In C++, `INT_MAX` is a macro defined in the `<limits.h>` header that represents the maximum value an `int` can hold, which is useful for initializing variables to a value that ensures comparisons work correctly.
Here's a simple code snippet demonstrating its use:
#include <iostream>
#include <limits.h>
int main() {
int maxInt = INT_MAX;
std::cout << "The maximum value of an int is: " << maxInt << std::endl;
return 0;
}
What is int_max in C++?
In C++, `int_max` represents the maximum value that can be stored in an integer data type. Understanding this value is vital for developers to handle numerical limits effectively, ensuring that their programs avoid overflow errors and maintain data integrity.
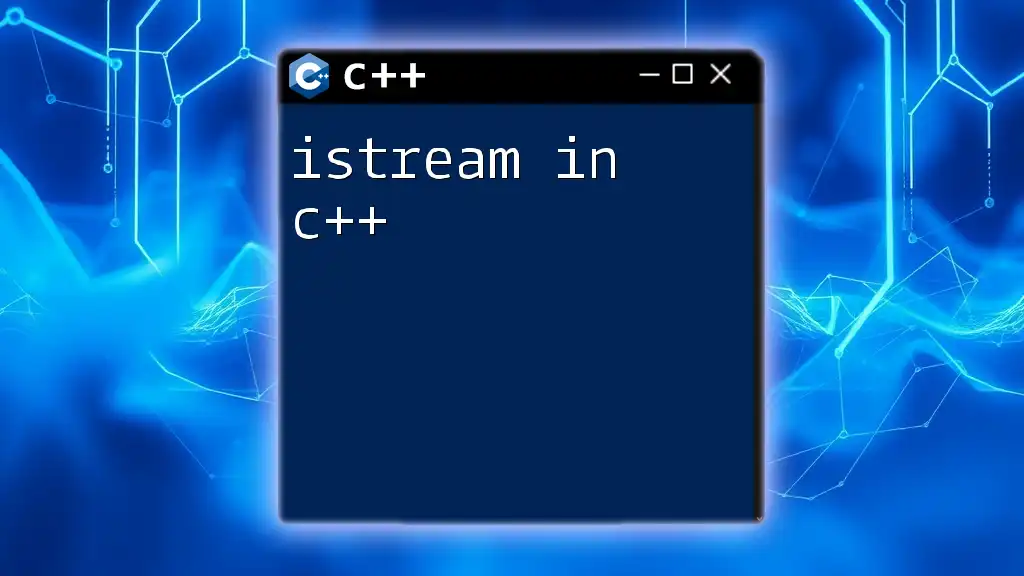
Understanding the Integer Limits
C++ int_max
The `int_max` constant defines the upper limit for integer values. It can be accessed through the `<limits>` header. The typical maximum value for an integer in C++ is 2,147,483,647 (or 2^31 - 1 on most platforms using 32-bit integers). This value signifies that any integer value above this threshold will lead to integer overflow, resulting in undefined behavior or unexpected results in your programs.
C++ int_min
Similarly, `int_min` signifies the minimum value an integer can hold, commonly defined as -2,147,483,648 (or -2^31). Being aware of this limit is essential, particularly when dealing with operations that might produce a value less than the minimum representable integer.
Comparison of int_max and int_min
Understanding both `int_max` and `int_min` helps developers write more robust code. For example, knowing the limits ensures safe arithmetic operations and proper handling of edge cases in algorithms, thus preventing critical errors in calculations. For instance, using these constants can help in implementing algorithms where the highest or lowest integer values are frequently referenced.
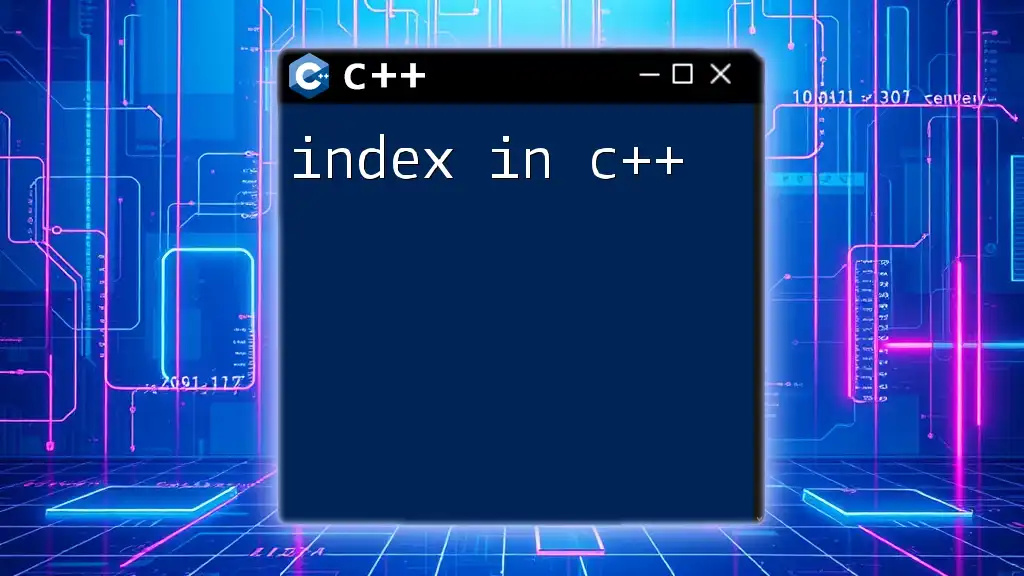
Using int_max in C++
Accessing int_max
To access `int_max`, you will need to include the `<limits>` header. Here’s how you can easily print the value of `int_max`:
#include <limits>
#include <iostream>
int main() {
std::cout << "The maximum value of int is: " << std::numeric_limits<int>::max() << std::endl;
return 0;
}
In this example, the `std::numeric_limits<int>::max()` call retrieves the maximum value of the integer data type, which is then printed to the console. This simple approach helps in understanding the bounds of integer values in C++.
Practical Use Cases for int_max
There are numerous scenarios where knowing the value of `int_max` becomes crucial. For instance, in algorithms that identify the maximum element in an array, initializing the maximum value to `int_max` can lead to incorrect results. Instead, starting with the minimum possible integer value ensures that all possible integers will be compared accurately.
Consider the following code snippet that demonstrates finding the maximum element in an array:
int findMax(int arr[], int size) {
int max = std::numeric_limits<int>::min(); // Start with the smallest possible integer.
for (int i = 0; i < size; i++) {
if (arr[i] > max) {
max = arr[i]; // Update max if the current element is greater.
}
}
return max;
}
This function initializes `max` with `int_min`, allowing all integers in the array to be appropriately considered, thereby avoiding the pitfalls of comparisons that may mistakenly include `int_max`.
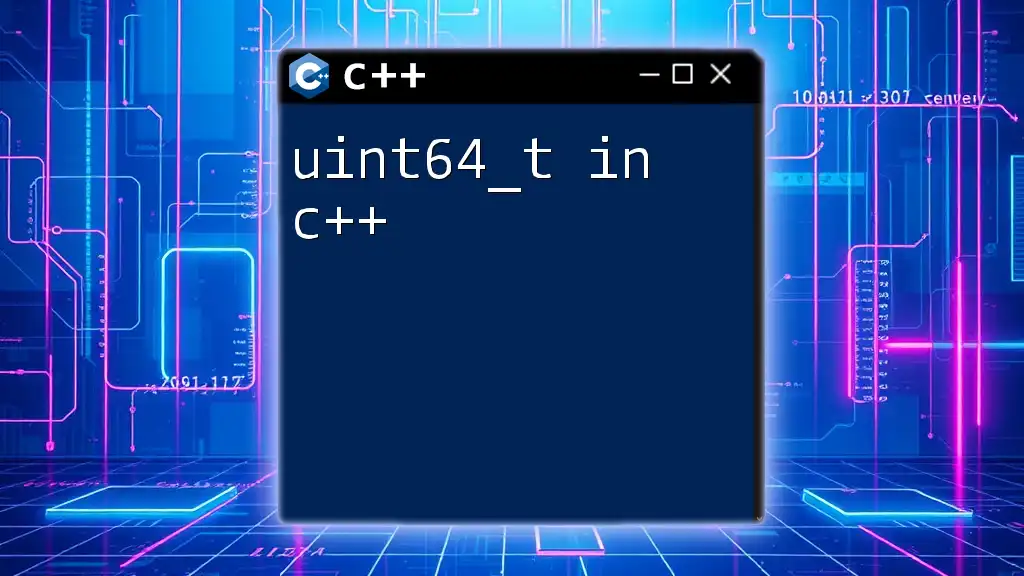
Handling Overflows with int_max
What is Integer Overflow?
Integer overflow occurs when an arithmetic operation exceeds the maximum limit a data type can handle, causing it to wrap around to the negative range. This can lead to bugs that are often hard to detect and diagnose, as the resultant value is typically invalid or unexpected.
Preventing Overflow with int_max
By using `int_max`, developers can implement checks in their arithmetic operations to prevent overflow situations. A safe approach when performing addition is to verify that the resultant value does not exceed `int_max`. Here’s an example function demonstrating this concept:
int safeAddition(int a, int b) {
if (a > 0 && b > 0 && a > std::numeric_limits<int>::max() - b) {
// Handle overflow case here, presumably by logging or returning an error code
std::cerr << "Overflow detected!" << std::endl;
return -1; // Indicate error
}
return a + b; // Safe to perform addition
}
In this function, the check `a > std::numeric_limits<int>::max() - b` ensures that adding `b` to `a` won't exceed `int_max`. If it does, the function throws an error and avoids the overflow, maintaining data integrity.
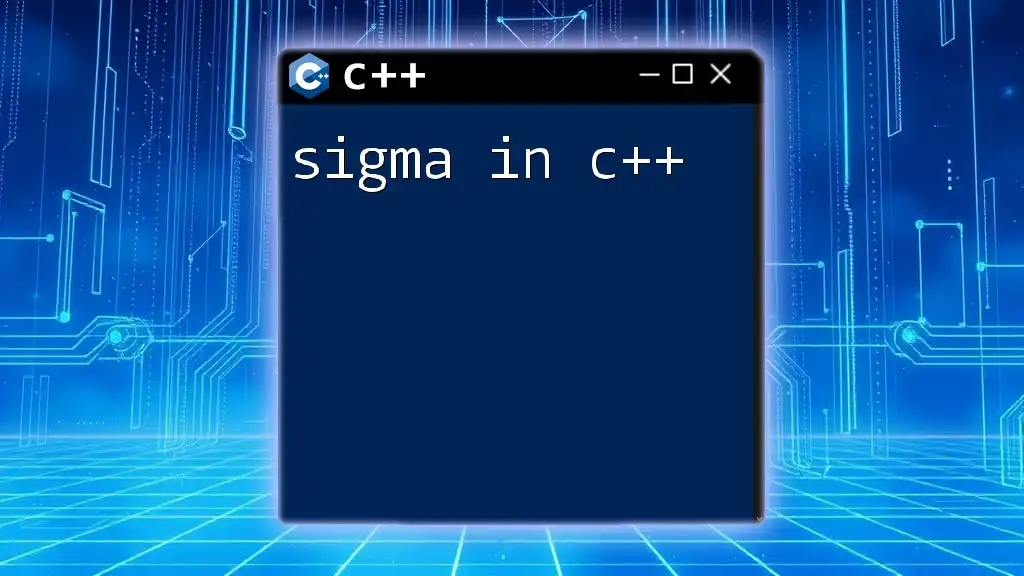
Summary of Key Points
In summary, understanding `int_max` in C++ is crucial for writing robust and safe code. It allows developers to effectively manage integer boundaries and prevents the unintended consequences of overflow. By using `int_max` and its counterpart `int_min`, programmers can create algorithms that handle edge cases gracefully and maintain correctness in calculations.
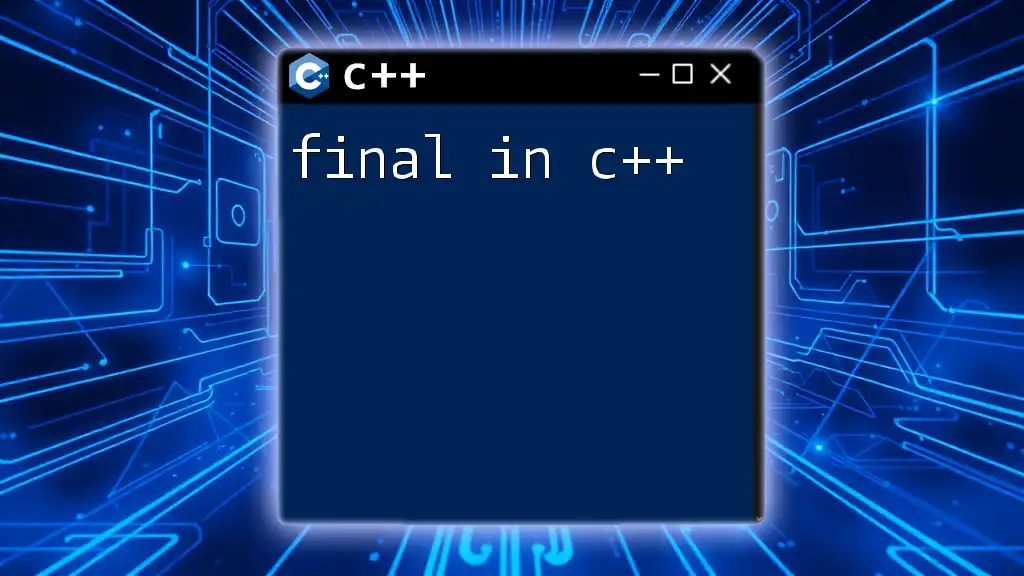
Conclusion
Grasping the concept of `int_max` is vital for any C++ programmer working with integer data types. This understanding helps in building reliable applications that behave predictably and minimizes the potential for errors due to overflow. By incorporating safe coding practices around integer limits, programmers can ensure that their applications remain resilient and efficient.
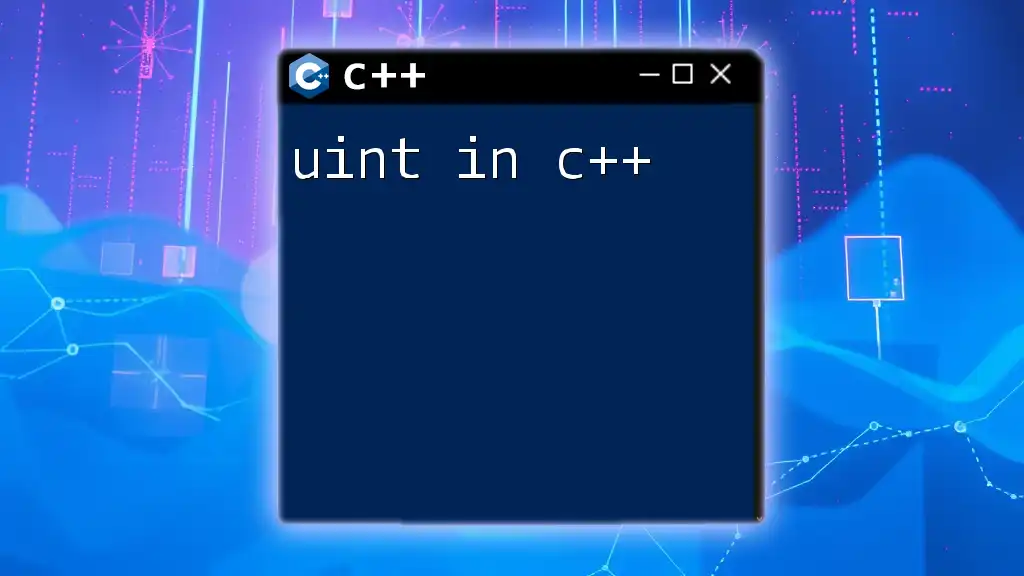
Additional Resources
For further reading, consider exploring resources that delve deeper into C++ integer types and their limits, as well as tutorials focusing on overflow management and safe programming techniques. These materials will reinforce your understanding and help sharpen your C++ programming skills.