The `std::map` in C++ is an associative container that stores elements in key-value pairs, allowing fast retrieval of values based on their associated keys.
Here’s a simple example of using `std::map`:
#include <iostream>
#include <map>
int main() {
std::map<std::string, int> ageMap;
ageMap["Alice"] = 30;
ageMap["Bob"] = 25;
// Accessing values
std::cout << "Alice's age: " << ageMap["Alice"] << std::endl;
std::cout << "Bob's age: " << ageMap["Bob"] << std::endl;
return 0;
}
Understanding the Basics of `std::map`
Definition and Syntax
The `std::map` in C++ is a standard library container that implements an associative array, allowing you to store key-value pairs. The syntax for declaring a map follows this general form:
std::map<KeyType, ValueType> mapName;
In this declaration, `KeyType` specifies the type of the keys, while `ValueType` defines the type of the values. The keys must be unique within a map, meaning that no two elements can have the same key.
How `std::map` Works
Internally, `std::map` organizes its elements in a balanced binary tree (typically a Red-Black tree). This guarantees that all keys are stored in sorted order, which enables efficient lookup, insertion, and deletion operations. The key-value pairs within a map allow for both fast access to the data and clear organization, making it a versatile tool in C++ development.
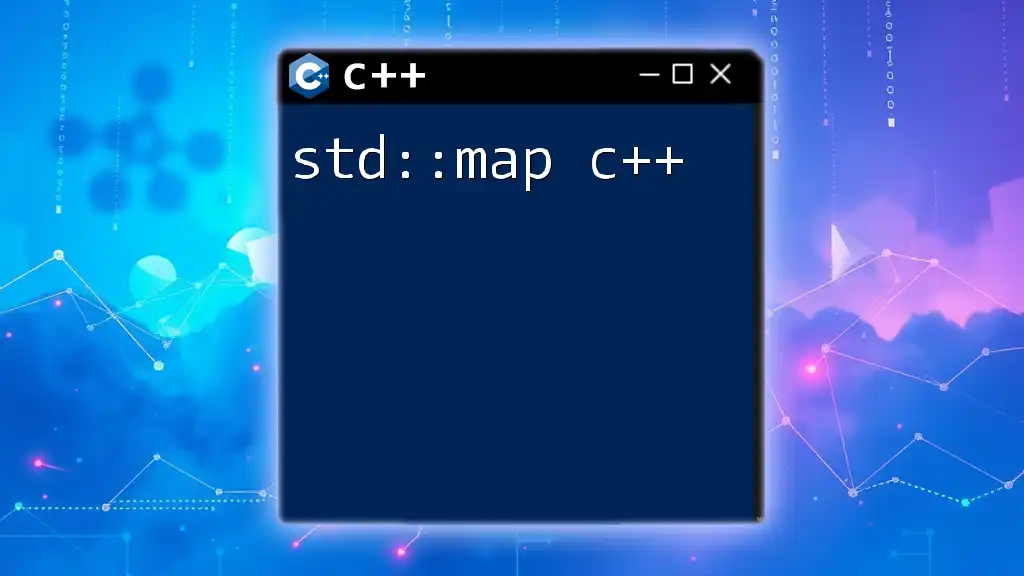
Benefits of Using `std::map`
Logarithmic Time Complexity
One of the most significant advantages of using `std::map` is its logarithmic time complexity for common operations such as insertions, deletions, and lookups. These operations typically run in O(log n) time, which means they are efficient even for larger datasets.
For example, inserting elements into a `std::map` can be done as follows:
std::map<int, std::string> myMap;
myMap.insert({1, "One"});
myMap.insert({2, "Two"});
Automatic Sorting
Another compelling feature of `std::map` is its ability to maintain the order of keys automatically. This is advantageous when you need to perform sorted operations or need to traverse the map in a specific order. Unlike some other associative containers, `std::map` handles sorting behind the scenes, saving you the hassle of manually managing order.
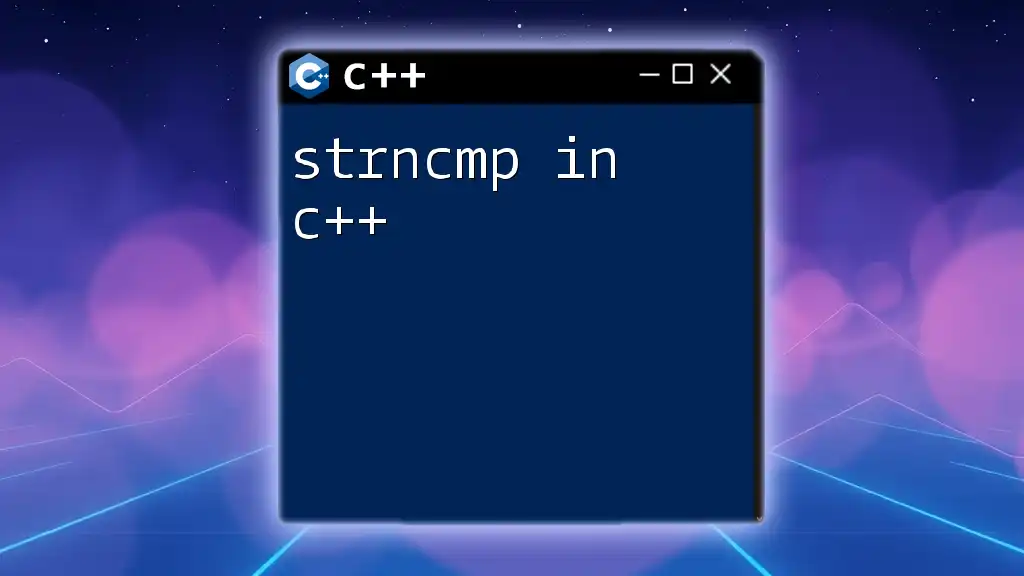
Key Operations on `std::map`
Inserting Elements
Inserting elements into a `std::map` can be accomplished using several methods. The most common methods are the `insert()` function and the index operator (`[]`).
Using the `insert()` method:
myMap.insert({3, "Three"});
Alternatively, you can use the index operator, which not only allows for insertion but also updates existing values:
myMap[4] = "Four"; // Insert a new key-value pair
myMap[1] = "One Updated"; // Updates the value of key 1
Accessing Elements
To retrieve values associated with a specific key, you can use the `at()` method or the index operator. Here’s a quick comparison:
Using the `at()` method:
std::cout << myMap.at(2) << std::endl; // Output: Two
Using the index operator:
std::cout << myMap[4] << std::endl; // Output: Four
Keep in mind, the `at()` method throws an `out_of_range` exception if the key does not exist, while using the index operator will insert a new element with a default value for the key if it doesn’t exist.
Deleting Elements
To remove elements from a `std::map`, you can utilize the `erase()` method. This method allows for deletion by key or by iterator. Removing an element by key is straightforward:
myMap.erase(2); // This removes the entry with key 2
Iterating Over a `std::map`
Retrieving all key-value pairs from a map is easy with iteration. You can use iterators or a range-based for loop. For instance, a range-based for loop would look like this:
for (const auto &pair : myMap) {
std::cout << pair.first << ": " << pair.second << std::endl;
}
This will display all keys and their corresponding values in the order they are stored.
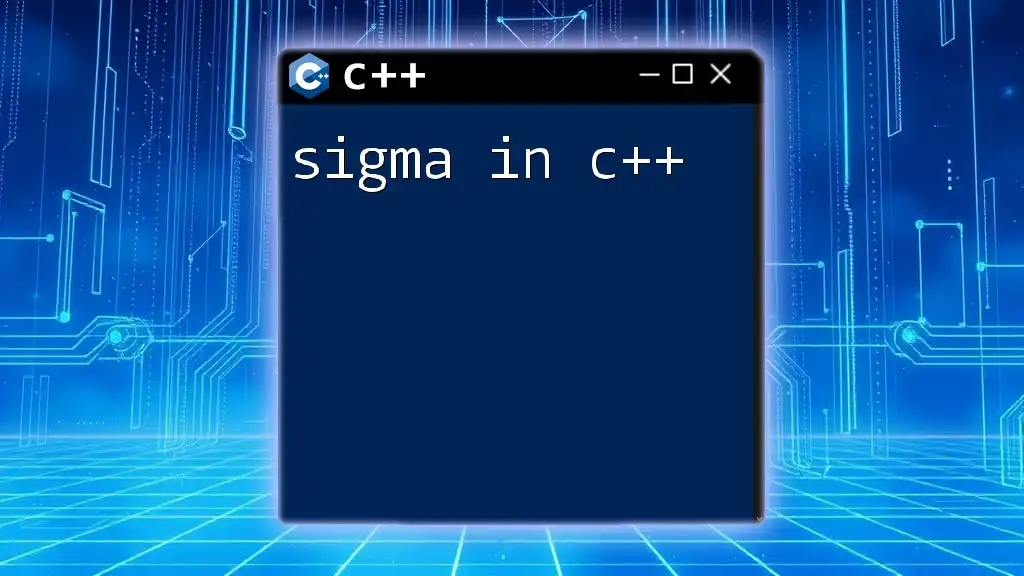
Advanced Usage of `std::map`
Custom Comparators
In some situations, you may need to customize the way keys are compared. This can be achieved by defining a custom comparator. Below is an example of implementing a map with a custom comparison that sorts keys in descending order:
struct CustomCompare {
bool operator() (const int &a, const int &b) const {
return a > b; // Sort in descending order
}
};
std::map<int, std::string, CustomCompare> customMap;
`std::map` vs. Other Associative Containers
Understanding when to use `std::map` versus other associative containers, like `std::unordered_map` or `std::set`, can significantly impact your program's efficiency. While `std::map` maintains order and provides logarithmic complexity, `std::unordered_map` generally offers average constant time complexity for lookups due to hashing.
Choose `std::map` when you require sorted keys or need to handle range queries. Conversely, favor `std::unordered_map` for faster access without the need for ordering.
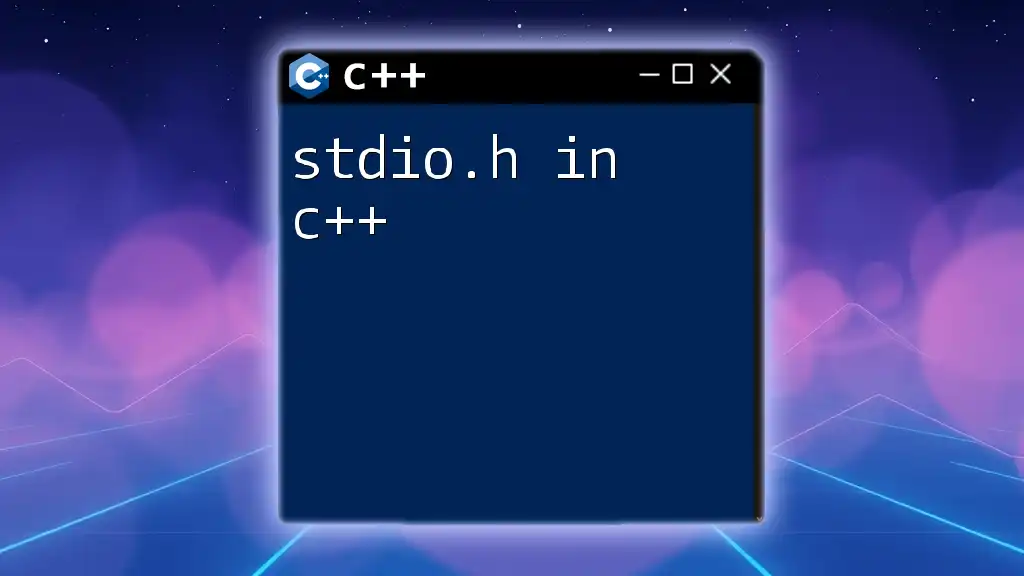
Common Mistakes and Best Practices
Avoiding Key Duplicates
It's crucial to understand that `std::map` does not allow duplicate keys. Attempting to insert a duplicate key will result in updating the existing key's value. For instance:
myMap[1] = "Another One"; // This updates the existing key 1
Choosing the Right Container
Selecting the appropriate data structure for your needs is essential in C++ programming. Use `std::map` when you need unique sorted keys with efficient lookup and dynamic updates. Being aware of the specific requirements of your application will help you leverage the full capabilities of `std::map` and ensure optimal performance.
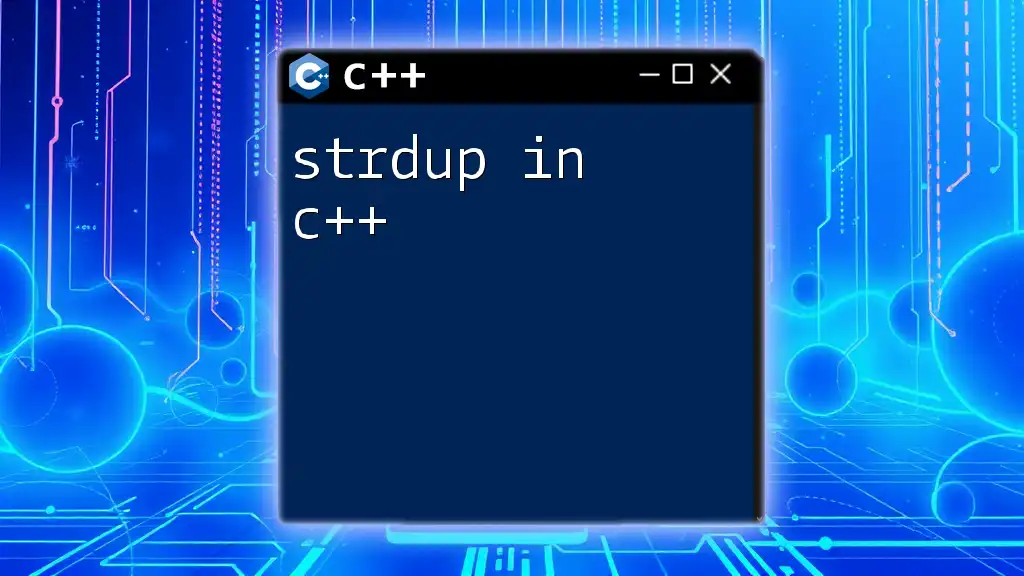
Conclusion
In summary, `std::map` in C++ is a robust and versatile associative container, ideal for storing key-value pairs with all keys sorted. By understanding how to efficiently insert, access, and manipulate elements within a map, as well as recognizing its performance characteristics compared to other containers, you will be well-equipped to utilize this powerful tool in your C++ projects. Remember, practical application and hands-on coding will solidify your understanding and mastery of `std::map`.