`std::copy` is a function in C++ that copies a range of elements from one location to another, requiring iterators to specify the source and destination ranges.
Here’s a simple code snippet demonstrating its use:
#include <iostream>
#include <vector>
#include <algorithm>
int main() {
std::vector<int> source = {1, 2, 3, 4, 5};
std::vector<int> destination(5);
std::copy(source.begin(), source.end(), destination.begin());
for (int num : destination) {
std::cout << num << " ";
}
return 0;
}
Understanding std::copy
Definition and Purpose
std::copy is a powerful algorithm provided by the C++ Standard Library that facilitates the copying of elements from one range to another. Its primary purpose is to enable efficient and straightforward duplication of data when handling collections, such as arrays or containers.
By utilizing this utility, programmers can manage memory better and reduce the risk of errors associated with manual copying. Whether you’re transferring elements from a vector to an array, duplicating content in a data structure, or simply reshaping datasets, `std::copy` serves as a go-to method for maintaining data integrity and efficiency.
Key Features
One of the features that makes `std::copy` an essential part of the C++ programmer's toolkit is that it is a template function. This means it can accept various data types, making it flexible and applicable across different scenarios.
Furthermore, `std::copy` relies on input and output iterators, which are a crucial aspect of how the algorithm operates. It allows data to be copied without requiring the underlying data structure to be revealed, therefore promoting encapsulation and reducing coupling between components.
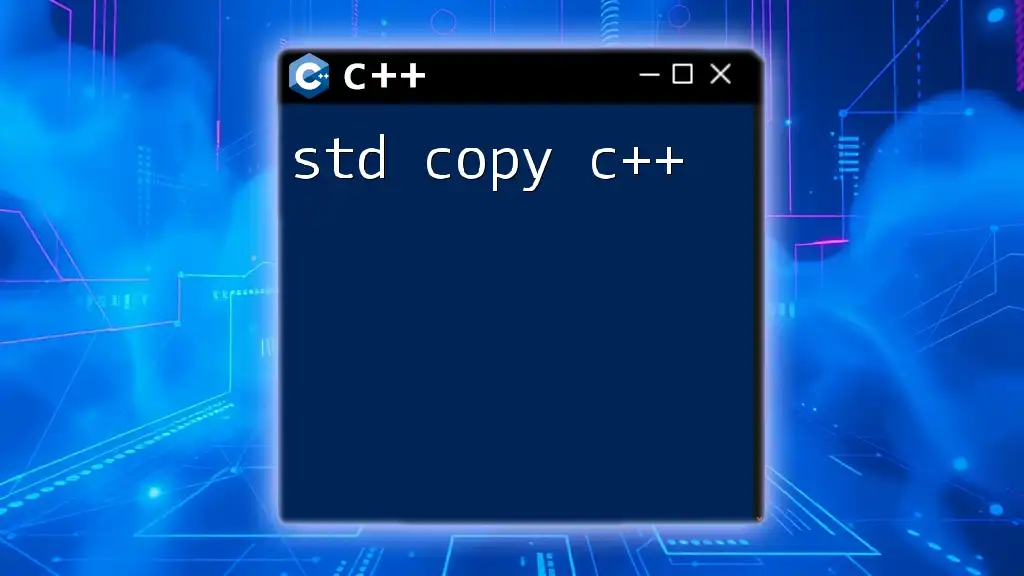
Syntax of std::copy
General Syntax
The syntax for using `std::copy` is straightforward:
std::copy(InputIterator first, InputIterator last, OutputIterator result);
- Parameters explanation:
- InputIterator first: This marks the start of the source range.
- InputIterator last: This parameter indicates the end of the source range.
- OutputIterator result: This defines where the copied elements should be placed in the destination range.
Example Syntax Usage
To see how `std::copy` works in practice, consider the following example that demonstrates copying elements from one vector to another:
#include <iostream>
#include <vector>
#include <algorithm>
int main() {
std::vector<int> source = {1, 2, 3, 4, 5};
std::vector<int> dest(source.size());
std::copy(source.begin(), source.end(), dest.begin());
// Outputting copied vector
for (const auto& elem : dest) {
std::cout << elem << " ";
}
return 0;
}
In this example, we initialize a source vector containing a list of integers and an empty destination vector of the same size. By invoking `std::copy`, we transfer the elements, which then allows us to print them out.
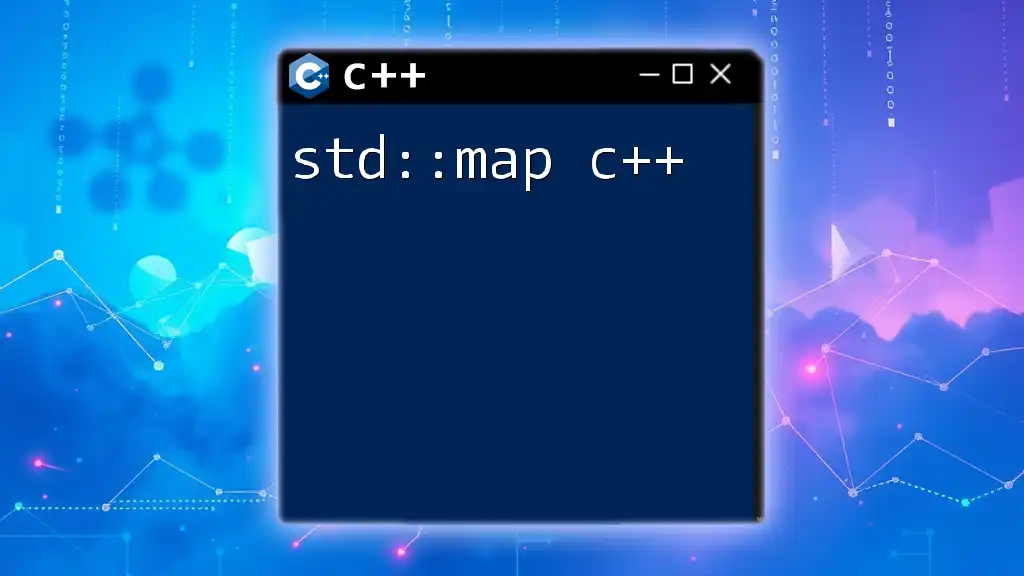
Invoking std::copy
Basic Example
In the previous example, we demonstrated how simple it is to copy contents between two vectors. The key takeaway is that by providing the begin and end iterators of the source vector, along with the begin iterator of the destination vector, we are able to efficiently duplicate elements without writing explicit loops or indices.
Copying Between Different Containers
You can also use `std::copy` to transfer data between different types of containers. For instance, consider copying data from a `std::vector` to a `std::array`:
#include <array>
std::vector<int> vec = {10, 20, 30};
std::array<int, 3> arr;
std::copy(vec.begin(), vec.end(), arr.begin());
In this code snippet, we create a vector containing integers and then declare a fixed-size array to hold the copied values. The `std::copy` function efficiently transfers data while ensuring that the types remain compatible.
Handling Overlapping Ranges
The Problem of Overlapping Ranges
When using `std::copy`, dealing with overlapping ranges can lead to undefined behavior. It’s essential to ensure that the input and output ranges do not overlap. This is a major consideration when manipulating arrays or other contiguous data structures.
Using std::copy with Overlapping Ranges
Despite this potential issue, there are situations where overlapping requires careful handling. If you find yourself needing to copy parts of a vector into another part of itself, consider using `std::copy` judiciously:
std::vector<int> data = {1, 2, 3, 4, 5};
std::copy(data.begin(), data.begin() + 3, data.begin() + 2);
In this scenario, we copy the first three elements of the `data` vector into a partially overlapping region. As a result, the content of the vector effectively becomes `{1, 2, 1, 2, 3}`. Note that in cases like this, ensuring that the destination does not interfere with the source values is critical.
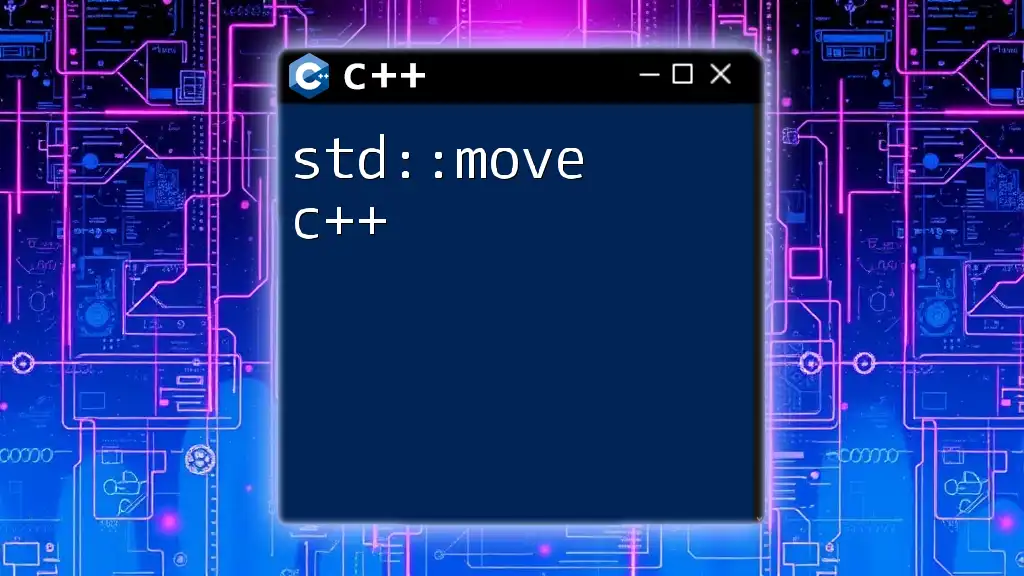
Alternatives to std::copy
std::move
While `std::copy` is excellent for duplicating values, there may be instances where you need to move resources instead of copying them. In such cases, `std::move` is a more suitable alternative. It transfers ownership and can significantly enhance performance, especially with large objects that are expensive to copy.
std::copy_if
If your copying needs are conditional based on certain criteria, consider using `std::copy_if`. This variant allows you to specify a predicate that determines which elements to copy, making it ideal for filtering data efficiently.
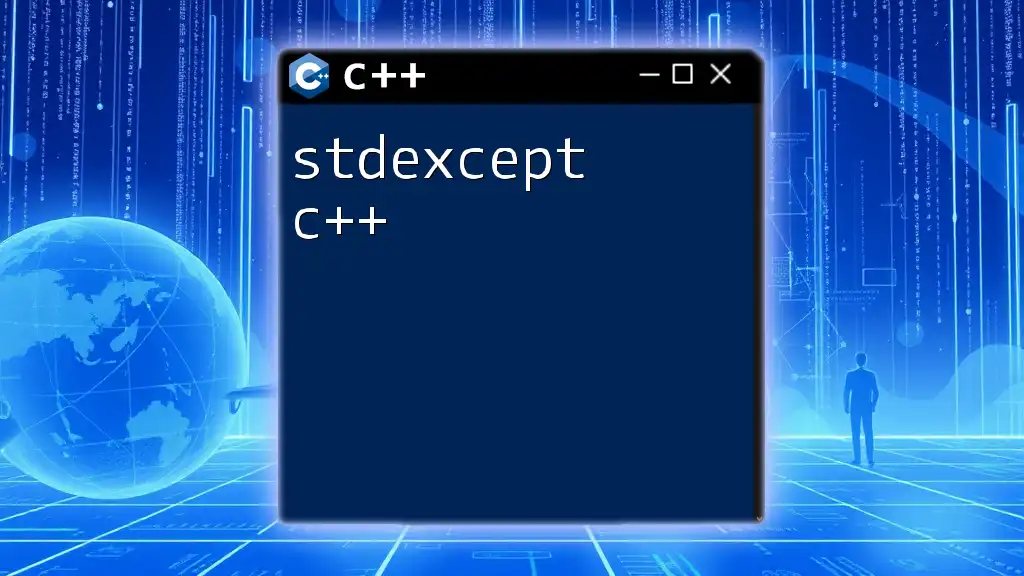
Best Practices
Consider Iterator Validity
When using `std::copy`, it’s imperative to ensure that the iterators provided are valid and do not lead to dereferencing errors. This includes checking whether the range defined by the iterators is valid.
Ensuring Destination Can Hold Source Data
Always verify that the destination can accommodate the elements being copied, especially in cases where the structures have different sizes. Failing to ensure adequate space can lead to buffer overflows and ultimately crashes.
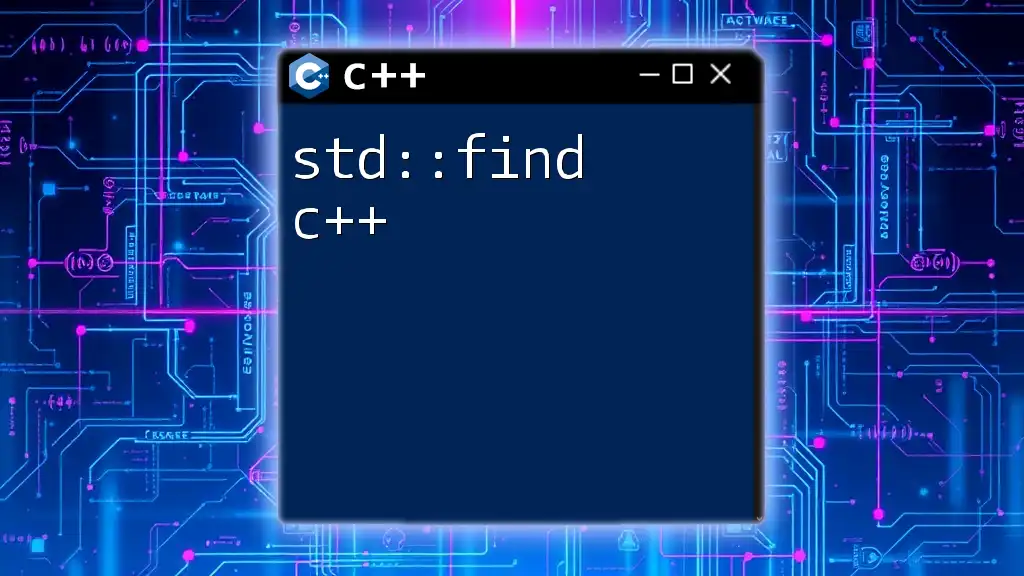
Common Errors
Misuse of Iterators
One of the most common pitfalls developers face is the misuse of iterators. If the end iterator is not greater than the start iterator, or if the range goes beyond the container's limits, you will likely encounter runtime errors.
Memory Issues
Another potential area of error lies within memory management, particularly when dealing with raw pointers or certain custom data types. Copying uninitialized memory or out-of-bounds addresses can lead to unpredictable behavior and should be avoided at all costs.
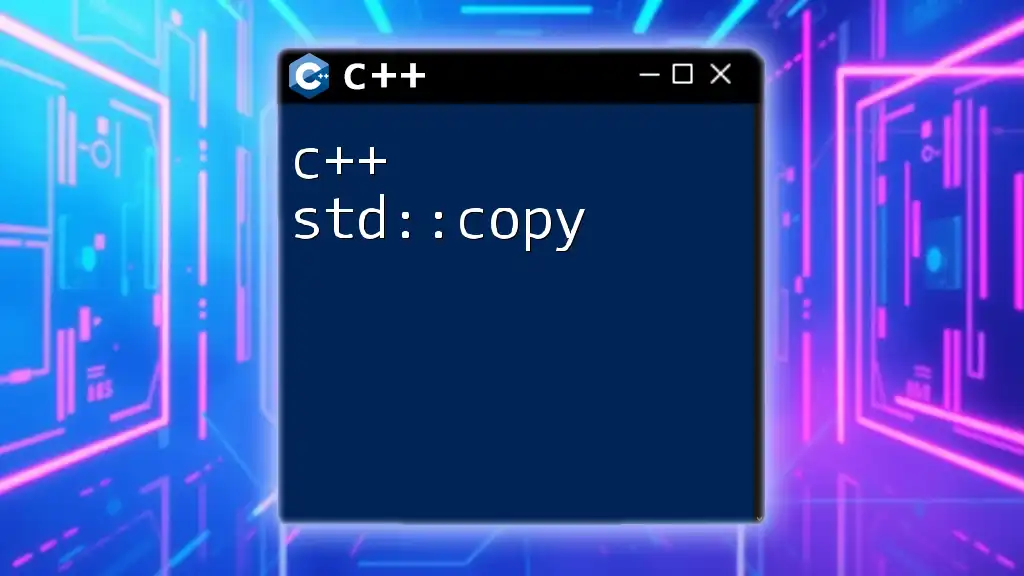
Conclusion
In conclusion, std::copy is a versatile and powerful tool in the C++ Standard Library that can enhance the efficiency and clarity of your code when managing data collections. Understanding how to utilize it effectively—from standard copying to managing overlapping data and exploring alternatives—can significantly improve your C++ programming skills. Embrace the power of `std::copy` and experiment with its various applications to ensure that your code remains clean, concise, and efficient.
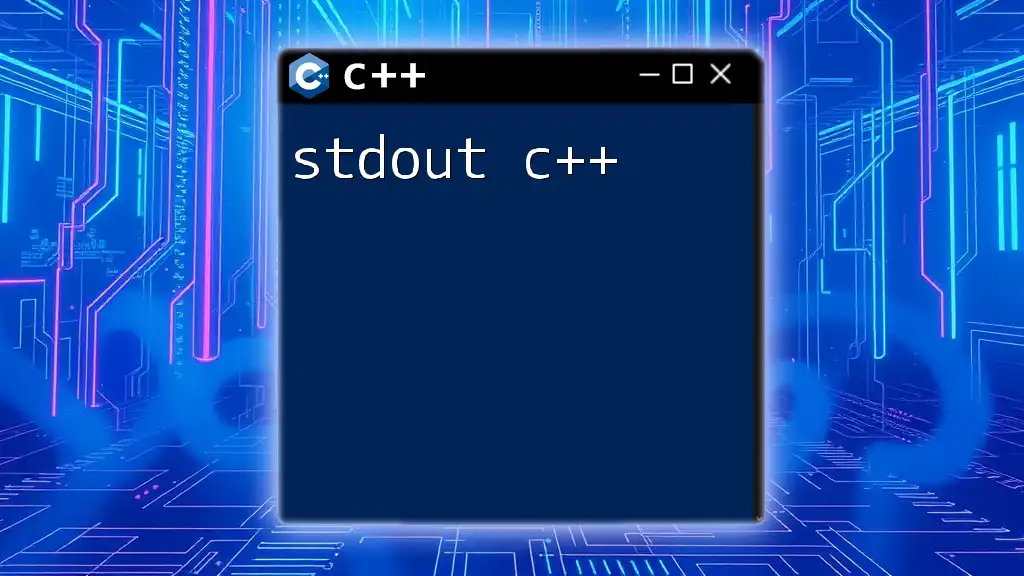
Additional Resources
For further exploration of `std::copy` and its capabilities, consider checking out the official C++ documentation or pursuing books and online courses focused on C++. These resources will deepen your understanding and provide hands-on practice to solidify your knowledge.
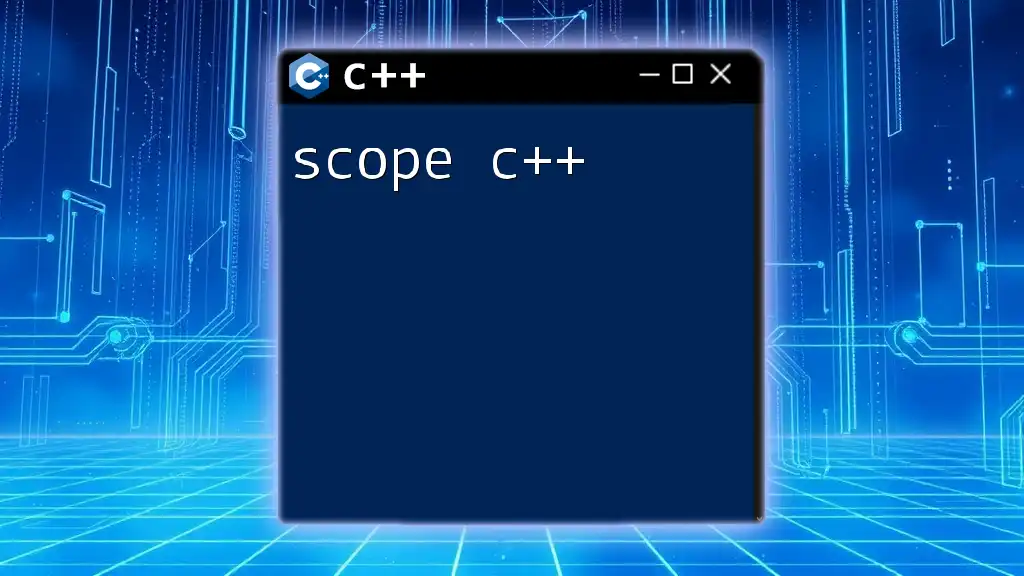
Call to Action
We encourage you to practice using `std::copy` in your projects. Share your experiences or any questions you may have in the comments! Your learning journey in C++ awaits!