In C++, the "if not" logic can be expressed using the `if` statement combined with the logical NOT operator (`!`) to execute a block of code when a certain condition is false.
Here's an example of how to use it:
#include <iostream>
int main() {
bool condition = false;
if (!condition) {
std::cout << "Condition is false!" << std::endl;
}
return 0;
}
Understanding Conditional Statements in C++
Conditional statements are fundamental constructs in C++ programming that enable developers to execute specific code blocks based on certain conditions. They serve the purpose of controlling the flow of a program, allowing for decisions to be made at runtime. The most common types of conditional statements in C++ include:
- Basic `if` statements: Used for simple true/false testing.
- `if-else` and `else if` statements: Allow for multiple conditions to be evaluated.
- Nested `if` statements: Permit complex conditional logic, where an `if` condition is placed within another `if`.
These conditionals are powerful tools that help shape the decisions your programs can make, ensuring efficient control flow.
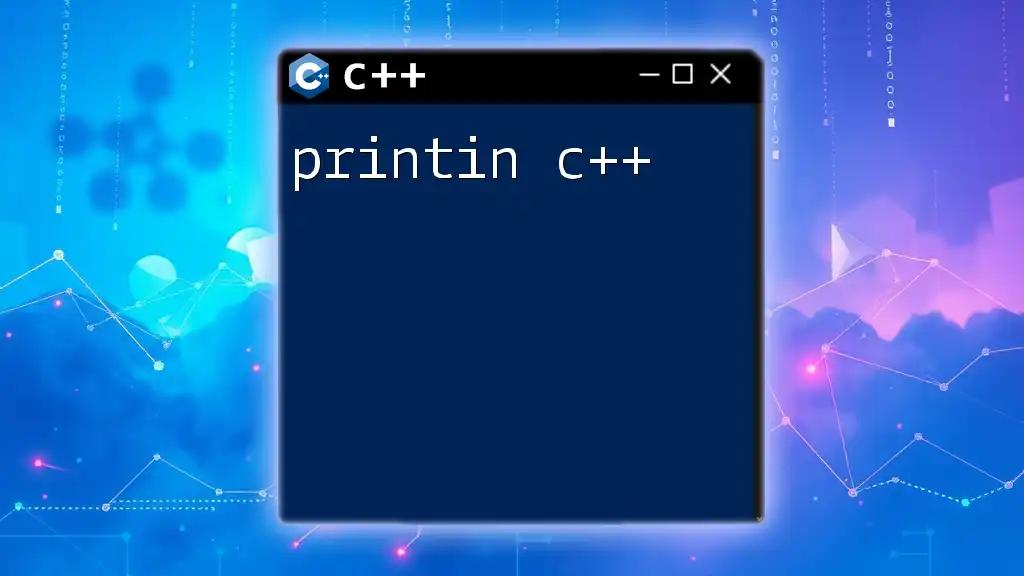
The Concept of "If Not"
In programming, the phrase "if not" introduces the concept of negation. It allows developers to execute code only when a condition is false. Understanding how to effectively use negation enhances your control over flow and logic.
In C++, the logical NOT operator is represented by `!`, which negates a boolean expression. When you encounter "if not," think of it as asking, "What should I do when a condition is false?" Here’s a simple example highlighting this concept:
if (!condition) {
// Execute code if condition is false
}
This structure is practical in many scenarios, such as validating input or checking conditions that influence program behavior.
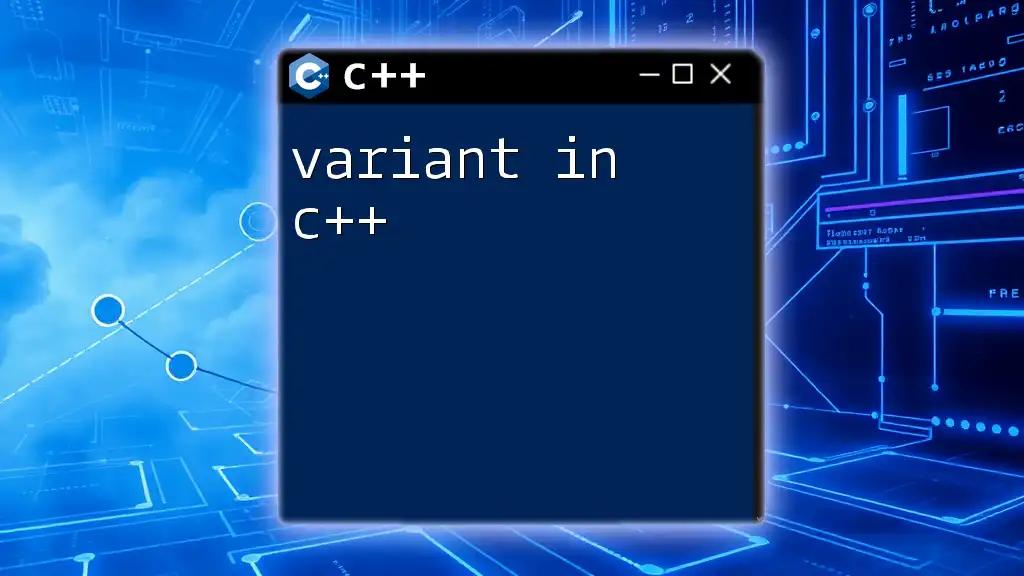
Detailed Example of "If Not" Implementation
To illustrate the usage of "if not in C++," let’s create a detailed example involving user input checking. The goal is to prompt the user for a simple choice and execute different actions based on their response.
#include <iostream>
using namespace std;
int main() {
string userInput;
cout << "Enter 'yes' or 'no': ";
cin >> userInput;
if (userInput != "yes") {
cout << "You did not enter 'yes'." << endl;
} else {
cout << "You entered 'yes'!" << endl;
}
return 0;
}
Explanation
In this example, the program checks the user input against the string "yes." If the input is not "yes" (i.e., the user has entered something else), the first branch of the condition is executed, informing the user of their input. Conversely, if the input matches "yes," the second branch acknowledges the correct input.
Notably, using `!=` (not equal) achieves the same logical result as checking for negation (`!`) directly. Both approaches are valid, but employing `!=` can improve readability, ensuring clarity in intention.

Alternatives to "If Not" Logic
Inverting Conditions for Clarity
When leveraging the concept of negation, you may encounter scenarios where it’s better to structure your logic to enhance readability. Instead of writing a condition in a way that involves negation, consider rephrasing it positively. For instance, rather than using:
if (!isEmpty) {
// Action when not empty
}
You may rephrase it as:
if (hasItems) {
// Action when there are items
}
This method prevents potential confusion and improves the overall clarity of your code.
Using `switch` Statements as an Alternative
In some cases, a `switch` statement may be a more efficient alternative to a series of `if-else` statements. When dealing with specific known values, `switch` can streamline your control logic. It is particularly useful for discrete value checks and can lead to clearer code. Here is an example of a simple `switch` statement:
switch (option) {
case 1:
cout << "Option 1 selected." << endl;
break;
case 2:
cout << "Option 2 selected." << endl;
break;
default:
cout << "Invalid option selected." << endl;
break;
}
Using `switch` can simplify decision making when many possible conditions are evaluated against a single variable.

Common Mistakes with "If Not"
Even seasoned developers can stumble over the subtleties of using negation within conditionals. Here are some common pitfalls to watch for:
- Logical errors: Misusing the logical operators can produce unintended outcomes. Always double-check your conditions.
- Confusion between `==` and `!=`: These are easy to misinterpret, particularly in complex expressions. Pay attention to your comparisons.
To avoid these mistakes, practice clear variable naming and utilize comments in critical areas of your code. This enhances readability and can help catch errors early in the coding process.

Best Practices for Using "If Not" in C++
As you incorporate "if not in C++" into your programming repertoire, considering best practices can tremendously impact the quality and maintainability of your code. Here are essential principles to follow:
- Structure your conditionals thoughtfully: Group related conditions together and avoid deeply nested structures where possible.
- Aim for clean code: Make clarity a priority. Use descriptive variable names and comment non-obvious logic to prevent confusion.
- Regularly test your logic: Employ unit tests and code reviews to ensure your conditional statements behave as expected.

Conclusion
Using "if not in C++" strategically allows you to control program logic effectively. The clarity and precision that come with mastering negation and conditional statements can greatly enhance your programming skills. Emphasize practicing these concepts to ensure their effective use in your own coding projects.

Additional Resources
To deepen your understanding of C++ conditionals and best practices, consider consulting official C++ documentation, recommended books on programming, and engaging in community forums. These resources will further assist you as you grow in your knowledge and application of C++.
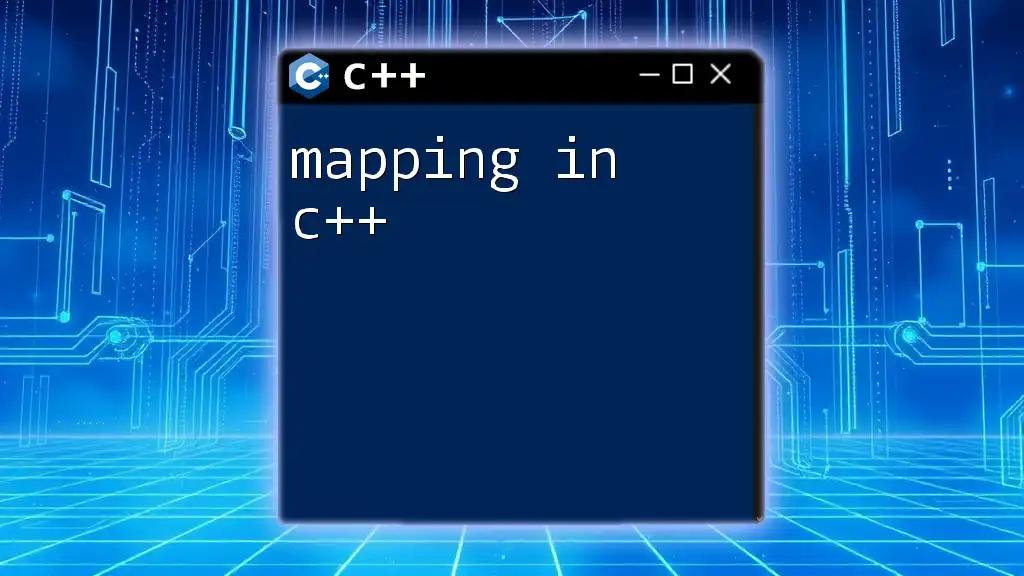
Call to Action
Share your own experiences with "if not in C++" in your programming journey. If you found this information valuable, consider subscribing for more insightful tips and tutorials designed to enhance your understanding of C++ and its command structures. Happy coding!