In C++, you can use a `std::map` to associate string keys with enum values for efficient lookups and type safety, as demonstrated in the following code snippet:
#include <iostream>
#include <map>
#include <string>
enum Color { RED, GREEN, BLUE };
int main() {
std::map<std::string, Color> colorMap = {
{"red", RED},
{"green", GREEN},
{"blue", BLUE}
};
// Example usage
std::string input = "green";
Color color = colorMap[input];
std::cout << "The enum value for " << input << " is " << color << std::endl;
return 0;
}
What is a Map in C++?
In C++, a map is a container that stores elements as key-value pairs. It provides the functionality to access values via unique keys, allowing for fast retrieval. Maps are particularly useful when you want to associate a unique identifier (the key) with some value.
Importance of Using Maps in C++
Using maps in C++ presents numerous advantages over other data structures such as arrays or lists:
- Fast Lookup: Maps provide average time complexity of O(log n) for search, insertion, and deletion operations.
- Dynamic Size: Unlike arrays, maps can grow dynamically to accommodate new elements without a predefined size.
- Clear Semantics: The use of key-value pairs makes code easier to understand and maintain.
Common use cases for maps include maintaining associations such as usernames with user data, product IDs with information, or, in our case, strings with enum values.
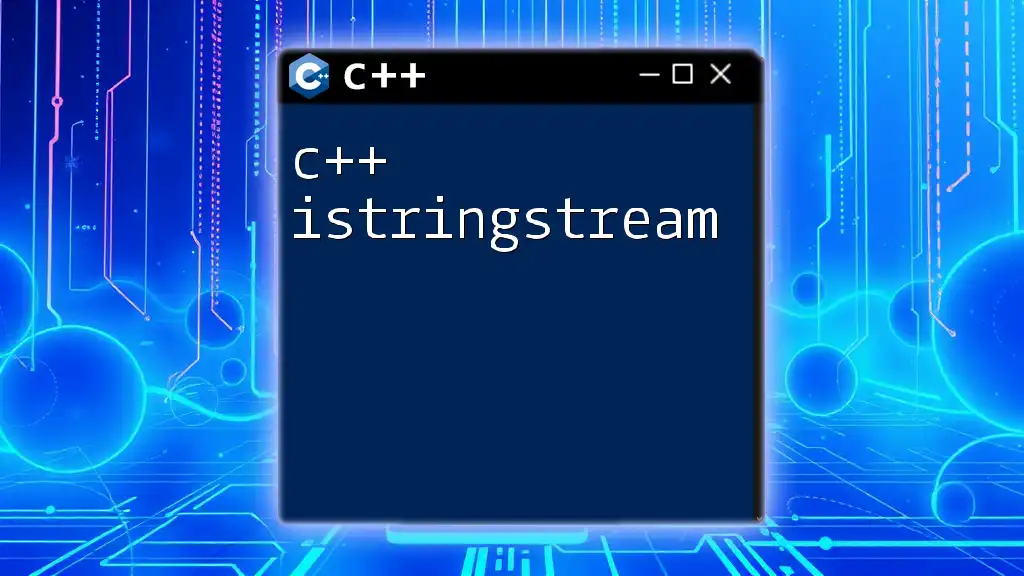
Understanding Enums in C++
What is an Enum?
An enum (enumeration) is a user-defined data type in C++ that consists of integral constants. It is a way to assign symbolic names to a set of related values, enhancing the clarity of code. Enums effectively increase the readability and maintainability of your programs.
Creating and Using Enums
Defining an enum is straightforward. Here's an example:
enum Color { RED, GREEN, BLUE };
In this snippet, we define an `enum` called `Color` with three potential values: RED, GREEN, and BLUE. When using enums instead of integer constants, you will avoid errors related to using incorrect values in your code.
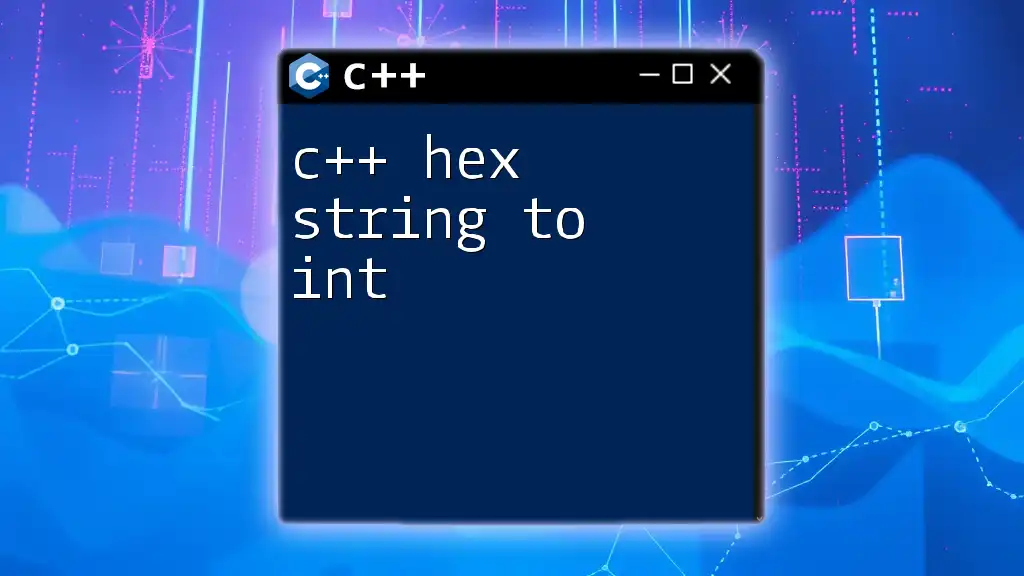
Mapping Strings to Enums
Why Map Strings to Enums?
Mapping strings to enums is essential when you want to convert user-friendly representations (like color names) into programmatic representations (like enum values). This approach is especially useful in applications like configuration files, user input, or GUI-related tools, where string inputs are more natural for users.
Implementing String to Enum Mapping
The simplest way to implement string-to-enum mapping in C++ is by using the `std::map` container. Below, we go through a practical example to illustrate this mapping.
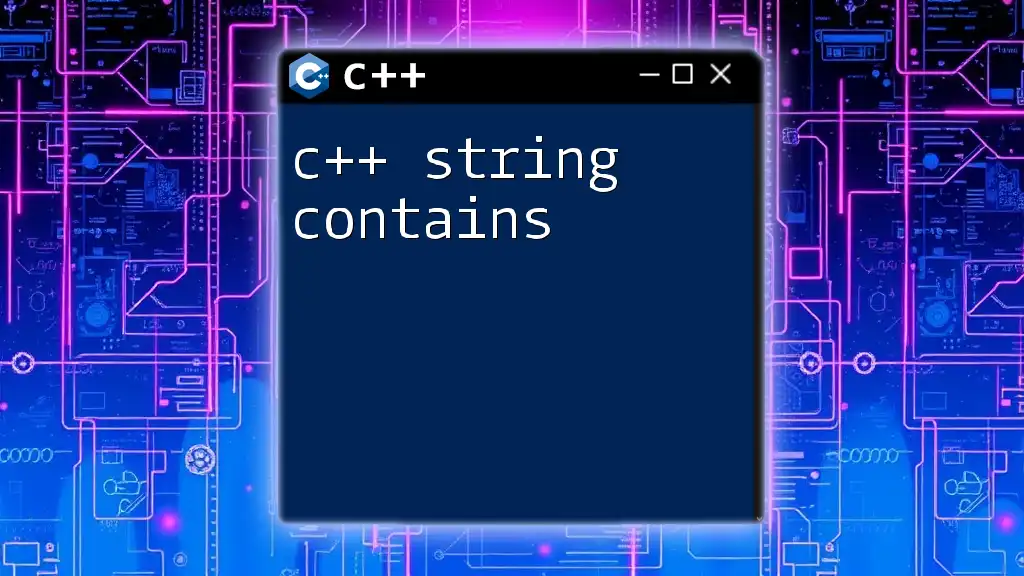
Using `std::map` for String to Enum Mapping
Creating a Map for String to Enum
To create a map that associates strings with enum values, you can use the following syntax:
#include <iostream>
#include <map>
#include <string>
enum Color { RED, GREEN, BLUE };
std::map<std::string, Color> colorMap = {
{"red", RED},
{"green", GREEN},
{"blue", BLUE}
};
In this code, we define a `std::map` called `colorMap` that links string representations of colors to their corresponding enum values.
Accessing Enum Values Using Strings
Retrieving enum values using the associated strings is straightforward. For example, you can access a color as follows:
Color c = colorMap["red"];
This line of code retrieves the enum value `RED` by looking it up via the string "red".
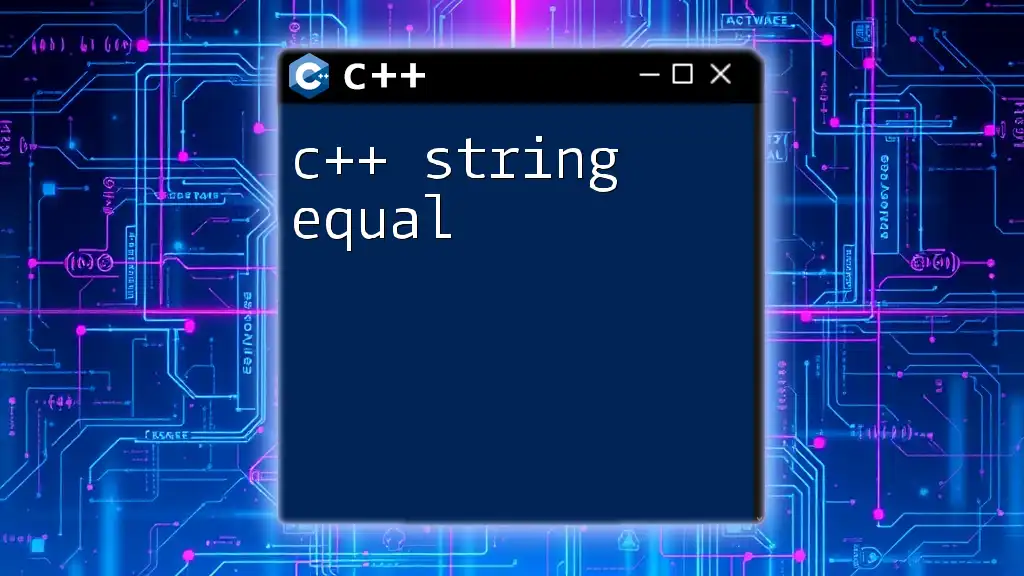
Handling Invalid String Inputs
Validating String Inputs
It's vital to handle cases where the input string might not correspond to an existing enum value. Ensuring input validation prevents runtime errors and improves the robustness of your program.
Example of Error Handling
One effective way to handle invalid inputs is by using the `std::map::find` method, which allows you to check if a key exists before accessing its value:
std::string colorName = "yellow";
auto it = colorMap.find(colorName);
if (it != colorMap.end()) {
Color c = it->second; // Valid color
} else {
std::cout << "Error: Color not found!" << std::endl; // Invalid color
}
In this example, if "yellow" isn't found in the map, the program outputs an error message instead of crashing or accessing a non-existent value.
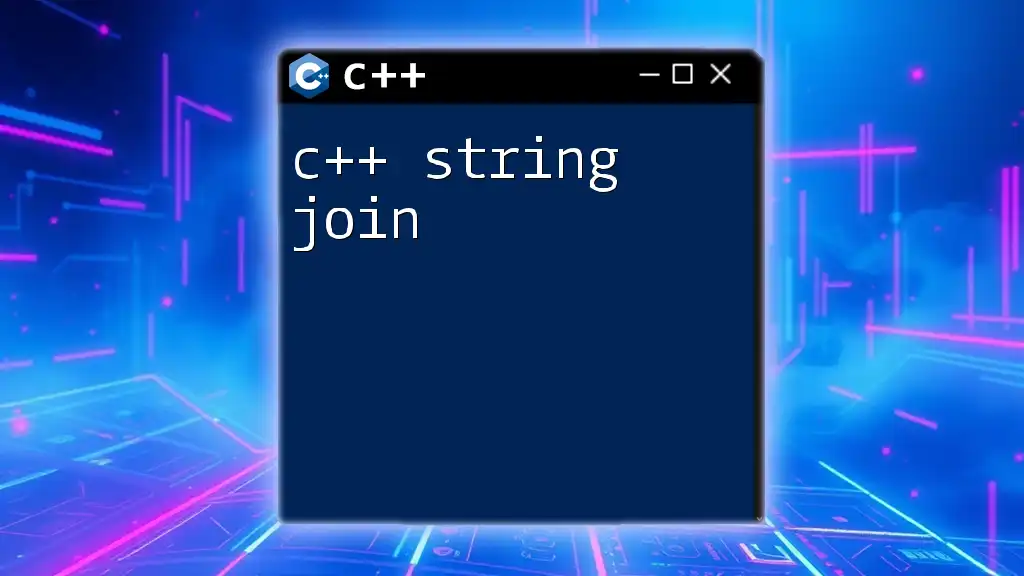
Advanced Techniques in Mapping
Using `std::unordered_map` for Efficiency
While `std::map` provides good performance, sometimes you can benefit from using an `std::unordered_map`. The latter uses a hash table for storage, achieving average time complexity for operations as O(1), offering faster lookups when the dataset is large.
Custom Hash Functions for Enums
If you decide to go with `std::unordered_map`, you can implement custom hash functions to enhance performance when using user-defined types like enums. This optimization technique can significantly speed up lookups compared to default behavior.
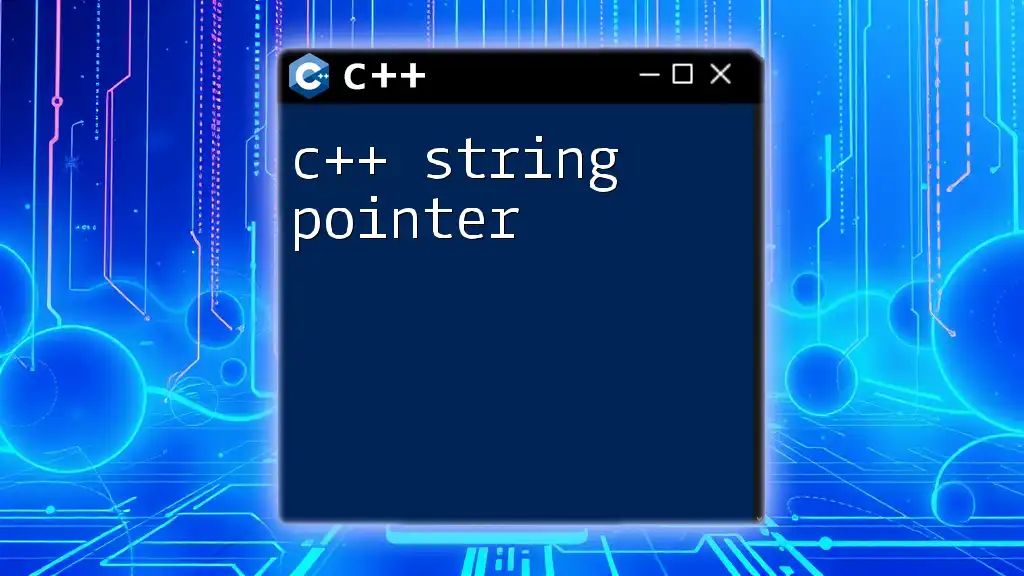
Conclusion
In summary, efficiently mapping strings to enums in C++ opens various possibilities for your applications. By understanding data structures like `std::map` and implementing robust error handling techniques, you can create maintainable code that is both user-friendly and error-resistant.
Further Reading and Resources
For further enhancement of your skills in C++, consider diving into more advanced topics such as templates, polymorphism, and data structures. Resources such as textbooks, online courses, and community forums can provide deeper insights and valuable knowledge to refine your coding capabilities.