The `cin.ignore()` function in C++ is used to ignore or discard a specified number of characters from the input buffer, typically to clear out unwanted newline characters left after reading an input.
Here’s a simple example:
#include <iostream>
using namespace std;
int main() {
int number;
cout << "Enter a number: ";
cin >> number;
cin.ignore(); // Ignore the newline character left in the buffer
string name;
cout << "Enter your name: ";
getline(cin, name); // Read a full line of input, including spaces
cout << "You entered: " << number << " and your name is " << name << endl;
return 0;
}
What is cin.ignore?
In C++, `cin.ignore()` is a member function of the `istream` class and is primarily used to skip characters in an input stream. Its main purpose is to handle unintended characters left in the input buffer, especially after reading different types of inputs where the newline character (`'\n'`) can cause issues.

How cin.ignore Works
Function Signature
The function signature for `cin.ignore()` is as follows:
istream& ignore(streamsize n = 1, int delim = EOF);
- Parameters:
- n: This parameter specifies the maximum number of characters to ignore. By default, it is set to 1.
- delim: This defines a delimiter. The function will stop ignoring characters if this delimiter is encountered. By default, it is set to `EOF`.
Important Note: The use of default parameters allows flexibility in how `cin.ignore()` is invoked. If you only want to ignore a single character, `cin.ignore()` can be called with no arguments.
Buffer Handling
Understanding the input buffer is crucial to grasp how `cin.ignore()` functions. When you input data from the user, it gets stored in a buffer. After reading a value (for example, an integer), the newline character generated by pressing "Enter" remains in the buffer. If you later attempt to read a string using `getline()`, it might capture this leftover newline character instead of waiting for new input. This is where `cin.ignore()` comes to the rescue by clearing out the unwanted characters.
![Understanding Unsigned Int in C++ [Quick Guide]](/_next/image?url=%2Fimages%2Fposts%2Fu%2Funsigned-int-cpp.webp&w=1080&q=75)
When to Use cin.ignore
Input Scenarios: The most common scenario requiring `cin.ignore()` occurs after reading a number when you subsequently want to read a string. Without calling `cin.ignore()`, the input stream may still hold the newline character, leading to unexpected behavior.
Practical Examples
Example 1: Ignoring newline after numeric input
In the following example, we read an integer and subsequently read a string. Without `cin.ignore()`, the program would not wait for a new input for the string:
#include <iostream>
using namespace std;
int main() {
int age;
cout << "Enter your age: ";
cin >> age;
cin.ignore(); // Clears the newline character from input buffer
string name;
cout << "Enter your name: ";
getline(cin, name);
cout << "Name: " << name << ", Age: " << age << endl;
return 0;
}
Explanation: After reading the integer `age`, the newline character that is left in the buffer would interfere with the subsequent call to `getline()`. By calling `cin.ignore()`, we clear that character away, allowing `getline()` to function correctly.
Example 2: Ignoring additional characters
In this example, we'll demonstrate how `cin.ignore()` can be decisive when multiple inputs are taken in sequence:
#include <iostream>
using namespace std;
int main() {
char letter;
cout << "Enter a character: ";
cin >> letter;
cin.ignore(); // Ignores the newline left in buffer
cout << "You entered: " << letter << endl;
return 0;
}
Explanation: Users might accidentally add a space or another character after inputting the letter. `cin.ignore()` ensures that we handle that scenario correctly.
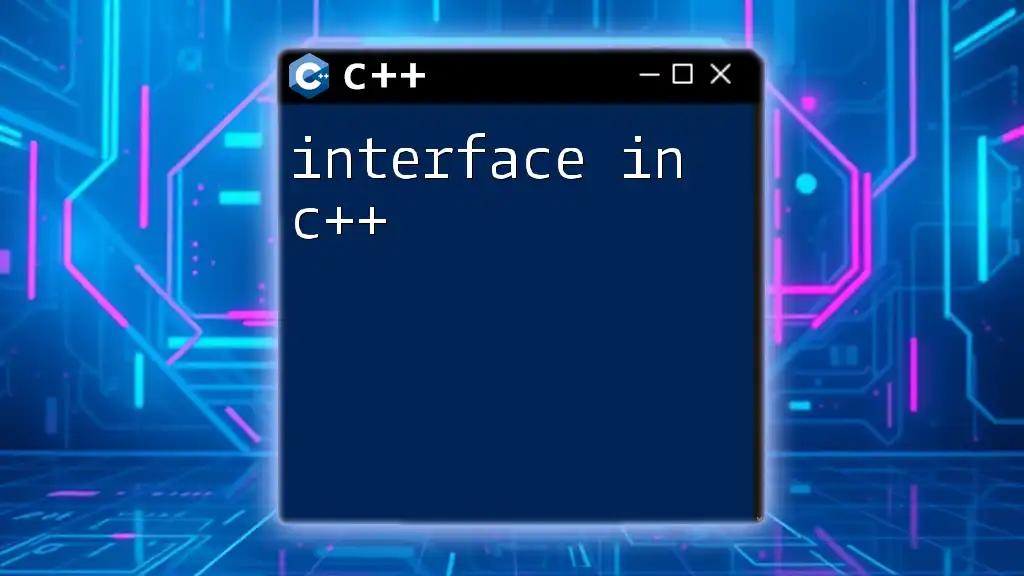
Common Mistakes with cin.ignore
Despite its usefulness, `cin.ignore()` can lead to confusion, particularly if developers do not grasp when to employ it.
Common Mistakes:
- Failing to use `cin.ignore()` after non-string input: This often results in unintentional input capturing.
- Neglecting the effect of the `delim` parameter: Not understanding how the delimiter can alter its behavior might lead to overlooking important input characters.
Debugging Tips
If you encounter issues related to unexpected behavior with input capturing, consider the following debugging tips:
- Check your input statements: Confirm you use the correct data types and that your sequence of `cin.ignore()` calls aligns with your intentions.
- Print the buffer state: This may help to visualize what characters are left before and after invoking `cin.ignore()`.
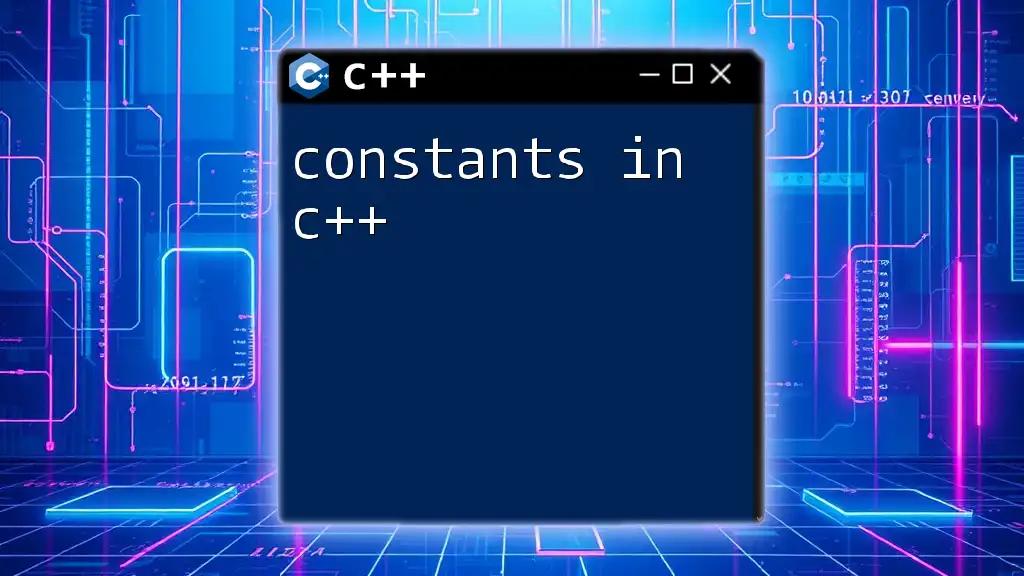
Best Practices for Using cin.ignore
To maximize the effectiveness of `cin.ignore()`, adhere to these best practices:
- Use it whenever switching input types: Always call `cin.ignore()` after reading inputs that may leave undesired characters in the buffer.
- Decide on a limit: Be deliberate with the parameters you choose for `cin.ignore()`, especially the delimiting character when your context requires precision.
- Consistent documentation: Comment your use of `cin.ignore()` within your code to avoid confusion for others reading it.
Summary of Best Practices
Being mindful of input handling in C++ is paramount. Regularly using `cin.ignore()` can help you avoid traps and take control of how your program interacts with user input. The benefits far outweigh the practice of leaving it out.
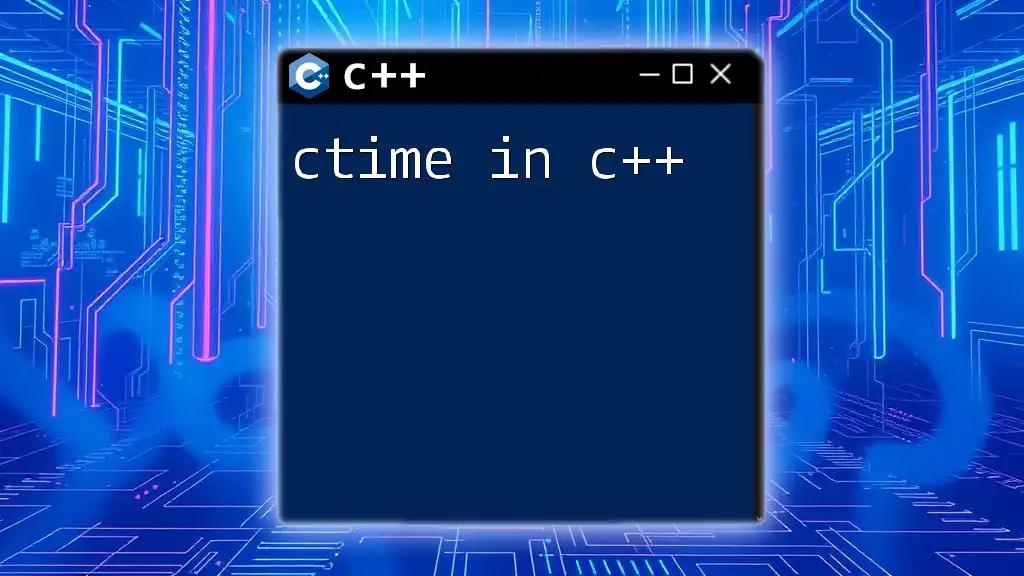
Conclusion
Understanding `cin.ignore` in C++ allows for better input handling and more robust programs. Grasping its principles promotes cleaner interaction with users and fewer unexpected behaviors. Practice consistently with different input scenarios to become proficient in using `cin.ignore()`, and remember that effective input handling is part of writing high-quality C++ code.
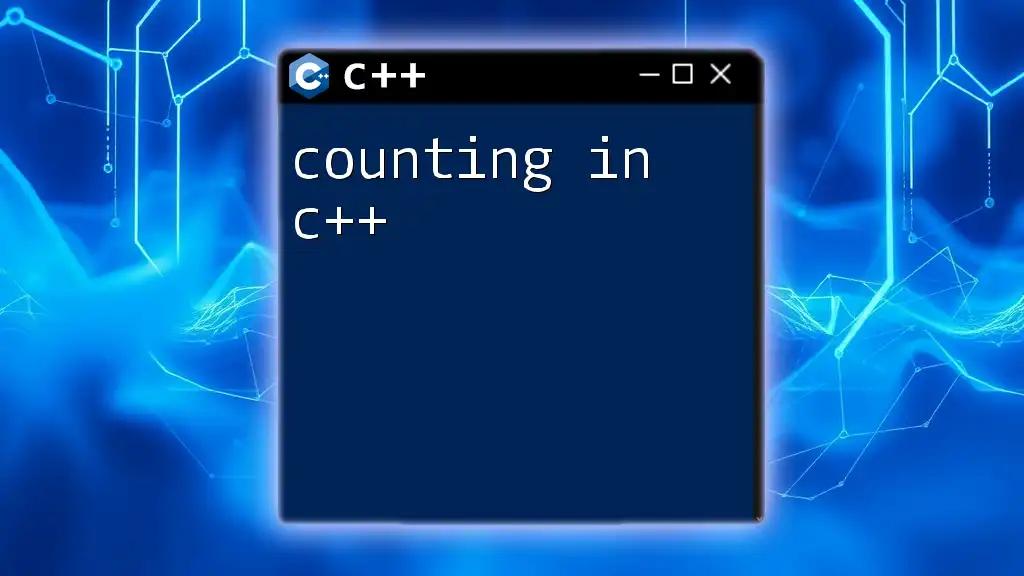
Further Reading and Resources
For a deeper understanding and additional resources, consider exploring:
- The C++ Standard Library documentation
- Online C++ tutorials tailored to input handling
- Recommended books on C++ programming that cover input and output in detail
By integrating these practices into your programming repertoire, you'll find yourself well-equipped to handle user input effectively in C++.