C++ formatted output can be achieved using the `iostream` library along with manipulators like `std::cout` and `std::fixed` to control decimal precision.
Here's a simple code snippet demonstrating formatted output:
#include <iostream>
#include <iomanip> // Include for std::setprecision
int main() {
double number = 3.14159;
std::cout << "Formatted output: " << std::fixed << std::setprecision(2) << number << std::endl;
return 0;
}
The Basics of C++ Output
Understanding C++ Output Options
In C++, the way we display information to users primarily revolves around output streams. The standard output stream, `std::cout`, is the default tool for displaying content on the console. There are other options like `std::cerr` for errors and `std::clog` for logging purposes. Understanding these streams is crucial for effective C++ formatted output.
Writing Basic Output Statements
The most straightforward way to write to the console is by using the `std::cout` object from the `<iostream>` library. For example, consider the following:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
In this snippet, `std::cout` sends the string "Hello, World!" to the standard output. Using `std::endl` not only outputs a newline but also flushes the output buffer, ensuring that all data is written. This basic format lays the groundwork for more complex output operations.
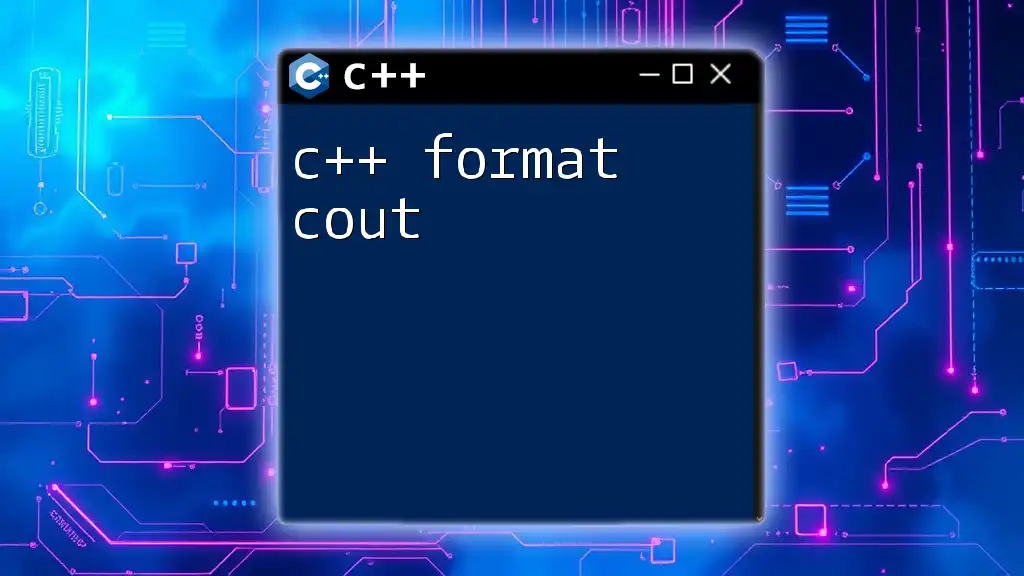
Introduction to the `<iomanip>` Library
What is `<iomanip>`?
The `<iomanip>` library is essential for manipulating the input and output of formatted data in C++. It includes various manipulators that enable developers to customize the way data is presented, making the output clearer and more user-friendly. Using this library, we can enhance the quality of C++ formatted output significantly, allowing for better presentation of data across various types and contexts.
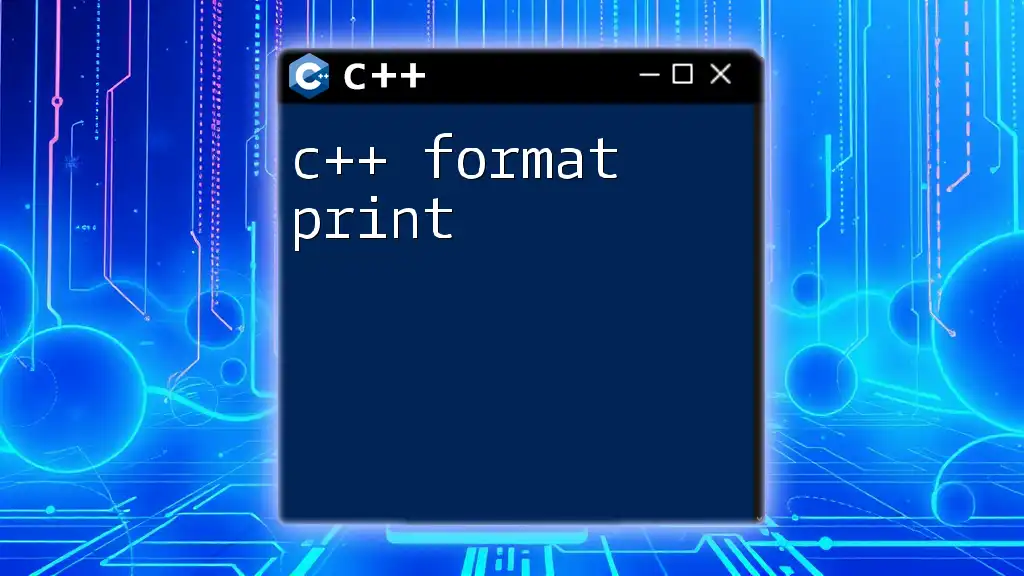
C++ Output Format with Manipulators
Using Standard Manipulators
Manipulators are special functions that affect the formatting of the output stream. Some common manipulators include `std::endl`, `std::flush`, and `std::setw`. The `std::setw` manipulator sets the width of the next output field.
For example:
#include <iostream>
#include <iomanip>
int main() {
std::cout << std::setw(10) << 42 << std::endl;
return 0;
}
In this example, `std::setw(10)` specifies that the number `42` should occupy a width of 10 spaces. If the number is shorter than the specified width, it is padded with spaces on the left. This technique is particularly useful for aligning multiple columns of data in a readable manner.
Customizing Decimal Precision
Floating-point precision can also be managed using the `std::setprecision` manipulator. This allows for control over how many digits appear after the decimal point.
#include <iostream>
#include <iomanip>
int main() {
double pi = 3.14159;
std::cout << std::setprecision(3) << pi << std::endl;
return 0;
}
In this snippet, the output will be `3.14`, demonstrating how precision can be adjusted to meet output specifications, such as in mathematical calculations.
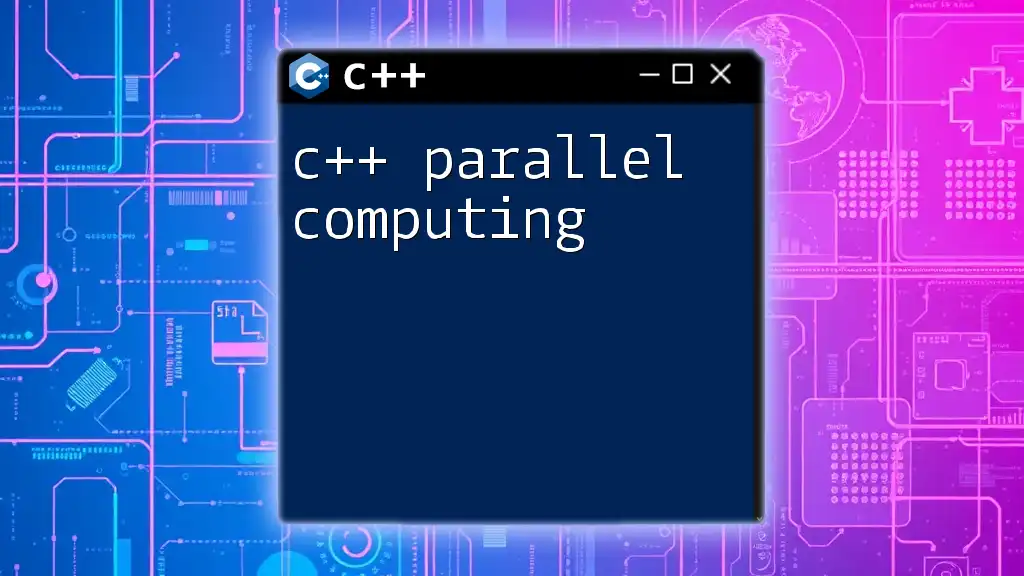
Formatting C++ Output for Different Data Types
Formatting Integers
C++ offers various ways to represent integer values using different numeral systems, such as hexadecimal, decimal, and octal. You can use manipulators like `std::hex`, `std::dec`, and `std::oct` to format integers as needed.
#include <iostream>
#include <iomanip>
int main() {
int num = 255;
std::cout << std::hex << num << std::endl; // Output in hexadecimal
return 0;
}
In this case, `255` will be displayed as `ff` in hex format. This capability allows for precise control over the representation of numbers and is particularly useful in programming situations where different numeral bases are required.
Formatting Floating-Point Numbers
For floating-point numbers, `std::fixed` and `std::scientific` manipulators can be used to dictate whether the values are displayed in fixed or scientific notation, respectively.
#include <iostream>
#include <iomanip>
int main() {
double value = 123.456789;
std::cout << std::fixed << std::setprecision(2) << value << std::endl; // Outputs: 123.46
return 0;
}
Here, using `std::fixed` along with `setprecision(2)` means that the output will round to two decimal places. Understanding these formatting options is crucial, especially in financial applications or when exact numerical representation is required.
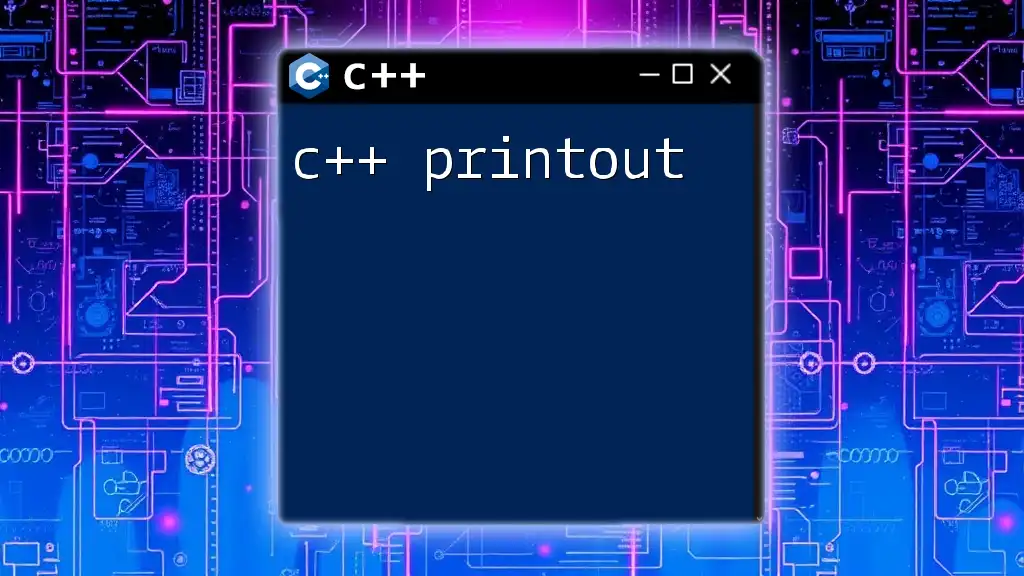
Advanced Output Formatting Techniques
Outputting Custom Formats with User-Defined Functions
For more complex formatting scenarios, you can create user-defined functions that encapsulate your formatting logic. This encapsulation helps in maintaining clean code and reusing formatting logic across your application.
#include <iostream>
#include <iomanip>
void printFormatted(double value) {
std::cout << std::setprecision(2) << std::fixed << value << std::endl;
}
int main() {
printFormatted(7.123456);
return 0;
}
In this example, the `printFormatted` function standardizes how floating-point numbers are printed by enforcing a specific precision and formatting approach. This serves to improve code maintainability and ensure consistency in output format.
Working with String Output Formatting
C++ allows for output formatting of string data as well. Using `std::setw` with strings can create nicely aligned outputs.
#include <iostream>
#include <iomanip>
#include <string>
int main() {
std::string name = "Alice";
std::cout << std::setw(10) << name << std::endl;
return 0;
}
The output will position "Alice" to start from the 10th column, thereby showcasing how to manage string formatting effectively.
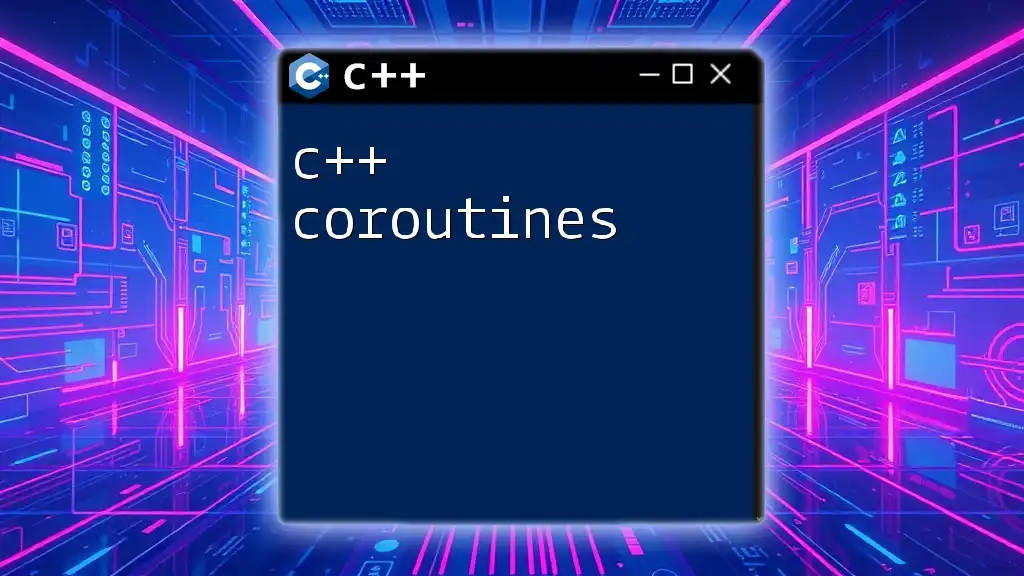
Common Mistakes in C++ Output Formatting
Pitfalls to Avoid
Even seasoned developers can run into pitfalls with formatted output. Common mistakes include forgetting to include the appropriate headers (like `<iomanip>`) or neglecting to reset manipulators between different output statements, which can lead to unexpected results.
Best Practices for Output Formatting
To maintain clarity and consistency, adhere to the following best practices:
- Always reset manipulators when changing output types.
- Ensure precision and width settings are applied meaningfully to maintain good readability.
- Keep formatting consistent throughout your application to enhance user experience.
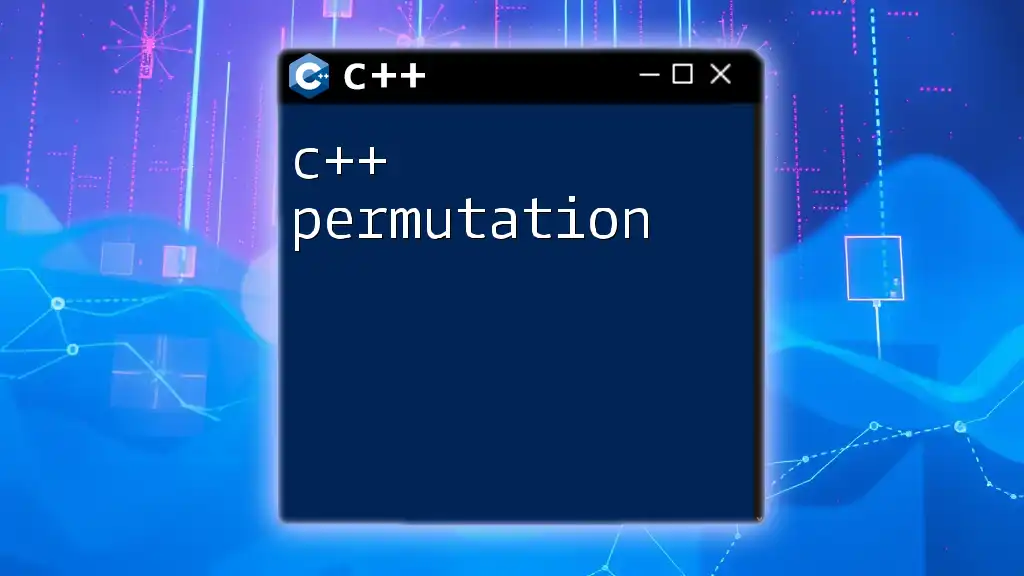
Conclusion
C++ formatted output plays a significant role in how data is presented and perceived. Mastery of the `<iomanip>` library and its manipulators empowers developers to customize their console output effectively. By applying the techniques discussed, you can elevate your C++ formatted output abilities, leading to clearer, more user-friendly applications. Embrace the challenge to explore these formatting options further and practice them through coding exercises to solidify your understanding.
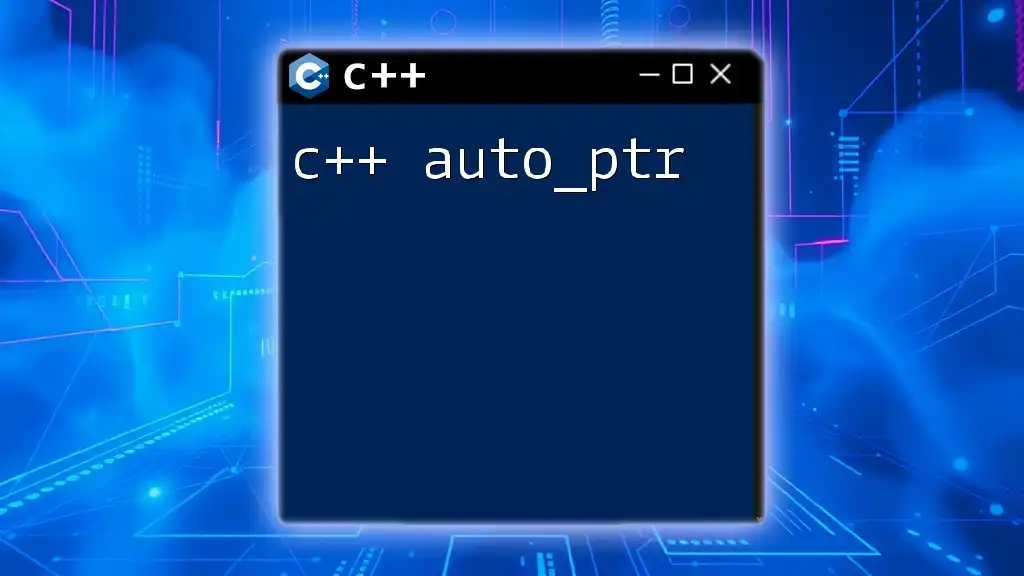
Additional Resources
For deeper dives, consider exploring books on C++ best practices, online courses tailored to C++ formatting, and official documentation on `<iomanip>` and output streams. Engaging in community forums can also provide insights and solutions to specific formatting challenges you might encounter.