In C++, a `set` is an associative container that stores unique elements following a specific order, enabling efficient retrieval and management of these elements.
#include <iostream>
#include <set>
int main() {
std::set<int> mySet = {3, 1, 4, 2};
mySet.insert(5);
for (int num : mySet) {
std::cout << num << " "; // Output: 1 2 3 4 5
}
return 0;
}
What is a Set in C++?
A set in C++ is a powerful associative container that stores unique elements following a specific order. The C++ Standard Library provides a built-in implementation of sets, which allows developers to efficiently manage collections of values with no duplicates. Sets automatically sort their elements in a specific order, typically implemented as a binary search tree. This characteristic differentiates sets from other data structures, such as arrays and vectors, where duplicates are common and order can be arbitrary.
Characteristics of Sets:
- Unicity: Each element in a set is unique. If you attempt to add an existing element, it will simply be ignored.
- Ordering: Sets usually maintain elements in ascending order according to the element’s value, although you can customize this behavior.
This reliance on strict uniqueness and order is what makes sets a fitting choice in various programming scenarios.
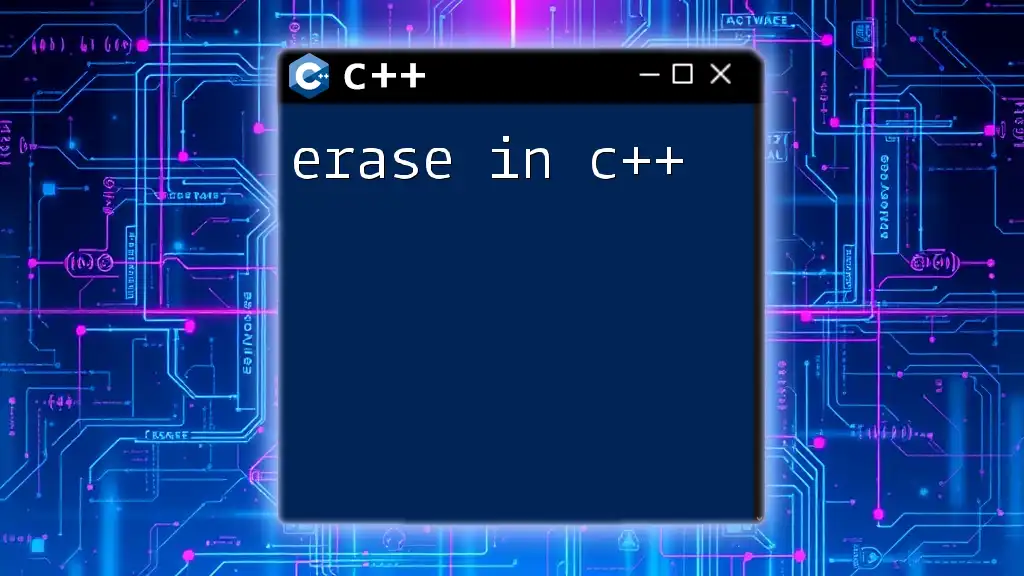
The C++ Standard Library and Sets
The C++ Standard Template Library (STL) is an essential part of modern C++ programming. It provides powerful algorithms and data structures, including sets. Using STL sets allows for better memory management, efficient searching and sorting capabilities, and code reusability, ultimately promoting cleaner and more maintainable code.
Benefits of using STL sets feature:
- Automatic sorting: You do not need to handle sorting manually.
- Performance: Operations like insertion, deletion, and searching can be performed in logarithmic time.
- Dynamic size: Sets can grow and shrink during runtime, adapting to varying data needs.
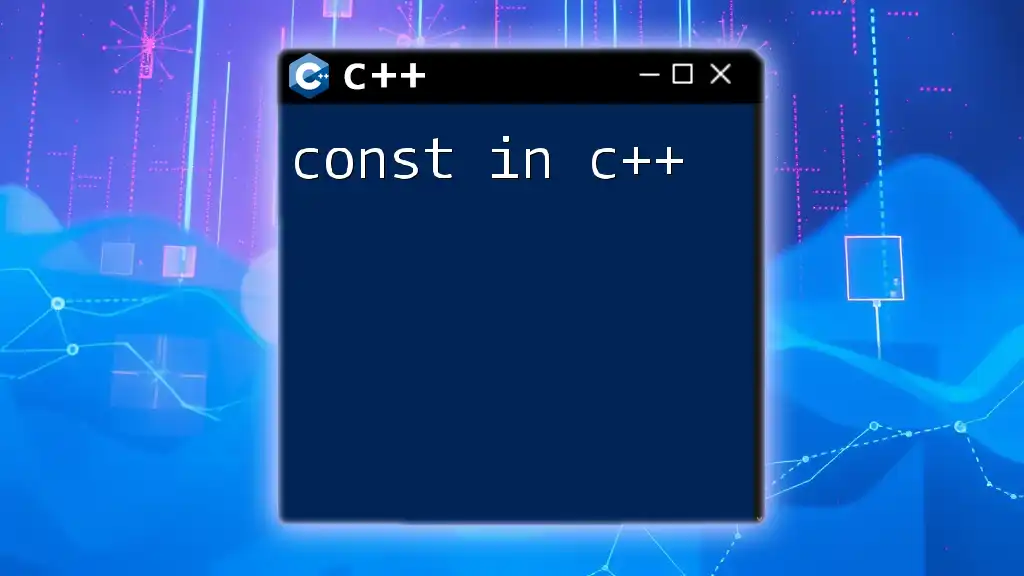
Declaring and Initializing Sets in C++
To declare a set in C++, you need to include the `<set>` header and use the following syntax:
#include <set>
std::set<int> mySet;
There are multiple ways to initialize a set:
std::set<int> mySet1 = {1, 2, 3}; // using an initializer list
std::set<int> mySet2(mySet1); // using the copy constructor
By initializing sets in various ways, you become versatile in managing data, depending on your requirements.
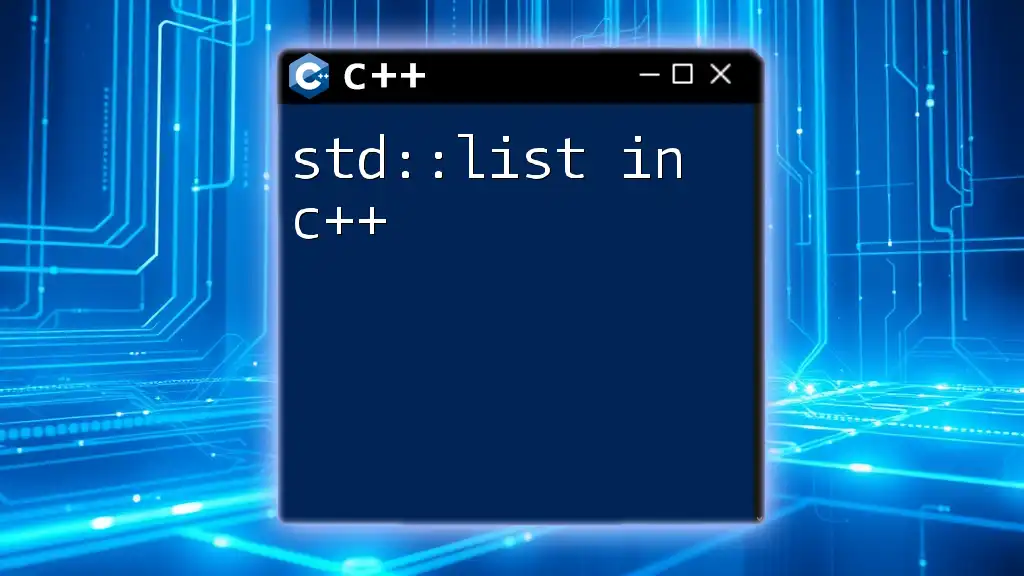
Basic Operations on Sets
Inserting Elements into a Set
Adding elements to a set is a fundamental operation. You can use the `insert` function, which ensures that only unique elements are added:
mySet.insert(4);
Alternatively, you can use `emplace()` for insertion, which constructs the object in-place and can be more efficient:
mySet.emplace(5);
Note: If you try to insert a duplicate element, it will not affect the set, and no error will be thrown.
Accessing Elements in a Set
Iterators play a significant role in accessing elements from a set. The ordering of elements makes the iteration inherently sorted:
for (const auto& element : mySet) {
std::cout << element << " ";
}
This concise method allows you to read through elements in a sorted manner and is useful for various operations.
Removing Elements from a Set
To erase an element, simply call the `erase` function:
mySet.erase(2);
If you want to clear the entire set, which removes all elements, you can use:
mySet.clear();
Remember that `erase()` will only remove the specified element, while `clear()` empties the set completely.
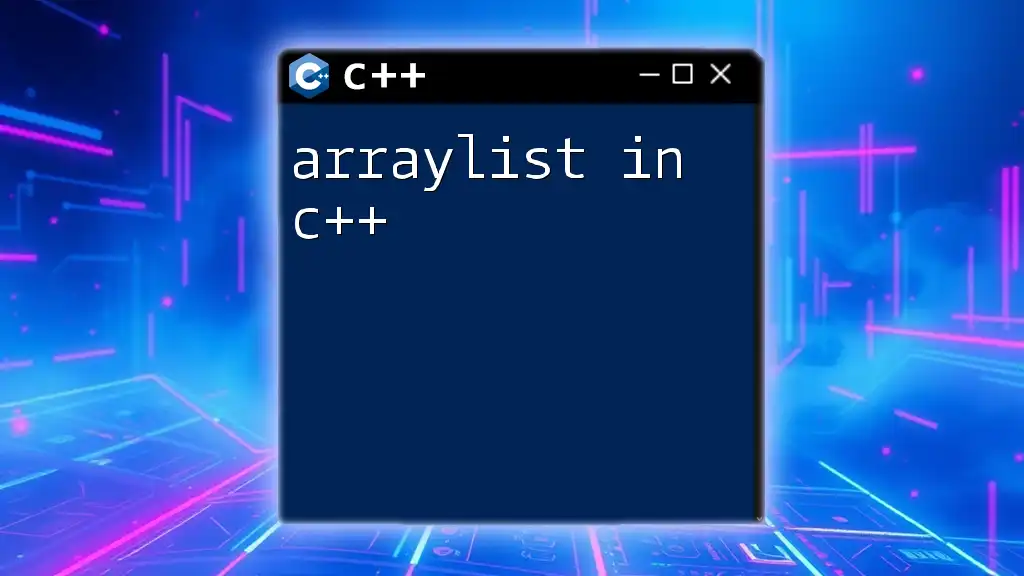
Advanced Features of Sets in C++
Set Operations
Working with multiple sets allows you to perform various operations such as union, intersection, and difference, further enriching your programming capabilities.
Example of Union:
std::set<int> setA = {1, 2, 3};
std::set<int> setB = {2, 3, 4};
std::set<int> unionSet;
std::set_union(setA.begin(), setA.end(), setB.begin(), setB.end(),
std::inserter(unionSet, unionSet.begin()));
Following this, you can similarly perform intersection and difference operations using `std::set_intersection` and `std::set_difference`.
Set Sorting and Ordering
By default, sets maintain ascending order based on their contained values. However, you can customize this behavior using a comparator. Below is an example that sorts elements in descending order:
struct CustomCompare {
bool operator() (int a, int b) {
return a > b; // descending order
}
};
std::set<int, CustomCompare> customSet;
This flexibility allows developers to tailor set behavior according to specific project requirements.
Multisets vs Sets
Multisets are a variation of sets that allow duplicate elements. They are useful when you need to keep track of how many times an item is added to a collection. Here’s how to declare and use a multiset:
#include <set>
std::multiset<int> myMultiSet;
myMultiSet.insert(1);
myMultiSet.insert(1); // Allows duplicate
When considering whether to use a multiset or a set, think about whether you need to handle duplicates. This understanding drives efficient data management.
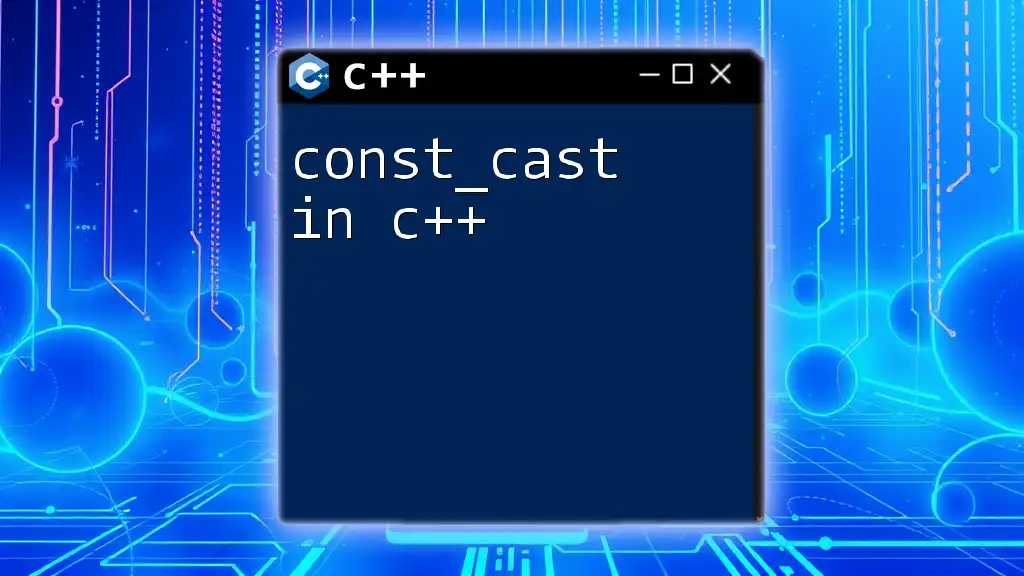
Real-World Applications of Sets in C++
Sets play a pivotal role in various practical programming scenarios. Some common applications include:
- Unique item storage: Ensuring that each stored item is unique, such as usernames or email addresses.
- Efficient searching functionalities: Quickly checking for the existence of an item without duplicates, making sets valuable in contexts like databases or user authentication.
- Counting unique elements: For instance, you can utilize a set to analyze text data and count unique words in a file simply by inserting each word into a set.
These real-world applications highlight the importance of using sets effectively in programming, showcasing their flexibility and utility.
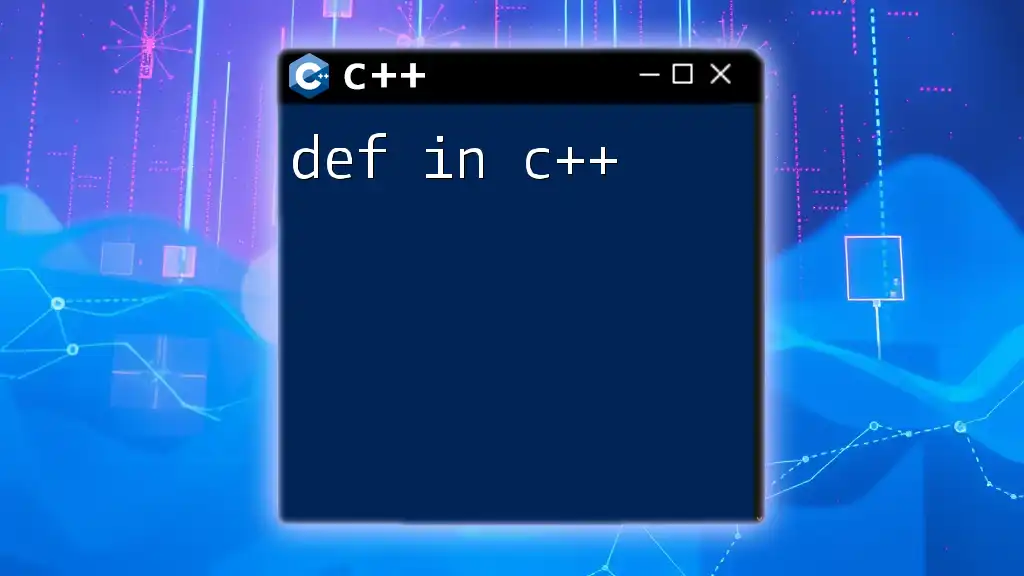
Best Practices for Using Sets in C++
When working with sets, consider the following best practices:
- Choose sets over other data structures when you need uniqueness and automatic sorting. They simplify duplicate management significantly.
- Evaluate performance considerations: While sets are efficient for insertion and removal, accessing elements can be a bit slower compared to vectors or arrays, especially when random access is required.
- Avoid common pitfalls: Ensure you understand the implications of ordering and uniqueness to prevent unexpected behavior during set operations.
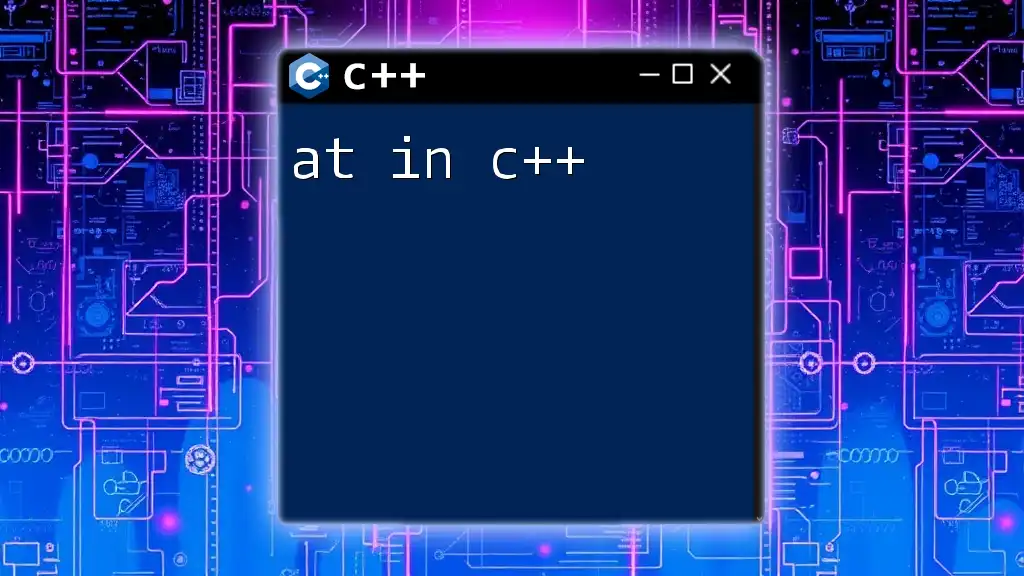
Conclusion
Understanding the intricacies of the set in C++ enhances your programming capabilities, facilitating effective data management while learning to leverage the STL. Mastering sets not only streamlines your coding experience but also unlocks new possibilities in problem-solving. Practice with examples, build your projects around sets, and witness how this essential container elevates your C++ programming skills.
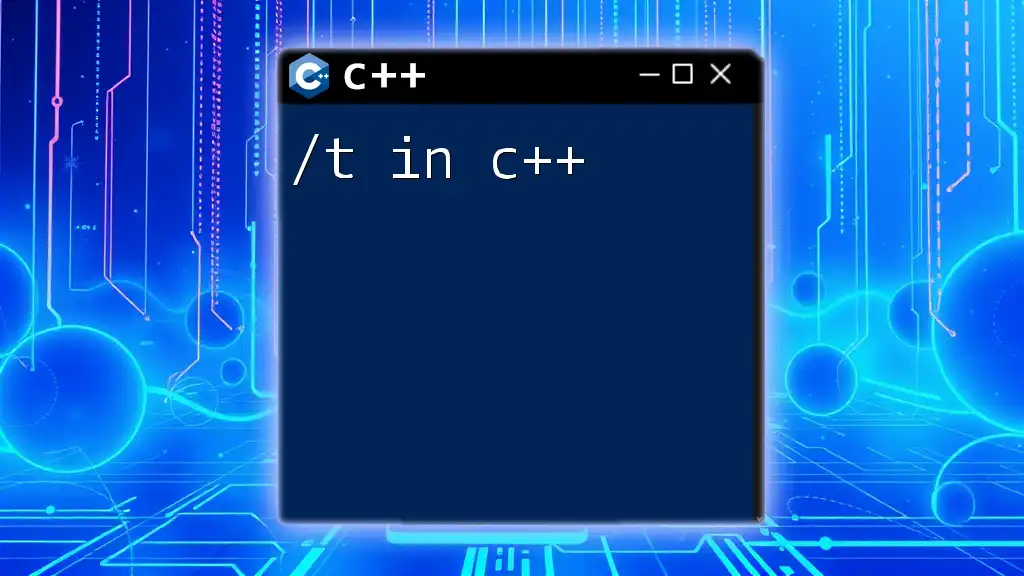
Additional Resources
For further exploration, consider checking C++ documentation, tutorials, and online courses specializing in STL and modern C++. Deepening your knowledge will arm you with practical insights and foster confidence in your programming capabilities.