C++ compiler support refers to the compatibility of various C++ features and standards across different compilers, which can impact how code is written and executed.
Here’s a simple code snippet demonstrating the use of the `#include` directive, which is widely supported across C++ compilers:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
What is a C++ Compiler?
A C++ compiler is a specialized tool that translates C++ code into machine-readable code. Its primary purpose is to transform the human-readable programming language into object code that the computer can execute. The process of compilation involves several stages, each with its functions:
- Lexer: Converts the sequence of characters into tokens.
- Parser: Analyzes the token sequence to form a parse tree.
- Semantic Analyzer: Validates the parse tree against the grammar of the language.
- Code Generator: Produces the final output in the form of machine code.
Understanding the components and the workflow of a C++ compiler is crucial for anyone looking to write efficient C++ code.
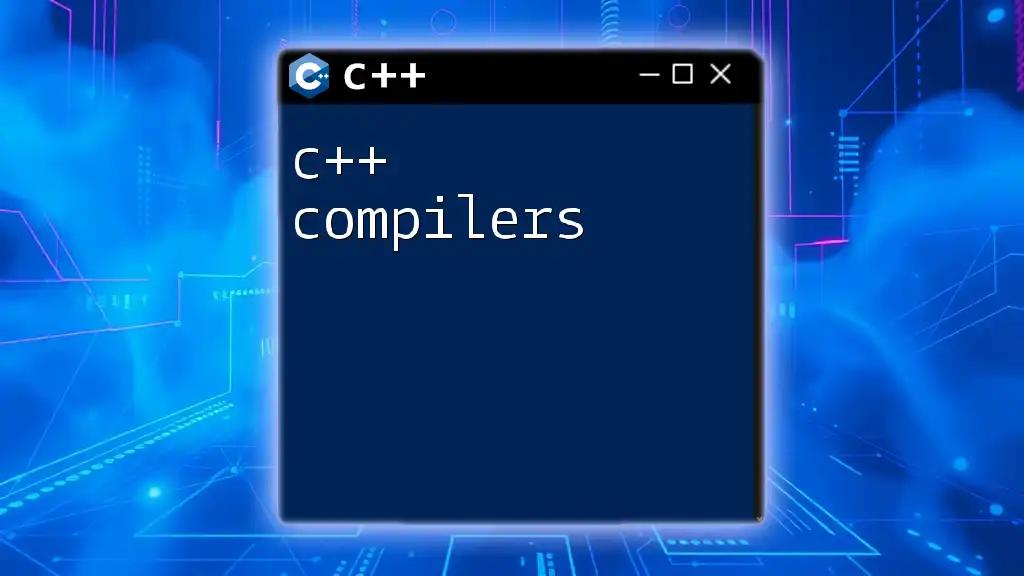
Types of C++ Compilers
Native Compilers
Native compilers are designed to generate machine code that is specific to the host system. This means that the compiled code will run directly on the target machine without the need for further translation or interpretation.
- Example: GCC (GNU Compiler Collection)
GCC is widely regarded for its robustness and efficiency. It supports various standards of the C++ language, including C++11, C++14, C++17, and C++20. Its ability to optimize code for a wide range of platforms makes it a popular choice among developers.
To use GCC, you can invoke it via the command line like this:
g++ my_program.cpp -o my_program
This command compiles `my_program.cpp` and generates an executable named `my_program`.
Interpreted Compilers
Interpreted compilers convert source code into intermediate code and execute it, rather than producing standalone machine code. This allows for dynamic execution but often results in slower performance compared to native compilation.
- Example: Clang
Clang is another highly optimized C++ compiler that is part of the LLVM project. It focuses on offering advanced optimizations and improved diagnostics.
Using Clang, you can compile a program similarly to GCC:
clang++ my_program.cpp -o my_program
Cross-compilers
Cross-compilers are capable of creating executable code for a platform different from the one they are run on. This is particularly useful for embedded systems or when the development environment differs from the target environment.
- Example: MinGW (Minimalist GNU for Windows)
MinGW is a popular cross-compiler that allows developers to generate Windows executables on other platforms. This flexibility is essential for developing applications that must run on different operating systems.
To compile with MinGW, you would typically do:
x86_64-w64-mingw32-g++ my_program.cpp -o my_program.exe
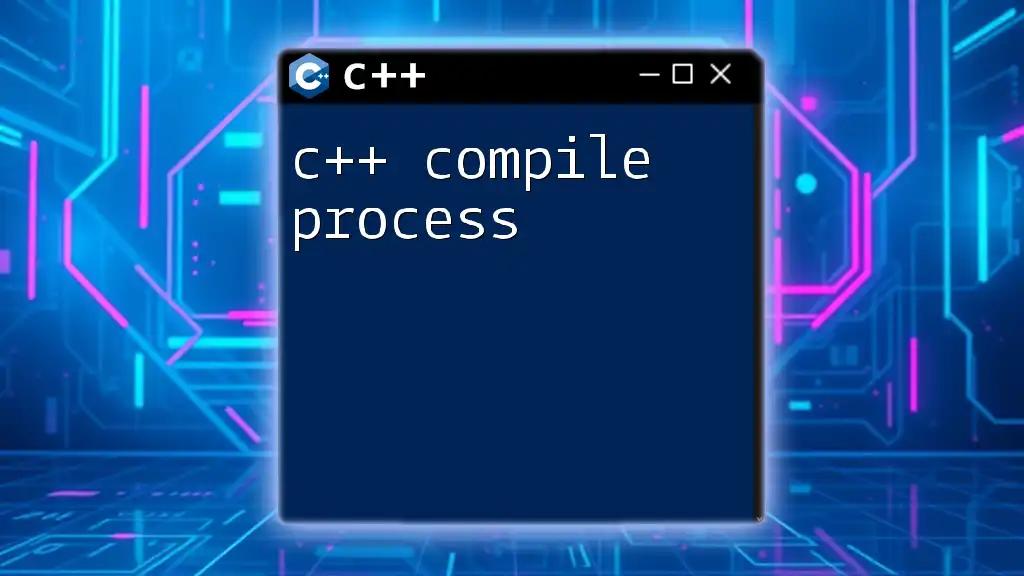
Popular C++ Compilers and Their Features
GCC
GCC is one of the most established and versatile C++ compilers available. It boasts support for all modern C++ standards as well as features like:
- Multi-threading support
- Optimizations for both speed and size
- Strong community and extensive documentation
Installing GCC is straightforward on most systems, usually via package managers. For example, on Ubuntu, one can simply run:
sudo apt install g++
Microsoft Visual C++ (MSVC)
MSVC is integral to C++ development on Windows and is part of the Microsoft Visual Studio suite. It offers features like:
- Rich debugging and diagnostic tools
- Integrated development environment (IDE) support
- Extensive libraries for Windows application development
A sample command to compile a simple program in MSVC would be:
cl my_program.cpp
Clang
Clang is known for its excellent compatibility with GCC, while offering additional performance enhancements and clearer error messages. It is particularly useful for projects that depend heavily on LLVM-based optimizations.
You can use Clang to compile your code with:
clang++ my_program.cpp -o my_program
Intel C++ Compiler
Targeted primarily at high-performance computing, the Intel C++ Compiler provides advanced optimizations for Intel processors. It is known for specific features like:
- Vectorization and multi-threading support
- Advanced optimization techniques, especially for numerical applications
Though this compiler is not free, it is invaluable for developers looking for cutting-edge performance.

How to Choose the Right Compiler
When selecting a C++ compiler, consider the following factors:
-
Project Requirements: Evaluate if you need cross-platform capabilities, advanced optimizations, or certain library dependencies.
-
Target Platform: Your choice might depend on whether you are developing for Windows, Linux, or MacOS. Compiler support may vary across platforms.
-
Community Support: Ensure you choose a compiler with active community or professional support, as it can significantly ease the learning curve.
Compiler Compatibility
Sticking to standard C++ ensures that your code is portable and can be compiled with different compilers without modification. Be cautious of proprietary extensions that might lock you into a single compiler ecosystem and restrict your code's portability.
Future-proofing
Selecting a compiler that continuously updates to support new C++ standards is vital for ensuring your projects remain relevant. Keeping abreast with the latest developments in the C++ ecosystem can help you select a tool that will grow with your needs.

Compiling C++ Code: A Step-by-Step Guide
Setting Up Your Environment
Before you begin compiling C++ code, you need to set up your development environment. Depending on your chosen compiler, you may need to install specific tools and configure your IDE. Popular IDEs for C++ development include Visual Studio, Code::Blocks, and Eclipse—all of which integrate seamlessly with different compilers.
Writing a Simple C++ Program
Here's a simple program to demonstrate the basics of C++:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
This traditional "Hello, World!" program serves as an excellent starting point for beginners.
Compiling Using Different Compilers
Using GCC
To compile your program using GCC, navigate to your terminal and execute:
g++ hello.cpp -o hello
Using MSVC
In a Visual Studio Developer Command Prompt, you can compile your code with:
cl hello.cpp
Using Clang
Using Clang, the command is almost identical to that of GCC:
clang++ hello.cpp -o hello

Common Compilation Errors and How to Fix Them
Syntax Errors
Syntax errors occur when the code violates the language's grammar rules. For instance, forgetting a semicolon can lead to a compilation failure. Always check your error messages to pinpoint the exact line and nature of the error.
Linking Errors
Linking errors usually arise when the compiler cannot locate the functions or variables referred to in your code. This often occurs when you forget to include a necessary library. Carefully review all library dependencies and ensure they are properly linked during compilation.
Runtime Errors
Runtime errors do not appear during compilation but occur when the program is running, typically due to logic errors, such as dereferencing a null pointer. Utilize debugging tools within your IDE to step through your code and identify the problem areas.
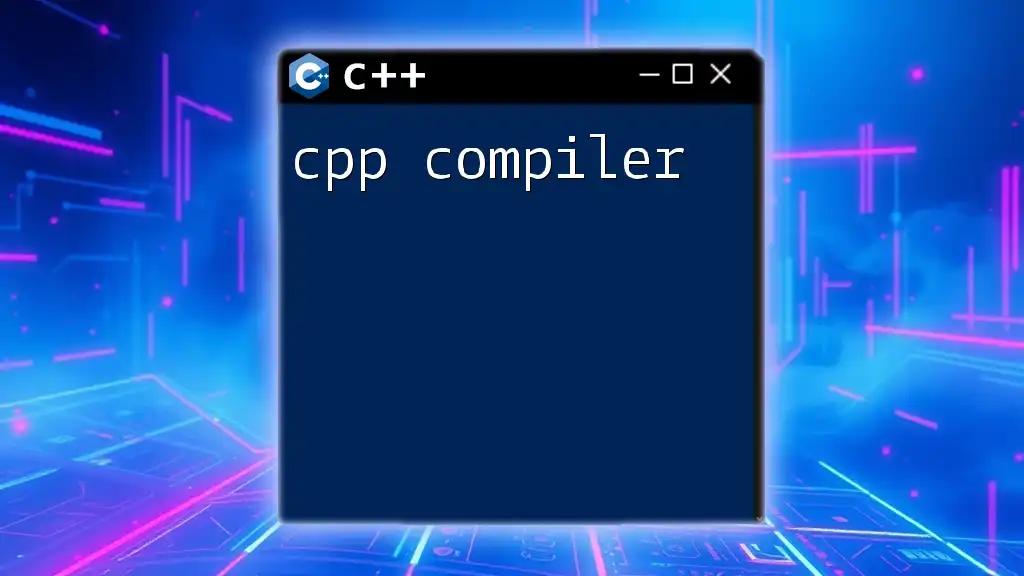
Conclusion
Understanding C++ compiler support is key for any developer striving for efficiency and portability in their code. Different compilers offer unique features, and selecting the right one can impact both your development experience and performance. Experimenting with various compilers can enhance your programming skills and increase your adaptability in a fast-evolving language landscape. For those looking to deepen their understanding of C++, consider joining our teaching platform for further insights into using C++ commands effectively.
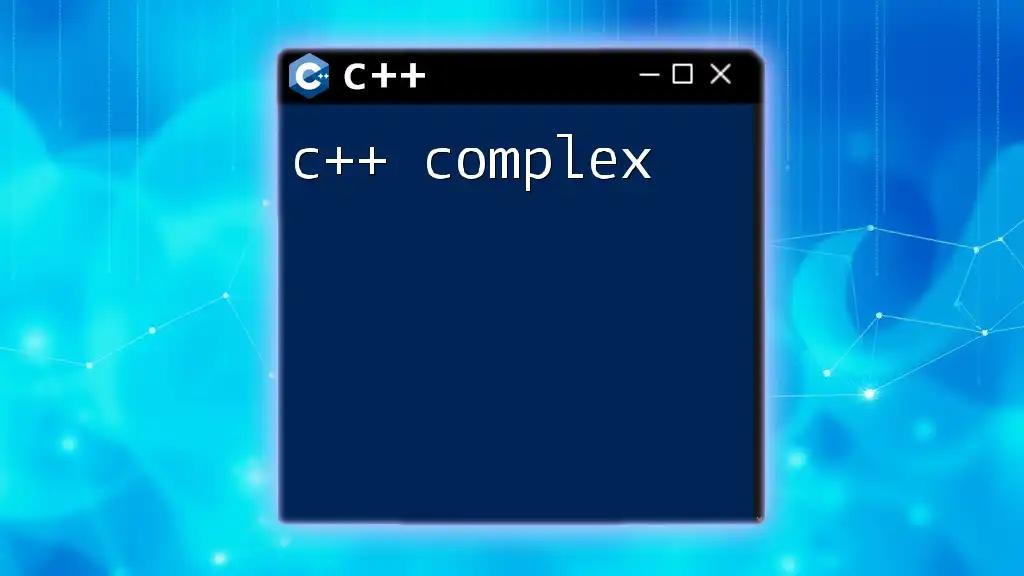
Additional Resources
For further information, please refer to the official documentation for each compiler mentioned, as well as recommended books and online courses tailored for C++ development. Keeping abreast of community contributions can further enhance your learning and usage of C++.