The `+` operator in C++ is used to perform addition on numeric types or to concatenate strings when applied to objects of user-defined types that implement the operator overloading for `+`.
Here's a simple example of using the `+` operator with integers and strings:
#include <iostream>
#include <string>
int main() {
// Using + operator with integers
int a = 5, b = 10;
int sum = a + b;
std::cout << "Sum: " << sum << std::endl;
// Using + operator with strings
std::string str1 = "Hello, ";
std::string str2 = "World!";
std::string greeting = str1 + str2;
std::cout << "Greeting: " << greeting << std::endl;
return 0;
}
What is the Operator+ in C++?
The C++ operator+ is a fundamental operator used for addition. It enables the addition of two operands, be they primitive data types such as integers and floating-point numbers, or user-defined types like classes. The ability to define how addition operates between objects makes it a powerful feature in C++, allowing programmers to create intuitive interfaces.
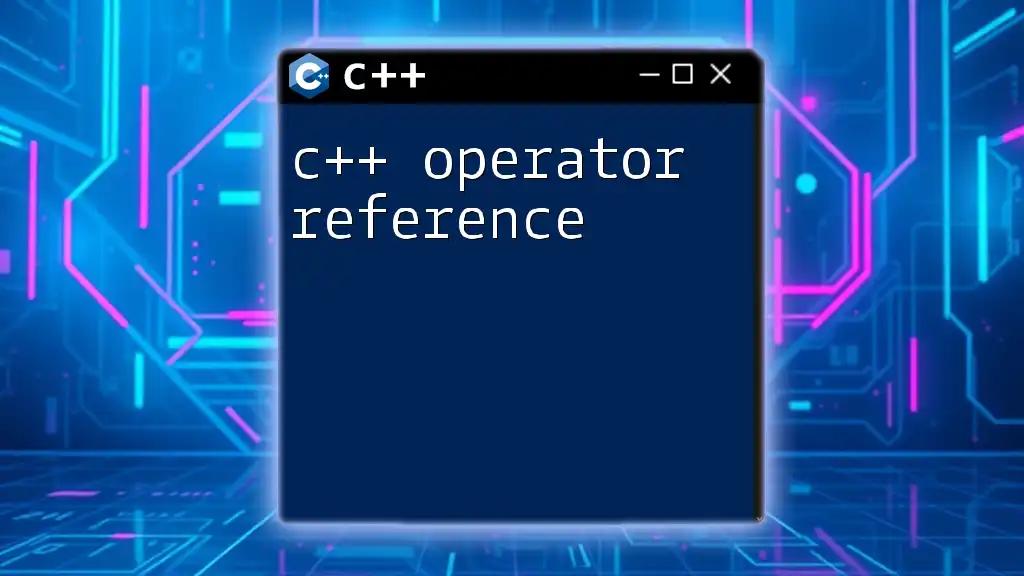
Understanding Operator Overloading
What is Operator Overloading?
Operator overloading is a feature in C++ that allows developers to redefine the way an operator works for user-defined types. By overloading operators, you can use standard operators like +, -, and * in ways that make sense for your custom classes.
Operator Overloading Syntax
To overload an operator, you typically define a function within a class. The syntax for this looks like:
ReturnType ClassName::operatorSymbol(Parameters) {
// implementation
}
For example, the following code demonstrates how to overload the subtraction operator (-):
class Example {
public:
Example operator-(const Example& obj) {
// implementation to subtract obj from current instance
}
};
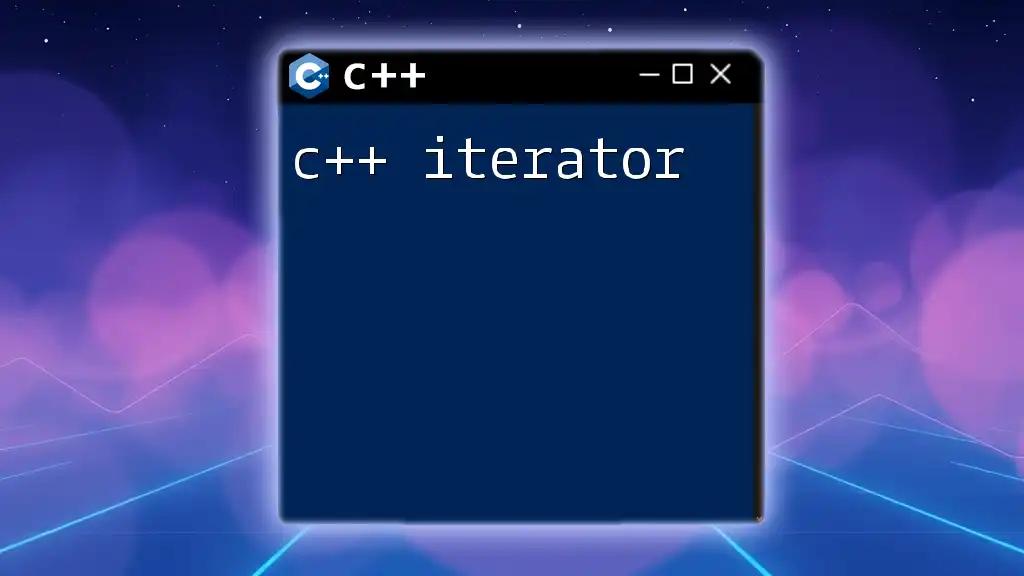
Implementing Operator+
Using Operator+ for Built-in Types
The C++ operator+ can seamlessly work with built-in types like integers, floating-point numbers, and even character types. Consider the following example that adds two integers:
int a = 5;
int b = 10;
int sum = a + b; // Returns 15
In this case, the `operator+` is used as expected, providing the numerical sum of `a` and `b`. This straightforward usage mimics the intuitive math concept of addition, which is one of the key benefits of having built-in operator functionality.
Using Operator+ for User-Defined Types
Creating a Class
When using the C++ operator+ with user-defined types, you first need to create a class. For instance, we can create a simple class `Vector` that represents a vector in 2D space:
class Vector {
public:
int x, y;
Vector(int x, int y) : x(x), y(y) {}
};
Overloading Operator+
Now, let's implement the `operator+` for our `Vector` class. This will allow us to add two vectors together by adding their respective x and y components.
class Vector {
public:
int x, y;
Vector(int x, int y) : x(x), y(y) {}
Vector operator+(const Vector& v) {
return Vector(this->x + v.x, this->y + v.y);
}
};
Code Explanation
In the `operator+` implementation, we define how to add two `Vector` instances. The method takes another `Vector` as a parameter and returns a new `Vector` object that represents the combined values. This design pattern promotes the principle of immutability since the original vectors remain unchanged.
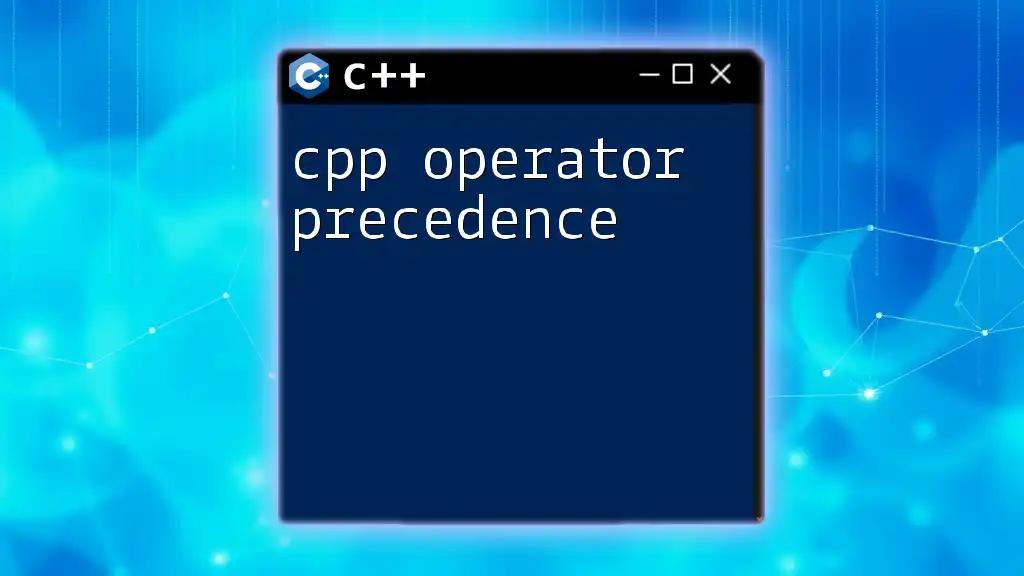
Practical Examples of Operator+
Example 1: Adding Complex Numbers
To deepen our understanding of the C++ operator+, let’s create a class to handle complex numbers and overload the addition operator. A complex number consists of a real and an imaginary part.
class Complex {
public:
float real, imag;
Complex(float r, float i) : real(r), imag(i) {}
Complex operator+(const Complex& c) {
return Complex(this->real + c.real, this->imag + c.imag);
}
};
In this example, the overloaded `operator+` combines both the real and imaginary parts of two complex numbers, providing an intuitive interface for adding them, similar to how you would do it mathematically.
Example 2: Concatenating Strings
Another useful application of the C++ operator+ is string manipulation. Let’s demonstrate this by overloading the addition operator to concatenate strings in a custom class.
class MyString {
private:
std::string str;
public:
MyString(const std::string &s) : str(s) {}
MyString operator+(const MyString& s) {
return MyString(this->str + s.str);
}
};
In this case, when you add two `MyString` objects, the `operator+` will combine their internal string representations into a new `MyString` object. This makes string manipulation more intuitive and aligns with how we naturally think about concatenating strings.
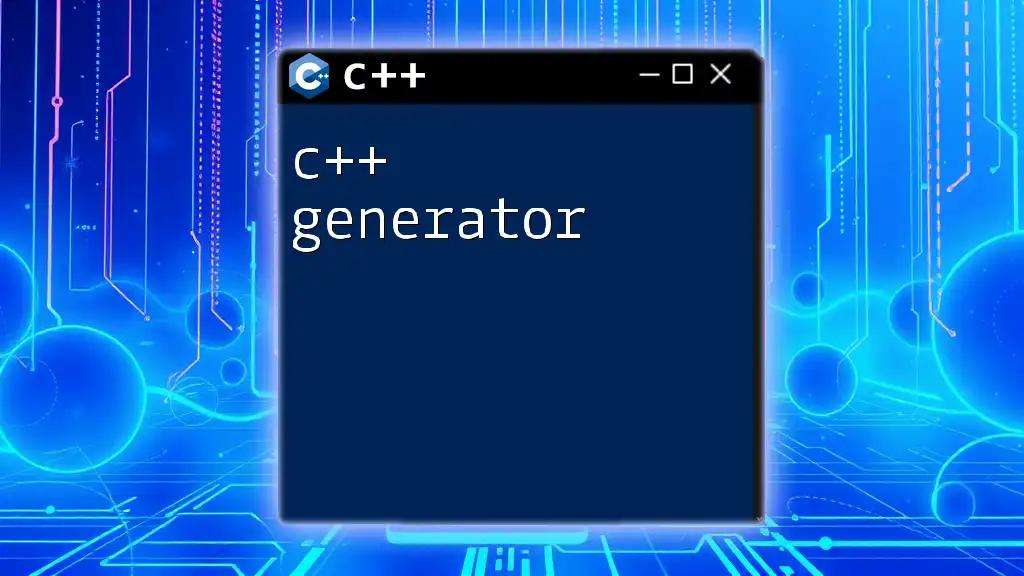
Common Pitfalls When Using Operator+
When implementing the C++ operator+, you may encounter several common pitfalls:
- Misleading Results: If the overloaded operator does not behave as users expect, it can lead to confusion. Always ensure the operation is consistent with conventional mathematical principles.
- Performance Considerations: Overloading operators may introduce performance overhead, especially if you are creating unnecessary copies of objects. It’s crucial to consider whether you need to return new objects or modify existing ones.
- Not Returning New Objects: If you do not return a new object in operators that function similarly to addition, the original objects might not reflect their intended changes, which can result in unexpected behaviors.

Best Practices for Overloading Operators
To make the most of operator overloading, adhere to these best practices:
- Clarity and Consistency: Ensure your overloaded operators behave as users would intuitively expect. If you overload the addition operator, it should only do what addition logically implies.
- Minimal Side Effects: When overloading `operator+`, aim to perform operations without altering the state of the involved objects, promoting immutability.
- Document Behavior: Clearly document how your overloaded operators work, especially if they deviate from standard behavior.
![Unlocking the C++ [] Operator: A Step-by-Step Guide](/_next/image?url=%2Fimages%2Fposts%2Fc%2Fcpp-operator.webp&w=1080&q=75)
Conclusion
In summary, understanding the C++ operator+ and the principles of operator overloading can significantly enhance your programming practices. Mastering this feature allows for more intuitive object manipulation and cleaner code, making it an essential skill for any C++ programmer. Don’t hesitate to experiment with your own classes and explore the endless possibilities that operator overloading can offer in your software development journey.
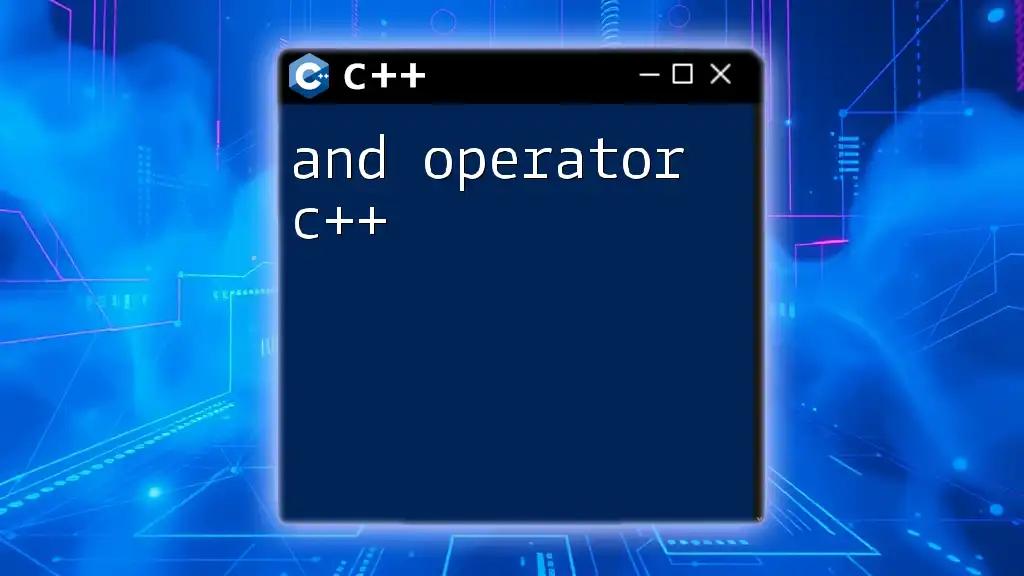
Additional Resources
For those eager to expand their knowledge further on C++ operator+ and overall C++ programming, consider exploring books such as "The C++ Programming Language" by Bjarne Stroustrup, engaging with online tutorials, or participating in forums to learn from experienced developers.
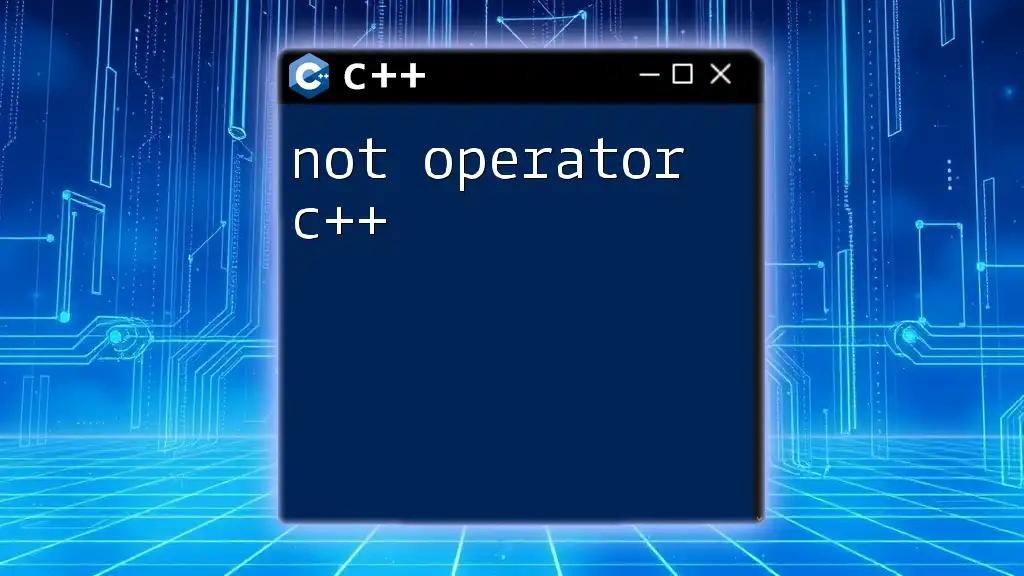
FAQs
-
What types can use `operator+`? Any data type, both built-in and user-defined, can utilize operator overloading.
-
Can `operator+` be overloaded for more than two operands? No, operator overloading in C++ is limited to binary operations, so each use of `operator+` operates on two operands at a time.
-
What happens if I don't return a new object in `operator+`? If you modify the same object instead of returning a new one, you might introduce unexpected behavior, making the code less intuitive. It goes against the principle of immutability, which is often beneficial in high-level programming concepts.