In C++, you can format strings with variables using the `std::ostringstream` class, which allows you to elegantly concatenate variables into a string. Here’s a simple example:
#include <iostream>
#include <sstream>
int main() {
int age = 25;
std::string name = "John";
std::ostringstream formatted;
formatted << "My name is " << name << " and I am " << age << " years old.";
std::cout << formatted.str() << std::endl;
return 0;
}
Understanding Format Strings
What is a Format String?
A format string is a string that specifies how variable data should be formatted for output. It allows programmers to create dynamic messages by inserting variable values into predefined text structures. Understanding format strings is essential for effective data presentation in C++ programming, as they enhance readability and maintainability.
The Role of Variables in Format Strings
Variables are fundamental to programming, as they hold data that can change throughout the execution of a program. In the context of format strings, variables provide the flexibility to insert dynamic content into static text. For instance, using format strings with variables allows you to generate customized messages that reflect the state of your program.
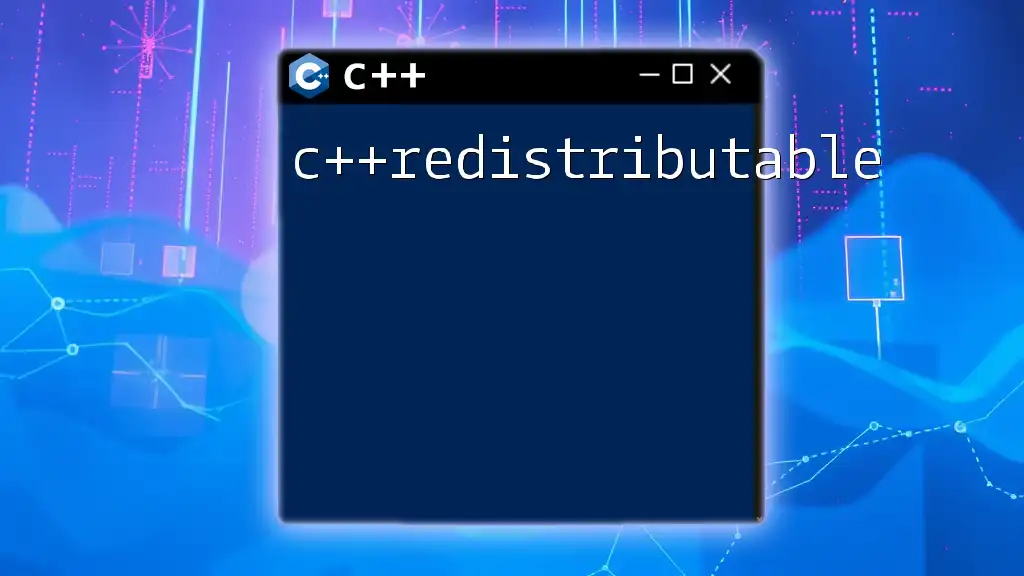
Formatting Strings in C++
Traditional C-style Formatting
In C++, you can use the C-style `printf` function from the C standard library to format strings. This approach primarily uses format specifiers to denote the type of data being inserted.
For example, consider the snippet below, which utilizes `printf` to output a formatted message:
#include <cstdio>
int main() {
int age = 25;
const char* name = "Alice";
printf("My name is %s and I am %d years old.\n", name, age);
return 0;
}
In this code:
- `%s` is used to format a string (the variable `name`).
- `%d` formats an integer (the variable `age`).
Although `printf` is powerful, you must be cautious about type matching, as a mismatch can result in undefined behavior.
Using C++ Standard Library (std::cout)
Another common method for formatting strings in C++ is using `std::cout`, which is part of the iostream library. This approach leverages the insertion operator (`<<`) for output.
Here's an example demonstrating how to use `std::cout`:
#include <iostream>
int main() {
int age = 25;
std::string name = "Alice";
std::cout << "My name is " << name << " and I am " << age << " years old." << std::endl;
return 0;
}
In this snippet, `std::cout` is used to concatenate and display the string message. One of the significant benefits of this method is its type safety; you don’t have to worry about mismatched type specifiers. With `std::cout`, the C++ compiler performs type checking at compile time.
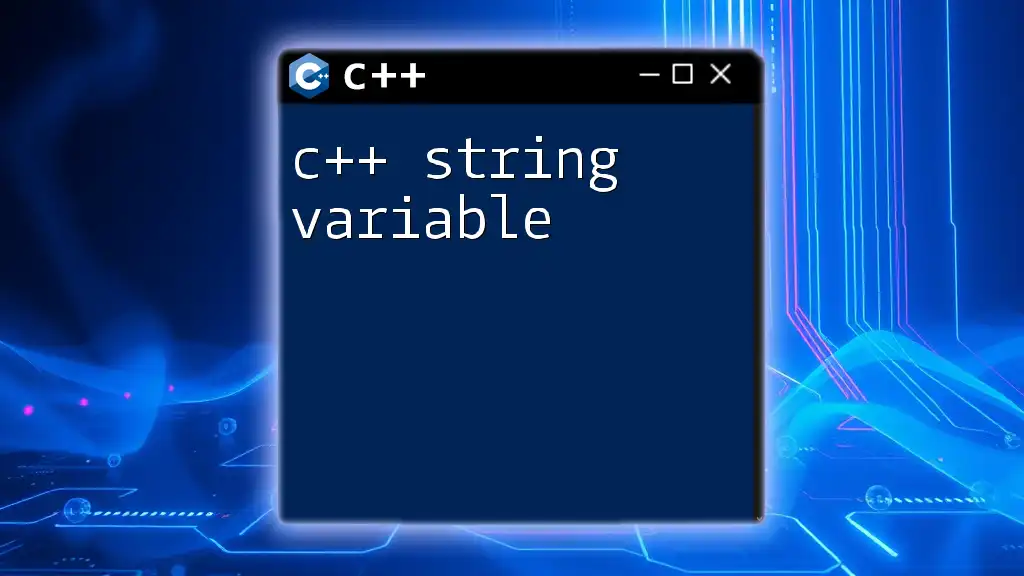
Advanced Formatting Options with C++
Using the std::format Function
Introduced in C++20, the `std::format` function offers a modern and safer approach to creating formatted strings. It provides a syntax that resembles `printf` while benefiting from type checking and easier maintenance.
Here’s how you can use `std::format`:
#include <format>
#include <iostream>
int main() {
int age = 25;
std::string name = "Alice";
std::string message = std::format("My name is {} and I am {} years old.", name, age);
std::cout << message << std::endl;
return 0;
}
In this example, `{}` acts as a placeholder for the variable values. The advantages of `std::format` include:
- Improved readability
- Type safety
- Eliminating the risk of buffer overflows common in `printf`
Custom Formatters (if applicable)
In more complex situations, you may need to create custom formatters for specialized data types. This feature allows you to define how a specific type should be converted to a string.
Here’s a simple example illustrating how to create a custom formatter for a user-defined class:
#include <iostream>
#include <string>
#include <format>
struct Person {
std::string name;
int age;
};
// Custom formatter for Person
template <>
struct std::formatter<Person> : std::formatter<std::string> {
auto format(const Person& p, format_context& ctx) {
return std::formatter<std::string>::format("My name is " + p.name + " and I am " + std::to_string(p.age) + " years old.", ctx);
}
};
int main() {
Person p{"Alice", 25};
std::cout << std::format("{}", p) << std::endl;
return 0;
}
In this example, we define a custom formatter that formats a `Person` object according to specific requirements. Custom formatters provide the flexibility to control how data types are presented when formatted.
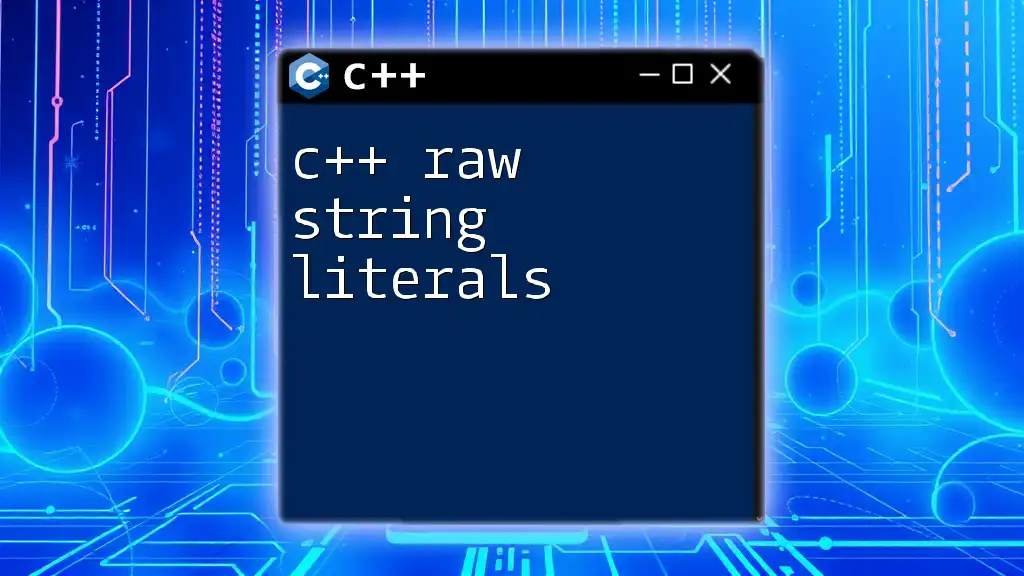
Error Handling in Format Strings
Common Pitfalls and Errors
Working with format strings can lead to several common pitfalls that can introduce bugs. One notable issue is using mismatched types in format specifiers. For example:
printf("My age is %s", age); // Wrong: 'age' is an int, not a string
This will cause undefined behavior, making it critical to match data types carefully.
Best Practices for Safe Formatting
To avoid errors, adhere to these best practices:
- Use `std::cout` or `std::format` whenever possible as they provide safer alternatives.
- Always verify the data types being used with format specifiers, particularly when using `printf`.
- Adopt a consistent formatting style throughout your codebase to maintain clarity and prevent confusion.
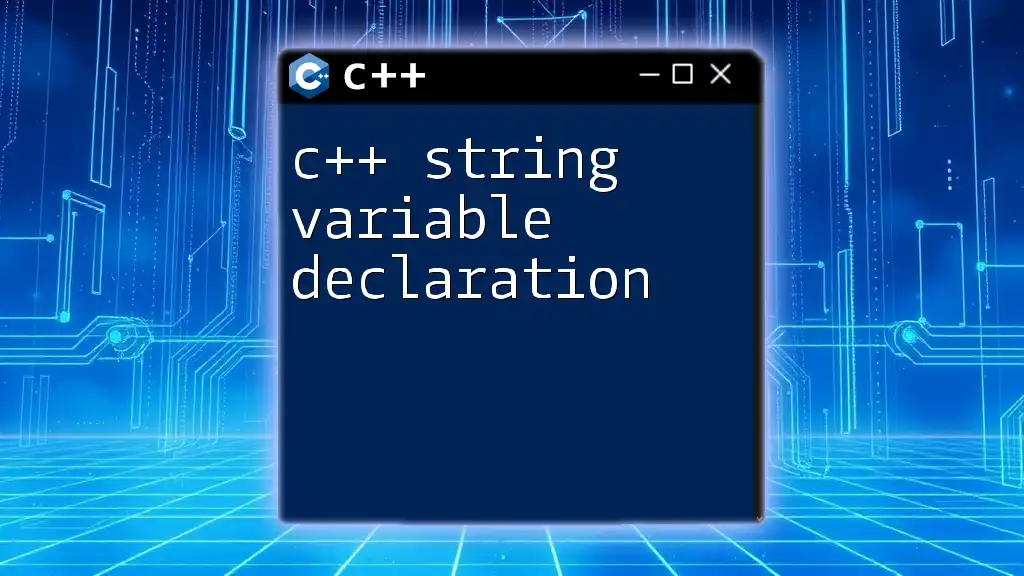
Conclusion
Mastering C++ format strings with variables is essential for creating dynamic and readable output in your applications. By leveraging both traditional C-style methods and modern C++ features like `std::cout` and `std::format`, you can produce clean and effective formatted strings. Remember to practice safe formatting techniques to mitigate errors and ensure that your code is both functional and maintainable.
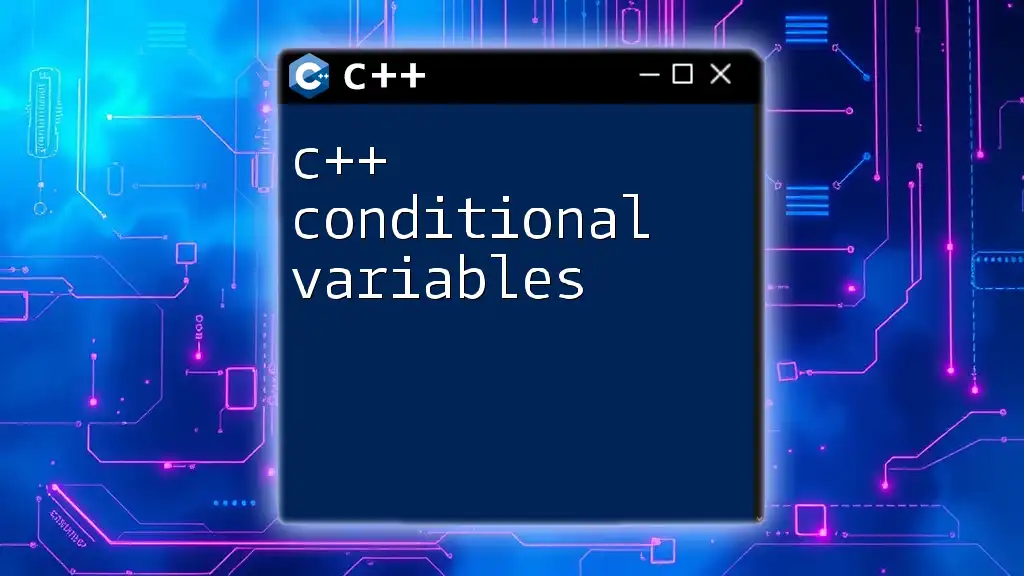
Additional Resources
Consider checking the C++ documentation for further learning and in-depth exploration of these concepts. You might also find value in books that offer comprehensive insights into the C++ programming language. Finally, online coding platforms are excellent for experimenting with various formatting techniques and solidifying your understanding.