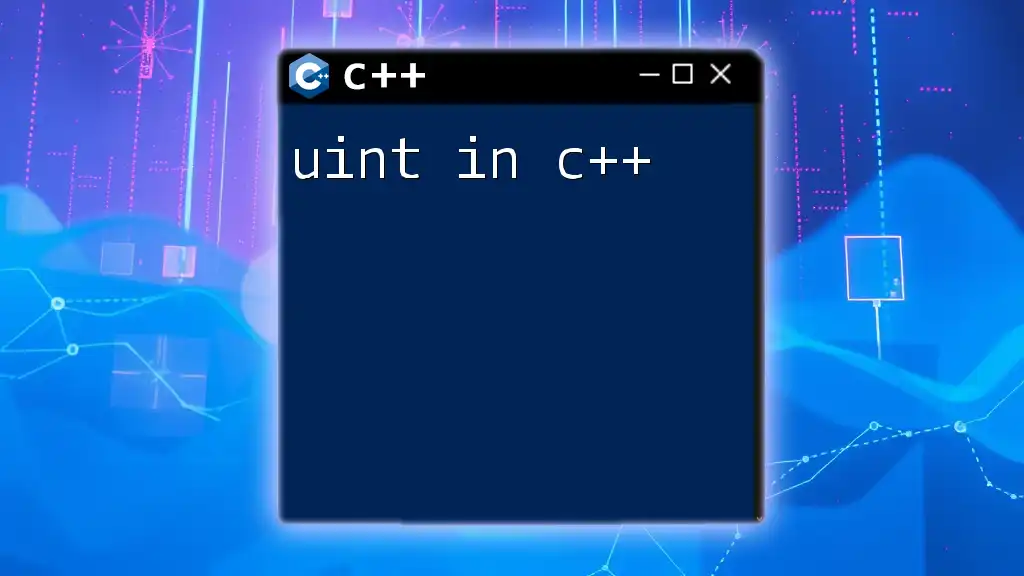
The `final` Keyword in Class Definitions
Definition of a `final` Class
A final class in C++ is one that cannot serve as a base class. By declaring a class as `final`, you indicate that it is the endpoint of inheritance; no subclasses can be created from it.
Code Example: Declaring a Final Class
class Base {
public:
virtual void show() {}
};
class FinalClass final : public Base {
public:
void show() override {
std::cout << "Final Class Show" << std::endl;
}
};
// Attempting to derive from FinalClass results in an error
// class Derived : public FinalClass { }; // Error: cannot derive from 'FinalClass'
In this example, the `FinalClass` cannot be inherited from, preventing any subclass from overriding its methods or altering its behavior.
Implications of a Final Class
When a class is defined as `final`, it establishes clear boundaries for its use. Not only does it prevent inheritance, but it also signifies that the class is designed to be complete and self-contained. Developers benefit from the performance optimizations provided by the compiler when it knows certain class behaviors can't be changed.
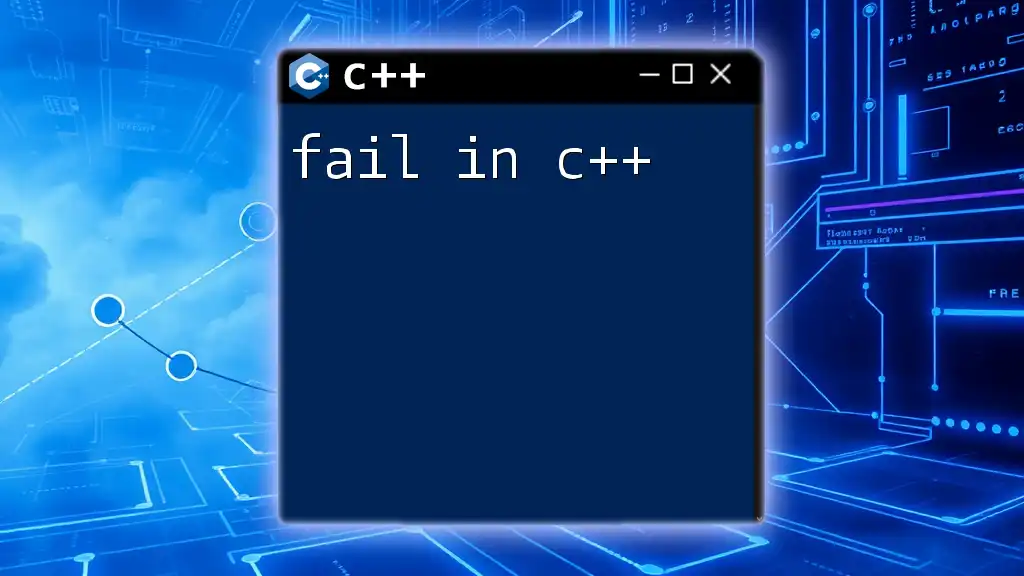
Using `final` with Virtual Functions
Understanding Virtual Functions
Virtual functions are an essential part of C++'s approach to polymorphism. They allow derived classes to override methods defined in base classes, offering flexibility in class behavior. However, sometimes it is necessary to constrain this flexibility.
Making Virtual Functions Final
Declaring a virtual function as `final` prevents it from being overridden in any subclasses. This guarantees that the implementation you define in a derived class remains as it is, safeguarding against future changes that could introduce bugs.
Code Example: Final Virtual Function
class Base {
public:
virtual void show() {}
};
class Derived : public Base {
public:
void show() final { // Cannot be overridden in further derived classes
std::cout << "Derived Class Show" << std::endl;
}
};
// class FurtherDerived : public Derived {
// public:
// void show() override; // Error: cannot override 'show' because it is final
// };
In this case, the `show` method in the `Derived` class is marked as `final`, ensuring that any attempt to override it in a further-derived class will result in a compilation error. This provides a strong contract that the behavior is intentionally locked down.
Why Finalize a Virtual Function?
When you finalize a virtual function, you create a contract that ensures certain behaviors remain intact. This is particularly useful in large codebases where multiple teams may be working on various elements of a project. By clearly marking methods as `final`, you reduce the potential for conflicts and clarifications in class design.
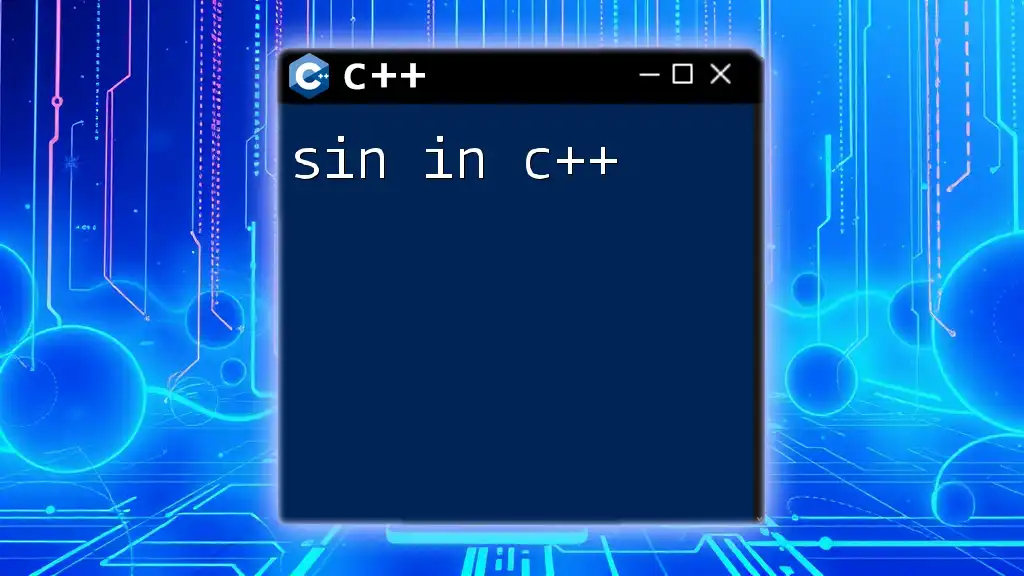
Practical Uses of the `final` Keyword
Common Patterns Utilizing `final`
The `final` keyword can play a significant role in design patterns, such as the Singleton Pattern. In such patterns, restricting inheritance prevents subclasses from altering the singleton behavior, ensuring that there is only one instance of a class for the entire application.
Enhancing Code Readability and Maintainability
Using `final` clarifies your intentions as a developer. When other programmers see a class or method marked as `final`, they instantly understand that it has specific constraints that should not be modified. This greatly enhances code maintainability and readability, allowing your team to work more effectively.
Code Example: Singleton Pattern with Final
class Singleton final {
public:
static Singleton& getInstance() {
static Singleton instance;
return instance;
}
private:
Singleton() {}
};
In this Singleton implementation, declaring the class as `final` emphasizes that its instantiation is strictly controlled and cannot be modified through inheritance.
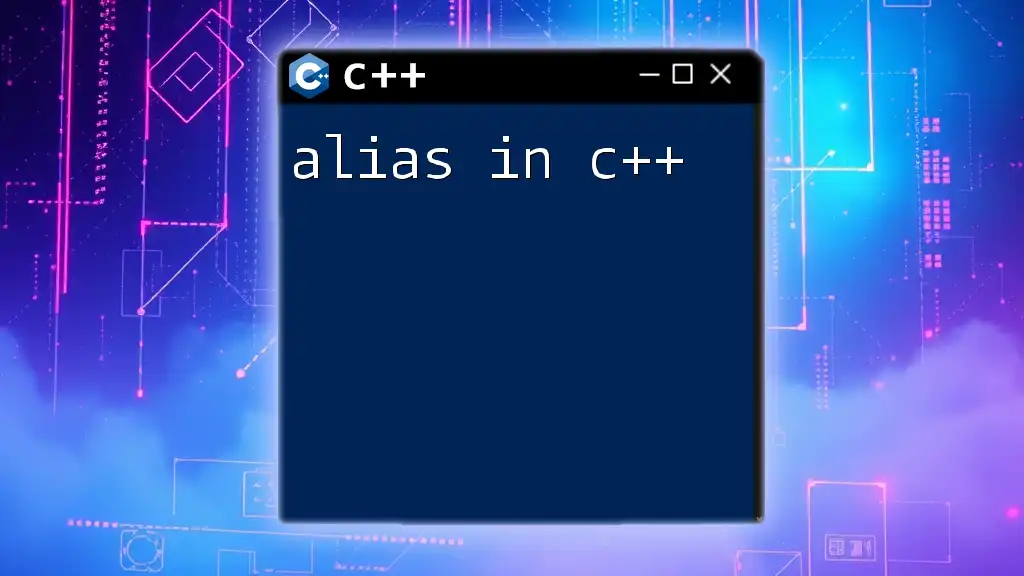
Best Practices for Using the `final` Keyword
When to Declare a Class or Function as Final
When deciding whether to declare a class or method as `final`, consider the following criteria:
- The class or method provides critical functionality that should not be altered.
- You want to enhance performance through compiler optimizations that come from a clear inheritance structure.
- You aim for increased clarity in your code, making it evident that certain classes and methods are not open to extension.
Avoiding Overuse of Final
While the `final` keyword is a useful tool, it can lead to inflexible designs if overused. Excessive restrictions can hinder the ability to extend or adapt your system in the future. Always evaluate each case carefully to prevent creating bottlenecks in your class hierarchy.
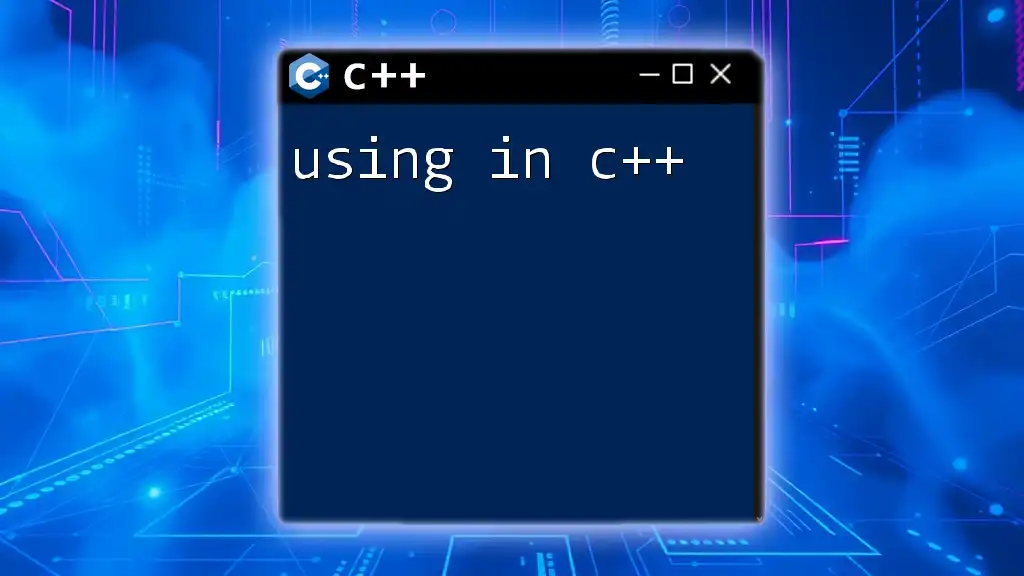
Conclusion
The `final` keyword in C++ is an invaluable feature for controlling class inheritance and method overriding. It promotes safe and clear class design by preventing unintended modifications, enhancing both performance and readability. Applying the `final` keyword judiciously can significantly improve the quality and maintainability of your C++ code.
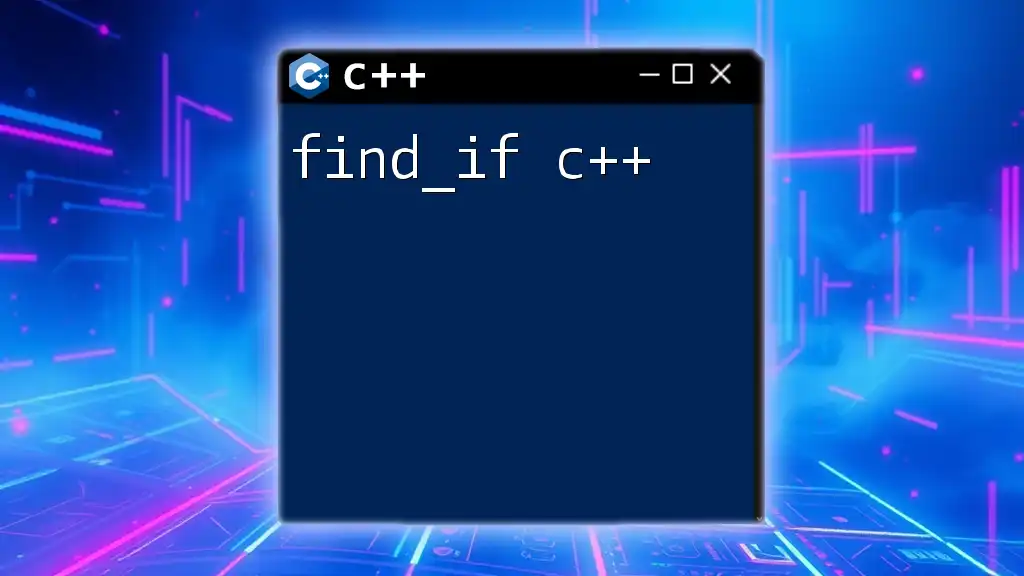
FAQs About the `final` Keyword in C++
What happens if I try to inherit from a final class?
If you attempt to inherit from a class marked as `final`, the compiler will generate an error message stating that inheritance from a final class is not allowed.
Can I use final with templates?
Yes, you can use the `final` keyword with template classes and functions, just as you would with non-template classes. This allows you to control inheritance and overriding behaviors within template-based designs.
Is final part of the C++11 standard and beyond?
Yes, the `final` keyword was introduced in C++11 and continues to be a part of the C++ language in subsequent standards, including C++14, C++17, and C++20. Its use is encouraged for cleaner and safer class designs in modern C++.