In C++, the `at()` function is used to access elements in a container (like vectors or strings) at a specified index, providing bounds checking to prevent out-of-bounds errors.
Here’s a code snippet demonstrating its usage with a vector:
#include <iostream>
#include <vector>
int main() {
std::vector<int> vec = {10, 20, 30, 40, 50};
std::cout << "Element at index 2: " << vec.at(2) << std::endl; // Outputs: 30
return 0;
}
Understanding the `at` Function
What is `at`?
The `at` function is a member function available for various C++ Standard Library containers that facilitates accessing elements. It is explicitly designed to provide a safer alternative to the subscript operator (`[]`). A significant distinction between these two lies in error handling; while `[]` does not perform bounds checking, `at` does, throwing an exception if the specified index is out of range.
Where is `at` Used?
The `at` function is commonly found in several C++ containers, notably:
- `std::vector`
- `std::map`
- `std::array`
- `std::deque`
Using `at` allows developers to maintain robustness and reliability when accessing container elements.
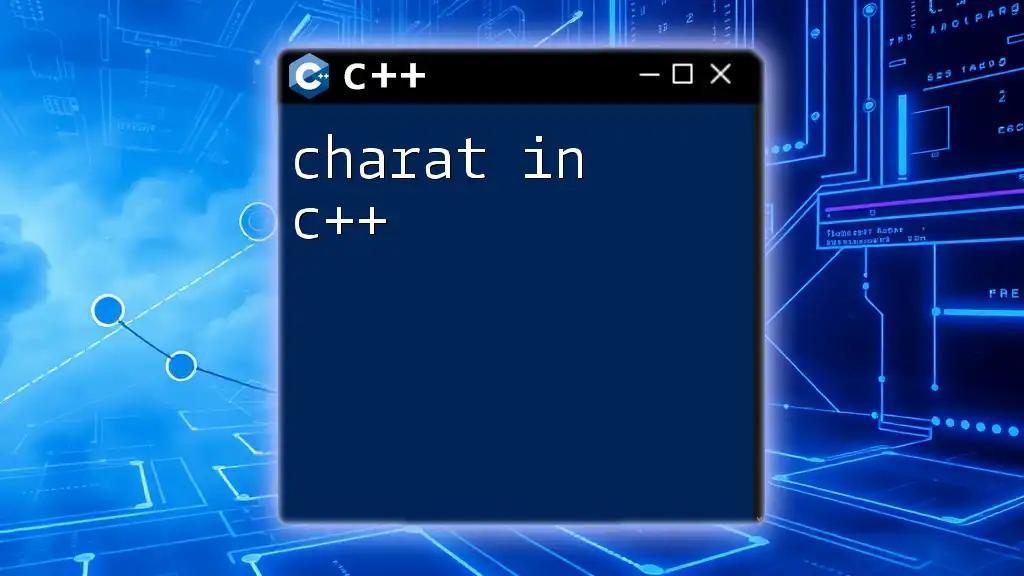
Benefits of Using `at`
Bounds Checking
One of the foremost advantages of using `at` is its bounds checking feature. When you attempt to access an element with `at`, it verifies whether the index is valid. If the index exceeds the container's size limits, `at` will signal this through an exception rather than returning undefined behavior, which is a significant risk with the subscript operator.
Error Handling
The `at` function enhances error handling by throwing a `std::out_of_range` exception. This enables developers to catch and manage errors gracefully. For instance, if your code attempts to access an element that is not present, using `at` would lead to an exception, allowing you to handle the situation appropriately.
Example Code Snippet:
#include <iostream>
#include <vector>
#include <stdexcept>
int main() {
std::vector<int> vec = {1, 2, 3};
try {
std::cout << vec.at(5); // Throws std::out_of_range
} catch (const std::out_of_range& e) {
std::cerr << "Error: " << e.what() << std::endl;
}
return 0;
}
In the example above, attempting to access an index that is out of bounds leads to an exception being thrown, which is caught and reported, thereby avoiding potential crashes or undefined behavior.
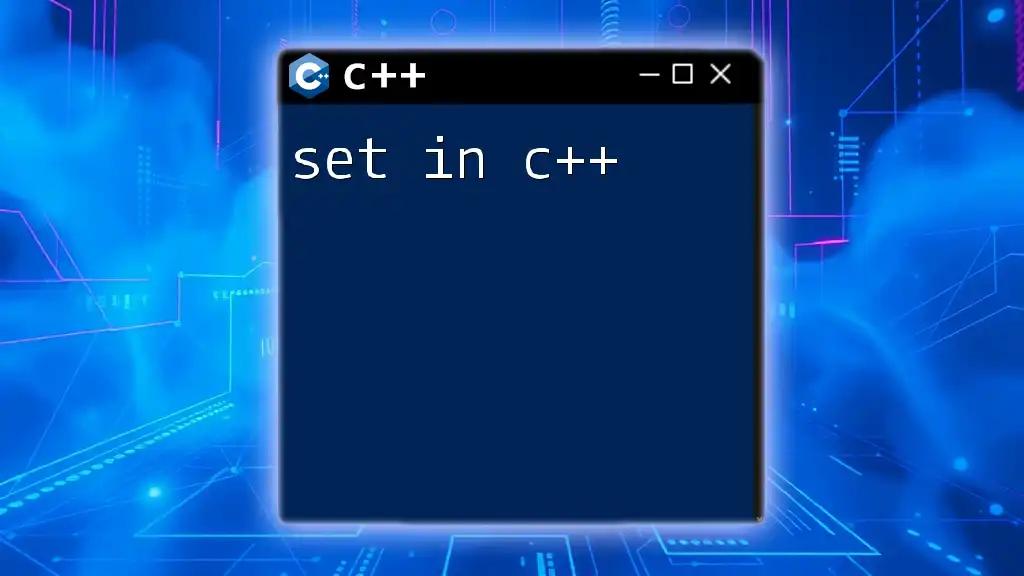
Using `at` with Different C++ Containers
Accessing Elements in `std::vector`
`std::vector` is one of the most commonly used containers in C++. The `at` function allows safe access to elements with the added assurance of bounds checking.
Example Code Snippet:
#include <iostream>
#include <vector>
int main() {
std::vector<int> vec = {1, 2, 3, 4, 5};
std::cout << vec.at(2); // Outputs: 3
return 0;
}
In the example above, calling `vec.at(2)` safely retrieves the third element in the vector, which is `3`.
Accessing Elements in `std::map`
When dealing with associative containers like `std::map`, you can also use `at` to access values based on keys.
Example Code Snippet:
#include <iostream>
#include <map>
int main() {
std::map<std::string, int> myMap = {{"apple", 1}, {"banana", 2}};
std::cout << myMap.at("banana"); // Outputs: 2
return 0;
}
Here, `myMap.at("banana")` retrieves the value associated with the key "banana", demonstrating how `at` can be utilized to access mapping pairs in a type-safe manner.
Usage with `std::array`
`std::array` is another container that supports `at`. It is a wrapper around raw arrays providing better safety and usability.
Example Code Snippet:
#include <iostream>
#include <array>
int main() {
std::array<int, 3> arr = {10, 20, 30};
std::cout << arr.at(1); // Outputs: 20
return 0;
}
In this case, `arr.at(1)` returns `20`, showcasing the straightforward usage of `at` for element access in an `std::array`.
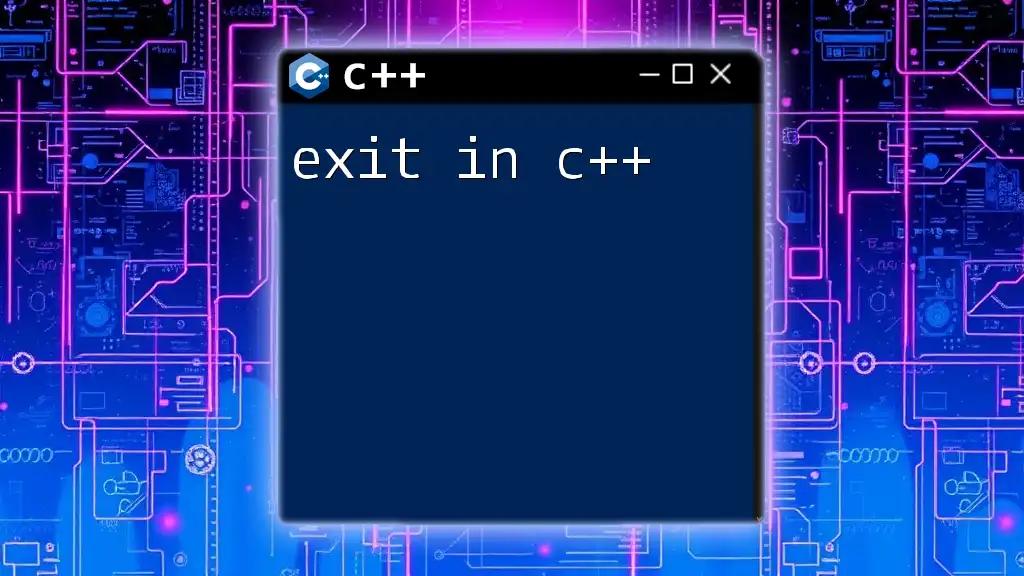
Error Handling with `at`
Exception Types
When using `at`, it is crucial to understand the `std::out_of_range` exception it may throw. This exception signals that the index provided exceeds the allowable limits of the container. Handling this exception is vital for robust software development.
Properly managing exceptions can greatly enhance the reliability of your code. Using `try-catch` blocks helps you maintain program stability in the face of potential run-time errors.

Common Pitfalls with `at`
Incorrect Indexing
One potential pitfall is incorrect indexing, where developers accidentally provide an index that is negative or exceeds the size of the container. This can lead to exceptions being thrown, interrupting the flow of the program. Always ensure that the index is within valid bounds before calling `at`.
Performance Overhead
While `at` provides safety in accessing elements, it introduces a performance overhead due to bounds checking. This becomes more apparent in intensive applications where multiple accesses are made in a loop. Hence, it is crucial to evaluate whether the benefits of using `at` outweigh the potential performance costs for your specific scenario.

When to Use `at` vs `[]`
When deciding between `at` and `[]`, consider the following:
- Use `at` when: You prioritize safety and need bounds checking, especially in scenarios where out-of-bounds access can lead to severe bugs.
- Use `[]` when: Performance is of utmost importance, and you can guarantee through logic that the index will always be valid.

Conclusion
The `at` function in C++ serves as a crucial tool for safe element access within various containers. Its ability to perform bounds checking and throw exceptions not only enhances robustness but also aids in maintaining the integrity of your programs. By understanding when and how to use `at`, you can create safer and more reliable applications.

Additional Resources
For further exploration of the `at` function, the following resources are recommended:
- The official C++ documentation for `std::vector`, `std::map`, and `std::array`.
- Books and online classes focused on modern C++ practices to deepen your understanding of container management.
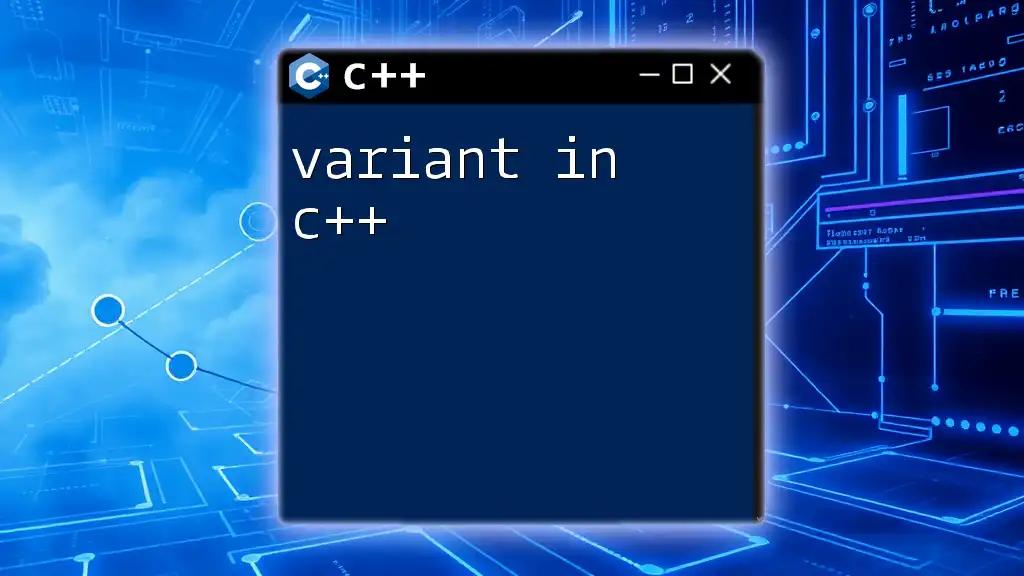
Call to Action
We encourage you to practice using the `at` function in your C++ projects, experimenting with various containers. If you have further questions or experiences to share, please feel free to engage in the comments section.