The VSCode C++ formatter improves code readability by automatically formatting your C++ code according to defined style conventions.
Here's a simple code snippet to illustrate its usage:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Understanding Code Formatting in C++
What is code formatting?
Code formatting refers to the consistent arrangement and organization of code within a programming language, aimed at enhancing readability and maintenance. Properly formatted code is easier to understand, which reduces the time and effort needed to debug and extend it. When you format your C++ code, you significantly improve its clarity, allowing both you and other developers to navigate the codebase efficiently without facing confusion.
Poor formatting can lead to several issues, such as misinterpretation of logic flow, difficulty in locating specific functions, and making maintenance cumbersome.
Common formatting styles used in C++
In C++, various formatting styles have emerged, each designed to improve code aesthetics and readability. Some of the most popular styles include:
- LLVM Style: Aimed at producing neat and consistent code, it emphasizes minimal spaces, with an indentation of two spaces for blocks.
- Google Style: Known for its rigorous rules, this style mandates specific practices that enforce readability and consistency across larger projects.
- Mozilla Style: This style includes conventions for JavaScript, but its adaptation for C++ shares common readability goals.
Understanding these styles is essential for developers as it helps foster communication and collaborative efforts within teams, ensuring everyone is on the same page regarding code standards.
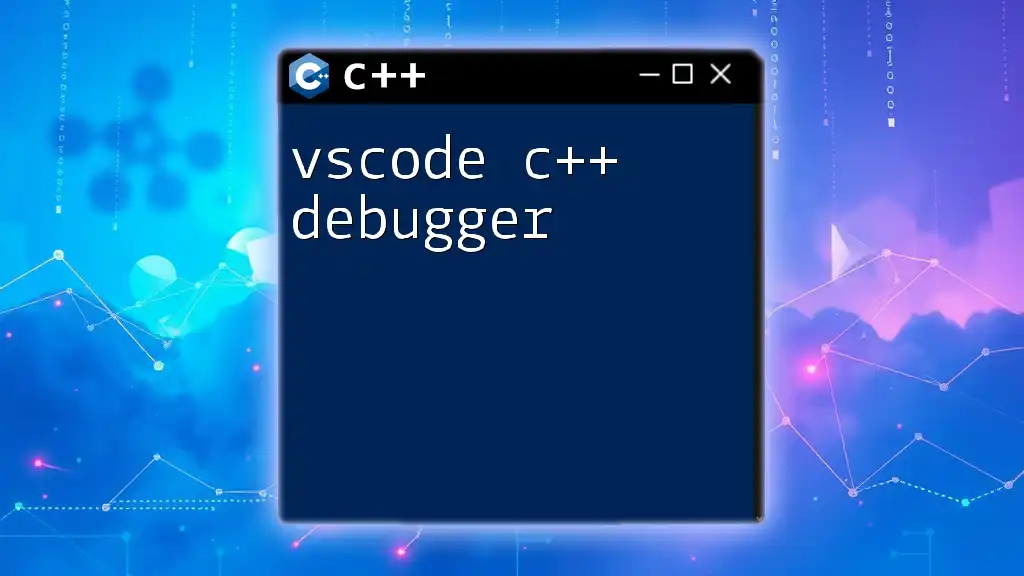
Setting Up VSCode for C++
Installing Visual Studio Code
To begin using the VSCode C++ formatter, the first step is downloading and installing Visual Studio Code. Visit the [official VSCode website](https://code.visualstudio.com/) to download the appropriate version for your operating system—Windows, macOS, or Linux.
Once downloaded, run the installer and follow the setup instructions. After completing the installation, start VSCode to explore its versatile features.
Adding C++ Extensions
To maximize the functionality of VSCode for C++ development, you must add some essential extensions. One notable extension is the C/C++ extension provided by Microsoft, which offers features such as IntelliSense, debugging, and code navigation.
To install the C/C++ extension:
- Open VSCode and go to the Extensions view by clicking on the Extensions icon in the sidebar or by pressing `Ctrl + Shift + X`.
- Search for "C/C++" in the marketplace.
- Click "Install" on the Microsoft C/C++ extension result.
Configuring VSCode Settings
VSCode has a settings menu that allows you to customize nearly every aspect of the editor. To access the settings, navigate to `File > Preferences > Settings` (or `Code > Preferences > Settings` on Mac).
Explore the settings options to tailor your environment. You can search for specific keywords such as "C++" to easily locate relevant settings.
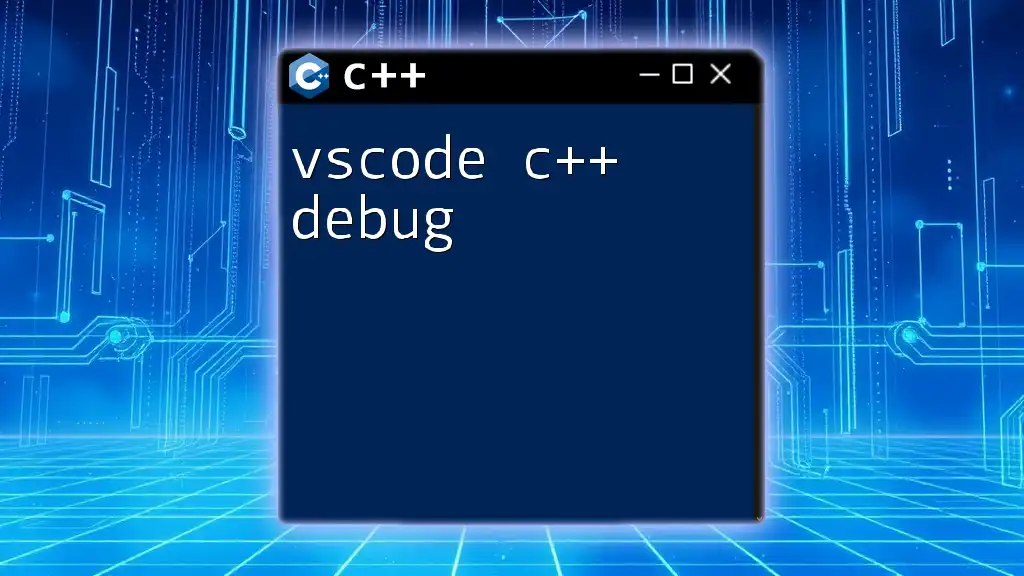
Using the C++ Formatter in VSCode
Accessing the Formatter
Once your environment is set up, formatting your code in VSCode is straightforward. The easiest way to format your code manually is by using shortcut keys.
For Windows/Linux, the default shortcut is `Shift + Alt + F`, and for Mac, it’s `Shift + Option + F`. Just place your cursor in the code file you want to format and use the shortcut to bring your code into a more readable arrangement.
To improve your workflow, you can enable auto-formatting upon saving your files. This feature automatically formats your code every time you save it, ensuring that your code remains clean and consistent.
- Open your `settings.json` file.
- Add the following line:
"editor.formatOnSave": true
Configuring the Formatter
For deeper customization, you can adjust formatting options directly in the `settings.json` file. To access this file:
- Go to `File > Preferences > Settings`.
- Search for `settings.json` and select "Edit in settings.json".
Here’s an example configuration you might consider:
{
"C_CPP.clang_format_style": "file",
"editor.tabSize": 4,
"editor.insertSpaces": true,
"editor.formatOnType": true
}
This configuration specifies that VSCode should utilize the formatting rules defined in your Clang-Format file while setting the tab size to four spaces and ensuring spaces are used instead of tabs. Adjusting `editor.formatOnType` to true allows format options to be applied as you type, further enhancing the coding experience.
Using Clang-Format with VSCode
Clang-Format is a powerful tool designed to format C and C++ code according to a specified style, integrating seamlessly with VSCode. To leverage Clang-Format effectively, first ensure it's installed in your development environment.
To check if Clang-Format is installed:
- Open your terminal or command line interface.
- Type `clang-format --version` to verify installation.
If you need to install Clang-Format, visit the [LLVM official website](https://releases.llvm.org/download.html) and follow the installation instructions for your operating system.
Once installed, you can configure Clang-Format within VSCode. To specify your style preferences, create a `.clang-format` file in the root directory of your project. Here’s a basic example of what this file can look like:
BasedOnStyle: Google
IndentWidth: 4
UseTab: Never
This configuration adopts the Google style as its base while specifying a four-space indentation and a rule against using tabs. You can experiment with various options available in Clang-Format to customize it according to your development needs.
Formatting Code Examples
Consider the following poorly formatted C++ code:
int main(){int x=10;int y=20;if(x<y){cout<<"x is less than y";}return 0;}
When applying the VSCode formatter, you'll find that the code becomes much clearer:
#include <iostream>
using namespace std;
int main() {
int x = 10;
int y = 20;
if (x < y) {
cout << "x is less than y";
}
return 0;
}
The improved formatting enhances readability and significantly aids in understanding the logic, ultimately leading to more maintainable code. Adopting a consistent formatting style ultimately leads to cleaner code that is easier for teams to navigate and collaborate on.
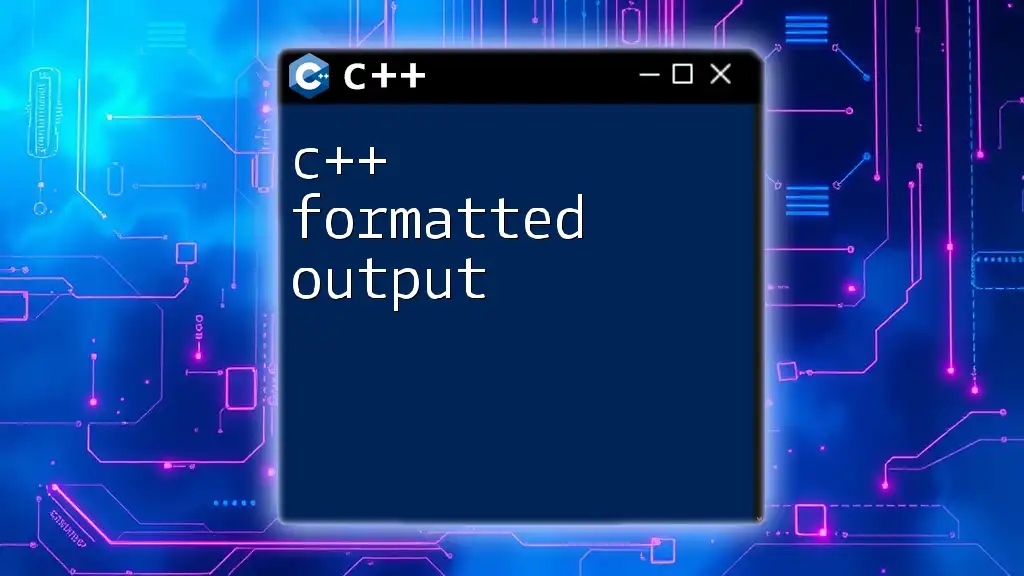
Advanced Formatting Techniques
Customizing Formatting Rules
One of the powerful features of Clang-Format is the ability to override its default rules to better suit your project’s specific needs. You can customize settings in your `.clang-format` file to enforce rules that align with your team’s coding standards.
For example, if you want to allow `colons` at the end of a line but wish to have a stricter line length, your configuration could look like this:
ColumnLimit: 80
AllowShortBlocksOnASingleLine: true
AllowShortCaseLabelsOnASingleLine: true
This customization allows you to keep your lines under 80 characters while still maintaining flexibility for shorter code constructs.
Enabling Language-Specific Formatting
VSCode supports multiple languages, and you can configure language-specific formatting settings. For C++, ensure your format settings override default global configurations effectively.
To do this, access the `settings.json` file and specify C++ settings like this:
"[cpp]": {
"editor.formatOnSave": true,
"editor.tabSize": 4
}
This will ensure that your tab size and formatting occurs specifically for C++. Engaging in such practices will help maintain clarity especially if you're working in a multi-language environment.
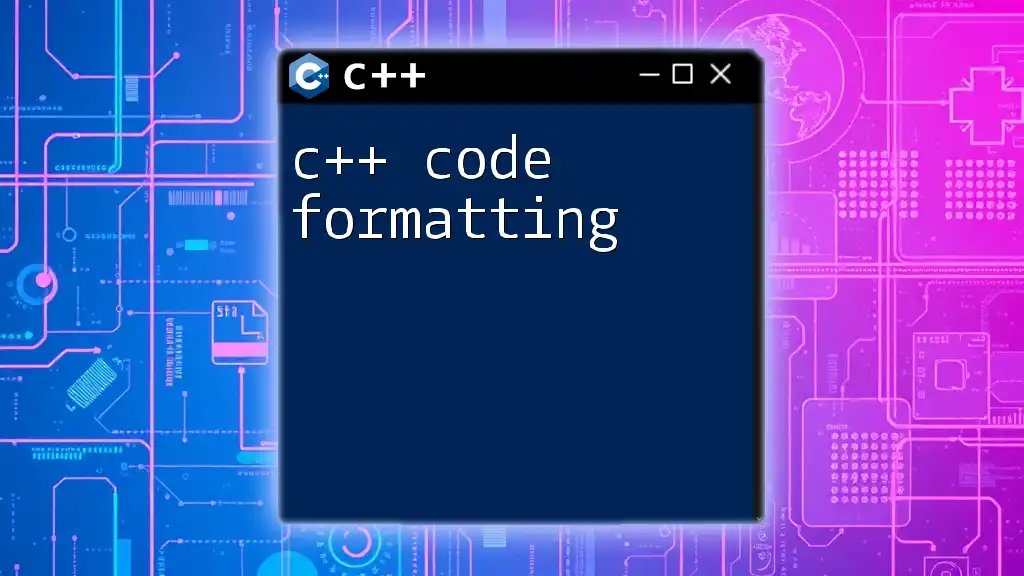
Troubleshooting Common Issues
Despite its many advantages, developers may encounter issues with the formatter. Here are some common problems and their solutions:
-
Formatter not working: If your formatter is inactive, check if the corresponding extension is properly installed and enabled. Also, ensure you don’t have conflicts with other formatting extensions.
-
Conflicts between different formatting tools: If several formatting tools are configured, it can lead to inconsistencies. Use the settings in `settings.json` to prioritize which formatter to utilize.
-
Language server issues: Sometimes, the language server can interfere with formatting. Make sure your C/C++ language server is up-to-date, as improvements and fixes are released regularly.
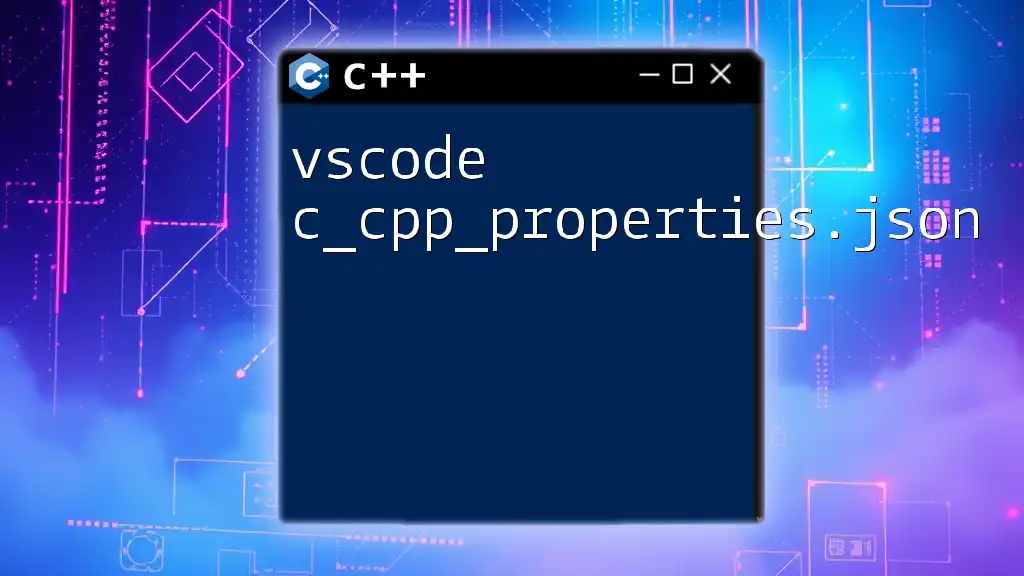
Conclusion
Utilizing the VSCode C++ formatter is crucial for developing high-quality code that is clean, maintainable, and collaborative. This guide has covered everything from setting up VSCode to configuring advanced formatting techniques to troubleshoot common issues effectively.
Emphasizing frequent formatting practices not only fosters better coding habits but promotes a culture of clear communication among developers. Test out these formatting techniques and observe how they enhance both your coding experience and team collaboration!
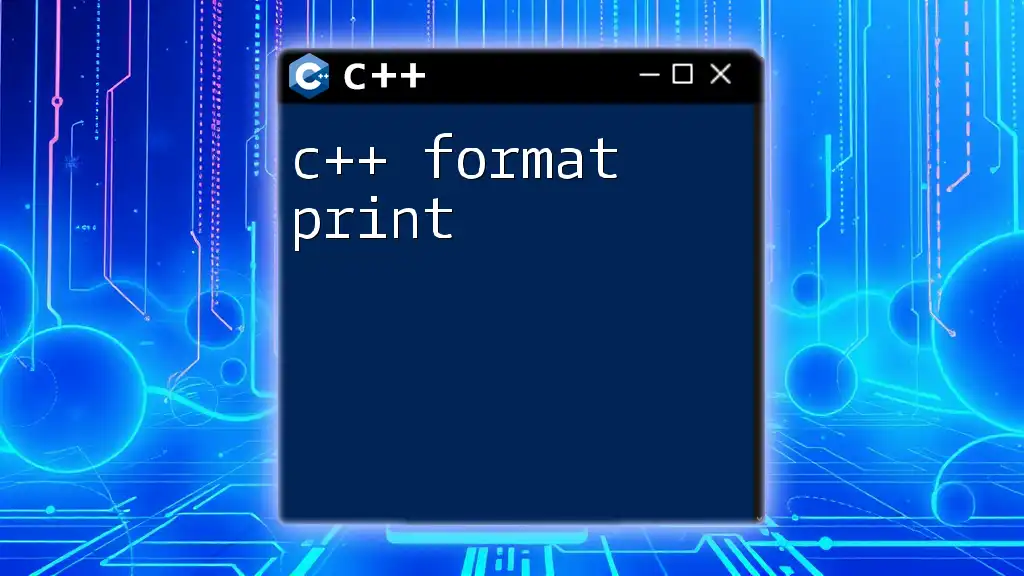
Call to Action
Try out VSCode's C++ formatter today and discover how it can transform your coding style! If you are looking for more advanced C++ techniques or need help mastering C++ commands, feel free to check our upcoming courses and resources. We invite you to share your experiences, feedback, or any formatting challenges you encounter in the comments below!