In C++, you can write a string to a file using the `ofstream` class from the `<fstream>` library.
Here's a simple code snippet demonstrating this:
#include <fstream>
#include <string>
int main() {
std::ofstream outfile("example.txt");
outfile << "Hello, World!";
outfile.close();
return 0;
}
Understanding File I/O in C++
File Input/Output (I/O) refers to the mechanism through which a program interacts with files on the file system. It is an essential aspect of programming, allowing for data storage, retrieval, and manipulation. In C++, learning how to read and write files is fundamental for managing persistent data.
When dealing with file I/O in C++, three primary types of file streams are crucial:
- `ifstream` (Input File Stream): Used for reading data from files.
- `ofstream` (Output File Stream): Used for writing data to files.
- `fstream` (File Stream): A combination of both input and output operations.
These streams provide the tools necessary for opening files, performing read/write operations, and closing files to ensure proper resource management.
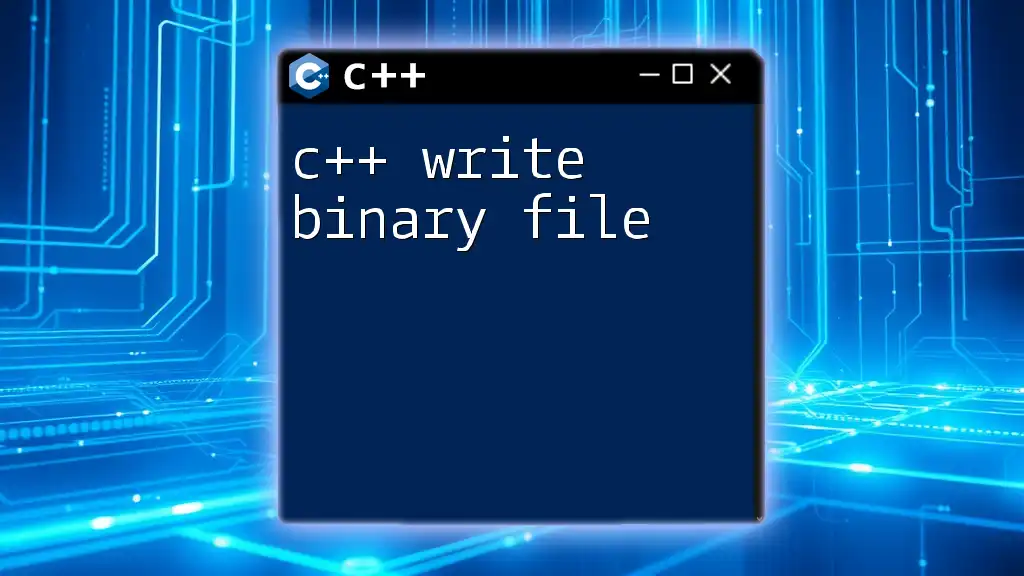
Setting Up Your C++ Environment
Before diving into the coding aspects of `c++ write string to file`, ensure that you are using a suitable C++ compiler. Popular choices include:
- G++: Commonly used on Linux systems.
- Clang: Versatile and often used in open-source projects.
- MSVC (Microsoft Visual C++): A choice for Windows users.
Instructions for installation may vary depending on the operating system, so consult the specific guidelines related to your platform.
For those who prefer a more user-friendly experience, setting up an Integrated Development Environment (IDE) can greatly improve productivity. Recommended IDEs include:
- Visual Studio: Rich in features, ideal for Windows users.
- Code::Blocks: Lightweight with excellent debugging tools.
- Eclipse: Includes support for various programming languages, including C++.
Once set up, creating a new project within your IDE will allow you to begin your exploration of file writing in C++.
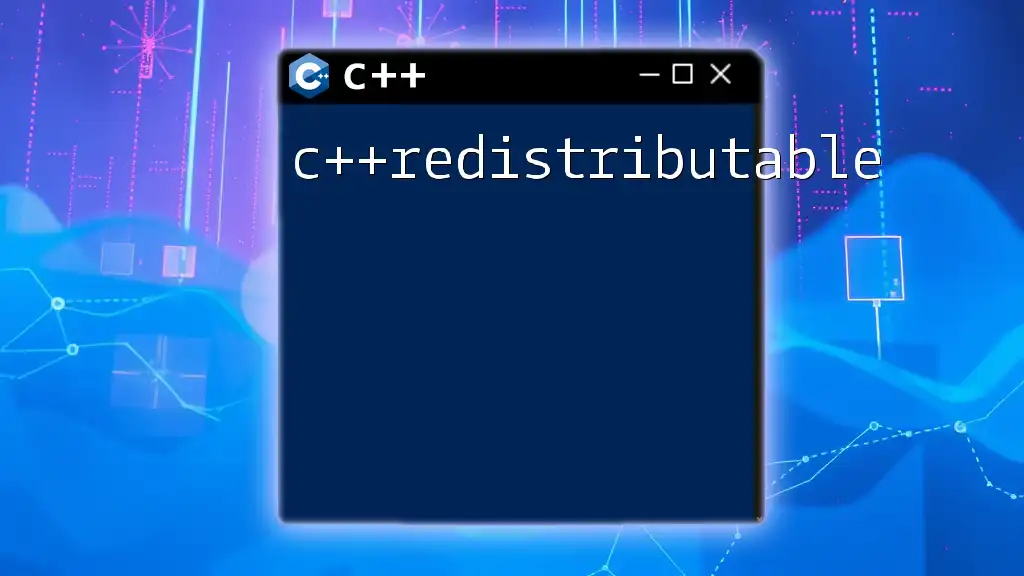
Opening Files in C++
Modes for Opening Files
When dealing with file operations, understanding the available modes is essential. The modes specify how the file will be handled during read/write operations. Key modes include:
- `ios::out`: Opens the file for writing. If the file exists, it will be truncated (emptied).
- `ios::app`: Opens the file for appending; data will be written at the end of the file.
- `ios::trunc`: Deletes the contents of the file if it already exists.
Code Snippet: Opening a File
To illustrate how to open a file for writing, consider the following code snippet:
#include <fstream>
int main() {
std::ofstream outfile("example.txt");
if (!outfile) {
std::cerr << "Error opening file!" << std::endl;
return 1;
}
// File operations go here
outfile.close();
return 0;
}
In this example, the code attempts to open a file named `example.txt` for writing. It includes error checking to ensure that the file is opened successfully, thereby preventing runtime errors when performing file operations.
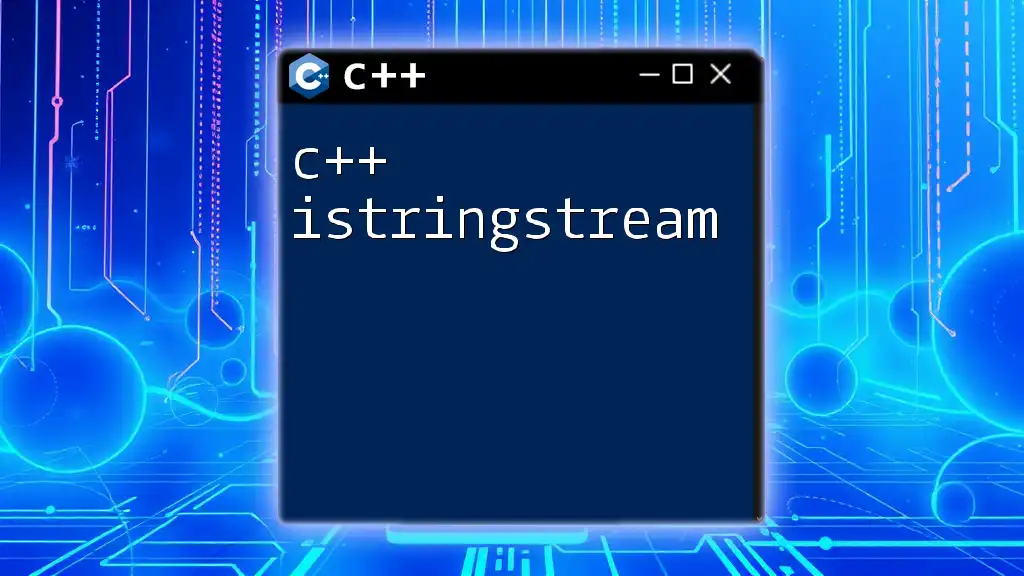
Writing Strings to a File in C++
Basic Writing Operation
The simplest way to write a string to a file is by using `std::ofstream`. Below is an example that demonstrates a basic write operation.
#include <fstream>
int main() {
std::ofstream outfile("example.txt");
outfile << "Hello, World!" << std::endl;
outfile.close();
return 0;
}
In this example, the string "Hello, World!" is written to `example.txt`. The use of `<<` operator allows for easy insertion of strings, and `std::endl` is used to insert a newline character.
Using Different Data Types
You can also write data types other than strings. For instance, appending integers alongside strings is straightforward:
#include <fstream>
int main() {
std::ofstream outfile("example.txt");
int age = 25;
outfile << "Age: " << age << std::endl;
outfile.close();
return 0;
}
This example shows how to write an integer value to the file, demonstrating the flexibility of the output stream in handling various data types.
Appending to an Existing File
If you want to add information to an already existing file, you can open it in append mode. Here’s how to do that:
#include <fstream>
int main() {
std::ofstream outfile("example.txt", std::ios::app);
outfile << "Appending this line." << std::endl;
outfile.close();
return 0;
}
By specifying `std::ios::app`, the program opens the file for appending, ensuring that new data is added at the end without affecting existing content.
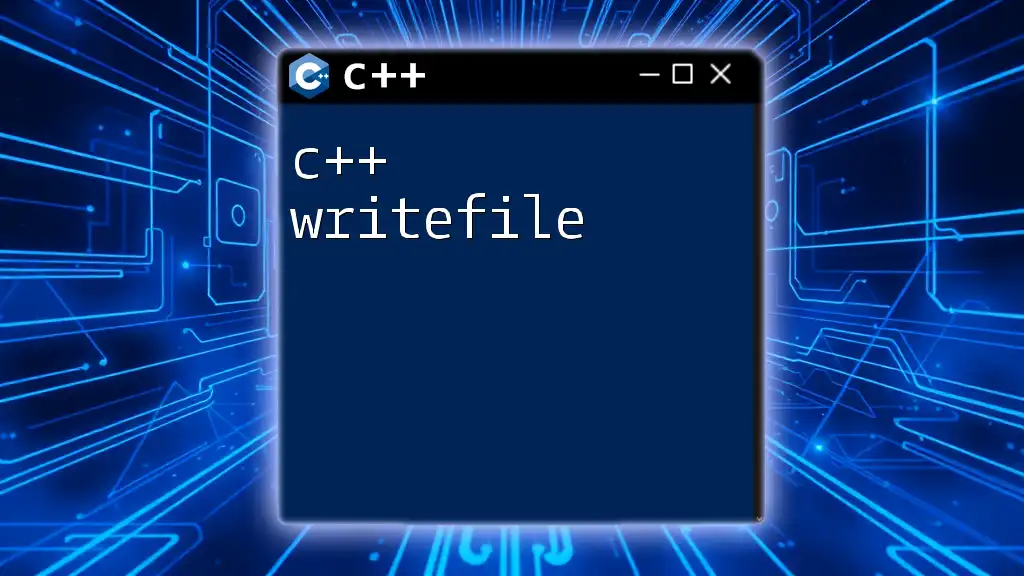
Error Handling in File Operations
Checking if File Opened Successfully
Ensuring that a file is opened successfully is crucial to avoid unexpected errors during execution. Always check for successful file opening before performing read/write operations:
if (!outfile) {
std::cerr << "Error opening file!" << std::endl;
}
This step is a safeguard against issues that may arise from incorrect file paths or permissions.
Handling Write Errors
Common write errors may occur if files are read-only, or if system permissions are restricted. To mitigate risks, structure your code to handle potential exceptions gracefully. Implementing proper error handling practices leads to more robust applications.
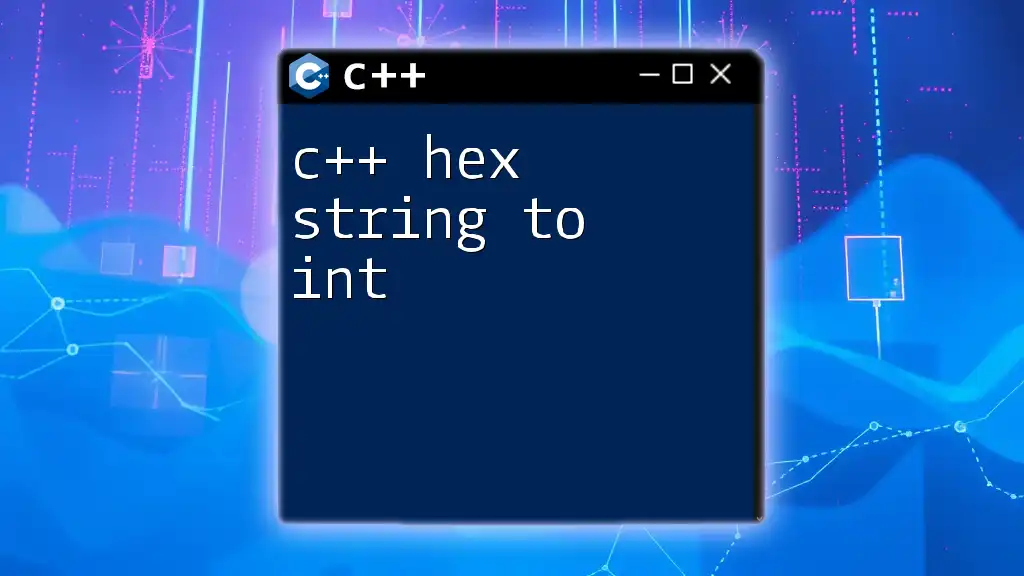
Closing the File
Importance of Closing Files
Once all operations are complete, it is critical to close the file. This action releases the file resources back to the system and ensures that all buffered data is flushed to the file. Neglecting this can lead to data loss or file corruption. The following example demonstrates how to close a file:
outfile.close();
Always remember to perform this step to maintain good resource management in your applications.
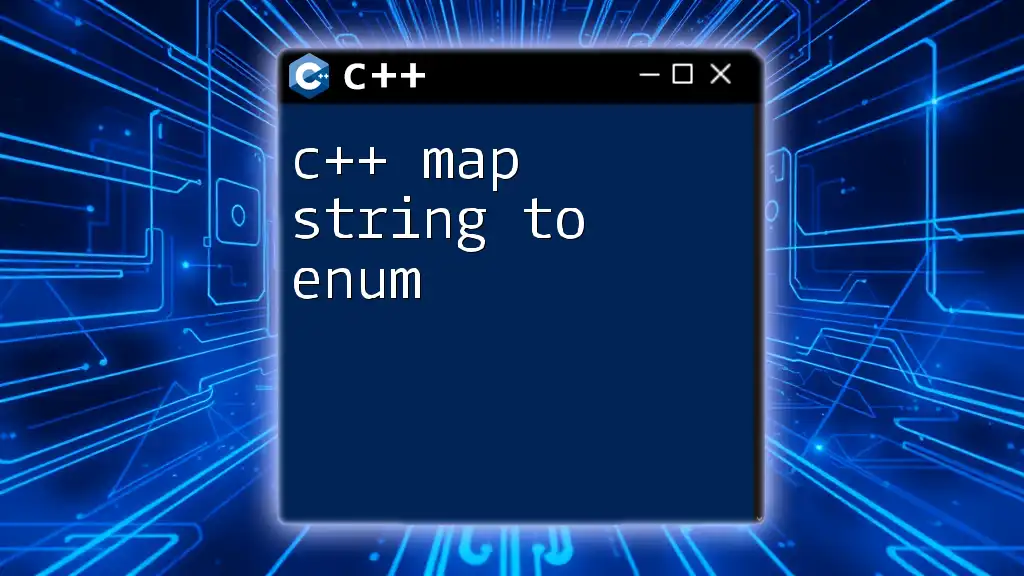
Reading the Written File (Bonus Section)
Confirming String Write
To verify that writing was successful, you can read from the file you just created. This operation is straightforward and is performed using `std::ifstream`. Below is a code snippet illustrating how to read and display the contents of a file:
#include <fstream>
#include <iostream>
#include <string>
int main() {
std::ifstream infile("example.txt");
std::string line;
while (std::getline(infile, line)) {
std::cout << line << std::endl;
}
infile.close();
return 0;
}
This example goes through each line of `example.txt`, printing it to the console, confirming the success of previous write operations.
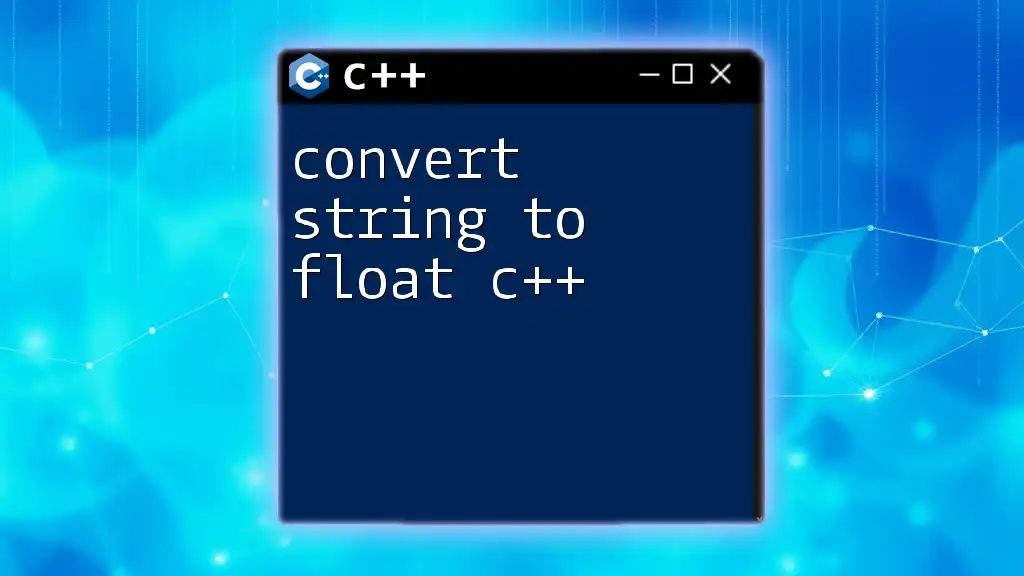
Conclusion
In this guide, we delved into the crucial aspects of how to use C++ to write strings to a file. We covered topics ranging from setting up your environment to basic writing operations, error handling, and even reading from files. Understanding these components allows you to handle file I/O effectively, enhancing your overall programming skills in C++.
Additional Resources
For further reading and exploration, consider the following resources:
- Books for a deep dive into C++ file handling.
- Online Courses that provide hands-on practice.
- C++ Communities where you can ask questions and share knowledge.
Armed with these tools and knowledge, you are now well-equipped to begin your journey into file manipulation in C++. Happy coding!