The `math.h` library in C++ provides a collection of mathematical functions and constants that enable developers to perform complex mathematical calculations easily.
Here's a simple example using `math.h` to calculate the square root of a number:
#include <iostream>
#include <cmath>
int main() {
double number = 25.0;
double result = sqrt(number);
std::cout << "The square root of " << number << " is " << result << std::endl;
return 0;
}
The Basics of Including math.h in C++
How to Include math.h in Your C++ Project
To utilize the powerful functions provided by the C++ mathematical library, you need to include it in your project. This can be accomplished simply with the following directive:
#include <math.h>
While `math.h` is derived from C, it can be seamlessly used in C++ programs. It's essential to note the alternative header, `cmath`, which is included in the C++ standard. The significant difference between `math.h` and `cmath` is primarily in how functions are defined with respect to namespaces. In C++, functions are included within the `std` namespace when using `cmath`, whereas they are in the global namespace with `math.h`. While both headers provide the same functionality, using `cmath` is preferred in C++ programming for better compatibility with modern C++ practices.
Importance of the Math Library
The `math.h` library is crucial for performing mathematical computations, offering a variety of functions ranging from simple arithmetic to complex calculations. This ensures that developers do not need to reinvent the wheel when performing standard mathematical functions.
The functionality of `math.h` standardizes math operations, allowing C++ developers to focus on algorithm development without worrying about the underlying implementation of math functions, thereby improving code longevity and readability.
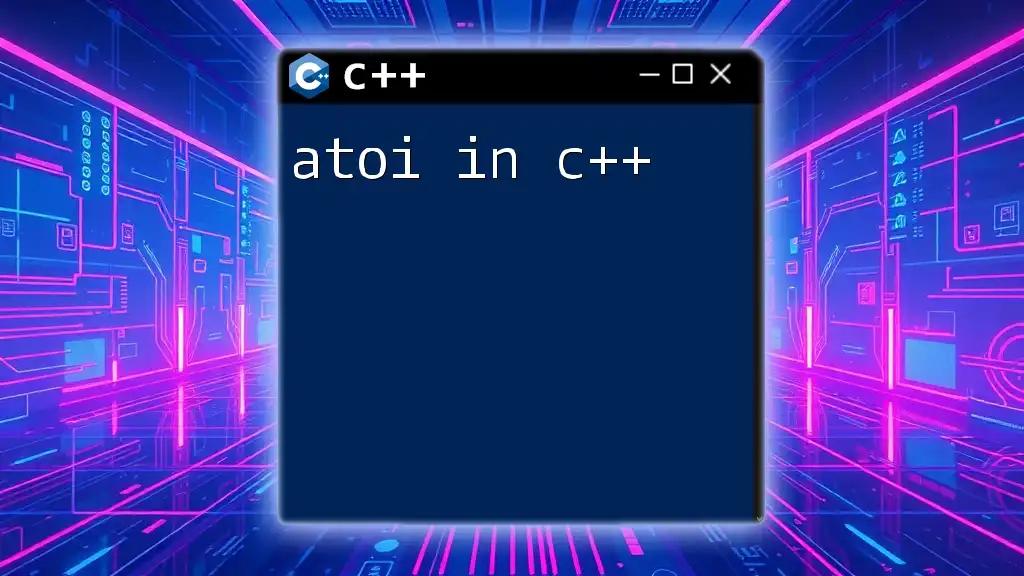
Key Functions Provided by math.h
Trigonometric Functions
The trigonometric functions provided by `math.h` allow for calculations involving angles, which are pivotal in fields such as physics, engineering, and computer graphics. The most commonly used functions include:
- `sin()`: Sine of an angle (in radians)
- `cos()`: Cosine of an angle (in radians)
- `tan()`: Tangent of an angle (in radians)
Here’s a simple usage example of these functions:
#include <iostream>
#include <math.h>
int main() {
double angle = 45.0; // Angle in degrees
double radians = angle * (M_PI / 180.0); // Convert to radians
std::cout << "Sin: " << sin(radians) << std::endl;
std::cout << "Cos: " << cos(radians) << std::endl;
std::cout << "Tan: " << tan(radians) << std::endl;
return 0;
}
This code snippet converts degrees to radians before computing the sine, cosine, and tangent, highlighting a common practice in mathematical programming.
Exponential and Logarithmic Functions
The exponential and logarithmic functions are essential in various domains, such as science and finance. They include:
- `exp()`: Returns e raised to the power of the argument
- `log()`: Natural logarithm of the argument
- `exp10()`: Base-10 exponential function
Consider the following example, which demonstrates the use of these functions:
#include <iostream>
#include <math.h>
int main() {
double value = 2.7183; // representing e
std::cout << "Exp: " << exp(value) << std::endl;
std::cout << "Natural Log: " << log(value) << std::endl;
return 0;
}
This code illustrates how `math.h` facilitates calculations involving the natural exponential function and logarithms.
Power and Root Functions
The power and root functions are core components of many mathematical operations. The most notable functions provided by `math.h` in this category include:
- `pow(base, exponent)`: Computes base raised to the exponent
- `sqrt()`: Returns the square root of a given number
Here’s how to use these functions effectively:
#include <iostream>
#include <math.h>
int main() {
double base = 3.0, exponent = 4.0;
std::cout << "Power: " << pow(base, exponent) << std::endl;
std::cout << "Square Root: " << sqrt(16.0) << std::endl;
return 0;
}
The example demonstrates both the ability to compute powers and square roots simply and effectively using `math.h`.
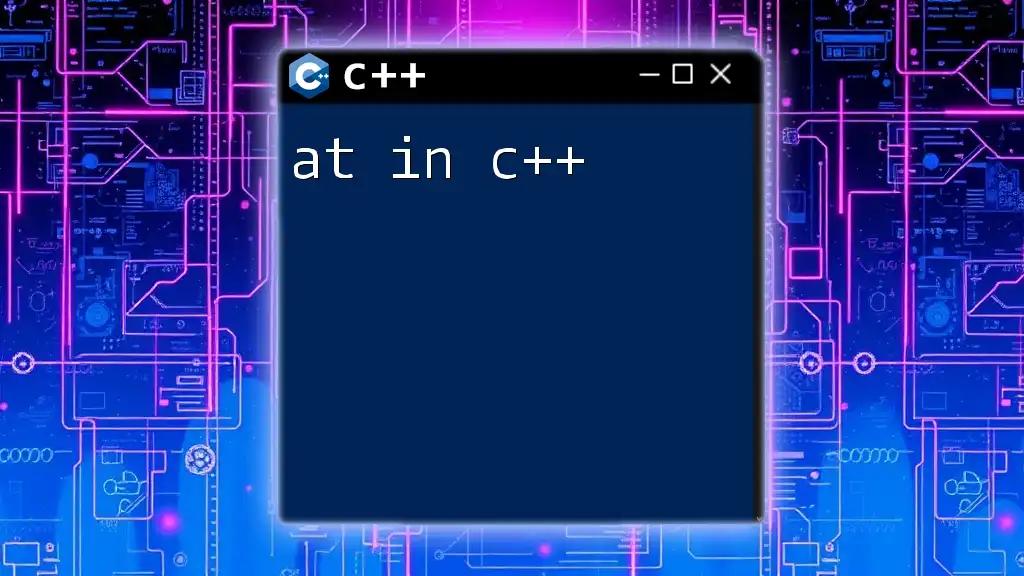
Special Functions in math.h
Rounding Functions
Rounding functions are particularly valuable in numerical analysis and applications requiring precision control. The key rounding functions in `math.h` include:
- `ceil()`: Rounds a number up to the nearest integer
- `floor()`: Rounds a number down to the nearest integer
- `round()`: Rounds a number to the closest integer according to standard rounding rules
#include <iostream>
#include <math.h>
int main() {
double num = 5.7;
std::cout << "Ceil: " << ceil(num) << std::endl;
std::cout << "Floor: " << floor(num) << std::endl;
std::cout << "Round: " << round(num) << std::endl;
return 0;
}
In this example, we demonstrate how these functions handle rounding for the given number, providing insight into floating-point arithmetic.
Error and Gamma Functions
In less common, but equally important scenarios, the `math.h` library includes the error function `erf()` and the gamma function `tgamma()`. The error function is widely used in statistics, particularly regressions, while the gamma function extends the factorial function beyond integers.
#include <iostream>
#include <math.h>
int main() {
double x = 0.5; // Argument for functions
std::cout << "Error Function: " << erf(x) << std::endl;
std::cout << "Gamma Function: " << tgamma(x) << std::endl;
return 0;
}
This snippet illustrates both functions in action, showcasing their utility in advanced mathematics.
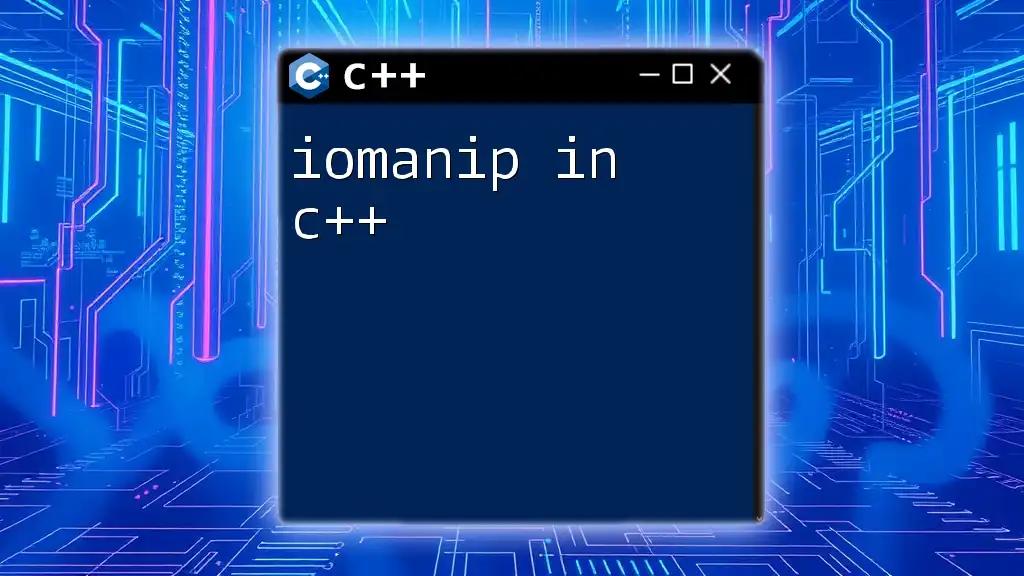
Practical Applications of math.h in C++
Applications in Real-World Projects
The trigonometric functions from `math.h` find their application across graphic renderings. For example, they can be leveraged to compute visual angles and coordinates in a 2D or 3D space.
Similarly, logarithmic functions are vital in data analysis, transforming exponentially growing datasets into manageable forms, enabling researchers and analysts to derive meaningful insights from raw data.
Performance Considerations
When using `math.h`, it is important to consider computational efficiency, especially when the mathematical function is called within loops or performance-critical sections of the code. Using intrinsic functions provided by compilers (often optimized) could yield better performance than their counterparts in `math.h`. Substituting alternative specialized libraries (such as Eigen or Boost.Math) can also improve efficiency for complex mathematical operations.
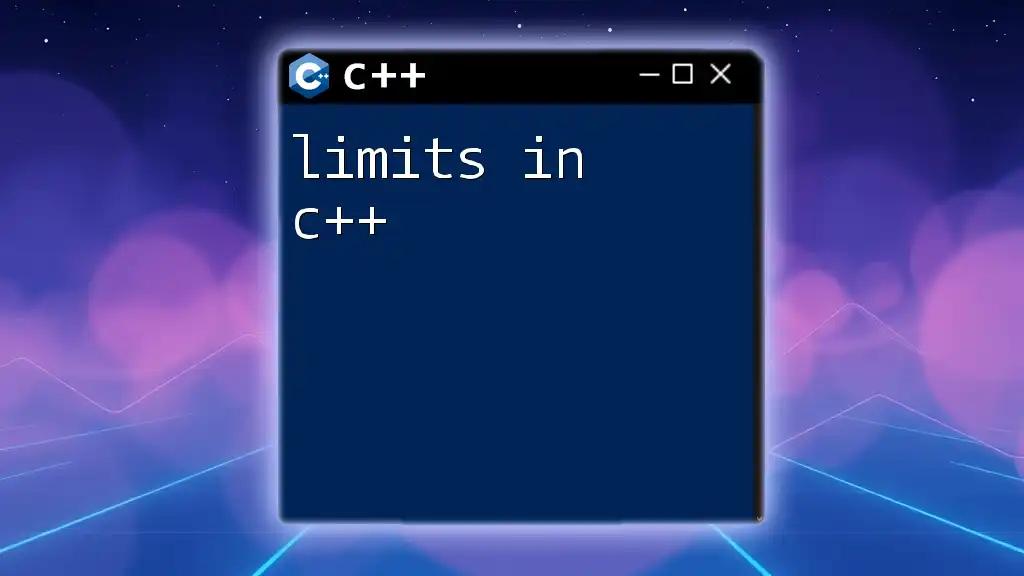
Conclusion
The mathematical functions provided by `math.h in C++` empower developers to perform a wide range of mathematical calculations effortlessly. From basic arithmetic to advanced mathematical operations, leveraging this library can significantly enhance your C++ projects. For those looking to deepen their understanding, continuous exploration of both `math.h` and alternatives like `cmath` is highly recommended. Implementing what you've learned in real-world projects is the best way to solidify your C++ math skills!
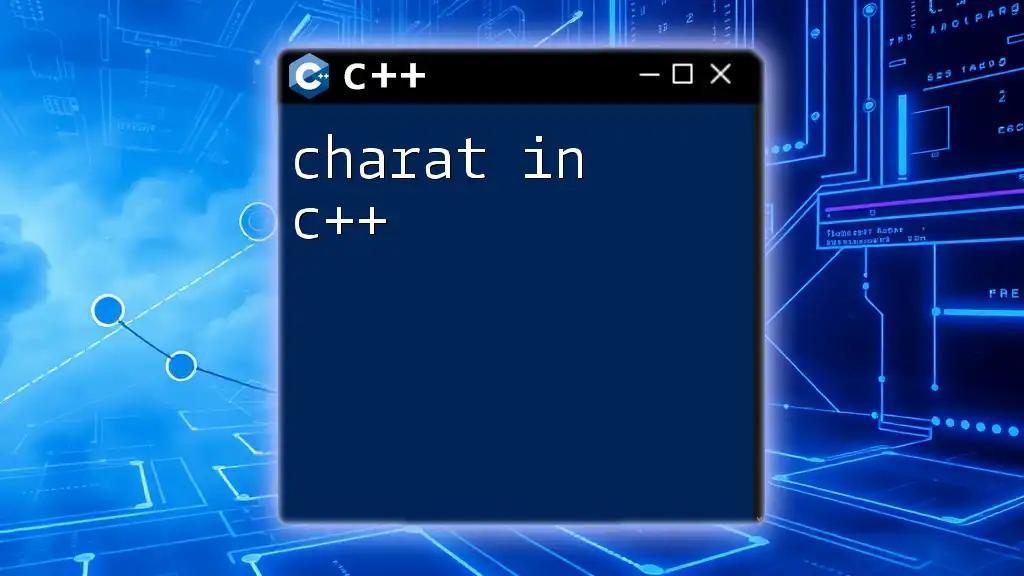
Further Resources
To continue your journey in enhancing your knowledge of `math.h`, consider exploring documentation and tutorials that dive deeper into mathematical principles applied within C++. Engaging with community forums and advancing your skills through practice will further enrich your programming expertise.