The `operator bool` in C++ allows a class to provide a conversion operator that enables instances of the class to be used in a boolean context, such as in conditional statements.
Here's a code snippet demonstrating how to implement `operator bool`:
#include <iostream>
class MyBool {
public:
MyBool(bool value) : value_(value) {}
// Conversion operator to bool
operator bool() const {
return value_;
}
private:
bool value_;
};
int main() {
MyBool myTrue(true);
MyBool myFalse(false);
if (myTrue) {
std::cout << "myTrue is true!" << std::endl;
}
if (!myFalse) {
std::cout << "myFalse is false!" << std::endl;
}
return 0;
}
What is `operator bool`?
`operator bool` is a user-defined type conversion operator in C++ that allows an object of a class to be implicitly converted to a boolean value. This operator enables custom objects to behave like built-in boolean types within expressions that expect a boolean result. By implementing `operator bool`, developers can seamlessly integrate their objects into conditional statements and logical operations, enhancing both usability and readability.
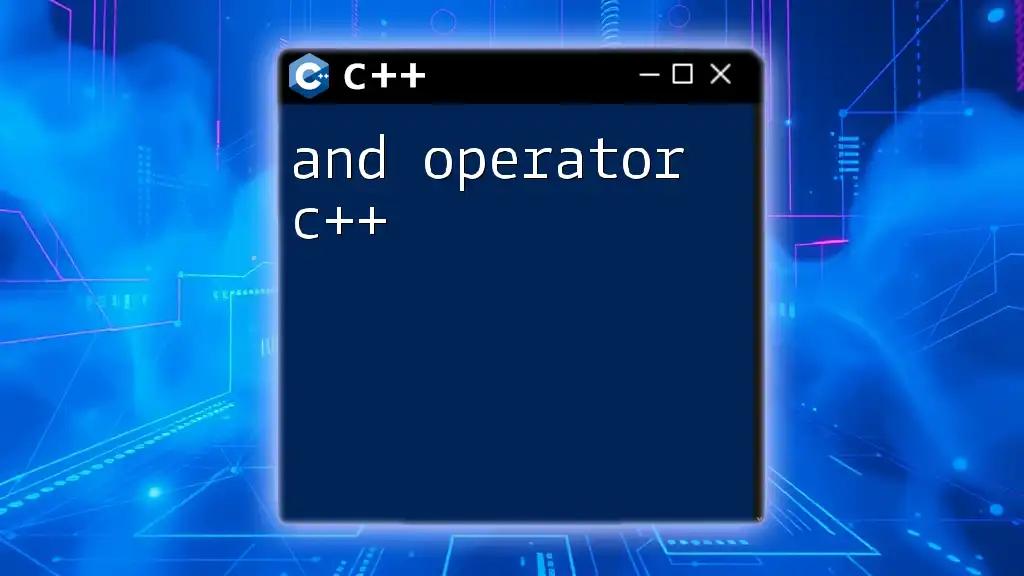
Why Use `operator bool`?
Simplifying Boolean Contexts
In C++, certain contexts demand a boolean value, such as conditionals (`if`, `while`). Implementing `operator bool` simplifies the interaction between objects of a user-defined class and the boolean context, allowing for cleaner and more intuitive code.
Improved Readability
When a custom class automatically converts to a boolean, it reduces the clutter and makes the code more readable. This is especially true in conditional expressions, where the object's intent becomes immediately clear.
Integration with Conditional Statements
With `operator bool`, developers can directly use their objects in conditions without needing additional checks or method calls. For example:
if (myObject) {
// Do something when myObject is true
}
Here, `myObject` can be used directly in the `if` statement because of the custom `operator bool`, improving both clarity and conciseness.
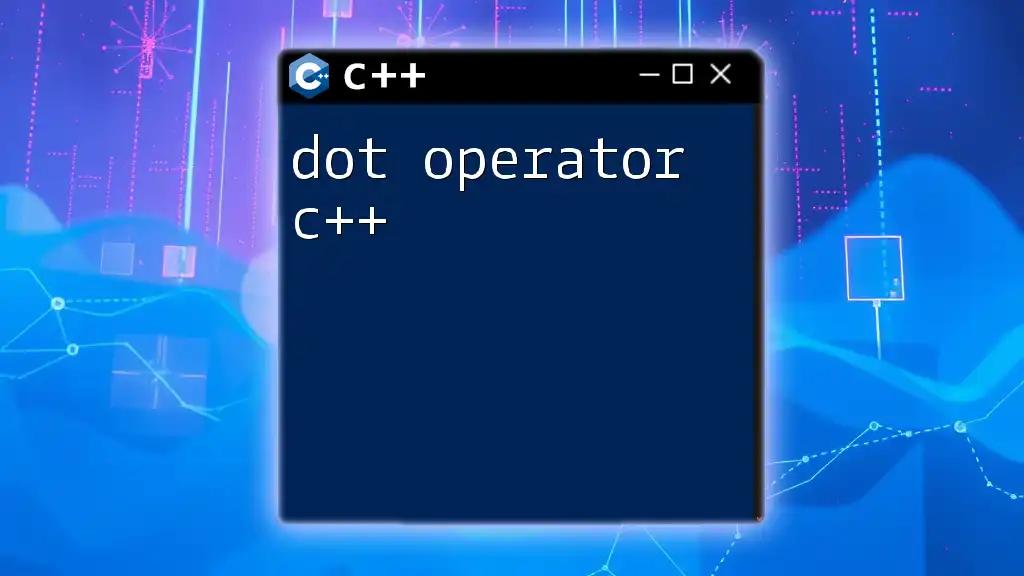
How to Implement `operator bool`
Basic Syntax
The syntax for implementing `operator bool` is straightforward. Here’s a basic template:
class MyBoolean {
public:
operator bool() const {
// Logic to determine true or false
}
};
In this code snippet, the `operator bool()` function is defined inside a class, returning a boolean value based on your custom logic.
Returning a Boolean Value
To effectively utilize `operator bool`, it’s crucial to define the logic that determines when the object should evaluate to `true` or `false`. For instance, consider a toggle switch represented by a class:
class ToggleSwitch {
public:
ToggleSwitch(bool initialState) : state(initialState) {}
operator bool() const {
return state; // Indicates if the switch is on or off
}
private:
bool state; // true for 'on', false for 'off'
};
In this example, the `state` variable represents whether the toggle switch is on or off. By implementing `operator bool`, the switch can be expressed in conditional checks seamlessly.
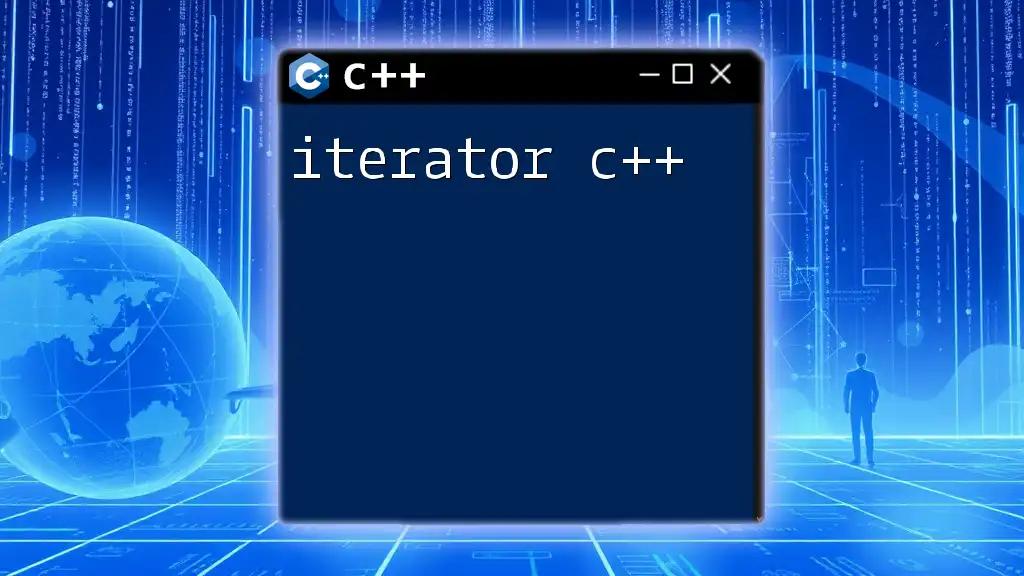
Example Implementations
Simple Example
Here's a simple class that utilizes `operator bool`:
class Light {
public:
Light(bool state) : isOn(state) {}
operator bool() const {
return isOn; // Returns true if isOn is true
}
private:
bool isOn;
};
In this code, `Light` holds a state that is checked during conversions. When you use an instance of `Light` in an if statement, it evaluates whether the light is on.
Practical Implementation
A more practical implementation could involve a database connection. This can illustrate how `operator bool` can signal a successful connection:
class DatabaseConnection {
public:
DatabaseConnection(bool status) : isConnected(status) {}
operator bool() const {
return isConnected; // Check if the connection is active
}
private:
bool isConnected;
};
Whenever you attempt to utilize a `DatabaseConnection` object in conditional logic, it would allow you to check if it is connected without explicitly calling any methods.
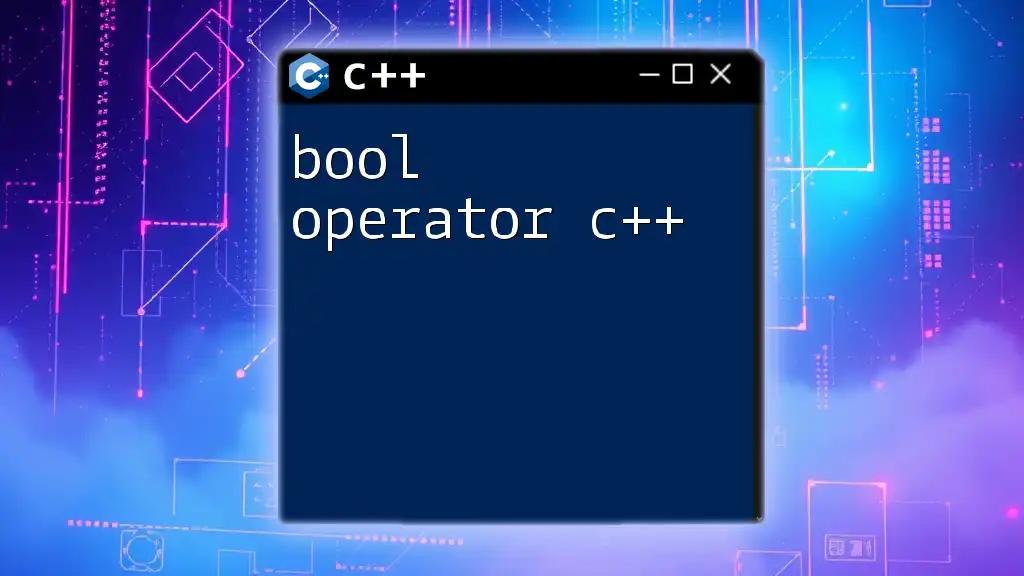
Risks and Considerations
Implicit Conversions
One of the potential risks of `operator bool` is the possibility of unintentional implicit conversions, which may result in unexpected behavior in your code. It is crucial to be aware of when automatic conversions are happening, as this can lead to subtle bugs.
Best Practices
To ensure code clarity and functionality, consider using `explicit` keyword when necessary, preventing unintended conversions. Here’s an example:
class MyClass {
public:
explicit operator bool() const {
// Logic here
}
};
This prevents implicit conversions and enforces clear intent in your code.
Overloading vs. Default Behavior
Understand the implications of overloading this operator. While it provides flexibility, it can also confuse users of your class if overloading isn't intuitive.
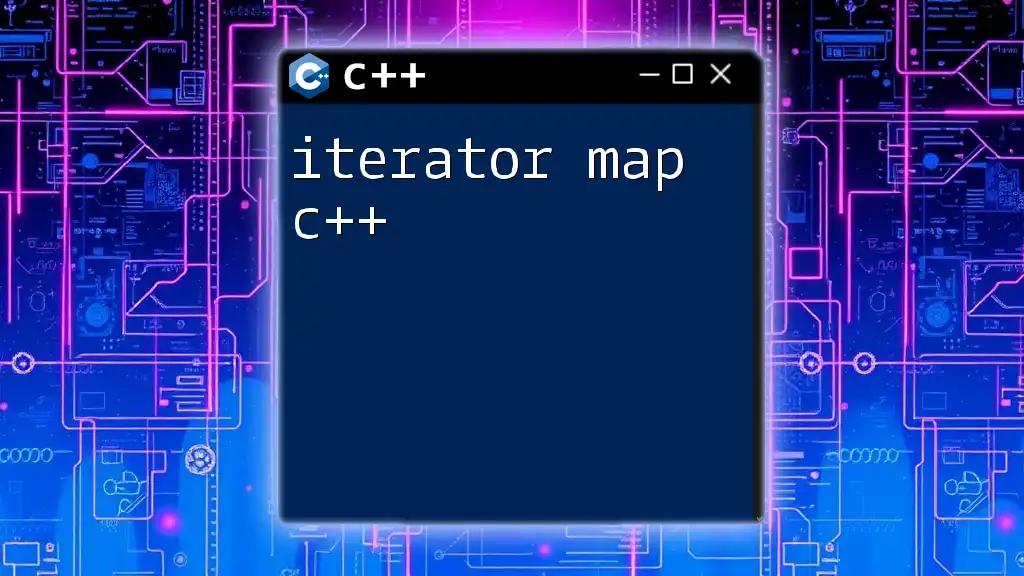
Alternative Approaches
Using a `bool` Getter Function
Although `operator bool` is useful, you might consider providing a separate getter function that returns a boolean value:
bool isOn() const { return isOn; }
This can make your intent clearer to users, as they can invoke the function to check the state rather than relying on implicit conversion.
Custom Boolean-like Classes
In certain situations, it may be beneficial to create classes that mimic boolean behavior without overloading. For instance, if a class represents a stateful object, you can couple it with a method providing accessible state rather than applying `operator bool`.

Common Pitfalls
Overloading Without Care
Carelessly overloading `operator bool` can create confusion in your codebase. If the logic defining true/false behavior isn’t clear, it can lead to difficult debugging scenarios. Always strive for concise, logical conditions that guide the operation's intended behavior.
Debugging `operator bool`
When issues arise with `operator bool`, verify the conditions and logic therein. Ensure that the code faithfully represents the state of the object. Including debug output during development can greatly assist in identifying unexpected behavior.

Conclusion
By understanding and correctly implementing the `operator bool` in C++, you can enhance code readability, simplify conditionals, and create more intuitive interfaces. However, it is essential to consider the risks and best practices associated with its use. As you develop your skills in C++, take the opportunity to explore `operator bool` in your custom classes to harness its power effectively.