`std::list` in C++ is a container that provides a doubly linked list, allowing for efficient insertions and deletions at any position within the sequence.
#include <iostream>
#include <list>
int main() {
std::list<int> myList = {1, 2, 3, 4, 5};
myList.push_back(6); // Add 6 to the end of the list
for (int i : myList) {
std::cout << i << " "; // Output: 1 2 3 4 5 6
}
return 0;
}
What is std::list?
The `std::list` in C++ is a standard template library (STL) container that implements a doubly linked list. This allows for efficient insertions and deletions from any position within the list, which makes it a highly versatile data structure. The key features of `std::list` include:
- Dynamic size: Unlike arrays, `std::list` can grow and shrink in size as required.
- Non-contiguous memory allocation: Each element can be stored at any location in memory, reducing the overhead of resizing when necessary.
- Bidirectional iterators: You can iterate through the list in both forward and backward directions.
Importance: By utilizing `std::list`, developers gain the ability to manage collections of data efficiently, particularly in situations where frequent insertions and deletions are necessary.
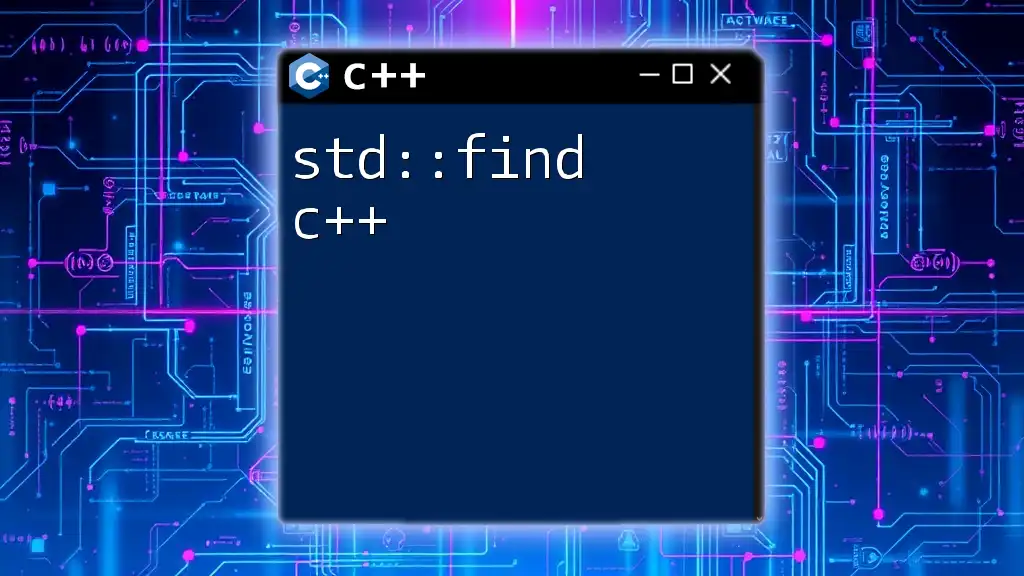
Why Use std::list?
Using `std::list` provides several advantages:
- Efficiency in Insertions/Deletions: It provides constant time complexity, O(1), for these operations, as there's no need to shift elements to maintain order.
- Memory Management: `std::list` dynamically manages memory, allowing natural handling of elements without worrying about underlying array sizes.
- Use Cases: Ideal for operations like maintaining a history of actions, implementing queues, or managing ordered collections where frequent modifications occur.
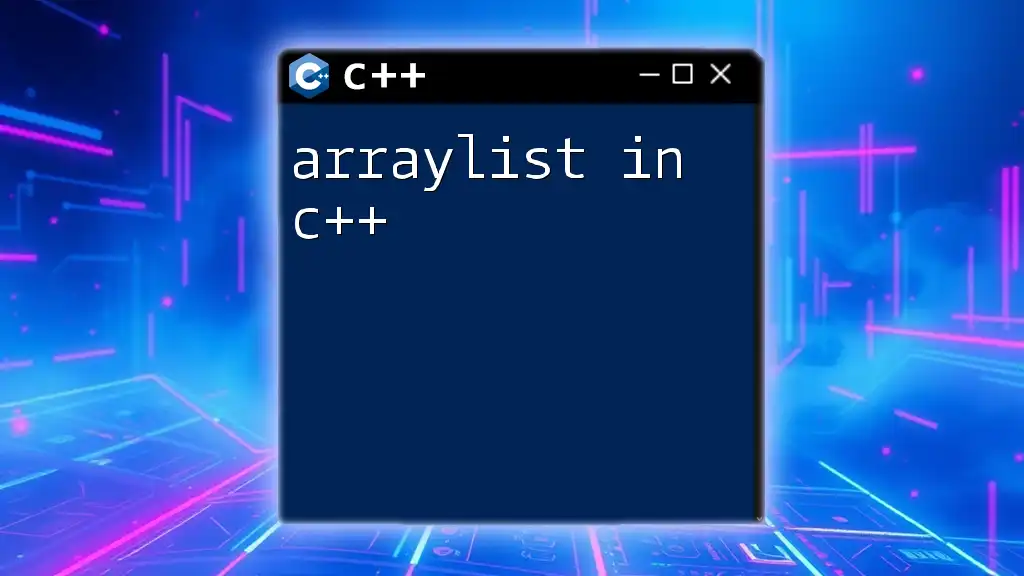
Getting Started with std::list in C++
Including the Necessary Header
To utilize `std::list`, include the following header at the beginning of your program:
#include <list>
This inclusion provides access to the `std::list` template and its member functions, allowing for powerful manipulation of linked lists.
Basic Syntax of std::list
Declaring a `std::list` is straightforward. The syntax requires a type declaration:
std::list<int> myList;
In this example, `myList` will hold integers. You can replace `int` with any data type, such as `float`, custom classes, etc.
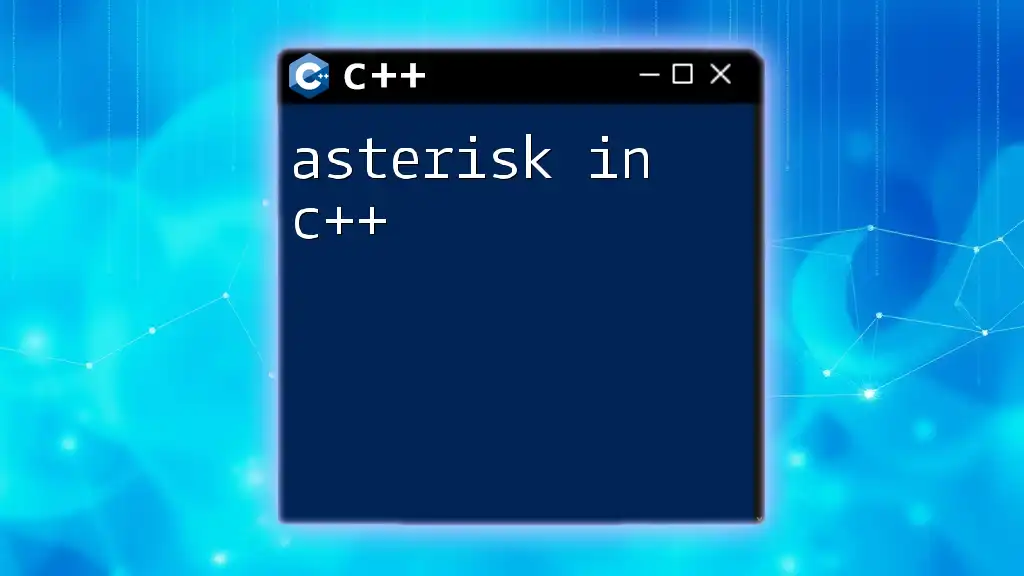
Creating and Initializing std::list
Default and Parameterized Constructors
`std::list` offers both default and parameterized constructors for instantiation:
std::list<int> defaultList;
std::list<int> initList = {1, 2, 3, 4, 5};
- Default Constructor: Creates an empty list.
- Parameterized Constructor: Initializes the list with specified values.
Other Initialization Techniques
You can also initialize a `std::list` from another container, like a vector:
std::vector<int> myVector = {1, 2, 3};
std::list<int> vectorList(myVector.begin(), myVector.end());
This transfers the elements from the vector to the list effectively.
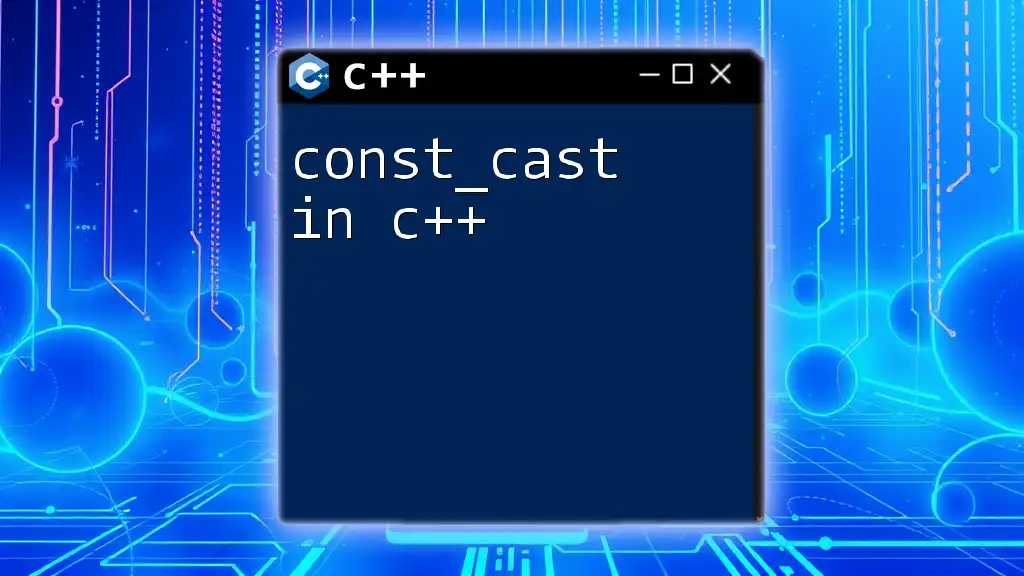
Common Operations with std::list
Adding Elements to a std::list
Inserting elements into a `std::list` can be efficiently done with the following member functions:
- push_back: Adds an element at the end of the list.
- push_front: Inserts an element at the beginning of the list.
Here’s an example demonstrating both:
myList.push_back(10); // myList: 10
myList.push_front(5); // myList: 5, 10
Removing Elements from a std::list
Removing elements from a `std::list` is equally intuitive with:
- pop_back: Removes the last element.
- pop_front: Removes the first element.
Example code for removal:
myList.pop_back(); // myList originally was 5, 10; now it is: 5
myList.pop_front(); // myList is now empty
Accessing Elements in std::list
To access elements, iterators are used effectively. Here’s how you can iterate through a list:
for (auto it = myList.begin(); it != myList.end(); ++it) {
std::cout << *it << " "; // Output each element of the list
}
Modifying Elements in std::list
You can also modify elements while iterating through the list:
for (auto it = myList.begin(); it != myList.end(); ++it) {
*it += 1; // Increment each value
}
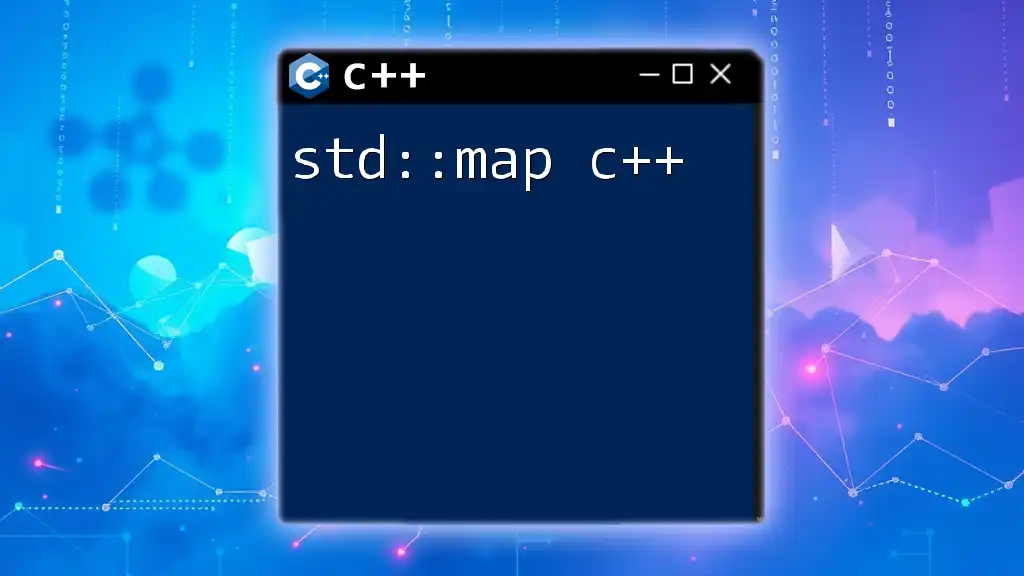
Advanced Features of std::list
Sorting std::list
Sorting can be performed directly using the `sort()` member function, which sorts the elements in ascending order by default:
myList.sort(); // Sorts elements in myList
Merging Two std::list
The `merge()` function allows merging two sorted lists into one:
std::list<int> anotherList = {6, 7, 8};
myList.merge(anotherList);
This essential function simplifies combining lists without additional looping or copying.
Reversing std::list
You can easily reverse the order of elements with the `reverse()` member function:
myList.reverse(); // Reverses the order of elements in myList
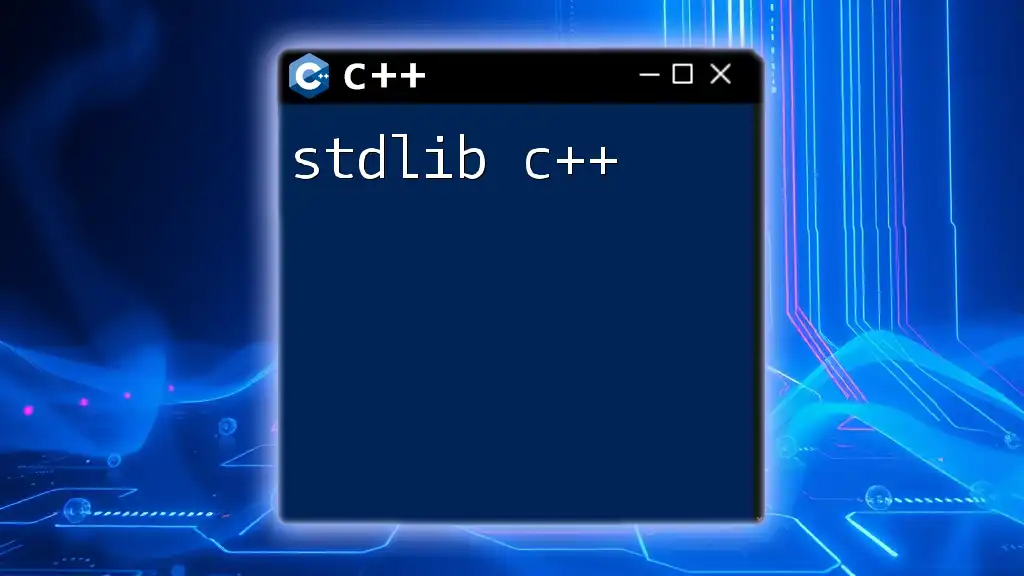
Best Practices When Using std::list
When to Choose std::list Over Other Containers
Select `std::list` when:
- You require frequent insertions and deletions from various positions.
- The order of elements matters, and you need stable iterators.
- Memory overhead of resizing other containers becomes a concern.
Common Mistakes to Avoid
Some common pitfalls include:
- Accessing Elements: Unlike vectors, `std::list` does not allow direct access to elements by index, leading to potential confusion.
- Iterators: Be cautious about invalidating iterators when elements are removed; always use iterators correctly to prevent undefined behavior.
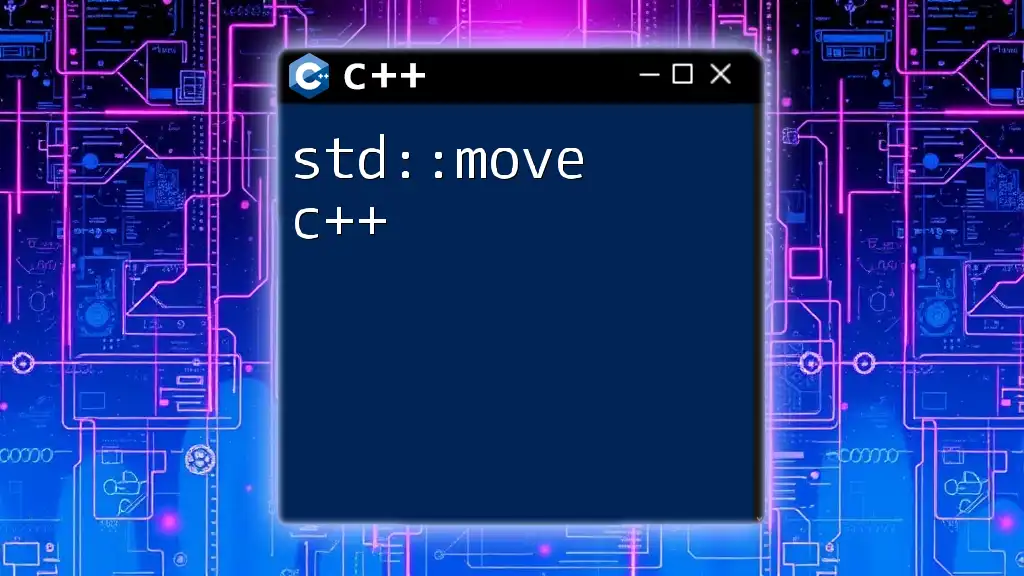
Conclusion
By leveraging `std::list in C++`, developers unlock a powerful tool for managing and manipulating collections of data. Understanding its features, functionalities, and appropriate use cases will elevate your coding proficiency and lead to more efficient algorithms in your projects.
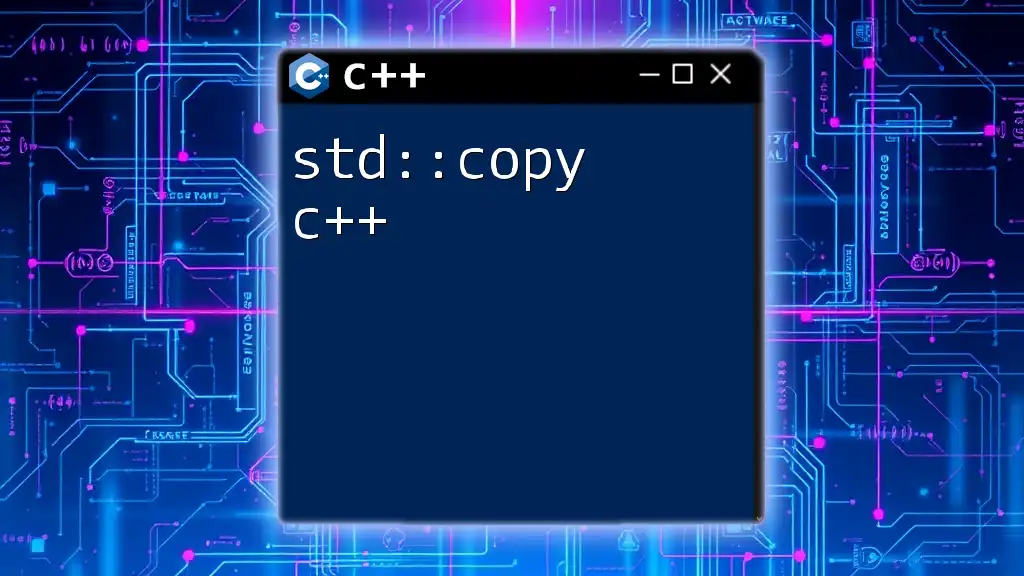
Additional Resources
For further reading and to deepen your understanding of `std::list`, refer to the official C++ Standard Library documentation and consider exploring additional books or online courses dedicated to C++ programming.