In C++, Object-Oriented Programming (OOP) is a paradigm that uses objects and classes to structure software in a way that promotes code reusability and organization.
Here's a simple example of a class and object in C++:
#include <iostream>
using namespace std;
class Dog {
public:
void bark() {
cout << "Woof!" << endl;
}
};
int main() {
Dog myDog;
myDog.bark(); // Output: Woof!
return 0;
}
What is OOP?
Object-Oriented Programming (OOP) is a programming paradigm that brings together data and the methods that operate on that data in a cohesive manner. Unlike procedural programming, which relies heavily on functions and sequences of actions, OOP focuses on objects as its fundamental building blocks. This model enhances modularity, code reuse, and the abstraction of complex systems, thus making it easier to manage and scale software solutions.
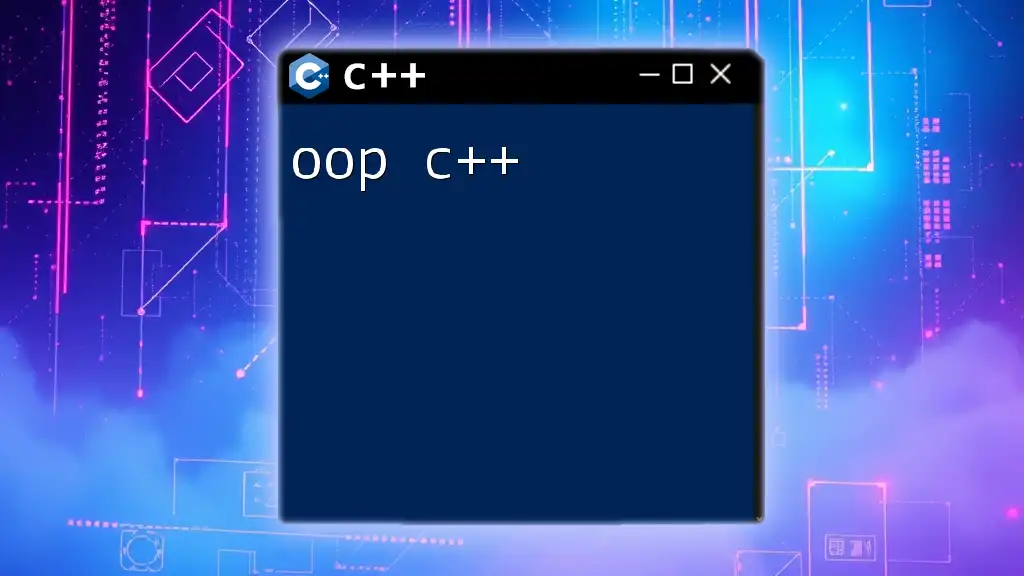
Why C++ for OOP?
C++ was designed with OOP in mind, allowing developers to leverage its powerful features to create flexible and maintainable software. The transition from C to C++ marked a significant shift, enabling programs to be modeled more intuitively through the concepts of classes and objects. The advantages of using C++ for OOP include:
- Performance Efficiency: C++ offers low-level manipulation capabilities, making it efficient for performance-critical applications.
- Rich Standard Library: It includes a vast array of functions and classes that streamline OOP implementation.
- Multi-Paradigm Approach: While inherently OOP, C++ allows for procedural paradigm features, giving developers the flexibility to choose their coding style.
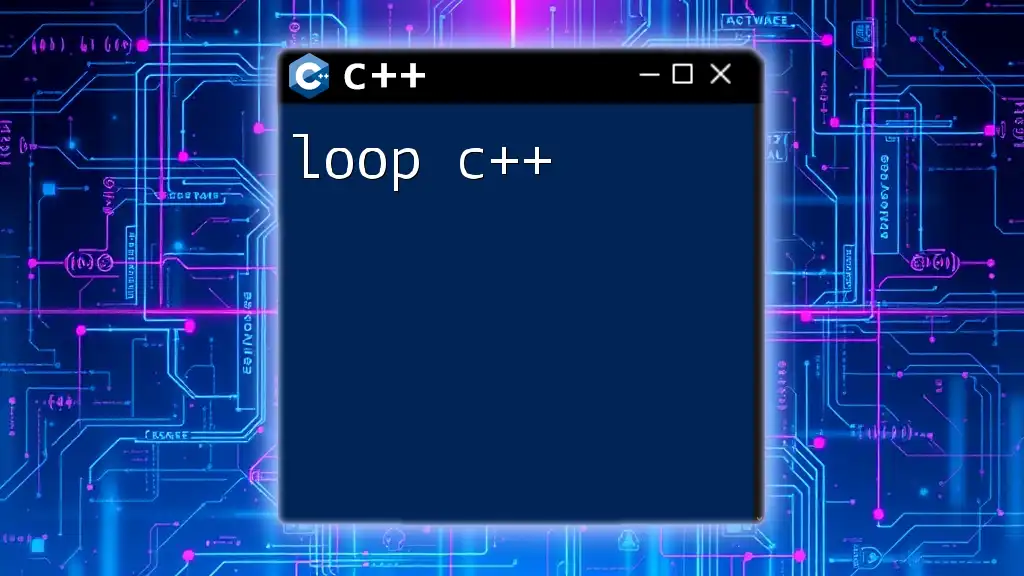
Key Concepts of OOP
Classes and Objects
Classes are blueprints for creating objects. An object is an instance of a class that encapsulates both state and behaviors.
Here’s a simple example of a class representing a `Dog`:
class Dog {
public:
void bark() {
std::cout << "Woof!" << std::endl;
}
};
In this example, the `Dog` class defines a `bark` method that displays a barking sound. To utilize this class, you can create an object:
Dog myDog;
myDog.bark(); // Outputs: Woof!
Encapsulation
Encapsulation is the principle of bundling the data (attributes) and methods (functions) that operate on the data into a single unit known as a class. This concept also involves restricting direct access to some of an object’s components, which is at the heart of data hiding.
Here’s how encapsulation works with a `BankAccount` class:
class BankAccount {
private:
double balance;
public:
void deposit(double amount) {
balance += amount;
}
double getBalance() {
return balance;
}
};
In this example, `balance` is a private member variable that cannot be accessed directly from outside the `BankAccount` class. Instead, you utilize the `deposit` and `getBalance` methods to interact with the balance, ensuring that the data is modified and retrieved appropriately.
Abstraction
Abstraction in OOP is the process of hiding complex implementation details and exposing only the necessary parts of an object. This simplification allows developers to interact with an application at a high level without needing to understand the underlying mechanics.
Consider an abstract class `Shape`:
class Shape {
public:
virtual void draw() = 0; // Pure virtual function
};
Here, `Shape` serves as a base class defining the `draw` method, but you cannot create an instance of `Shape`. Instead, derived classes such as `Circle` or `Rectangle` would implement the `draw` function, thus promoting flexibility and extensibility in your code.
Inheritance
Inheritance allows a class to inherit properties and methods from another class. This powerful concept facilitates code reuse and establishes a natural hierarchy between classes.
You can implement single inheritance as follows:
class Animal {
public:
void eat() {
std::cout << "Eating..." << std::endl;
}
};
class Dog : public Animal {
public:
void bark() {
std::cout << "Woof!" << std::endl;
}
};
In this example, `Dog` inherits the `eat` method from `Animal`, allowing the `Dog` class to utilize this behavior without redefining it.
Additionally, multiple inheritance is possible in C++, allowing a derived class to inherit from more than one base class, but it must be utilized carefully to avoid ambiguity.
Polymorphism
Polymorphism is the ability of different classes to be treated as instances of the same base class, primarily through method overriding. It allows for dynamic method resolution at runtime.
Consider the following example demonstrating runtime polymorphism:
class Animal {
public:
virtual void sound() {
std::cout << "Generic sound" << std::endl;
}
};
class Cat : public Animal {
public:
void sound() override {
std::cout << "Meow" << std::endl;
}
};
In this code, both the `Animal` and `Cat` classes have a `sound` method. By using a pointer to the base class, you can call the correct derived class method at runtime:
Animal* myAnimal = new Cat();
myAnimal->sound(); // Outputs: Meow
This flexibility allows for more adaptable and scalable code.
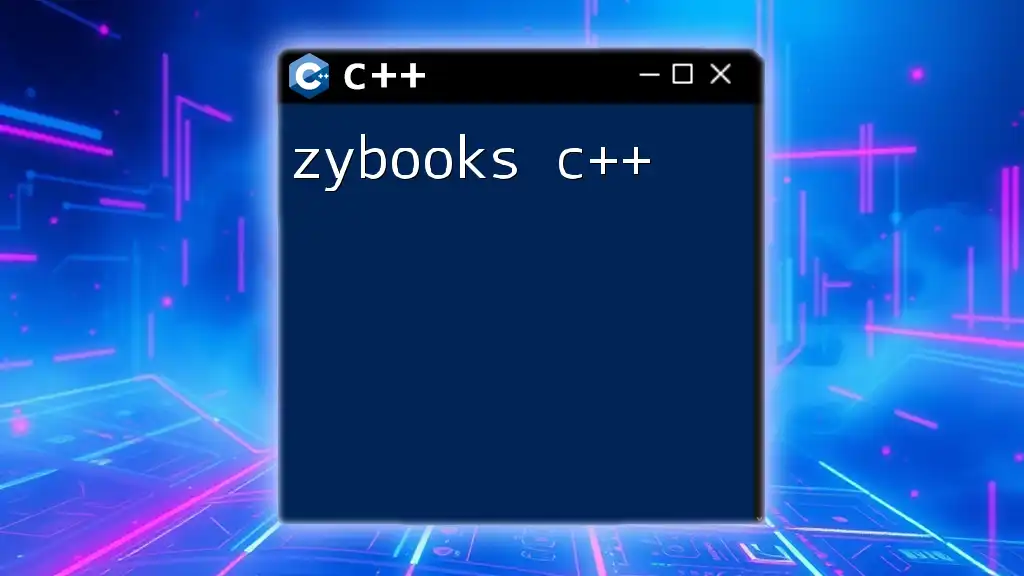
Practical Applications of OOP in C++
OOP principles can be applied effectively in various fields:
- Game Development: Designing objects like players, enemies, and items with behaviors and interactions allows for sophisticated gaming experiences.
- Enterprise Software Solutions: Modular design is essential for managing complex systems, facilitating updates and maintenance.
Developers frequently encounter complex systems, and the application of OOP principles can lead to organized codebases. By segregating responsibilities into classes, it becomes easier for teams to collaborate and manage the code.
Common OOP Design Patterns
Understanding OOP design patterns can significantly enhance your programming efficiency. Design patterns are standard solutions to common problems in software design.
A popular design pattern is the Strategy Pattern, which allows selecting an algorithm's behavior at runtime. Here’s a simple leveraging of this pattern:
class Strategy {
public:
virtual void execute() = 0; // Pure virtual function
};
class ConcreteStrategyA : public Strategy {
public:
void execute() override {
std::cout << "Strategy A" << std::endl;
}
};
This approach helps decouple the algorithm from the clients that use it, promoting flexibility and minimizing changes in other parts of your codebase.
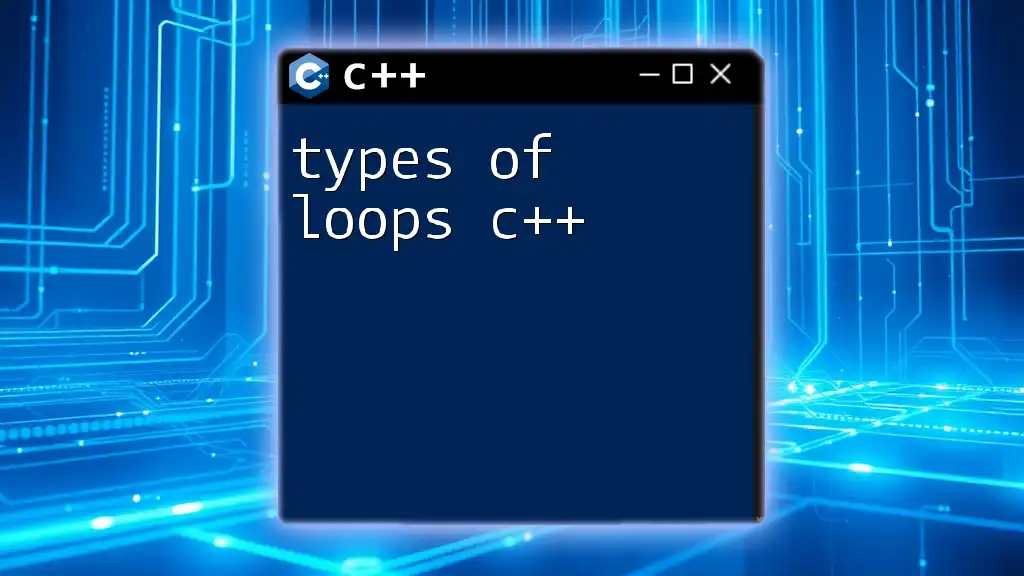
Best Practices for Implementing OOP in C++
To maximize the benefits of OOP, adhere to best practices:
-
Code Readability and Maintainability: Prioritize clear and descriptive naming for classes and methods. This clarity will help both you and others in the future.
-
Avoid Common Pitfalls: Don’t misuse inheritance indiscriminately. Favor composition over inheritance where possible to prevent tightly-coupled designs.
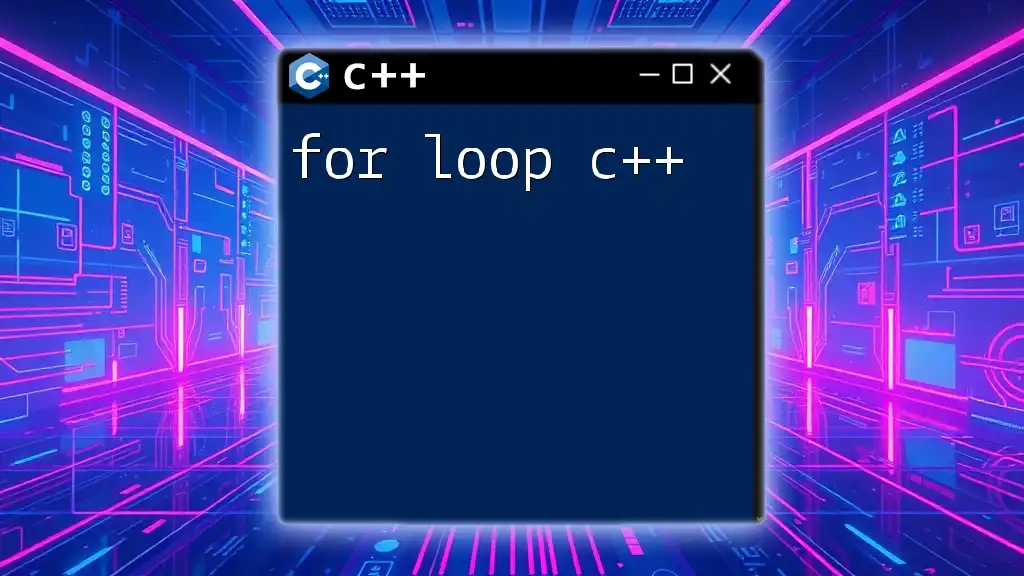
Conclusion
In summary, OOP enhances how developers design and structure their applications, promoting modularity and reusability. The fundamental concepts—classes, encapsulation, abstraction, inheritance, and polymorphism—form a coherent framework that allows for more structured and scalable code.
As you delve deeper into C++, continuously explore resources that can augment your understanding of OOP principles. Engaging with online forums, attending workshops, and contributing to projects can further develop your proficiency.
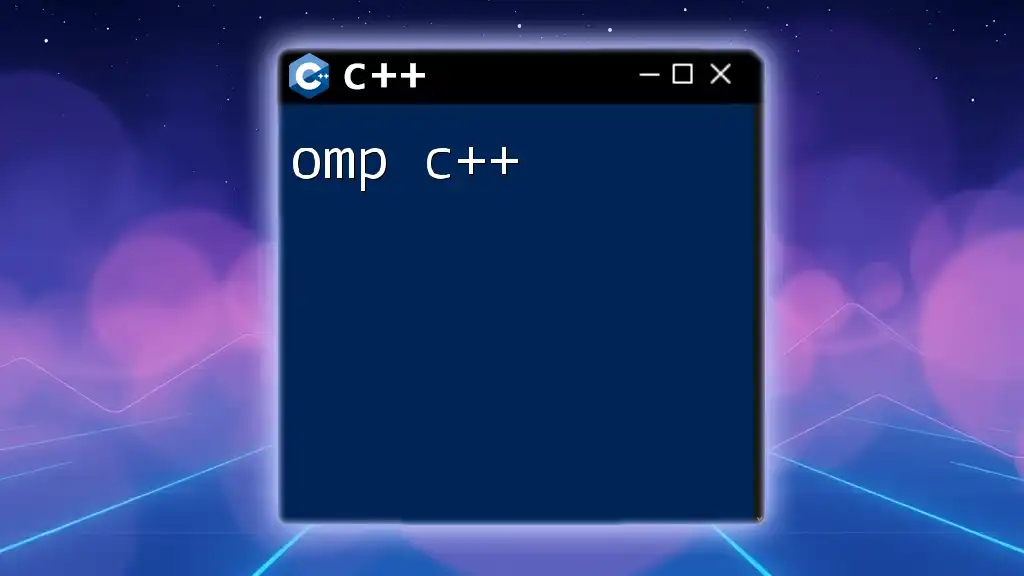
Call to Action
We encourage you to share your experiences with OOP and C++ within our community. Join us in our upcoming workshops and tutorials to enhance your skills and connect with fellow learners passionate about object-oriented programming!