In C++, you can sort a collection of elements using the `std::sort` algorithm from the `<algorithm>` header, which rearranges the elements into ascending order.
#include <iostream>
#include <algorithm>
#include <vector>
int main() {
std::vector<int> nums = {5, 1, 4, 2, 3};
std::sort(nums.begin(), nums.end());
for(int num : nums) {
std::cout << num << " ";
}
return 0;
}
What Does "Sorted" Mean in C++?
In the realm of programming, sorted data refers to an arrangement of elements based on a defined order—typically ascending or descending. In C++, having sorted data is crucial because it allows algorithms to operate more efficiently, leading to improved performance in search operations, and data management. For instance, consider an array of integers: if it’s sorted, finding a particular number becomes significantly faster, as you can apply binary search algorithms rather than scanning through each element linearly.
To contrast, an unsorted data set might look like this: `[5, 2, 8, 1, 3]`. In contrast, a sorted array would be arranged as `[1, 2, 3, 5, 8]`. The ability to quickly identify and retrieve data points makes sorting an essential operation in many applications, from databases to graphical applications.
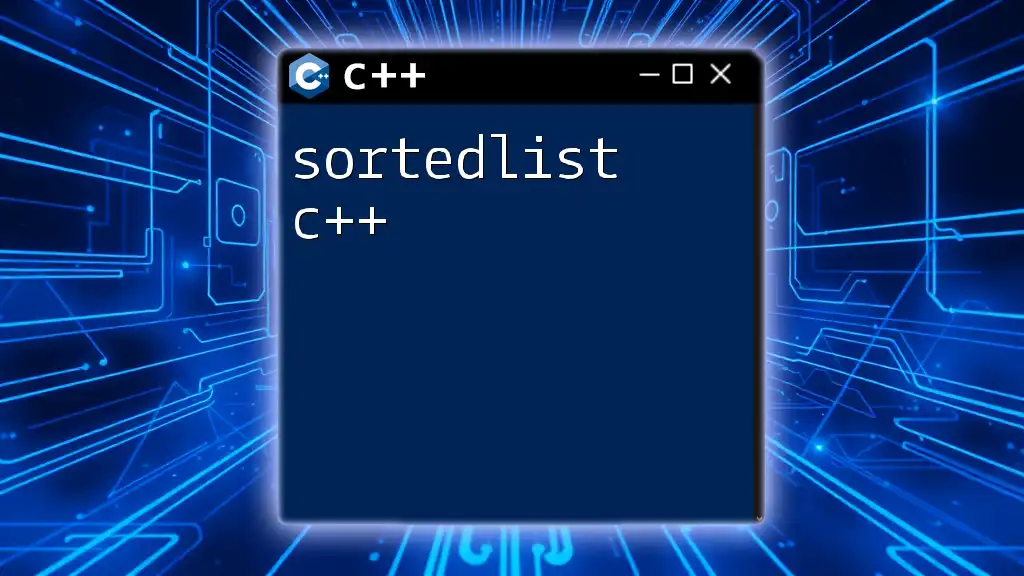
The Sort Function in C++
What is `std::sort`?
`std::sort` is a powerful function provided by the C++ Standard Library, specifically within the `<algorithm>` header. Utilized for sorting collections of elements, it primarily implements a hybrid sorting algorithm called IntroSort, which combines QuickSort, HeapSort, and InsertionSort to deliver an efficient sorting process. The beauty of `std::sort` lies not just in its efficiency but also in its simplicity of use.
Syntax of `std::sort`
The general syntax for `std::sort` is as follows:
template <class RandomIt>
void sort(RandomIt first, RandomIt last);
Here, `first` and `last` denote iterators that define the range of the container you would like to sort, effectively sorting from `first` to `last`—excluding `last`.
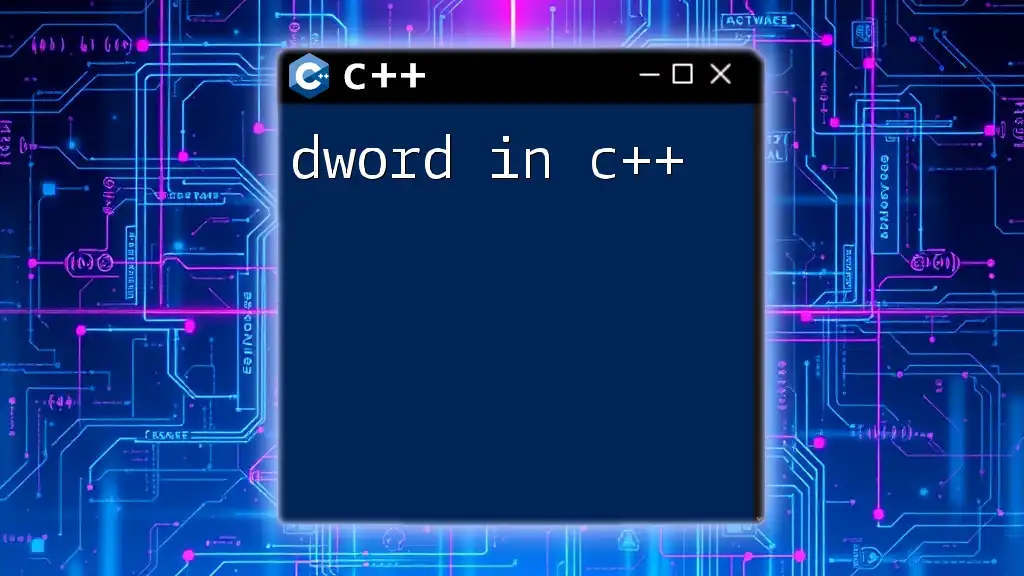
How to Use `std::sort`
Step-by-Step Tutorial
-
Include Required Headers Before utilizing the sort function, you need to include the necessary header:
#include <algorithm>
-
Create a Sample Dataset For demonstration, let’s begin with a vector of integers:
#include <iostream> #include <vector> #include <algorithm> int main() { std::vector<int> numbers = {5, 2, 8, 1, 3}; // Sorting will occur here }
-
Call `std::sort` Here’s how to apply the sort function directly on the dataset:
std::sort(numbers.begin(), numbers.end());
-
Display Sorted Data Finally, you can easily print the sorted results using a loop:
for (int num : numbers) { std::cout << num << " "; } // Output: 1 2 3 5 8
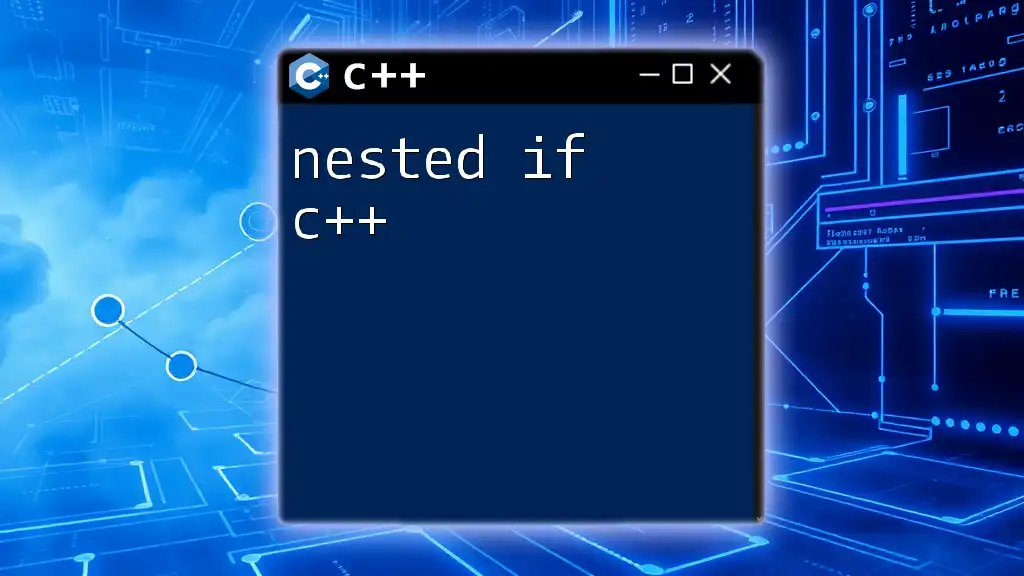
Different Ways to Sort in C++
Sorting with Custom Comparison Functions
Using Comparators
While `std::sort` defaults to sorting in ascending order, you may want to sort in descending order or based on custom conditions using comparators. For instance, with a lambda function, you can specify:
std::sort(numbers.begin(), numbers.end(), [](int a, int b) {
return a > b; // Sorts in descending order
});
This functionality allows for greater flexibility when sorting collections.
Sorting Complex Data Types
Structs and Classes
You might often need to sort collections of more complex data types, such as structs or classes. For example, consider a custom struct for storing a person's data:
struct Person {
std::string name;
int age;
};
std::vector<Person> people = {{"Alice", 30}, {"Bob", 25}};
std::sort(people.begin(), people.end(), [](const Person& a, const Person& b) {
return a.age < b.age; // Sorts by age
});
This snippet demonstrates how `std::sort` can handle any data type, provided you define the appropriate comparison logic.
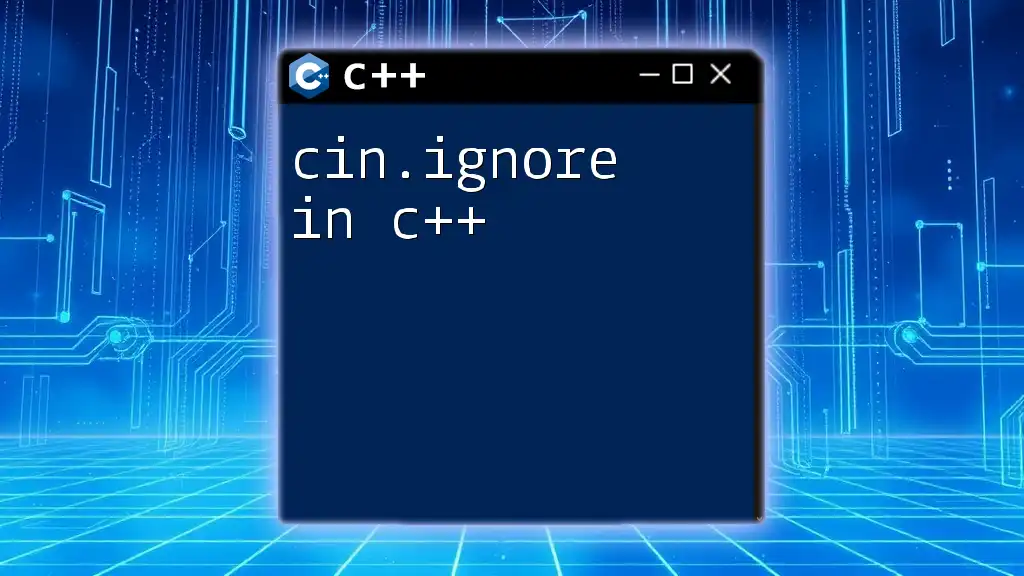
Other Sorting Functions in C++
`std::stable_sort`
While `std::sort` is efficient, it does not guarantee the preservation of the relative order of equal elements. `std::stable_sort`, on the other hand, maintains the order of equal elements. This might be crucial in cases where data integrity based on initial ordering matters.
You can utilize it similarly to `std::sort`:
std::stable_sort(people.begin(), people.end(), [](const Person& a, const Person& b) {
return a.name < b.name; // Sorts by name while preserving order
});
`std::partial_sort`
`std::partial_sort` is beneficial when you only need the top N elements sorted, rather than the entire dataset. In this scenario, `std::partial_sort` allows for efficient retrieval of the smallest or largest elements.
Here’s how you might implement it:
std::partial_sort(numbers.begin(), numbers.begin() + 3, numbers.end());
// Only the first three smallest numbers will be sorted
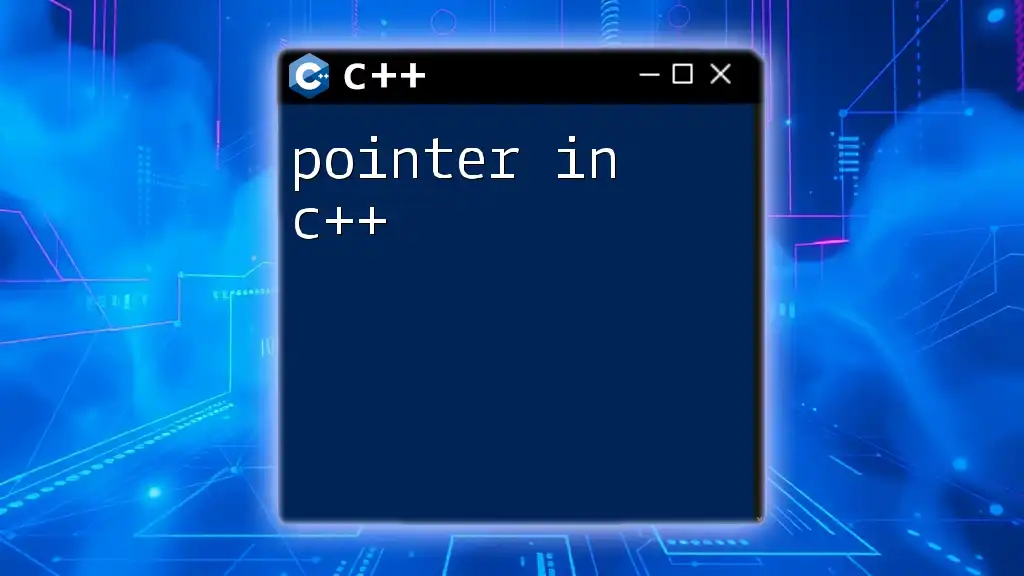
Performance Considerations
Time Complexity
Understanding time complexity is vital when choosing a sorting algorithm. The average time complexity for `std::sort` is O(n log n), while the worst-case scenario can reach O(n^2). Comparatively, `std::stable_sort` runs at O(n log n) in all scenarios, making it a reliable choice when stability is required.
Space Complexity
While sorting, the space complexity varies based on the algorithm in use. `std::sort` generally operates with a small auxiliary space, typically O(log n), whereas `std::stable_sort` may require more space, around O(n), to maintain the order.
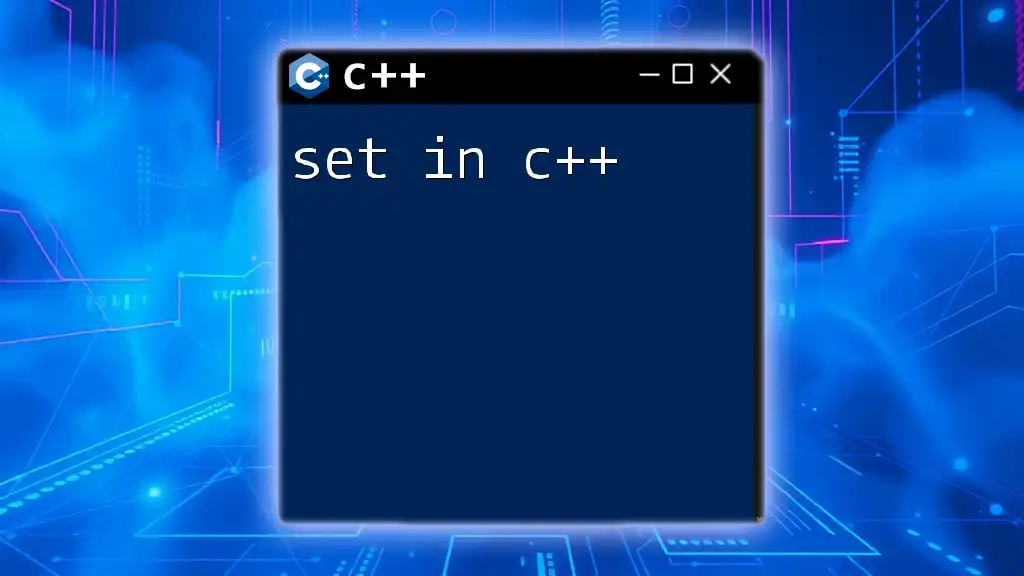
Common Errors and Debugging
Common Pitfalls
One frequent mistake is using incorrect iterators—ensuring you’re using valid range boundaries is crucial. If you fail to define the range correctly, it might lead to undefined behavior or runtime errors. For instance, calling `std::sort` with a range that exceeds the container sizes could cause segmentation faults.
To keep your sorting operations sound, always double-check iterator validity and consider leveraging strong type checking where applicable.
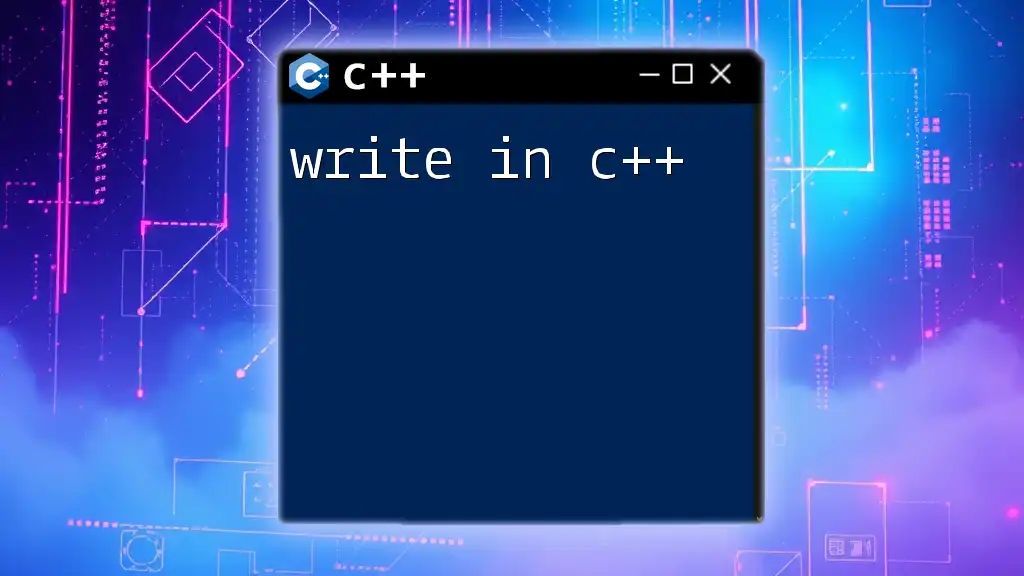
Conclusion
Sorting in C++ is a fundamental skill that enhances data manipulation and retrieval methods. By leveraging functions such as `std::sort`, `std::stable_sort`, and `std::partial_sort`, C++ developers can create more efficient and effective applications. Experimenting with various sorting techniques provides practical insights into data organization and algorithm optimization. As you delve deeper into C++, mastering sorting algorithms will undoubtedly empower you to build sophisticated data-driven solutions.
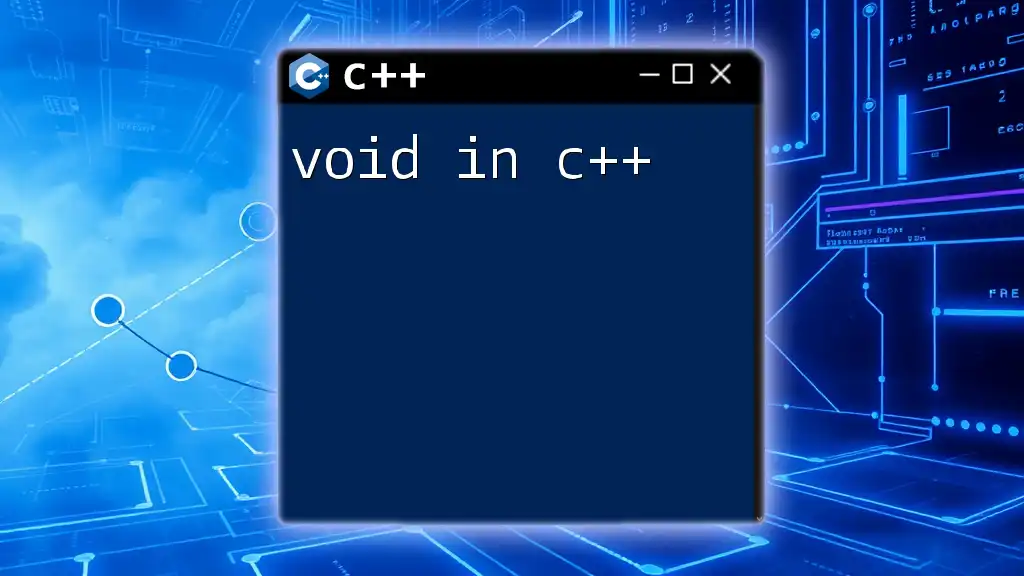
Further Reading and Resources
To enhance your understanding of sorting in C++, refer to the C++ documentation or additional tutorials online. There are numerous books and online courses that delve deeper into sorting and other data structure operations, paving the way for more advanced programming skills.