In C++, scope refers to the visibility and lifetime of variables, functions, and objects, determining where they can be accessed and modified within the code.
#include <iostream>
void exampleFunction() {
int x = 10; // x is in the scope of exampleFunction
std::cout << x << std::endl;
}
int main() {
exampleFunction();
// std::cout << x; // This would cause an error, as x is not accessible here
return 0;
}
Understanding Scope in C++
Scope in programming refers to the region of the code where a defined variable can be accessed. Understanding scope in C++ is crucial, as it affects variable lifespan, visibility, and how variables interact with each other across different areas of the code.
Different types of scope exist in C++, which fundamentally determine how variables are accessed and manipulated throughout a program.
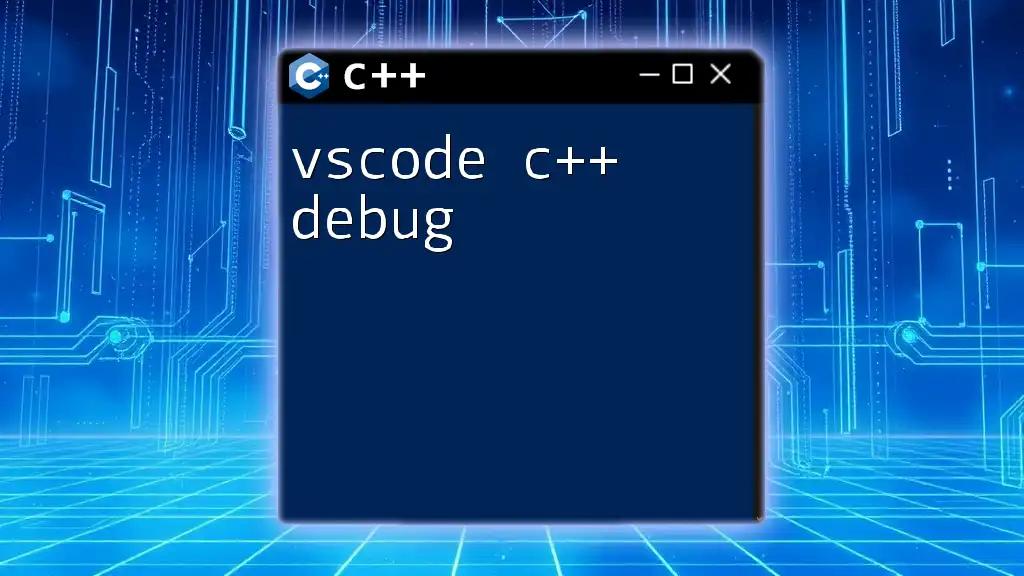
Types of Scope in C++
Global Scope
A variable that is declared outside of all functions possesses global scope. Such variables can be accessed by any function in that file, and even in other files if properly declared.
Example of Global Variables:
int globalVar = 100; // Global variable
void printGlobal() {
std::cout << "Global Variable: " << globalVar << std::endl; // Output: 100
}
Local Scope
Local scope is defined within a specific function or block. Variables declared within a function are only accessible within that function.
Example of Local Variables:
void exampleFunction() {
int localVar = 50; // Local variable
std::cout << "Local Variable: " << localVar << std::endl; // Output: 50
}
// localVar cannot be accessed here
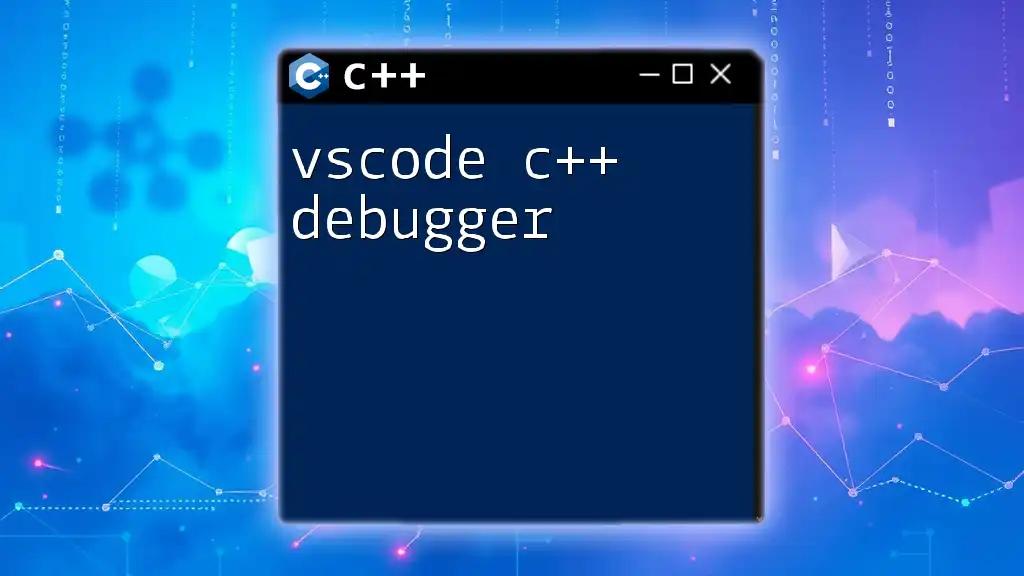
The Concept of Scope Resolution
What is Scope Resolution?
The scope resolution operator `::` allows the programmer to specify which variable or function to use when there are naming conflicts, particularly when a variable in the local scope shares the same name as a global variable.
Using the Scope Resolution Operator
By using the scope resolution operator, you can easily distinguish between local and global variables.
Accessing Global Variables from Local Scope:
int x = 10; // Global variable
void display() {
int x = 20; // Local variable
std::cout << "Local x: " << x << std::endl; // Output: 20
std::cout << "Global x: " << ::x << std::endl; // Output: 10
}
In this example, the local variable `x` overrides the global `x` within the `display` function. However, by using `::x`, we can explicitly access the global variable.
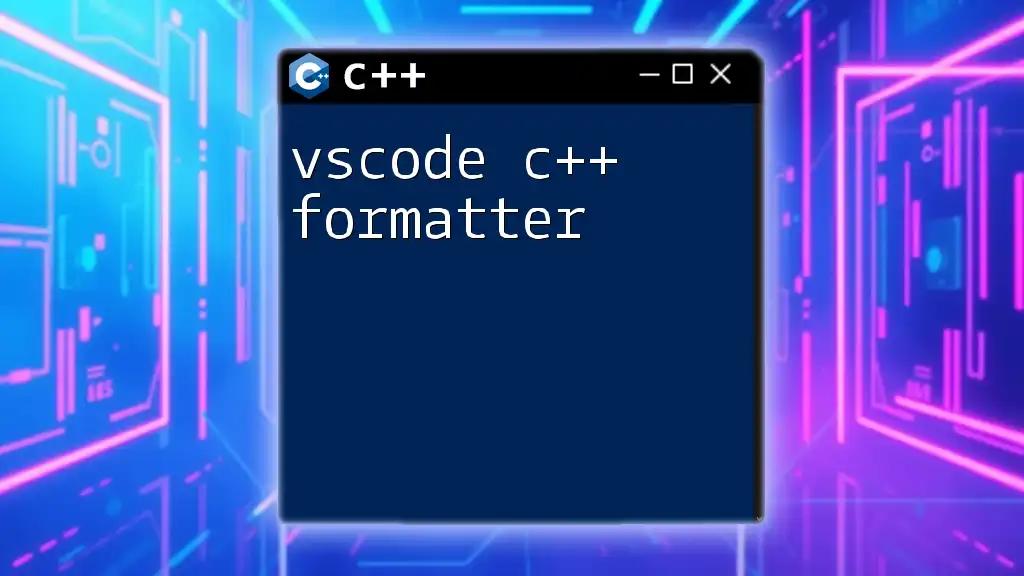
Different Scopes in C++
Block Scope
Block scope occurs within a pair of braces `{}`. Variables declared inside a block are not accessible outside of it.
Example of Block Scope with Conditional Statements:
void checkScope() {
int a = 5;
if (a > 0) {
int b = 10; // Block scope
std::cout << "Inside block b: " << b << std::endl; // Output: 10
}
// std::cout << b; // Error: b is not accessible here
}
Here, `b` is only accessible within the `if` block, showcasing how block scope controls visibility.
Function Scope
Function scope refers to the area within a function where variables are declared. Parameters of a function are also included in this scope.
Example of Function Parameters:
void exampleFunction(int param) { // param has function scope
std::cout << "Parameter: " << param << std::endl; // Output: Corresponding value passed
}
The parameter `param` is accessible only within `exampleFunction`, reinforcing the principle that scopes limit visibility.
Class Scope
In C++, class scope encompasses all members—variables and methods—defined within a class. Accessibility of members depends on their access modifiers: `public`, `private`, and `protected`.
Example Using Class Members:
class MyClass {
int classVar; // Class scope
public:
void setVar(int val) {
classVar = val; // Accessing class variable
}
int getVar() {
return classVar; // Returning class variable
}
};
The class variable `classVar` is only accessible through the public methods defined, exemplifying how class scope operates.

The Importance of Understanding Scope
Avoiding Naming Conflicts
Understanding scope is essential to avoid naming conflicts. If two variables in different scopes share the same name, C++ automatically resolves which one to use based on proximity. This prevents accidental overwrites and logical errors.
Memory Management
Scope also plays a crucial role in memory management. Local variables are allocated on the stack and automatically released at the end of their scope, which can help free up memory. Conversely, global variables remain in memory for the duration of the program, potentially leading to memory leaks if not managed carefully.
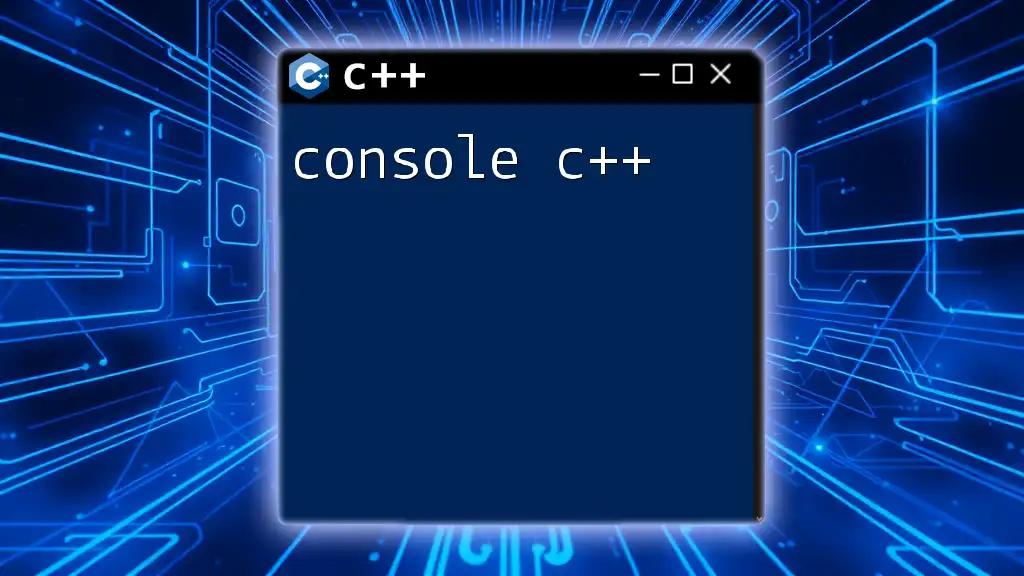
Best Practices
Using Local Variables Whenever Possible
Minimizing the use of global variables is a best practice in C++. Local variables enhance encapsulation and help prevent unwanted interactions between parts of a program. They create fewer side effects and make functions easier to manage.
Example Illustrating Potential Pitfalls of Global State:
int globalState = 5;
void unsafeFunction() {
globalState += 10; // Modifying global variable
}
The function `unsafeFunction` changes `globalState`, making the code harder to follow and debug. It’s safer to keep variables confined to their respective scopes.
Clarity in Naming Conventions
Clear naming conventions can significantly help avoid confusion. When naming variables, provide context and utilize prefixes or suffixes to denote scope or purpose. This practice aids code readability and maintenance.
Example Illustrating Effective Naming Strategies:
int globalCounter; // clear that this is global
void processLocalCounter() {
int localCounter; // clear that this is local
}

Conclusion
Mastering the concept of scope in C++ is fundamental to writing clean, understandable, and maintainable code. Each type of scope serves its purpose—global, local, block, function, and class scope—all contribute to how your program operates and uses memory.

Further Reading and Resources
For those seeking to dive deeper into scope in C++, consider resources such as the official C++ documentation, online coding platforms for hands-on practice, or dedicated courses that expand on the concepts presented in this guide. Understanding scope will empower you to write more effective and efficient code in C++.