In C++, a matrix can be represented as a two-dimensional array where elements are stored in rows and columns, enabling easy manipulation and access of data.
Here's a simple code snippet to illustrate how to declare and initialize a 3x3 matrix:
#include <iostream>
int main() {
int matrix[3][3] = {
{1, 2, 3},
{4, 5, 6},
{7, 8, 9}
};
// Displaying the matrix
for (int i = 0; i < 3; i++) {
for (int j = 0; j < 3; j++) {
std::cout << matrix[i][j] << " ";
}
std::cout << std::endl;
}
return 0;
}
What is a Matrix in C++?
In the context of C++, a matrix is essentially a two-dimensional array of numbers arranged in rows and columns. It serves as a powerful data structure capable of representing various types of data and performing numerous operations relevant to a multitude of applications.
The different types of matrices such as square matrices (where the number of rows is equal to the number of columns) and rectangular matrices (where this condition doesn't hold) are fundamental concepts in linear algebra, heavily utilized in algorithms, graphics calculations, game development, and more.
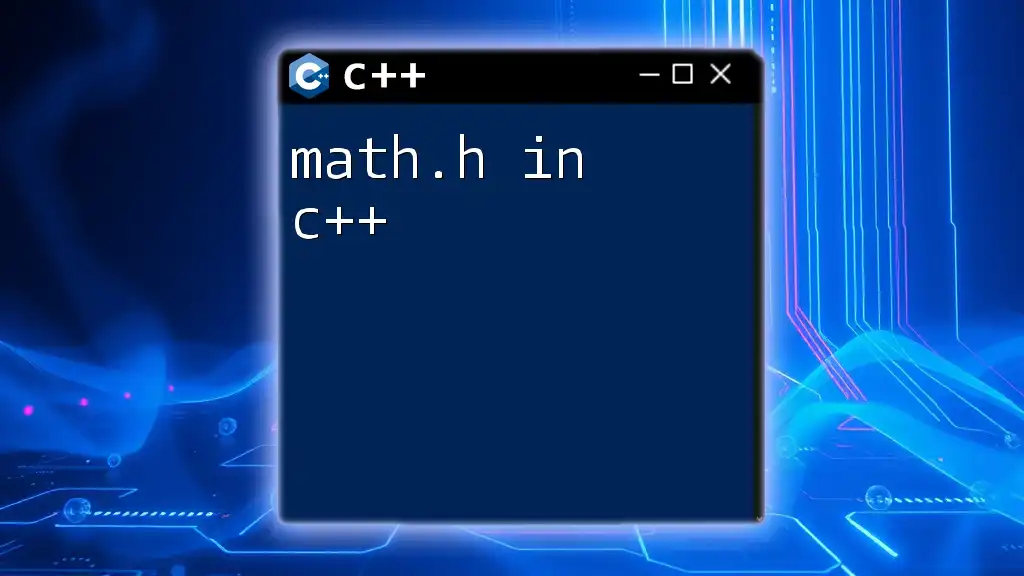
Creating a Matrix Using Arrays
Static arrays are one common way to create a matrix in C++. When you know the dimensions of your matrix beforehand, static arrays are straightforward and efficient to use.
For example, the following code demonstrates how to declare and initialize a 3x3 matrix using static arrays:
int matrix[3][3] = { {1, 2, 3}, {4, 5, 6}, {7, 8, 9} };
In this example, `matrix` is a two-dimensional array containing integers arranged in three rows and three columns. However, static arrays can be limiting due to their fixed size, prompting some developers to prefer dynamic alternatives, such as vectors.
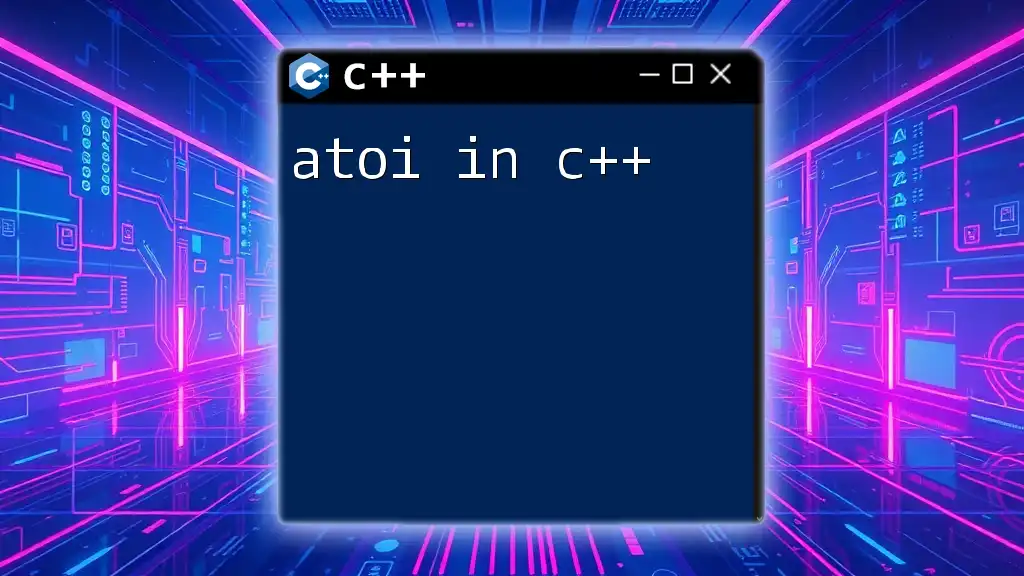
Creating a Matrix Using Vectors
Using vectors offers flexibility in defining matrix dimensions at runtime, helping avoid inefficiencies associated with static arrays. Here's how to create a matrix in C++ utilizing a vector of vectors:
#include <vector>
std::vector<std::vector<int>> matrix(3, std::vector<int>(3, 0)); // 3x3 matrix initialized to 0
In this snippet, we declare a 3x3 matrix initialized with zeroes. Vectors allow resizing and offer built-in methods for easier management of the data stored within the matrix, making them a preferred choice for many C++ developers.
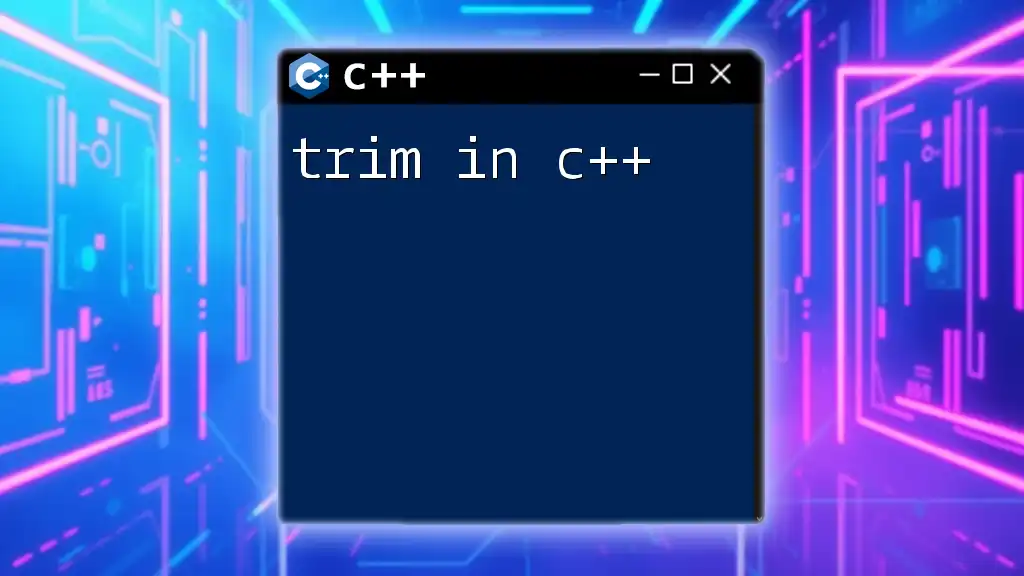
Common Matrix Operations
Matrix Addition
Matrix addition is a fundamental operation where two matrices of the same dimensions are added together element-wise. The following code snippet illustrates how to add two matrices in C++:
std::vector<std::vector<int>> addMatrices(const std::vector<std::vector<int>>& A, const std::vector<std::vector<int>>& B) {
std::vector<std::vector<int>> result(A.size(), std::vector<int>(A[0].size()));
for (size_t i = 0; i < A.size(); ++i)
for (size_t j = 0; j < A[0].size(); ++j)
result[i][j] = A[i][j] + B[i][j];
return result;
}
In this snippet, we loop through each row and column of matrices A and B, summing corresponding elements to form the resultant matrix. This operation is frequently utilized in graphics and data manipulation applications.
Matrix Multiplication
Matrix multiplication goes beyond simple element addition and involves calculating the dot product of rows and columns. Note that matrix A's number of columns must equal the number of rows of matrix B for multiplication to occur. The following code performs matrix multiplication in C++:
std::vector<std::vector<int>> multiplyMatrices(const std::vector<std::vector<int>>& A, const std::vector<std::vector<int>>& B) {
size_t rowsA = A.size(), colsA = A[0].size(), colsB = B[0].size();
std::vector<std::vector<int>> result(rowsA, std::vector<int>(colsB, 0));
for (size_t i = 0; i < rowsA; ++i)
for (size_t j = 0; j < colsB; ++j)
for (size_t k = 0; k < colsA; ++k)
result[i][j] += A[i][k] * B[k][j];
return result;
}
In this code, nested loops calculate the resulting matrix's elements by looping through each element of rows from A and columns from B. This operation is widely used in scientific computations, machine learning applications, and gaming.
Transposing a Matrix
Transposing a matrix means flipping it over its diagonal, effectively turning rows into columns and vice versa. Below is the code to transpose a matrix:
std::vector<std::vector<int>> transposeMatrix(const std::vector<std::vector<int>>& matrix) {
std::vector<std::vector<int>> transposed(matrix[0].size(), std::vector<int>(matrix.size()));
for (size_t i = 0; i < matrix.size(); ++i)
for (size_t j = 0; j < matrix[0].size(); ++j)
transposed[j][i] = matrix[i][j];
return transposed;
}
This code snippet will create a new matrix where the rows of the original matrix become the columns in the transposed version. Transposing is often essential in mathematical computations and algorithms such as the Gaussian elimination method or when working with certain machine learning models.
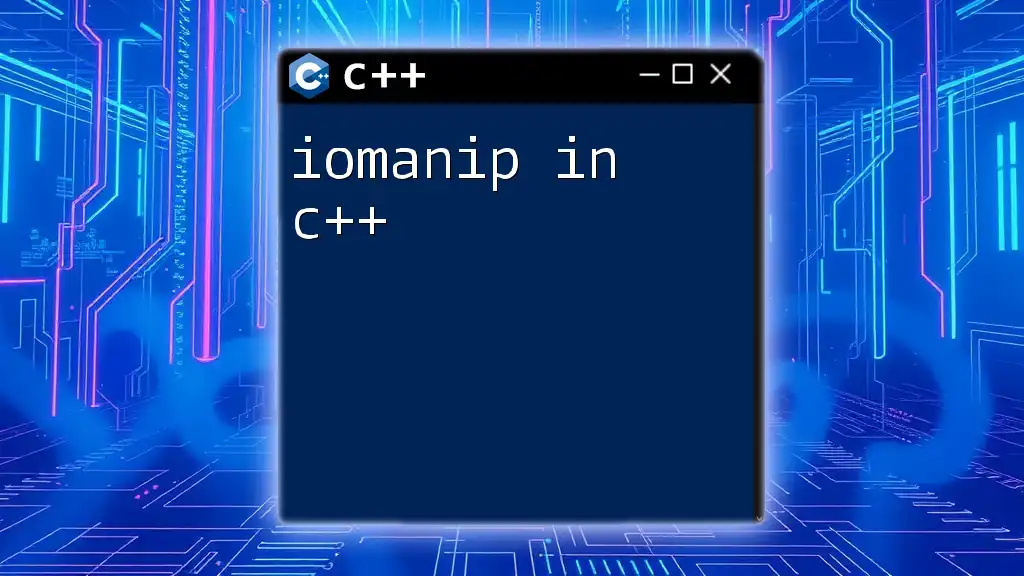
Advanced Matrix Concepts
Dynamic Matrix Allocation
Using pointers is another advanced method for creating matrices when sizes are not known at compile time. Here’s how you can allocate a matrix dynamically:
int** createMatrix(int rows, int cols) {
int** matrix = new int*[rows];
for (int i = 0; i < rows; ++i)
matrix[i] = new int[cols];
return matrix;
}
In this snippet, we dynamically allocate memory on the heap for a matrix. However, be cautious to delete the allocated matrices after use to prevent memory leaks, emphasizing the importance of memory management in C++ programming.
Error Handling with Matrices
As with any programming operation, error handling is crucial in matrix operations to avoid crashes from mismatched dimensions or other issues. Implementing checks for matrix dimensions before performing operations, such as addition or multiplication, is a best practice. For instance:
if (A[0].size() != B.size()) {
throw std::invalid_argument("Incompatible matrix dimensions for multiplication");
}
This check helps ensure your code is robust and can handle unexpected input gracefully.
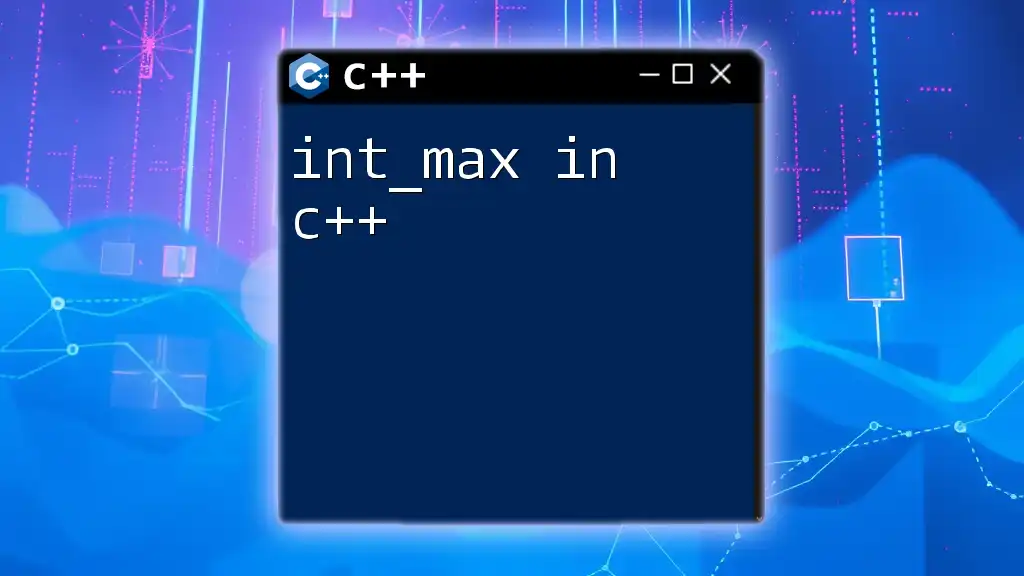
Practical Applications of Matrices
Matrices in Graphics Programming
In graphics programming, matrices are employed extensively for transformations such as scaling, rotation, and translation of objects within a scene. These transformations fundamentally rely on matrix multiplication, allowing complex manipulations of graphical elements to be performed efficiently.
Matrices in Machine Learning
Matrices serve as the backbone for representing data in machine learning algorithms. From feature vectors to training datasets, understanding how to manipulate matrices becomes essential for anyone looking to dive into machine learning. Operations like matrix multiplication and transposition are often used in constructing neural networks, optimizing models, and processing training data.
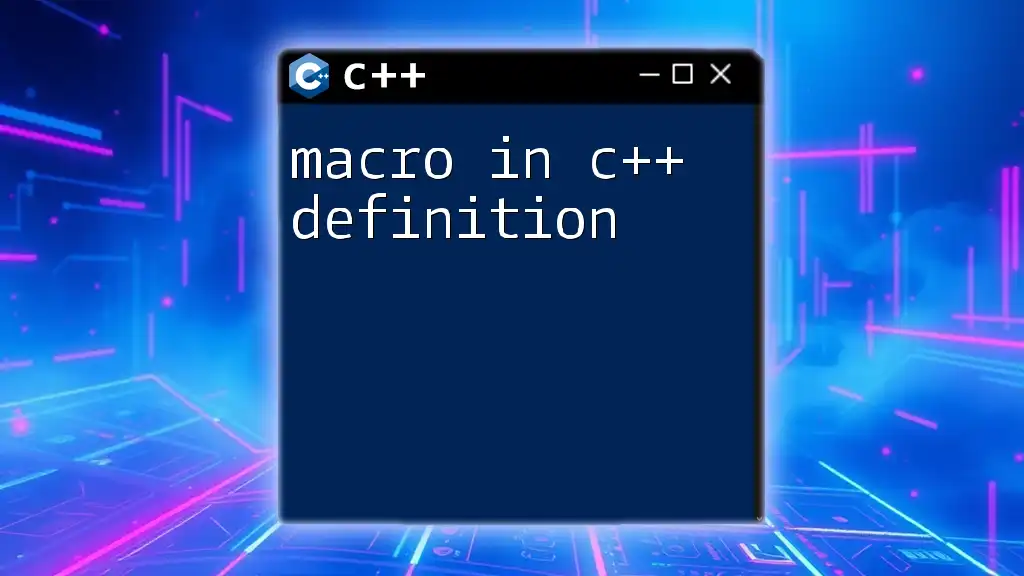
Conclusion
Understanding matrices and their operations in C++ opens up a myriad of possibilities for developers, from scientific computing to graphics and machine learning. By mastering the concepts explored in this guide, you’ll be well-equipped to leverage the power of matrices in your applications.
Now is the perfect time to practice these concepts through hands-on coding projects, ensuring that your skills with the matrix in C++ continue to grow. Explore our lessons to deepen your understanding and become proficient in C++ programming!
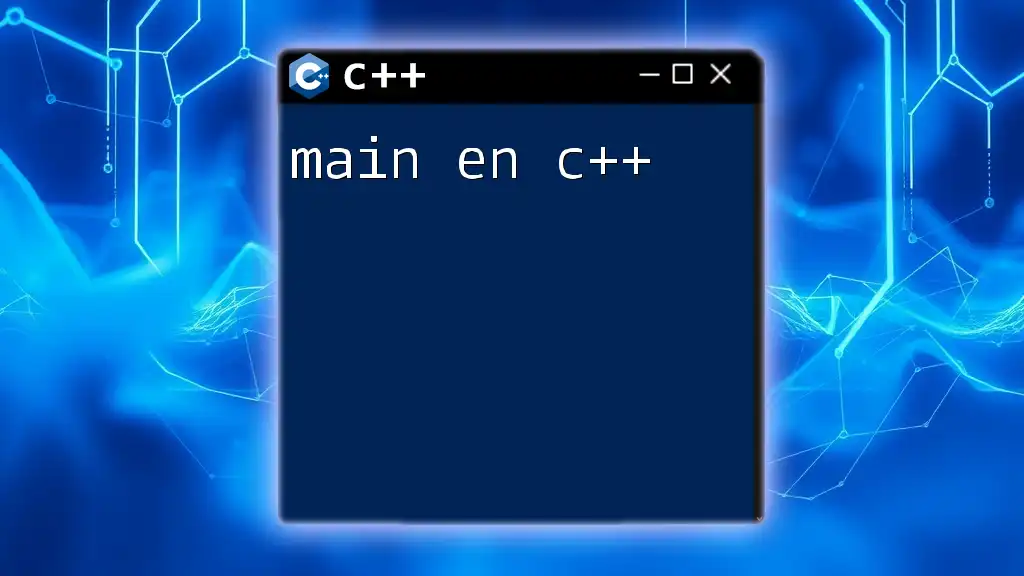
Additional Resources
For those seeking to enhance their knowledge of matrices in C++, consider exploring recommended books, websites, and courses that cover both fundamental and advanced topics. Engaging with these materials will solidify your skills and empower your programming journey.