The `stdio.h` header in C++ provides functionalities for input and output operations, such as reading from the standard input or writing to the standard output.
Here’s a simple example of using `stdio.h` in C++ to print a message:
#include <stdio.h>
int main() {
printf("Hello, World!\n");
return 0;
}
What is stdio.h?
Definition and Purpose
`stdio.h` is a standard input/output library in C and C++ that provides functions to perform input and output operations. Its deeply embedded role in programming lies in its ability to facilitate interaction between the program and the user or files. The functions offered by `stdio.h` manage data input from the keyboard and output to the console or files, making it an essential tool for developers.
History and Evolution
Initially developed for the C programming language, `stdio.h` has found its way into C++ as well, preserving compatibility while allowing for improvements aligned with modern programming paradigms. While C++ offers more advanced input/output facilities through the `<iostream>` library, `stdio.h` endures, especially among systems programmers and situations where performance is crucial.
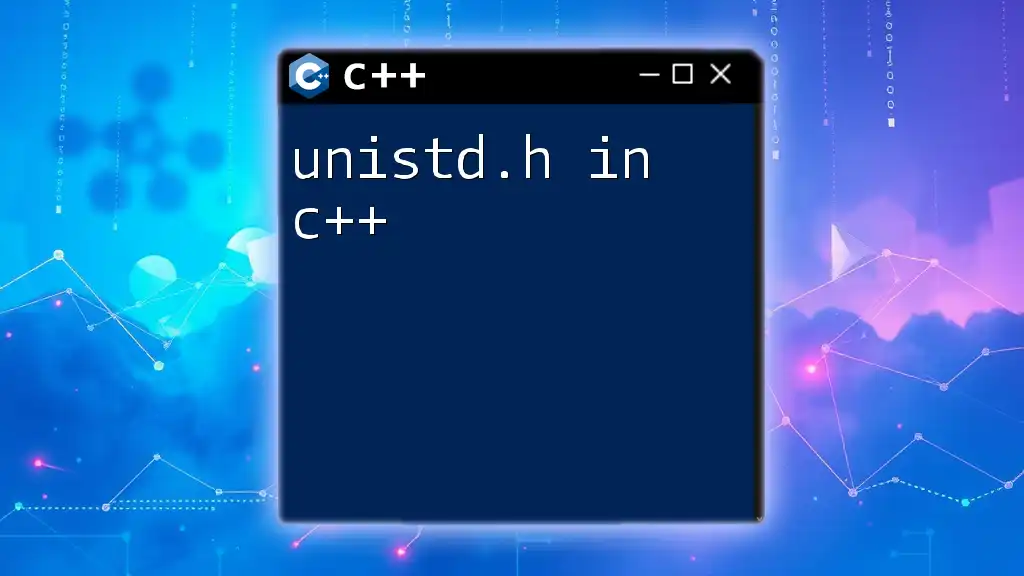
Key Functions of stdio.h in C++
printf() Function
Usage and Syntax The `printf()` function is a cornerstone of `stdio.h`, used to send formatted output to the standard output stream, typically the console. Its syntax is as follows:
int printf(const char *format, ...);
Example Here's a basic usage of the `printf()` function:
#include <stdio.h>
int main() {
printf("Hello, World!\n");
return 0;
}
Common Format Specifiers Understanding format specifiers is crucial for correctly formatting output:
- %d: Displays integers.
- %f: Displays floating-point numbers.
- %s: Displays strings.
- %c: Displays characters.
scanf() Function
Usage and Syntax `scanf()` serves as the counterpart to `printf()`, allowing the program to read input from the user. Its syntax is structured as follows:
int scanf(const char *format, ...);
Example Here's how you might use `scanf()` to gather user input:
#include <stdio.h>
int main() {
int number;
printf("Enter a number: ");
scanf("%d", &number);
printf("You entered: %d\n", number);
return 0;
}
Input Specifiers and Best Practices Using `scanf()` involves several common pitfalls:
- Ensure variables' addresses are correctly passed using the `&` operator.
- Always validate input to guard against format mismatches and overflow errors.
File Handling Functions
fopen()
To interact with files in C++, the `fopen()` function permits opening files in specified modes. This is how it works:
FILE *fopen(const char *filename, const char *mode);
Example Here’s a practical example of using `fopen()`:
#include <stdio.h>
int main() {
FILE *file = fopen("example.txt", "r");
if (file) {
// File operations here
fclose(file);
}
return 0;
}
This example opens a file called "example.txt" in read mode and ensures the file is closed afterward to free up system resources.
fclose()
Closing a file is just as important as opening it. `fclose()` is used to close files that have been opened with `fopen()`.
Purpose and Usage Properly closing files helps avoid memory leaks and keeps file data consistent. Following is an example of using `fclose()` in conjunction with `fopen()`:
#include <stdio.h>
int main() {
FILE *file = fopen("output.txt", "w");
fprintf(file, "Hello, File!\n");
fclose(file); // Always remember to close the file after use
return 0;
}
fprintf() and fscanf()
These functions allow for formatted output to files and input from files, respectively.
Example of fprintf()
FILE *file = fopen("output.txt", "w");
fprintf(file, "Hello, File!\n"); // Write to the file
fclose(file);
Example of fscanf()
FILE *file = fopen("input.txt", "r");
int number;
fscanf(file, "%d", &number); // Read an integer from the file
fclose(file);
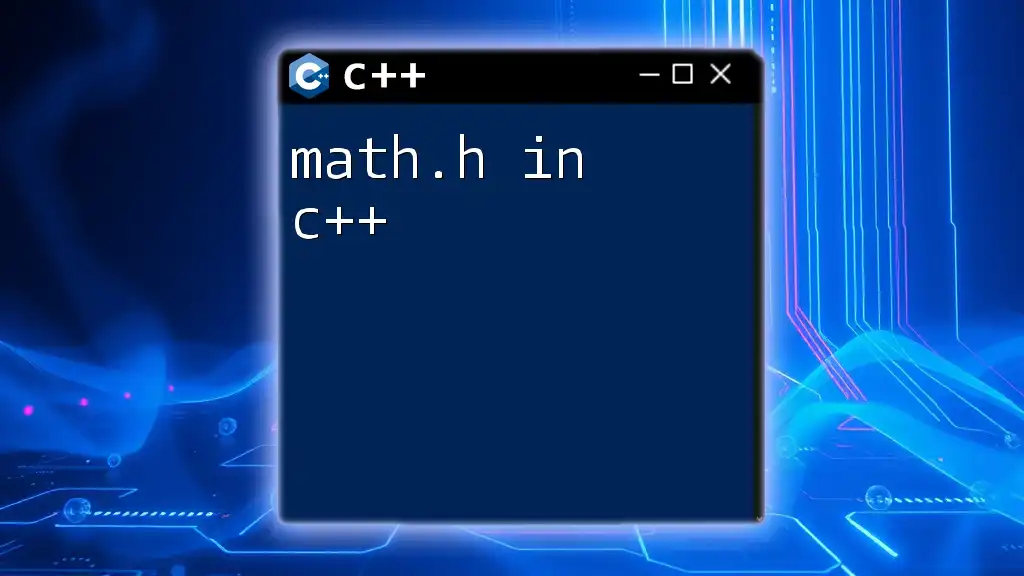
Benefits of Using stdio.h in C++
Performance Considerations
In scenarios stressing performance and efficiency, `stdio.h` functions tend to outperform C++ stream classes. This is due to their lower overhead, particularly advantageous in systems programming or when rapid input/output operations are paramount. For instance, using `printf()` and `scanf()` may yield faster results than employing `std::cout` and `std::cin`, albeit at the cost of type safety.
Portability
`stdio.h` provides reliable cross-platform compatibility. Programs that utilize `stdio.h` functions often work seamlessly across different operating systems, making them particularly useful for developers targeting diverse environments or working on legacy codebases.
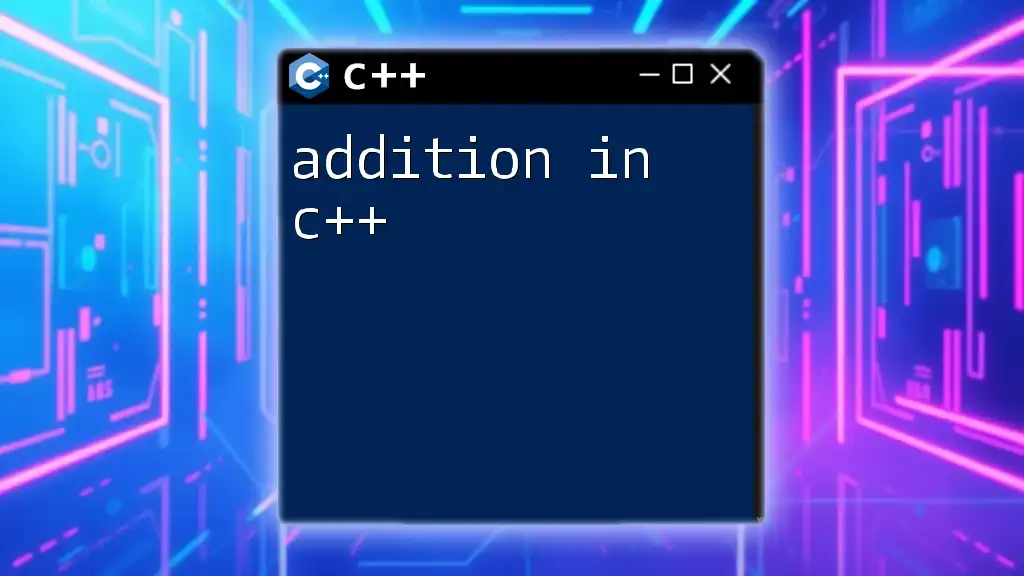
Challenges and Limitations of stdio.h
Error Handling
One notable drawback of using functions from `stdio.h` is the lack of robust error handling. For instance, `printf()` does not provide direct feedback on whether it executed successfully. Developers must frequently implement additional checks to manage potential errors effectively, a task that is more straightforward with C++'s exception handling.
Type Safety
Type safety remains a significant issue with `stdio.h`. Functions such as `printf()` and `scanf()` do not enforce type checks, leading to potential issues if format specifiers and argument types are mismatched. This often results in undefined behavior, making type checks critical when using these functions.
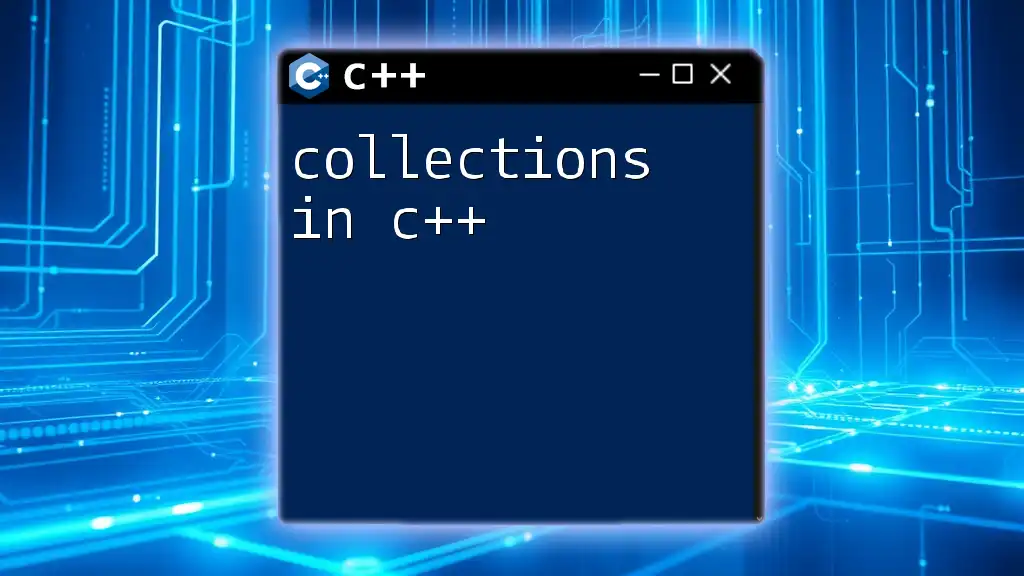
Best Practices for Using stdio.h in C++
Code Readability
When utilizing `stdio.h`, ensure that code remains readable and maintainable. This can be achieved by using descriptive variable names, consistent formatting, and incorporating comments explaining the purpose of particular sections of code. Clear documentation fosters better understanding for both you and any future collaborators.
Combining with C++ Features
Integrating `stdio.h` with C++ features can yield powerful results. For example, while it's common to use `cout` for output, leveraging `printf()` within C++ classes can allow for clean formatting when required. Just remember to keep type safety in check.
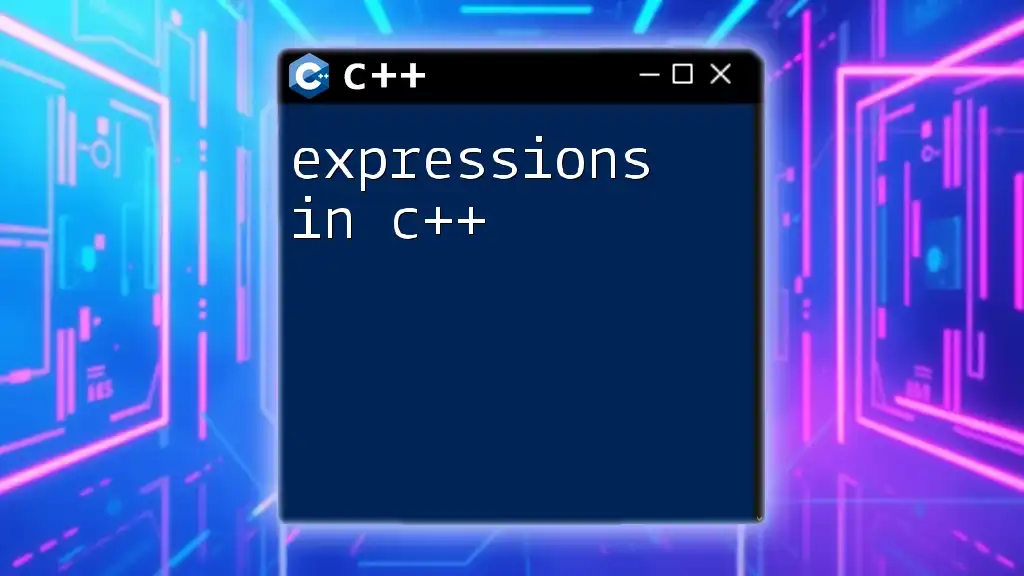
Conclusion
`stdio.h in C++` represents a foundational aspect of input and output operations, coming with its own strengths and limitations. While modern C++ provides alternative libraries, understanding and using `stdio.h` can enhance both performance and compatibility across projects. Developers are encouraged to explore `stdio.h` along with C++'s `<iostream>`, embracing the richness of tools at their disposal to cater to different programming needs.
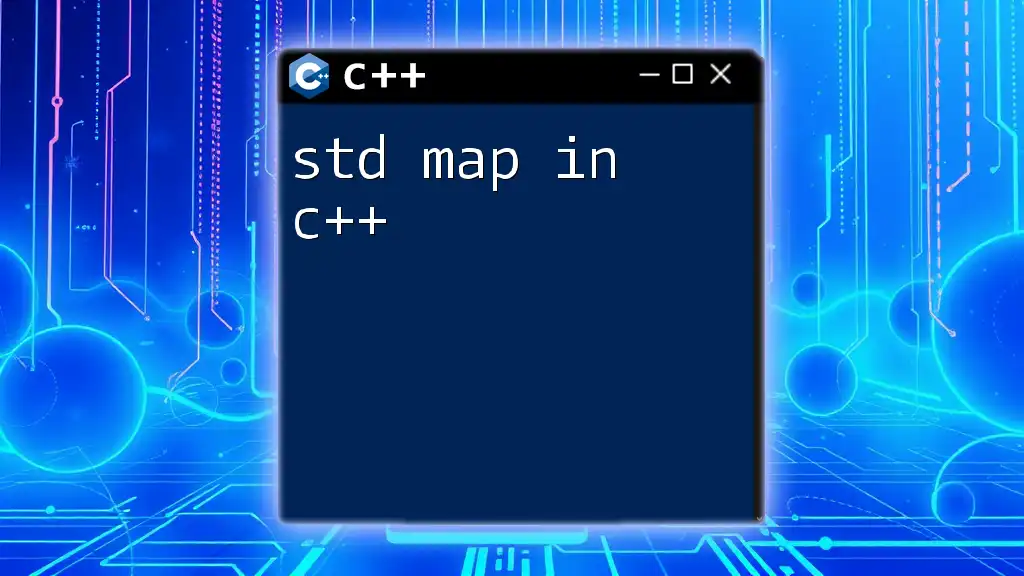
Additional Resources
For further reading and resources, explore tutorials and documentation on `stdio.h` and related C/C++ input/output operations. Developers can expand their skills through both online courses and comprehensive guides, ensuring they master this critical component of programming.