In C++, the `end` function is typically used with iterators to signify the end of a container, such as a vector or list, and it returns an iterator pointing to the position just past the last element of the container.
Here's a code snippet demonstrating its use with a vector:
#include <iostream>
#include <vector>
int main() {
std::vector<int> vec = {1, 2, 3, 4, 5};
for (auto it = vec.begin(); it != vec.end(); ++it) {
std::cout << *it << " ";
}
return 0;
}
Understanding Iterators
What are Iterators?
Iterators are a fundamental concept in C++ that provide a unified method to access elements of various data structures (like arrays, lists, or maps) without having to understand the specifics of their underlying implementations. They act like pointers that allow you to traverse the elements sequentially. Understanding iterators is crucial for working with the Standard Template Library (STL), which extensively utilizes them.
Types of Iterators
C++ provides several types of iterators:
- Input Iterators: Allow reading elements from a container in a single pass.
- Output Iterators: Allow writing elements to a container in a single pass.
- Forward Iterators: Allow traversal in one direction and can be read multiple times.
- Bidirectional Iterators: Allow traversal in both forward and backward directions.
- Random Access Iterators: Provide direct access to any element within a container, similar to pointers.
Each type of iterator has specific use cases and understanding when to use each is vital for optimal and efficient programming.
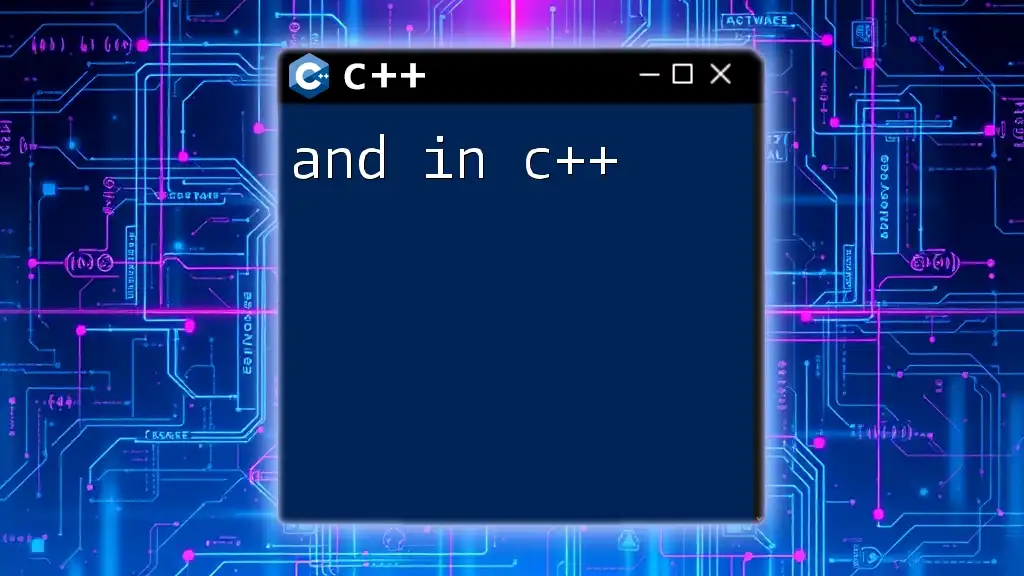
The `end` Function
What is `end`?
The `end()` function is an important function in C++ that returns an iterator pointing to one element past the last element of a container. This is essential for iteration processes since it signifies the end of the container. Unlike the last element, which can be accessed using `end() - 1`, the iterator returned by `end()` itself cannot be dereferenced.
Syntax of `end()`
ContainerName::iterator end();
This syntax indicates that `end()` is a member function of the container class, returning an iterator that points to the end of the container. Different containers can return iterators of various types, so it's crucial to know what you're working with.
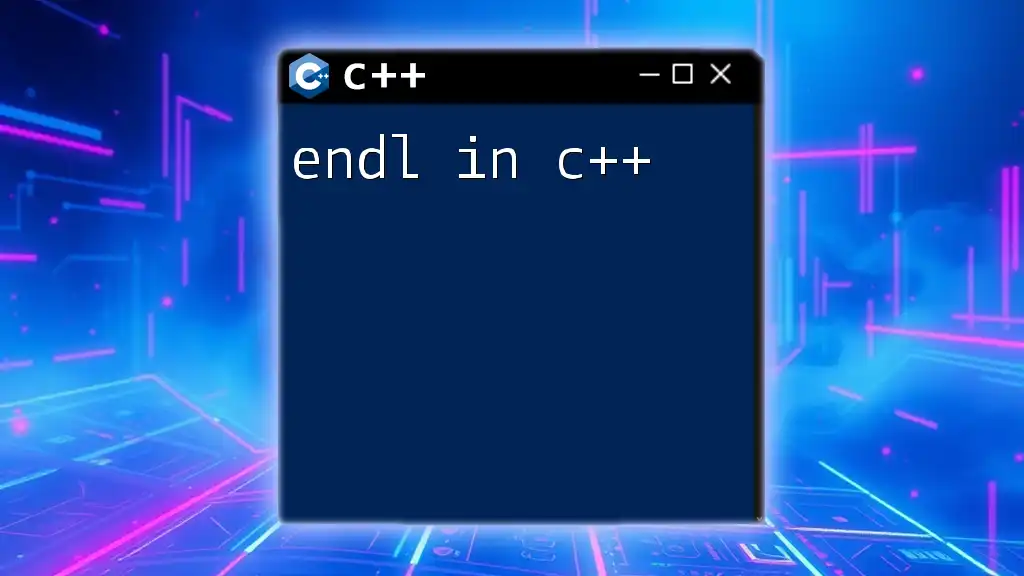
How `end` Works in Different Containers
Using `end` with Vectors
When utilizing `end()` with vectors, it serves as a way to navigate through the data structure conveniently.
Example of Using `end` with Vectors
#include <iostream>
#include <vector>
int main() {
std::vector<int> numbers = {1, 2, 3, 4, 5};
for (auto it = numbers.begin(); it != numbers.end(); ++it) {
std::cout << *it << " ";
}
return 0;
}
In this example, the program initializes a vector with five integers. The `for` loop employs `begin()` to start from the first element and utilizes `end()` to identify when to stop looping. Each iteration prints the dereferenced iterator.
Using `end` with Lists
Lists in C++ offer a similar yet nuanced approach to using `end()`.
Example of Using `end` with Lists
#include <iostream>
#include <list>
int main() {
std::list<std::string> fruits = {"Apple", "Banana", "Cherry"};
for (auto it = fruits.begin(); it != fruits.end(); ++it) {
std::cout << *it << " ";
}
return 0;
}
Here, we create a list of strings. The traversal mechanism using `begin()` and `end()` remains the same, showcasing the versatility of the `end` function across different containers.
Using `end` with Other STL Containers
Maps and Sets
When dealing with associative containers like `std::map` and `std::set`, `end()` behaves similarly but offers additional features such as pair access in maps.
Example Code Snippet
#include <iostream>
#include <map>
int main() {
std::map<std::string, int> ages = {{"Alice", 30}, {"Bob", 25}};
for (auto it = ages.begin(); it != ages.end(); ++it) {
std::cout << it->first << ": " << it->second << "\n";
}
return 0;
}
This example iterates over a map, showcasing how we can access both keys and values using the iterator returned by `begin()` and `end()`.
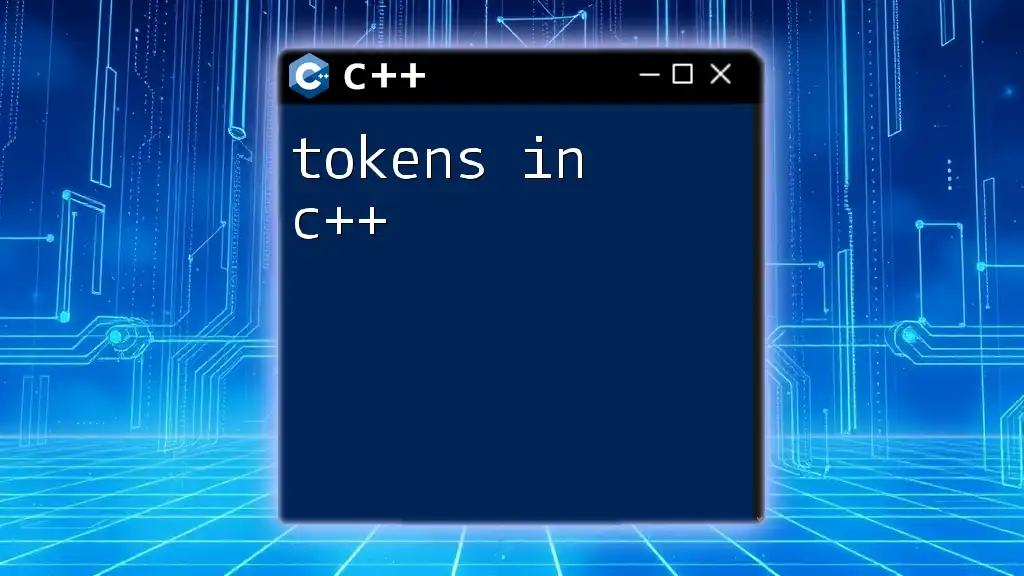
Differences Between `end` and `end()`
`end()` vs. `end`
It's essential to distinguish between `end()` as a function call and the term "end" as it might appear in other contexts. In C++, `end()` is always a call to return an iterator, while "end" may be a generic term. Misunderstanding this distinction can lead to confusion, especially when reading through documentation or code.
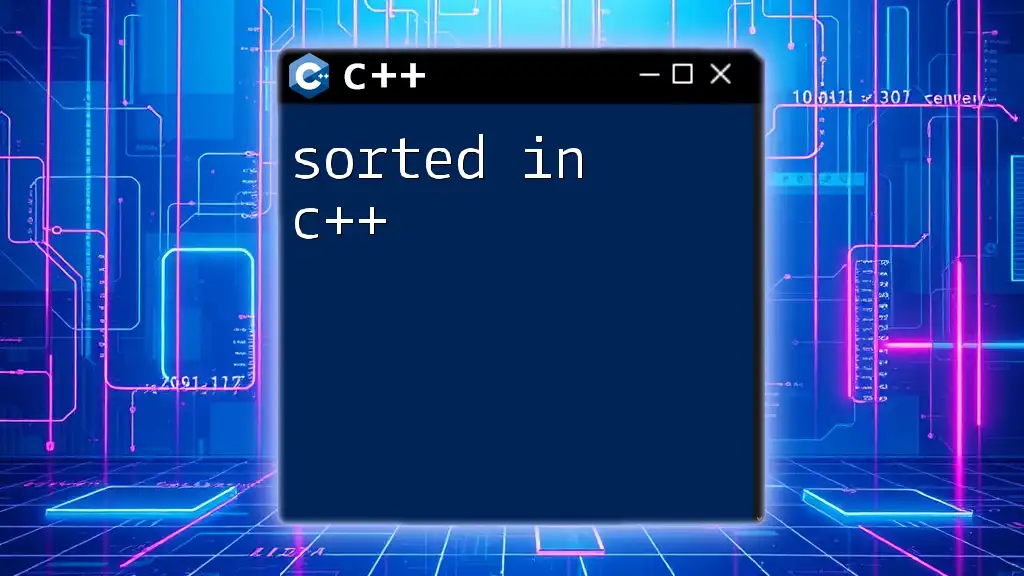
Common Mistakes with `end`
Off-By-One Errors
A common mistake developers encounter when using `end()` arises from off-by-one errors during loops. When iterating, users may incorrectly assume that `end()` returns the last element instead of one past the last. This could lead to dereferencing an invalid iterator.
Using `end` with Const Iterators
In some situations, you might want to avoid modifying the elements of the container. Using `const_iterator` with `end()` is an effective way to enforce this.
Code Snippet Examples
#include <iostream>
#include <vector>
int main() {
const std::vector<int> numbers = {1, 2, 3};
for (auto it = numbers.cbegin(); it != numbers.cend(); ++it) {
std::cout << *it << " ";
}
return 0;
}
In this example, `cbegin()` and `cend()` return iterators that do not allow modifications to the vector's content, thus enabling safer access.
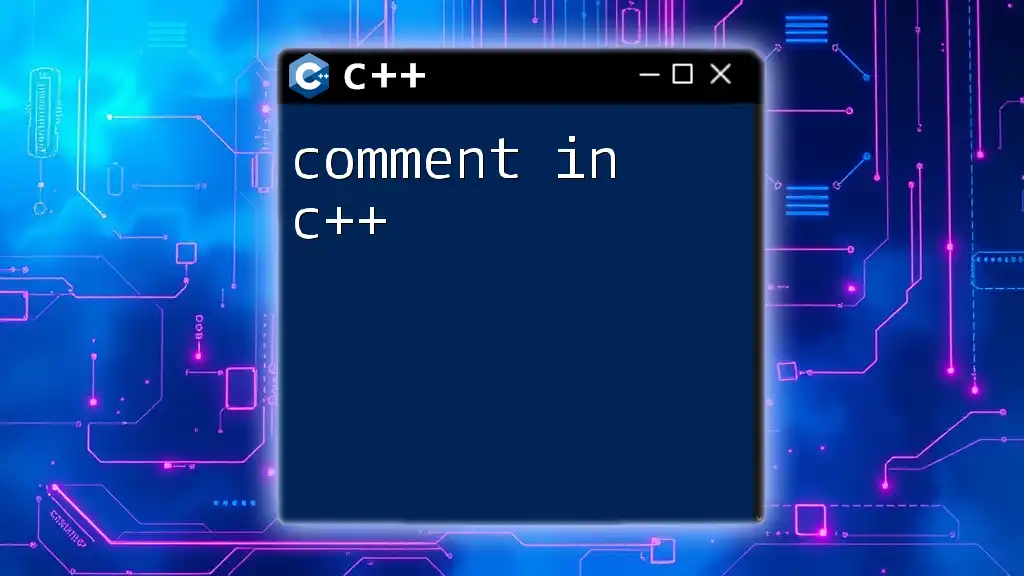
Practical Use Cases
Iterating Over a Container
Effectively using `end()` can greatly simplify tasks where traversal through a container is necessary. By combining it with STL functions, programming logic, or algorithms can become seamless.
Combining `end` with Other Algorithms
One of the best aspects of `end()` is how it integrates with STL algorithms. Since these algorithms often require iterators as parameters, using `end()` provides a clear boundary of where the operation finishes.
Code Snippet Example
#include <algorithm>
#include <vector>
#include <iostream>
int main() {
std::vector<int> numbers = {5, 3, 1, 4, 2};
std::sort(numbers.begin(), numbers.end());
for (auto it : numbers) {
std::cout << it << " ";
}
return 0;
}
This code sorts the vector using `end()` to determine the extent of the sorting operation. The output confirms that the original vector is correctly sorted.
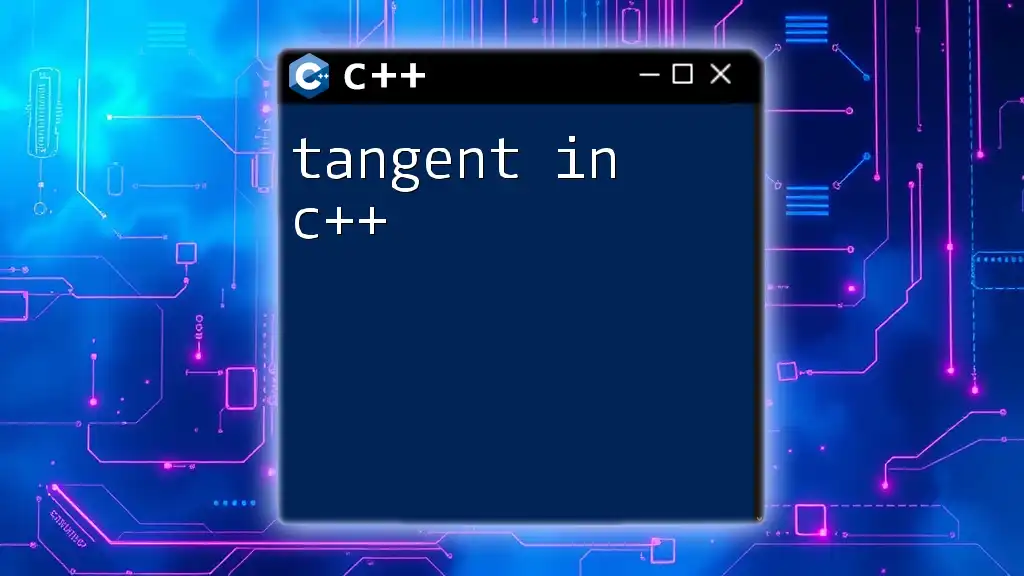
Conclusion
The `end` function in C++ is a critical tool for navigating containers within the STL. Understanding how to utilize `end()` effectively is pivotal for proper iteration and ensuring your code is efficient and error-free. As you practice with different containers and see how `end()` interacts with various features, your proficiency will enhance, solidifying your capability in C++ programming. Embrace the learning journey, and explore additional resources to continue expanding your knowledge of C++ STL!