Zybooks C++ is an interactive learning platform that provides students with engaging content and programming challenges to help them master C++ concepts quickly and effectively.
Here's a simple code snippet demonstrating the basic syntax of a C++ program:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
What is zyBooks?
zyBooks is a revolutionary platform designed to enhance the educational experience in various subjects, particularly in programming. It combines text, animations, and interactive elements to foster a more engaging learning environment. zyBooks focuses on ensuring that students not only absorb information but also actively participate in their learning journey. The C++ module of zyBooks is tailored to equip learners with the necessary skills to excel in the programming language, emphasizing both theoretical understanding and practical application.
The Importance of Learning C++
As technology continues to evolve, the significance of learning programming languages like C++ cannot be overstated. C++ is not only one of the oldest programming languages but also one of the most versatile. Its applications span across various industries, including software development, game development, systems programming, and embedded systems. By mastering C++, learners gain a competitive edge, as it offers a solid foundation for understanding other programming languages.
zyBooks plays a critical role in this learning process by transforming the way students engage with C++. The platform's interactive nature facilitates the mastery of concepts and the development of troubleshooting skills essential in programming.
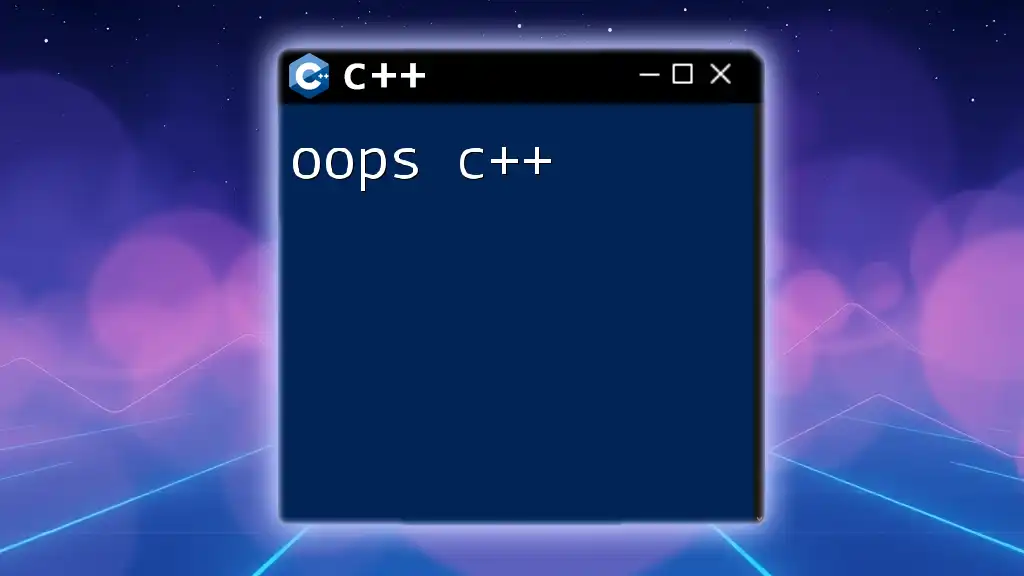
Features of zyBooks C++ Module
Interactive Learning
One of the standout features of zyBooks is its interactive learning approach. Traditional textbooks can often lead to passive learning, where students read but do not actively engage with the content. In contrast, zyBooks incorporates various interactive elements, such as animations and coding exercises, which motivate students to participate actively in their learning.
For example, students can manipulate variables and see real-time updates on results, reinforcing their understanding of how code operates while enhancing their problem-solving skills.
Real-time Feedback
The importance of instant feedback in learning programming cannot be overstated. zyBooks provides real-time feedback, which helps learners identify mistakes and misconceptions immediately, facilitating a faster learning curve. When students are able to see where they went wrong and receive tips on how to improve, it significantly enhances their coding experience.
For instance, if a student incorrectly implements a syntax rule in a coding exercise, zyBooks responds instantly with hints and corrections, allowing learners to adjust their understanding right away.
Comprehensive Content Coverage
The zyBooks C++ module covers a wide range of topics essential for both beginners and advanced programmers. The content is well-structured, starting from the basics and progressively building toward more complex concepts. Key topics include:
- Data Types, Operators, and Input/Output
- Control Structures and Conditional Statements
- Functions, Recursion, and Scope
- Object-Oriented Programming and Inheritance
This comprehensive coverage means that students can rely on zyBooks for all their C++ learning needs, making it a preferred choice for many educational institutions.
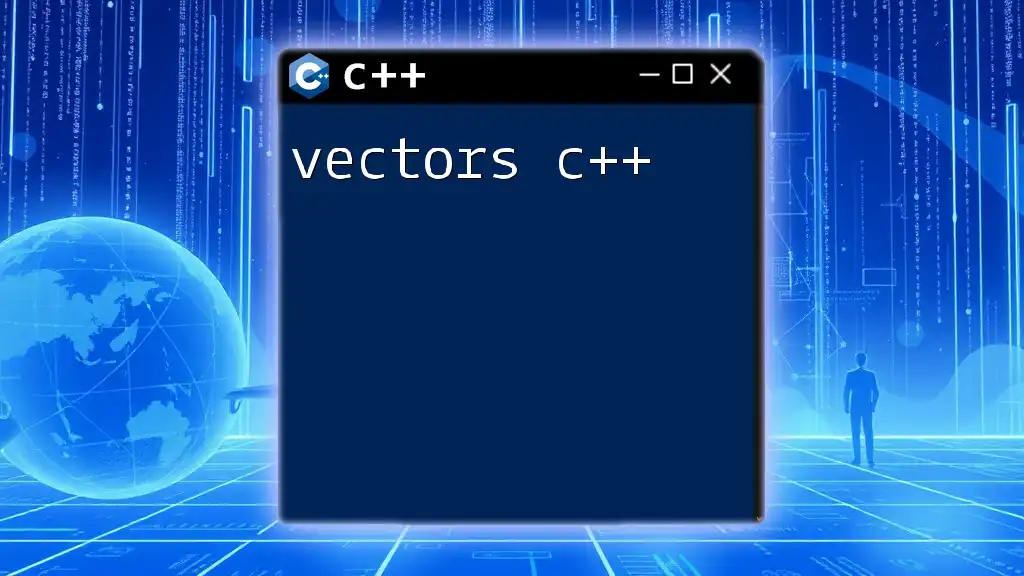
Key Concepts Explored in zyBooks C++
Fundamentals of C++
The zyBooks C++ module begins by introducing students to the core fundamentals of the language. This includes understanding different data types, operators, and how to perform basic input and output operations.
For example, consider the following code snippet demonstrating how to interact with users:
#include <iostream>
using namespace std;
int main() {
int number;
cout << "Enter a number: ";
cin >> number;
cout << "You entered: " << number << endl;
return 0;
}
In this example, students learn about `iostream`, which is essential for performing input and output operations, and practice using `cin` and `cout` for data entry and retrieval.
Control Structures
As students progress, they dive into control structures, which are vital for dictating the flow of a program. zyBooks emphasizes the use of both conditional statements and loops, teaching how to implement decision-making in applications.
For instance, the use of `if`, `else`, and `switch` statements can significantly alter program behavior based on user input. Additionally, looping constructs like `for`, `while`, and `do-while` allow for the execution of repetitive tasks. Here’s an example demonstrating the use of a `for` loop:
#include <iostream>
using namespace std;
int main() {
for (int i = 0; i < 5; i++) {
cout << "Loop iteration: " << i << endl;
}
return 0;
}
This code snippet provides a clear introduction to loops, showcasing how control structures are integrated within programs.
Functions and Scope
Functions are a crucial aspect of C++, allowing for optimized code organization and reusability. In zyBooks, learners explore how to declare, define, and invoke functions effectively. This understanding helps them break down complex problems into manageable, reusable components.
Here's a simple example of a function:
int add(int a, int b) {
return a + b;
}
int main() {
cout << "Sum: " << add(5, 10) << endl;
return 0;
}
In this example, students will learn about parameters, return types, and function scope, enhancing their programming logic and problem-solving capabilities.
Object-Oriented Programming in C++
Object-Oriented Programming (OOP) is one of the fundamental paradigms in C++ that zyBooks emphasizes heavily. Learners are introduced to core OOP concepts, including classes, objects, inheritance, and polymorphism.
For example, consider the following practical illustration of defining a simple class:
class Dog {
public:
void bark() {
cout << "Woof!" << endl;
}
};
int main() {
Dog dog;
dog.bark();
return 0;
}
This code introduces students to the basic structure of a class and demonstrates how methods can be used to encapsulate functionality, providing a basis for more advanced OOP concepts.
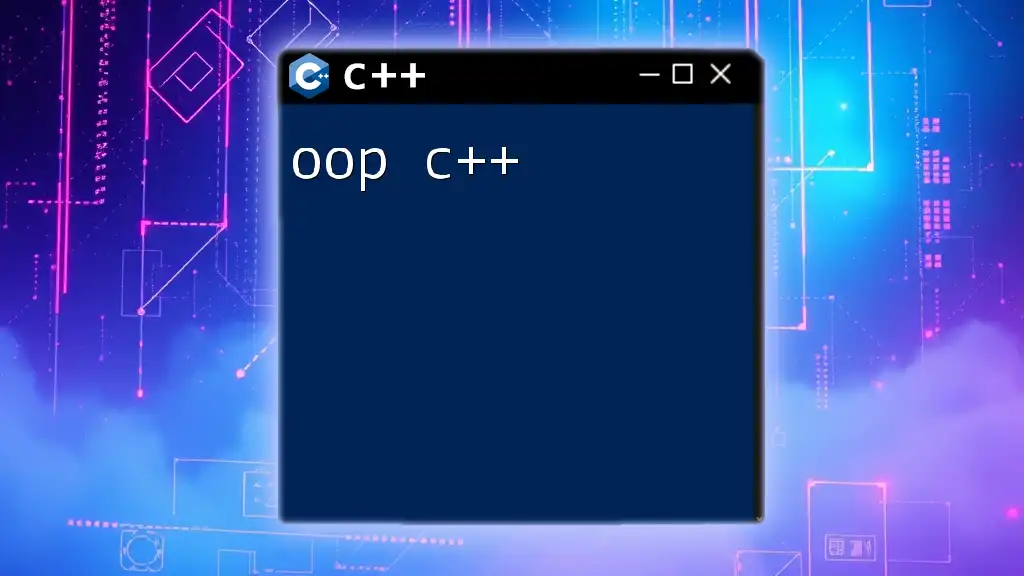
Assessments and Practice in zyBooks C++
Homework and Exercises
zyBooks integrates a rich array of homework and exercises designed to reinforce learning. These assignments encourage learners to apply the concepts they have studied and develop their coding skills. Students are urged to approach homework by breaking down problems into smaller tasks, leveraging zyBooks’ interactive resources to find solutions.
Self-assessment Tools
In addition to homework, zyBooks offers self-assessment tools like quizzes and practice tests. These assessments allow students to gauge their understanding and identify areas where they may need further study. By regularly engaging with self-assessment activities, students can track their progress and solidify their comprehension of key concepts.
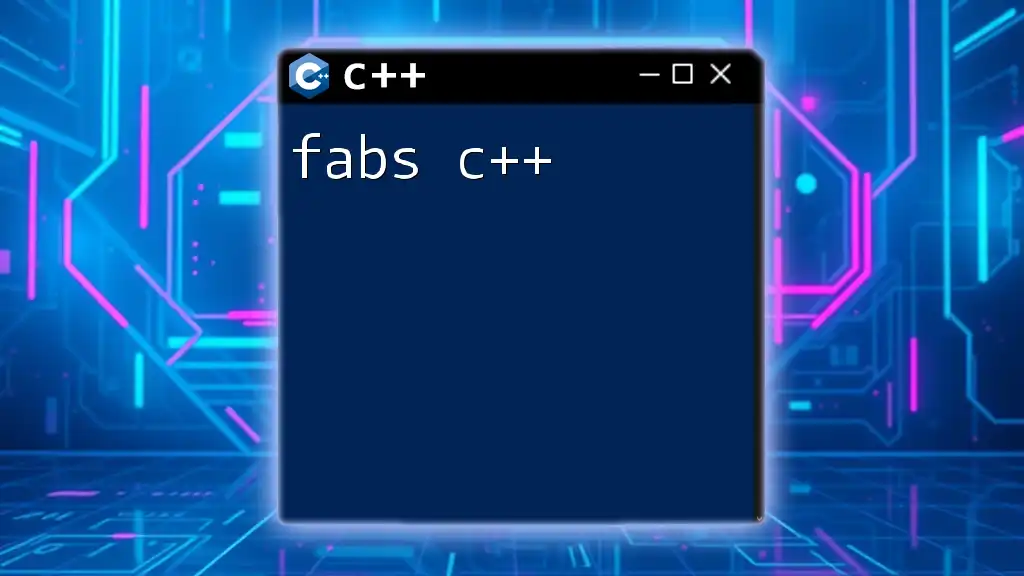
Tips for Success with zyBooks C++
Best Practices for Using zyBooks Efficiently
To maximize their learning experience with zyBooks, students should adopt certain best practices. Practicing effective time management by setting aside dedicated study times for zyBooks learning can lead to better retention. Additionally, setting specific goals for each study session can keep learners focused and motivated.
Leveraging zyBooks Resources
zyBooks offers various resources, including forums and discussion boards, where learners can ask questions and interact with peers. Engaging with fellow students and instructors can provide valuable insights and enhance understanding of complex topics.
Continuous Learning and Improvement
Lastly, students should recognize the importance of continued practice outside of zyBooks. They are encouraged to seek out further resources—such as programming books, coding challenges, and online courses—to enhance their skills and ensure they stay current in the evolving programming landscape.
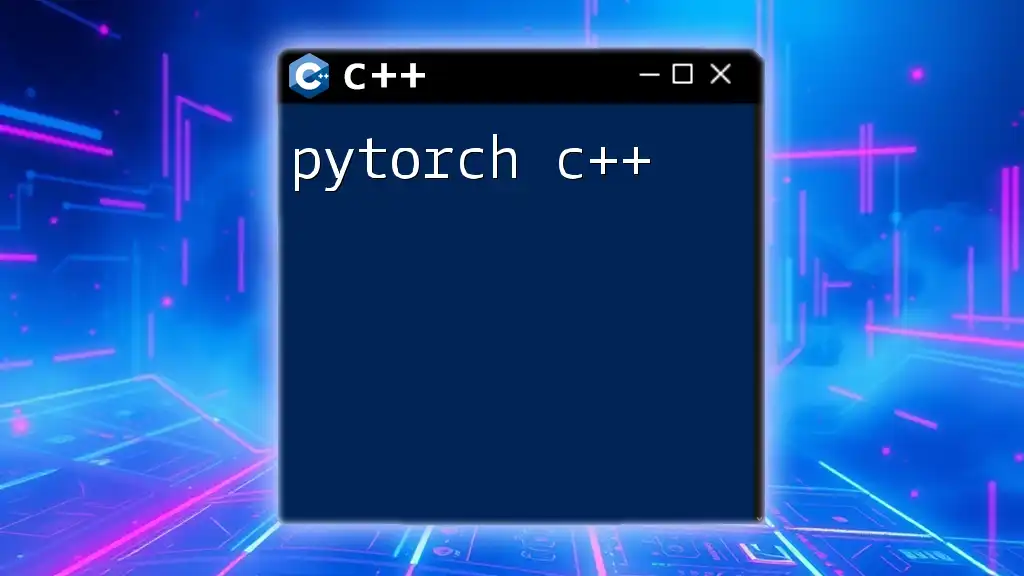
Conclusion
zyBooks C++ presents an engaging and effective platform for mastering programming concepts. Through its interactive learning environment, real-time feedback, and comprehensive content, zyBooks equips learners with the tools they need to become proficient C++ programmers. By embracing the resources and strategies outlined in this guide, students can embark on a successful journey toward coding mastery, ensuring they are well-prepared for their future endeavors in technology.