The FizzBuzz problem in C++ is a common programming challenge that requires you to print the numbers from 1 to n, replacing multiples of three with "Fizz", multiples of five with "Buzz", and multiples of both with "FizzBuzz".
#include <iostream>
using namespace std;
int main() {
for (int i = 1; i <= 100; i++) {
if (i % 3 == 0 && i % 5 == 0)
cout << "FizzBuzz";
else if (i % 3 == 0)
cout << "Fizz";
else if (i % 5 == 0)
cout << "Buzz";
else
cout << i;
cout << endl;
}
return 0;
}
Understanding the FizzBuzz Logic
What is FizzBuzz?
FizzBuzz is a classic programming challenge that is often used to teach the basic principles of coding and to assess a programmer's understanding of logic and conditions. The main goal is simple: for a given range of numbers, print each number on a new line. However, for multiples of three, you print “Fizz” instead of the number, for multiples of five, you print “Buzz”, and for numbers that are multiples of both three and five, you print “FizzBuzz”.
Why Use C++ for FizzBuzz?
Using C++ to implement FizzBuzz is an excellent choice for several reasons. C++ is a powerful programming language that combines efficiency with abstraction. Mastering even simple exercises like FizzBuzz can enhance your understanding of basic control structures, conditionals, and loop mechanics in C++. It also serves as a solid foundation for further exploration into more complex algorithms.
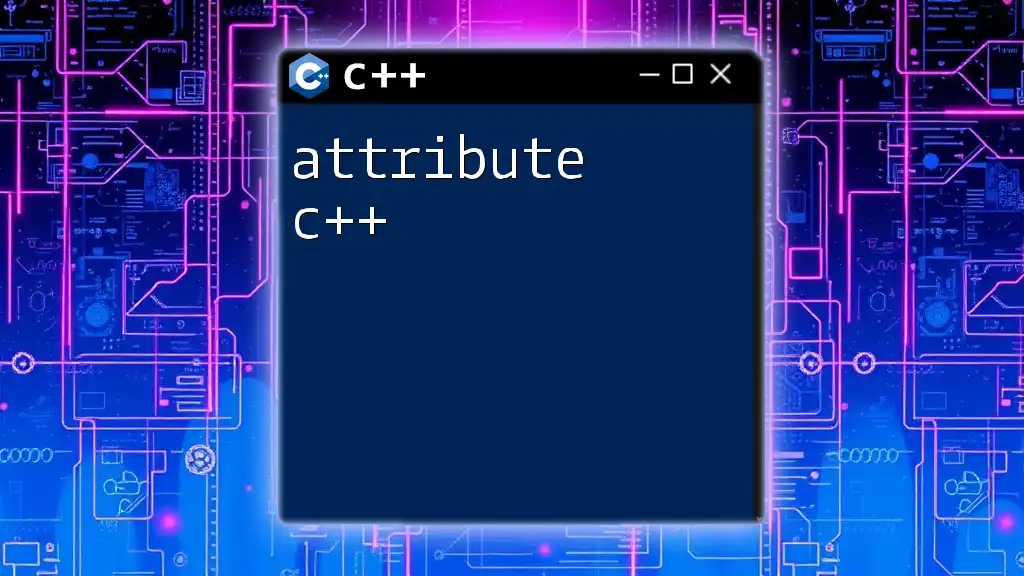
Setting Up Your C++ Environment
Installing a C++ Compiler
To get started, you’ll need a C++ compiler. Some popular choices include:
- g++ (GNU C++ Compiler): A common open-source compiler available on various platforms.
- Clang: A modern, fast compiler that is suitable for C++ development.
The setup process is straightforward, and tutorials can be easily found online for your specific operating system.
Using an IDE for C++
To simplify the coding experience, using an Integrated Development Environment (IDE) can be beneficial. Some popular IDEs include:
- Code::Blocks: Lightweight and user-friendly.
- Visual Studio: Feature-rich and widely used for professional development.
An IDE provides helpful features such as syntax highlighting, code suggestion, and debugging tools, which can significantly enhance your coding productivity.
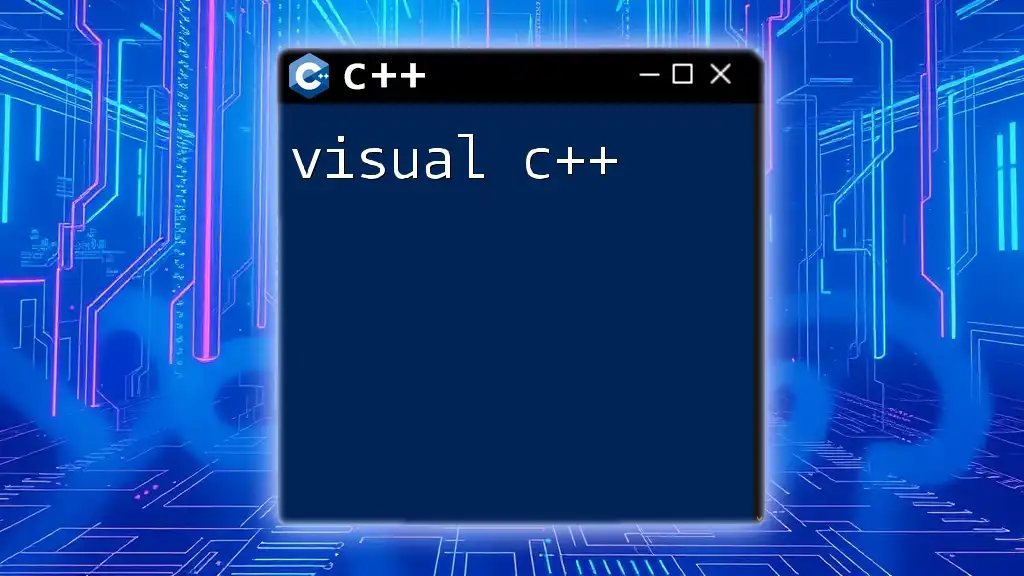
Writing Your First FizzBuzz Program in C++
Basic C++ Program Structure
Before coding FizzBuzz, it's essential to understand the structure of a simple C++ program. Every program begins with including necessary libraries and defining the main function. A typical structure might look like this:
#include <iostream>
int main() {
// Your code will go here.
return 0;
}
Implementing the FizzBuzz Algorithm
Now, let's put the logic into action with a basic implementation of FizzBuzz in C++. The following code snippet demonstrates a simple solution:
#include <iostream>
int main() {
for (int i = 1; i <= 100; i++) {
if (i % 3 == 0 && i % 5 == 0) {
std::cout << "FizzBuzz" << std::endl;
} else if (i % 3 == 0) {
std::cout << "Fizz" << std::endl;
} else if (i % 5 == 0) {
std::cout << "Buzz" << std::endl;
} else {
std::cout << i << std::endl;
}
}
return 0;
}
Explanation of Code:
- Header File: The line `#include <iostream>` is necessary for using input-output streams in C++.
- Main Function: The execution starts from the `main()` function.
- Loop: The `for` loop iterates from 1 to 100 (or any range you specify).
- Conditional Statements: Inside the loop, `if`, `else if`, and `else` statements evaluate whether the current number is divisible by 3, 5, or both, and accordingly print “Fizz”, “Buzz”, or “FizzBuzz”.
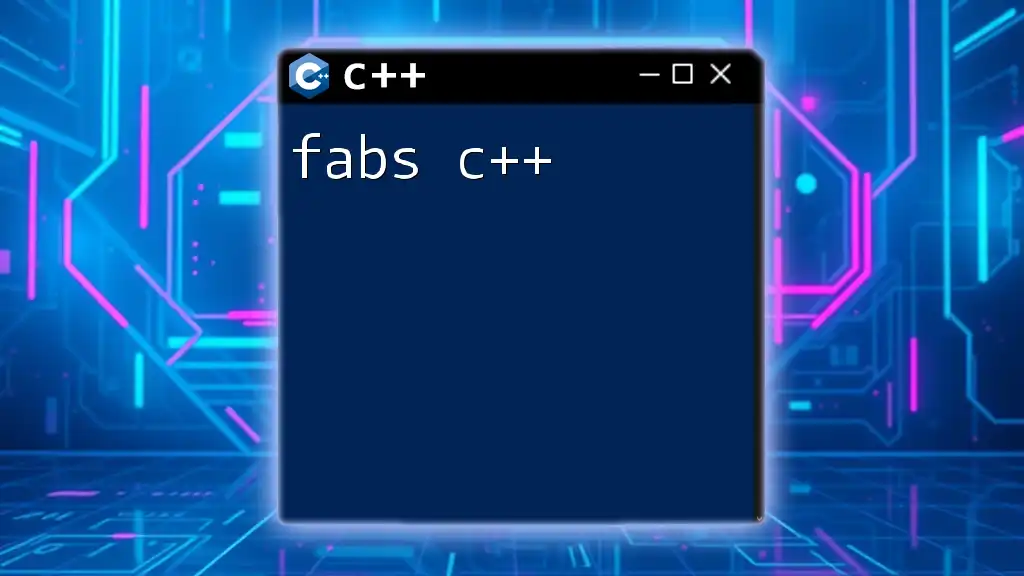
Exploring Variations of FizzBuzz
Advanced Variations
Mastering the basic FizzBuzz program opens the door to various enhancements and customizations. You can modify the conditions to include other numbers or add different outputs. For example, if you wanted to add a rule for multiples of 7, your logic might expand further.
Enhancing User Input
One interesting variation of FizzBuzz could involve allowing the user to set the range of numbers. This interactive element enhances user engagement and showcases your ability to handle input in C++. Here’s an example of how you might implement this:
#include <iostream>
int main() {
int range;
std::cout << "Enter the range: ";
std::cin >> range;
for (int i = 1; i <= range; i++) {
if (i % 3 == 0 && i % 5 == 0) {
std::cout << "FizzBuzz" << std::endl;
} else if (i % 3 == 0) {
std::cout << "Fizz" << std::endl;
} else if (i % 5 == 0) {
std::cout << "Buzz" << std::endl;
} else {
std::cout << i << std::endl;
}
}
return 0;
}
This program introduces the concept of user input and demonstrates how to capture it using `std::cin`. It allows for more flexible interaction with the program.
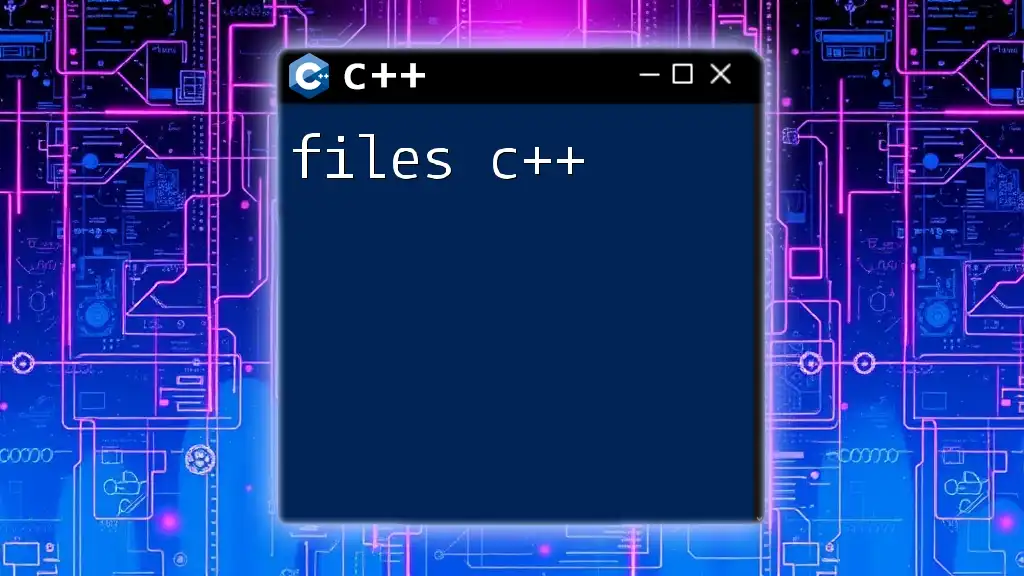
Conclusion
Successfully implementing FizzBuzz in C++ is a vital exercise for beginners looking to strengthen their programming skills. This exercise not only helps you grasp the concepts of loops and conditionals but also sets the stage for more complex programming challenges. As you explore further, consider diving into other algorithms and data structures that can deepen your understanding of the C++ language.
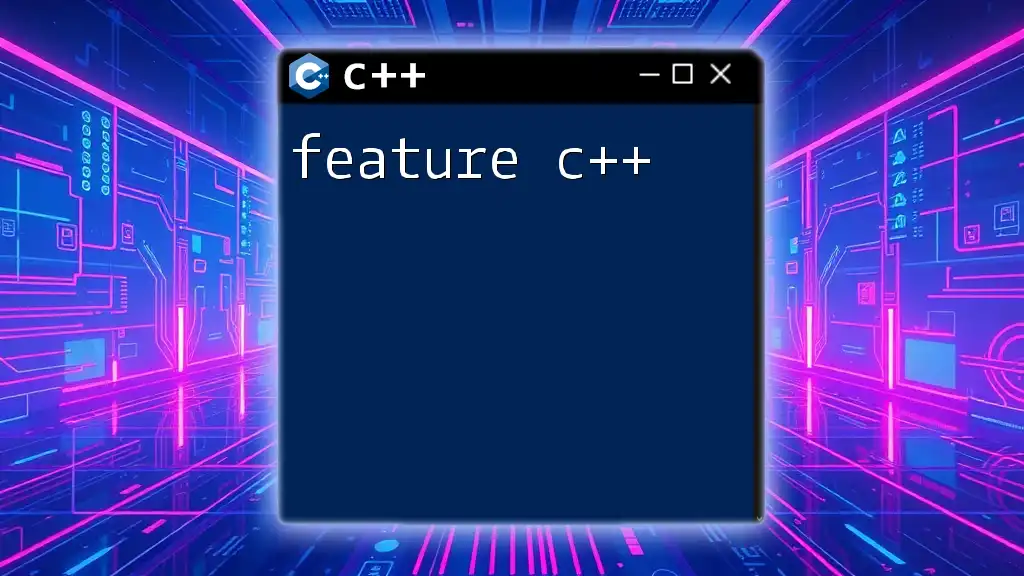
FAQs about FizzBuzz in C++
You may encounter various questions while learning FizzBuzz, such as:
-
What is the best way to practice FizzBuzz?
Create variations or use it as a warm-up exercise before tackling more challenging problems. -
Why is FizzBuzz commonly used in interviews?
It's a straightforward way to evaluate a candidate's problem-solving skills and basic programming knowledge.
By familiarizing yourself with these concepts, you'll be better prepared to tackle FizzBuzz and other programming tasks in C++.