The `lower_bound` function in C++ finds the first position in a sorted range where a specified value can be inserted without violating the order.
Here's a code snippet to illustrate its usage:
#include <iostream>
#include <vector>
#include <algorithm>
int main() {
std::vector<int> v = {1, 2, 4, 4, 5};
int key = 4;
auto it = std::lower_bound(v.begin(), v.end(), key);
std::cout << "The lower bound of " << key << " is at position: " << (it - v.begin()) << std::endl;
return 0;
}
Understanding the Basics of lower_bound in C++
What is lower_bound?
The lower_bound function in C++ is a part of the C++ Standard Library and is essential for performing efficient searches in sorted ranges. Its primary purpose is to find the first position in a sorted container where a specified value can be inserted without violating the order of the container. This makes it especially useful for operations on sorted data structures.
Key Characteristics of lower_bound
The lower_bound function has some key characteristics that differentiate it from other searching algorithms:
- It returns an iterator pointing to the first element not less than the specified value.
- If all elements are less than the specified value, it returns an iterator to the end of the range.
- The function is designed to work on sorted ranges, and it assumes that the elements are ordered according to the specified criteria.
- It operates in O(log n) time complexity, making it efficient for large datasets.
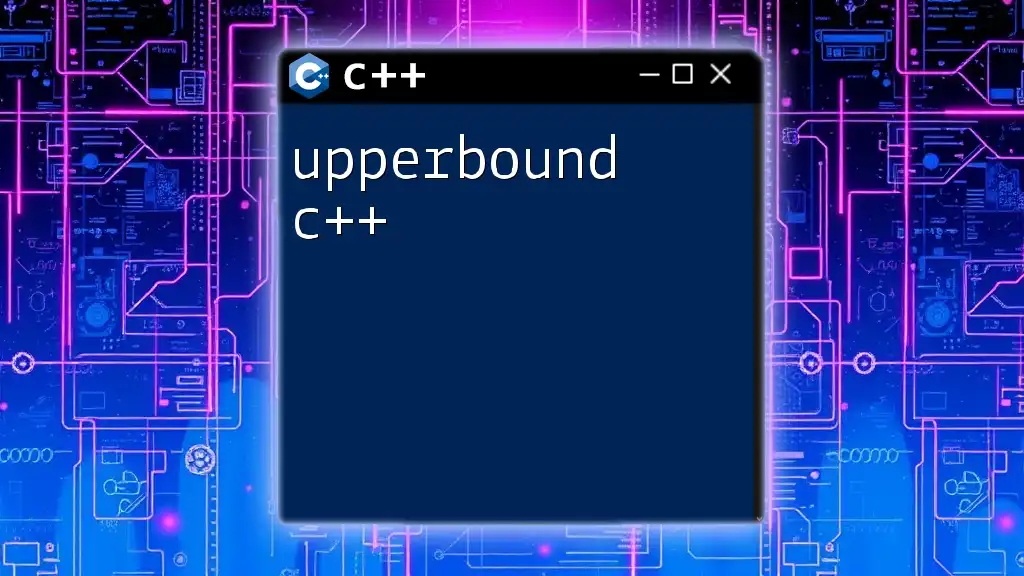
The Syntax of lower_bound in C++
Function Signature
The syntax for using lower_bound is as follows:
iterator lower_bound(iterator first, iterator last, const T& value);
iterator lower_bound(iterator first, iterator last, const T& value, Compare comp);
Parsing this function signature requires an understanding of its parameters. first indicates the starting point of the search, last marks the endpoint (exclusive), and value is the element to search for within the range. You can also specify a custom comparison function with the comp parameter for more advanced searches.
Parameters Explained
- first: Iterator pointing to the beginning of the range.
- last: Iterator pointing to the end of the range (exclusive).
- value: The value you are searching for.
- comp: (optional) A custom comparison function that you can define for tailored search conditions.
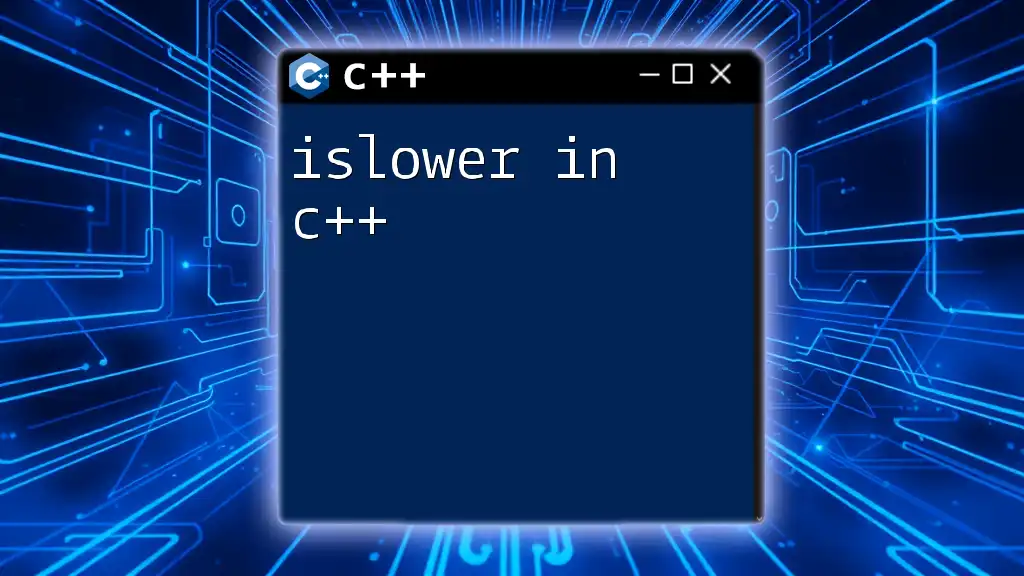
How lower_bound Works
Searching in Sorted Containers
The lower_bound function operates under the assumption that the range it searches is sorted. When you invoke this function, it looks for the lowest index at which the target value could be inserted while maintaining the order. This binary search mechanism efficiently narrows down the search space, ensuring optimal performance.
Example: Finding a Lower Bound
Here’s an example demonstrating the use of lower_bound in a simple scenario:
#include <iostream>
#include <vector>
#include <algorithm>
int main() {
std::vector<int> arr = {1, 2, 4, 4, 5, 6};
auto it = std::lower_bound(arr.begin(), arr.end(), 4);
std::cout << "The lower bound of 4 is at index: " << (it - arr.begin()) << '\n';
}
In this example, the vector `arr` contains several integers sorted in ascending order. We use lower_bound to find the first occurrence of the value `4`. The returned iterator points to the first `4` in the vector, and the output will display the index of that position. Importantly, it allows for efficient searches, even in larger datasets.
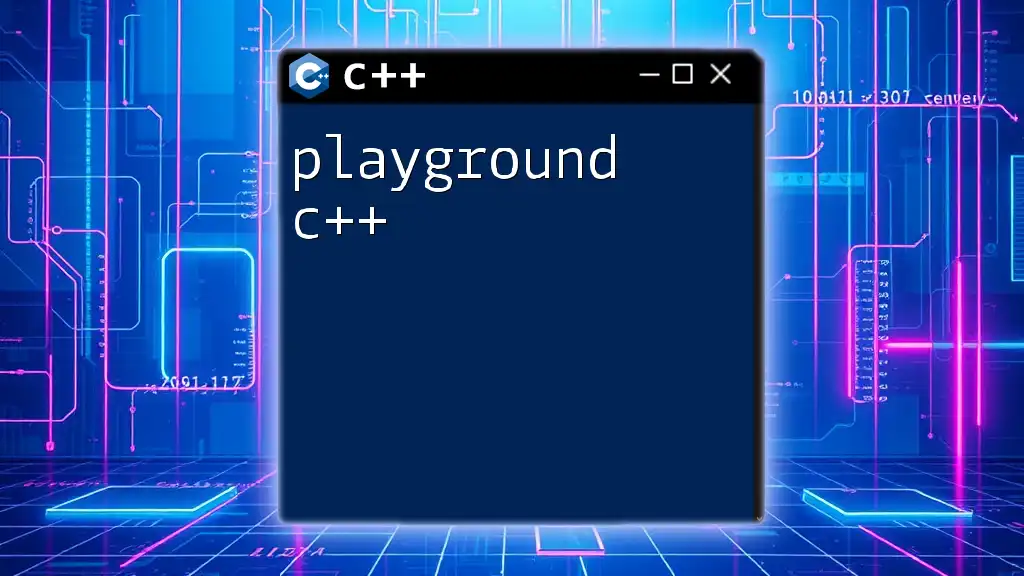
Practical Applications of lower_bound in C++
Use Cases in Algorithms
lower_bound is commonly utilized in several algorithmic scenarios:
- Binary Search Applications: It is often used in implementing binary search algorithms due to its efficiency.
- Insertion Operations in Sorted Data: Before inserting a new value into a sorted container, you can find the proper index using lower_bound to maintain the order.
Lower Bound in Custom Comparison Scenarios
You can harness the power of lower_bound with custom data structures by defining a comparison function. For example:
struct Item {
int value;
// other fields
};
// Custom comparator
bool compare(const Item& a, const Item& b) {
return a.value < b.value;
}
// Usage
auto it = std::lower_bound(items.begin(), items.end(), targetItem, compare);
This example allows you to search for an `Item` based on its `value`, making your usage of lower_bound versatile and adaptable to various data types.
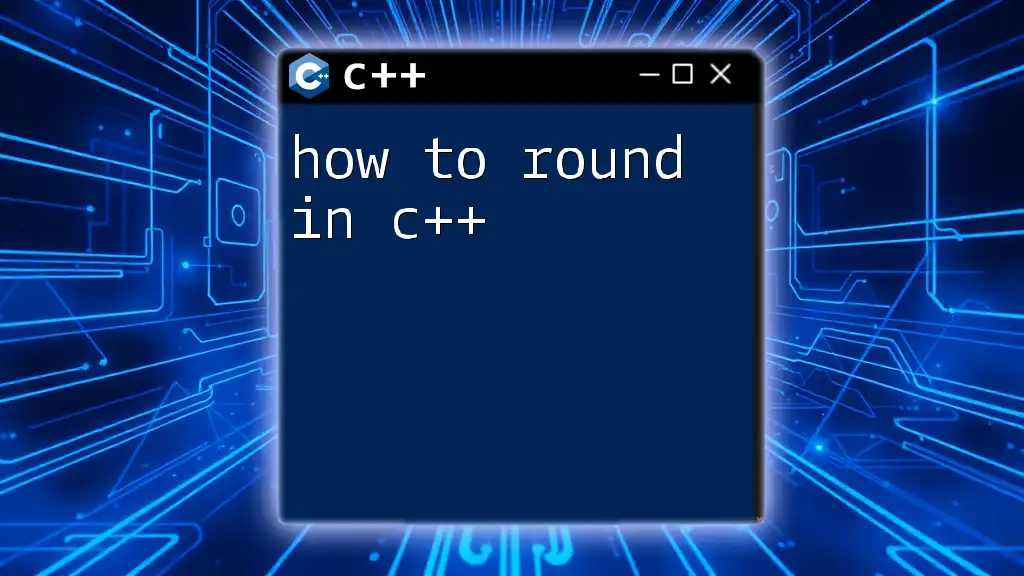
Comparative Analysis
lower_bound vs upper_bound
While lower_bound finds the first occurrence where a value could be inserted, upper_bound identifies the next position past a specified value. This distinction helps in finding ranges of elements quickly, allowing you to choose the appropriate function based on your needs.
lower_bound vs find
The find function, in contrast, searches for an exact match rather than a potential insertion point. While find runs in O(n) time, lower_bound maintains a much faster O(log n) time complexity, making it a better option for sorted containers where performance is critical.
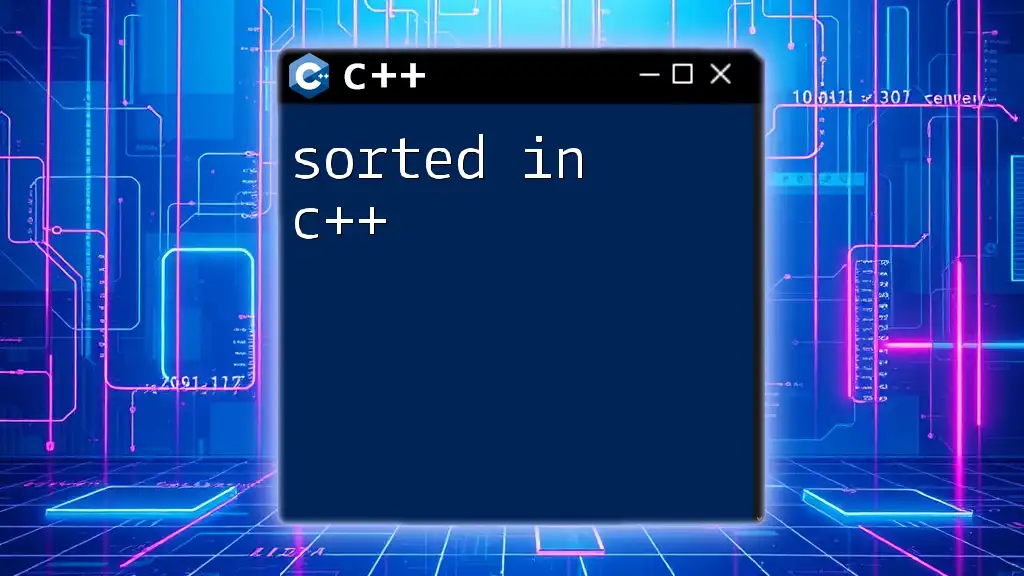
Common Mistakes to Avoid
Misusing lower_bound
One of the most frequent errors made when using lower_bound is applying it to an unsorted range. This will lead to undefined behavior and incorrect results. Always ensure that the target range is sorted before invoking this function.
Another common pitfall is not handling iterators properly. If the iterator returned indicates a position where the target value does not exist, it is crucial to check against the end iterator to avoid dereferencing a potentially invalid memory location.
Debugging Issues
When using lower_bound, common issues may arise related to incorrect assumptions about the data ordering. Ensuring that your input data is sorted and implementing error checking can aid in resolving such issues. Always validate your results against expected outcomes to catch these bugs early.
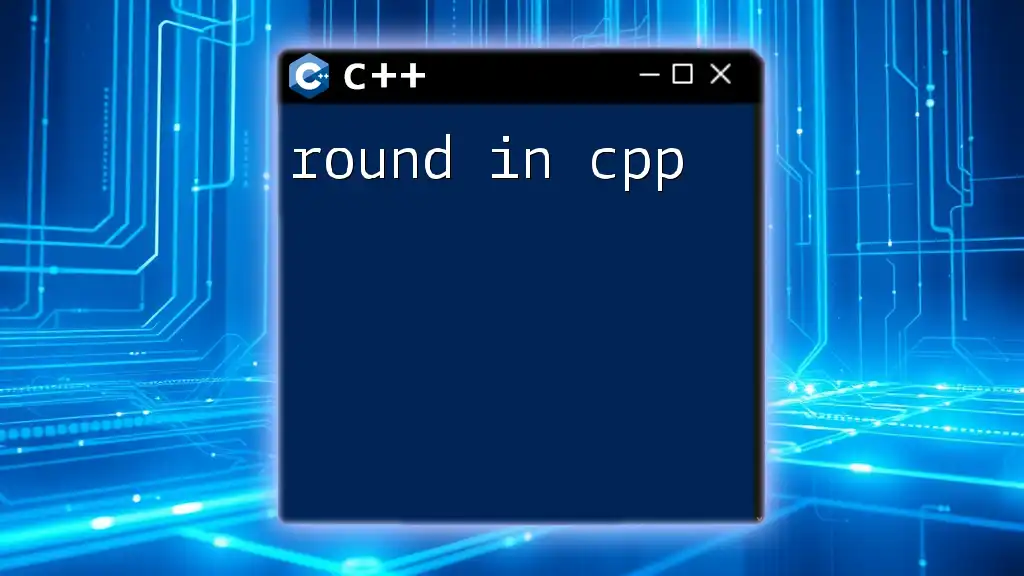
Conclusion
In summary, understanding the functionality and applications of lower_bound in C++ is vital for efficient programming. This function not only optimizes search operations in sorted datasets but also empowers you to implement more effective algorithms. By practicing and integrating this essential function into your coding toolkit, you will find yourself equipped to tackle a variety of programming challenges with ease.
![Understanding Literals in C++ [A Quick Guide]](/images/posts/l/literals-in-cpp.webp)
Further Reading and Resources
For those keen on deepening their understanding of C++ and enhancing their programming skills, consider exploring the following resources:
- Official C++ documentation: The go-to source for comprehensive information on the Standard Library.
- Tutorials and coding challenges: Websites such as LeetCode or HackerRank offer excellent platforms for hands-on practice.
- Books and courses: Seek out literature and instruction specifically focusing on C++ to build a solid foundation in this powerful language.
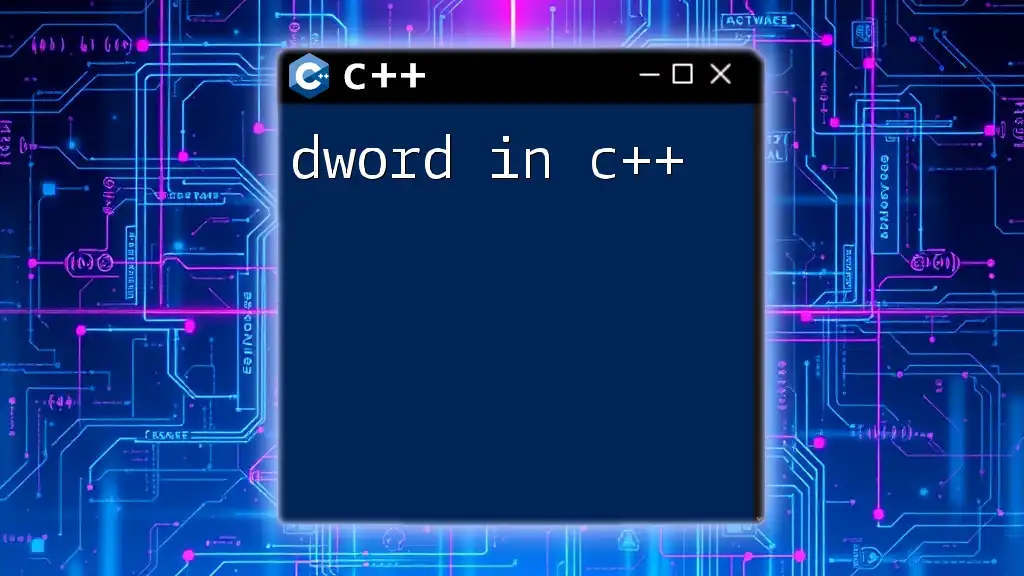
Call to Action
We encourage readers to engage with the content—leave any questions in the comments, experiment with lower_bound in your personal projects, and subscribe for more insightful C++ tips and guides. Happy coding!