In C++, the `/t` command is often used in console applications to add tab spaces in formatted output, enhancing the readability of the displayed text.
Here’s a simple code snippet demonstrating its use with escape sequences for tab:
#include <iostream>
int main() {
std::cout << "Name\tAge\tLocation\n";
std::cout << "Alice\t30\tNew York\n";
std::cout << "Bob\t25\tLos Angeles\n";
return 0;
}
What is /t in C++?
Understanding the Role of the `/t` Character
In C++, the character combination `/t` does not exist as a standalone construct. However, the escape sequence `\t` plays a crucial role in formatting output on the console. Knowing the difference is essential as `/t` might be a typographical error when referring to the tab character `\t`. This discussion centers around `\t`, the tab character used in string literals to create horizontal space in output.
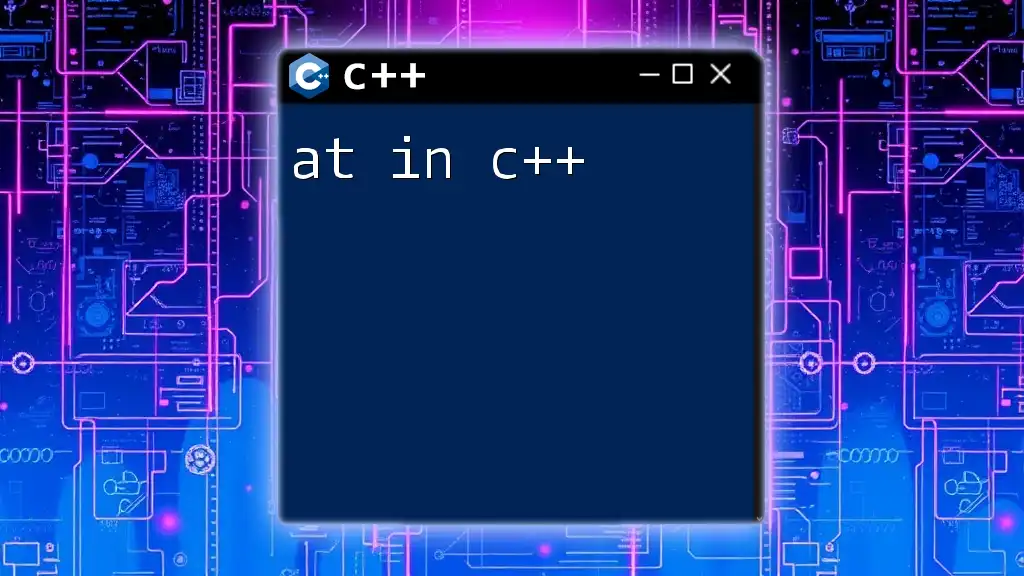
The Significance of `\t` in C++
What Does `\t` Stand For?
The `\t` character represents an escape sequence in C++. Escape sequences are used to incorporate special characters in strings, ones that cannot be typed directly. The `\t` specifically stands for a horizontal tab, and it is used to align text nicely in console output. Using `\t` can greatly enhance the legibility of data when outputting structured or tabular information.
Why Use `\t` in Your Code?
Utilizing `\t` has several advantages:
- Improving Code Readability: By adding tabs, your output can maintain proper alignment, making it easier for users to read.
- Tabular Data Formatting: When dealing with lists or tables, including tab spaces ensures that columns are visually distinguishable.
- Use Cases in Console Output: This character is often employed in situations where formatted output is necessary, such as displaying the results of calculations or processing data.
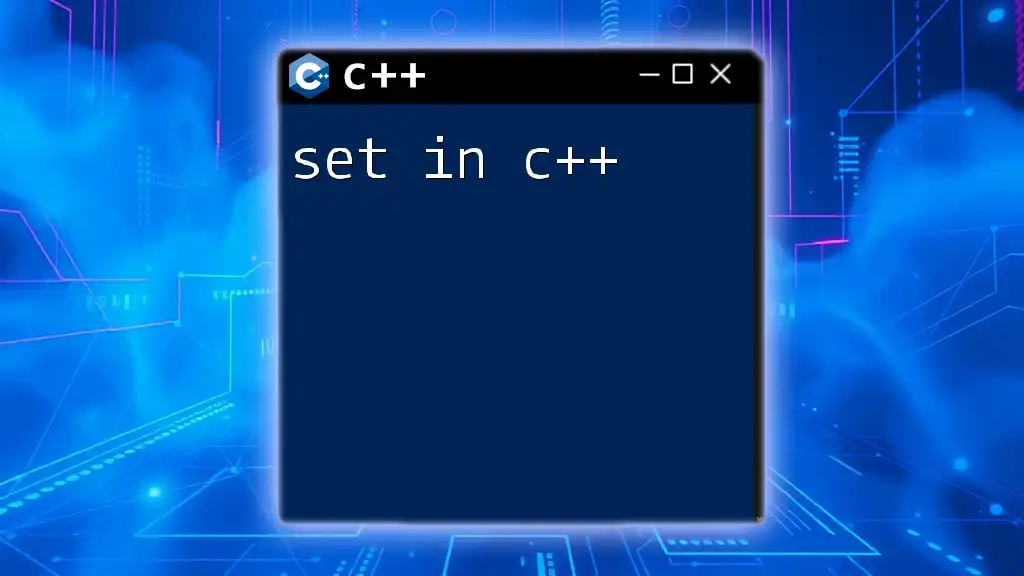
Implementing `\t` in C++: Code Examples
Basic Usage of `\t`
Implementing `\t` is straightforward. Here’s a basic example:
#include <iostream>
using namespace std;
int main() {
cout << "Name\tAge\tLocation" << endl;
cout << "Alice\t30\tNew York" << endl;
cout << "Bob\t25\tLos Angeles" << endl;
return 0;
}
In this snippet, `\t` is used to create a tabbed format between the headings "Name," "Age," and "Location". Each row aligns neatly underneath these headings, illustrating how `\t` can enhance readability effectively.
Combining `\t` with Other Escape Sequences
Combining `\t` with other escape sequences, such as `\n` (newline), allows even greater control over formatting. Consider this example:
#include <iostream>
using namespace std;
int main() {
cout << "Item\tPrice\n";
cout << "Apple\t$1.00\n";
cout << "Banana\t$0.50\n";
return 0;
}
Here, `\n` is used to move to a new line after each item in the list, while `\t` ensures that the price is vertically aligned. This combination of newline and tab creates a vertical layout that is both professional and easy to read.
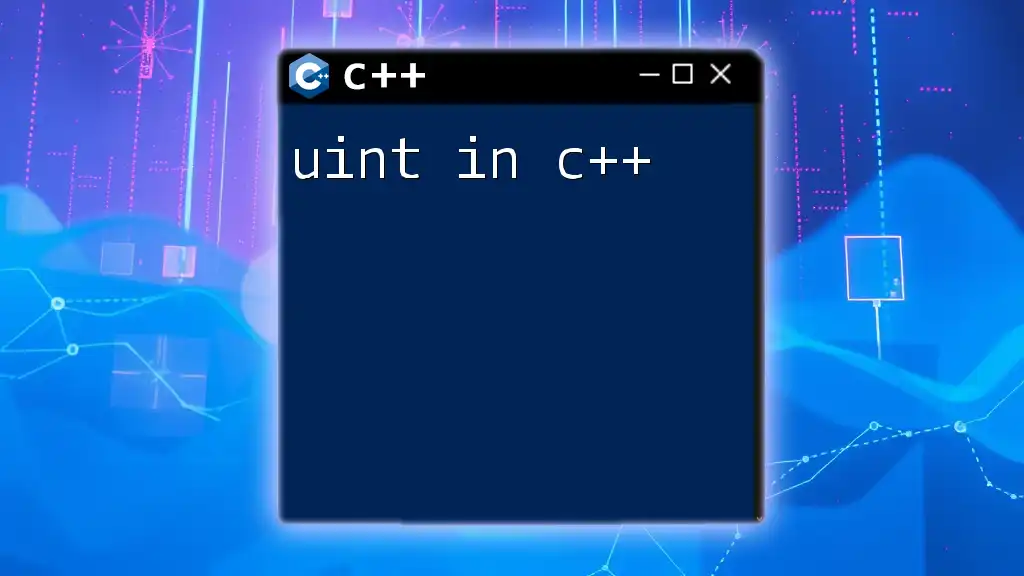
Common Mistakes with Using `\t`
Misinterpretations of Tab Characters
One of the most significant pitfalls when using `\t` involves how different editors display tabs. Some editors use spaces instead of actual tab characters, leading to inconsistent formatting when the code is viewed in different environments. It is critical to ensure that tabs are used consistently throughout your codebase for alignment.
When Not to Use `\t`
While `\t` provides many advantages, there are scenarios where its use may be inappropriate. For instance, in fixed-width formatting projects like ASCII art or when precise visual representation is required, using spaces may offer better control over the layout. This approach eliminates variability across different systems or editors.
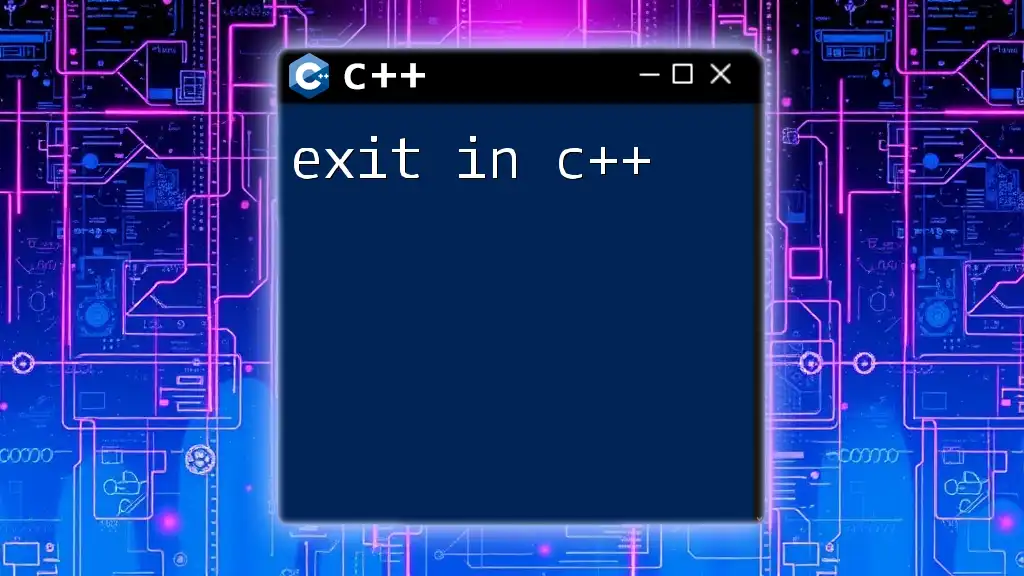
Tips for Using `\t` in C++ Applications
Best Practices for Readability
To maximize the effectiveness of `\t`, adhering to best practices in code formatting is vital. Always maintain consistent use of tabs or spaces across your entire codebase. This not only enhances readability for yourself but also for any collaborators who may work on the project.
Compatibility across Different Platforms
Tab settings can differ significantly between operating systems. Therefore, maintaining uniformity when distributing your code across multiple environments becomes critical. To ensure the output remains consistent, familiarize yourself with the tab configurations of the various platforms you're targeting.
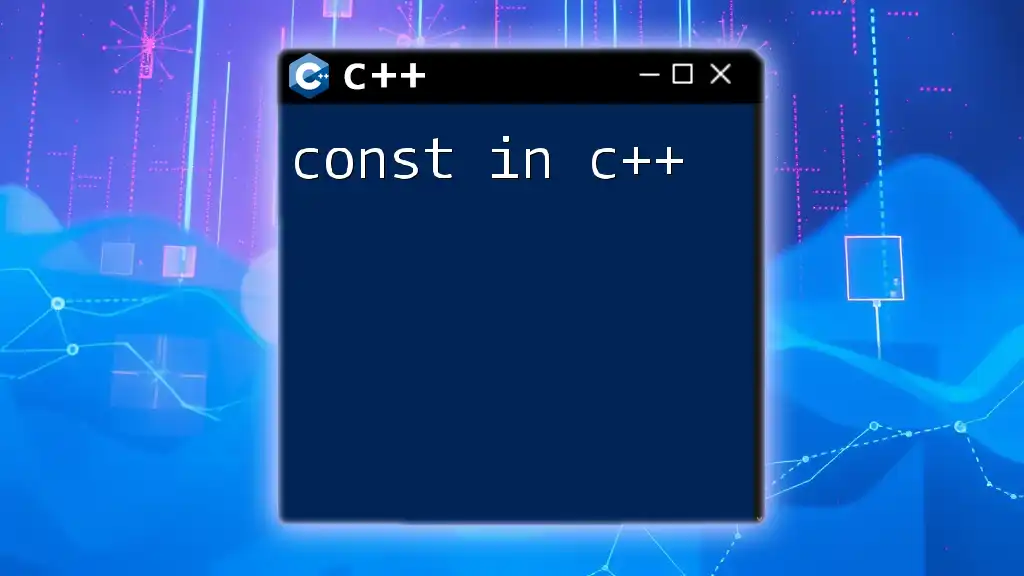
Conclusion
Recap of Key Takeaways
In summary, the `\t` character in C++ serves as a powerful tool for enhancing the formatting and readability of console output. By implementing it correctly, developers can improve the presentation of data, making it more accessible and user-friendly.
Encouragement to Experiment
Now that you have learned about `\t`, I encourage you to experiment with it in your coding projects. Try implementing different formatting strategies and share your experiences or queries on effectively using `\t` to create organized output.