Object-Oriented Programming (OOP) concepts in C++ primarily include encapsulation, inheritance, polymorphism, and abstraction, which help structure code and promote reusability. Here's a simple code snippet demonstrating these concepts:
#include <iostream>
using namespace std;
// Base class
class Animal {
public:
virtual void speak() { cout << "Animal speaks"; }
};
// Derived class
class Dog : public Animal {
public:
void speak() override { cout << "Woof"; } // Polymorphism
};
int main() {
Animal* myDog = new Dog(); // Inheritance
myDog->speak(); // Calls the Dog's speak method
delete myDog; // Clean up
return 0;
}
What is Object-Oriented Programming?
Object-Oriented Programming (OOP) is a programming paradigm that utilizes "objects" to design software. These objects can encapsulate data and behaviors, mirroring real-world entities and their interactions. OOP emphasizes the use of objects to represent both data and functionality, streamlining program structure and enhancing code reusability.
Historically, programming has evolved from procedural paradigms, which focused on sequential execution of instructions, to object-oriented paradigms. While procedural programming organizes logic into functions—often leading to complex and unmanageable code as projects grow—OOP introduces a more modular approach that promotes code organization through abstraction and encapsulation.
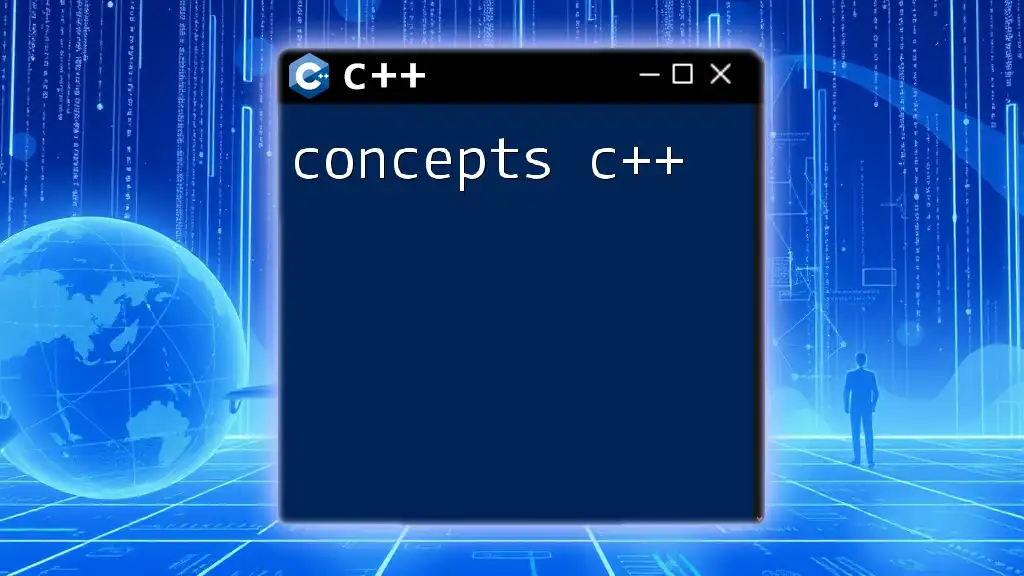
Core OOP Concepts in C++
Encapsulation
Encapsulation is the principle of bundling the data (attributes) and methods (functions) that manipulate the data into a single unit, known as a class. This not only protects the internal state of an object from unintended interference but also simplifies the interface through which the object can be used.
Access modifiers play a crucial role in encapsulation. In C++, the main access modifiers are:
- Public: Members are accessible from outside the class.
- Private: Members are accessible only within the class.
- Protected: Members are accessible within the class and by derived classes.
For example, consider the following C++ class representing a bank account:
class Account {
private:
int balance;
public:
void deposit(int amount) {
balance += amount;
}
int getBalance() {
return balance;
}
};
In this code, the `balance` variable is protected from direct access, ensuring that it can only be modified through the `deposit` method. This provides a clear and safe interface for interacting with the data.
Benefits of Encapsulation include:
- Data protection and integrity: Only authorized methods can alter the data.
- Simplified interface: Users interact with a clear set of methods without concerning themselves with the implementation details.
Inheritance
Inheritance is a powerful feature allowing classes to inherit attributes and methods from other classes, promoting code reusability and establishing a hierarchical relationship between classes.
Base classes serve as the foundation, while derived classes extend or override the functionality of the base class. This reusability simplifies code maintenance and fosters a clear structure in programming.
Here's an example to illustrate inheritance:
class Animal {
public:
void eat() {
cout << "Eating" << endl;
}
};
class Dog : public Animal {
public:
void bark() {
cout << "Barking" << endl;
}
};
In this code, `Dog` inherits from `Animal`, allowing it to use the `eat` method. This promotes reusability, as any other animal class can inherit from `Animal` without needing to rewrite the `eat` method.
Benefits of Inheritance include:
- Reusability of code: Reduces redundancy by enabling classes to share behavior.
- Establishing hierarchical relationships: Enhances code clarity by grouping related classes.
Polymorphism
Polymorphism allows objects of different classes to be treated as objects of a common base class, providing flexibility in method invocation. This is a crucial concept in object-oriented programming, as it enables a single interface to represent different types.
Polymorphism can be classified into two main types:
-
Compile-time polymorphism: Achieved through method overloading, where multiple methods share the same name but differ in parameters.
-
Runtime polymorphism: Achieved through method overriding, where a derived class provides a specific implementation of a method already defined in its base class.
Here's an example of compile-time polymorphism through method overloading:
class Math {
public:
int add(int a, int b) {
return a + b;
}
double add(double a, double b) {
return a + b;
}
};
In this example, the `add` method functions differently based on the type of the input parameters.
Runtime polymorphism can be illustrated with the following code:
class Shape {
public:
virtual void draw() {
cout << "Drawing Shape" << endl;
}
};
class Circle : public Shape {
public:
void draw() override {
cout << "Drawing Circle" << endl;
}
};
Here, the `draw` method is defined in both `Shape` and `Circle`. At runtime, the appropriate method is called based on the object type, enabling flexibility and reducing coupling.
Benefits of Polymorphism are significant:
- Flexibility in code implementation: It simplifies the addition of new classes without altering existing code.
- Simplification of code maintenance: Changes in one part of the code do not disproportionately impact others.
Abstraction
Abstraction in programming is about simplifying complex systems by exposing only the necessary or relevant features while hiding unnecessary details. This provides a clear interface for the user and allows them to interact with the system without needing to understand its complexities.
In C++, abstraction is implemented through abstract classes and interfaces. An abstract class contains at least one pure virtual function (a method with no implementation). This ensures that any derived class must provide an implementation for these functions.
Consider the following example:
class AbstractShape {
public:
virtual void draw() = 0; // Pure virtual function
};
class Rectangle : public AbstractShape {
public:
void draw() override {
cout << "Drawing Rectangle" << endl;
}
};
In this code, `AbstractShape` defines a common interface for shapes, which requires derived classes like `Rectangle` to implement the `draw` method.
Benefits of Abstraction include:
- Improved code readability and usability: Users can focus on high-level functionality without getting bogged down by details.
- Focus on what an object does rather than how it does it: This streamlines development and reduces potential errors.
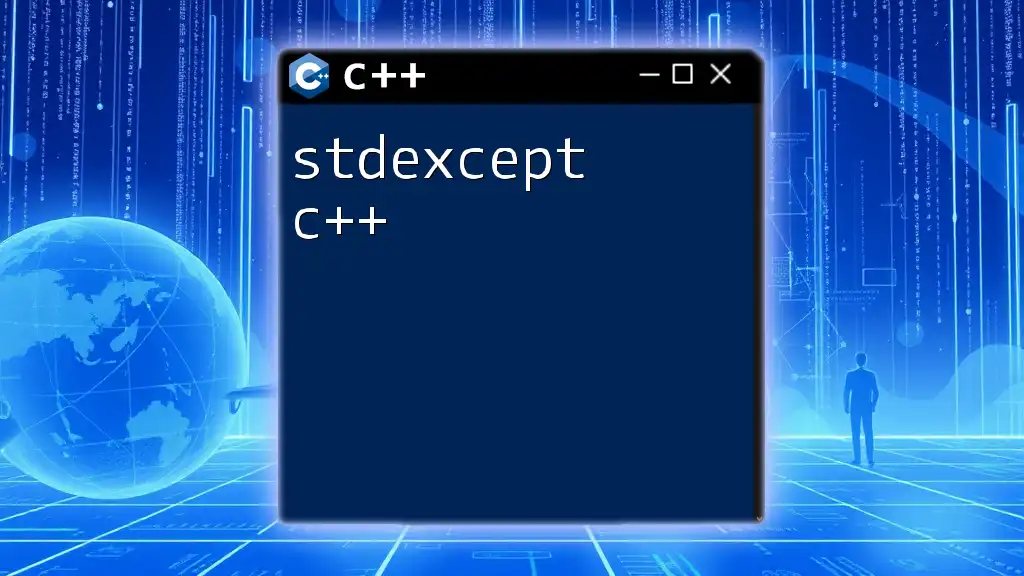
Summary of OOP Concepts in C++
In summary, OOP concepts in C++—encapsulation, inheritance, polymorphism, and abstraction—serve as the foundation for designing robust, scalable, and manageable software architecture. These concepts work synergistically, allowing developers to emulate real-world scenarios and build applications that are easier to understand and maintain.
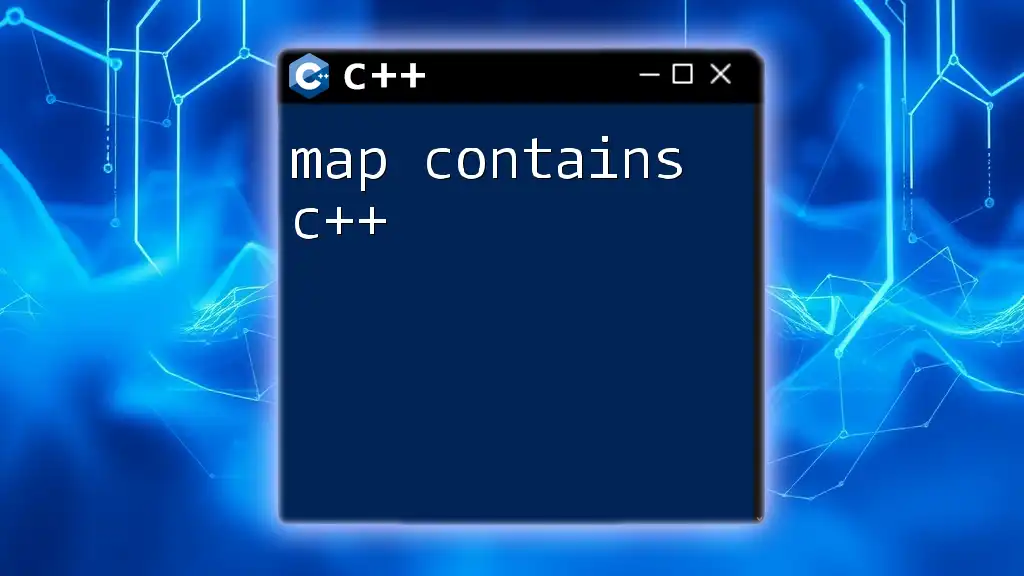
Best Practices for Applying OOP in C++
To effectively implement OOP concepts in C++, consider the following best practices:
- Use meaningful class names that reflect their functionality.
- Keep classes focused: Ensure that each class has a single responsibility.
- Favor composition over inheritance to promote loose coupling and flexibility.
- Utilize interfaces to define clear contracts and expectations for classes.
Furthermore, strive to avoid common pitfalls, such as excessive inheritance leading to a fragile class hierarchy or overusing public access modifiers, which can compromise encapsulation.
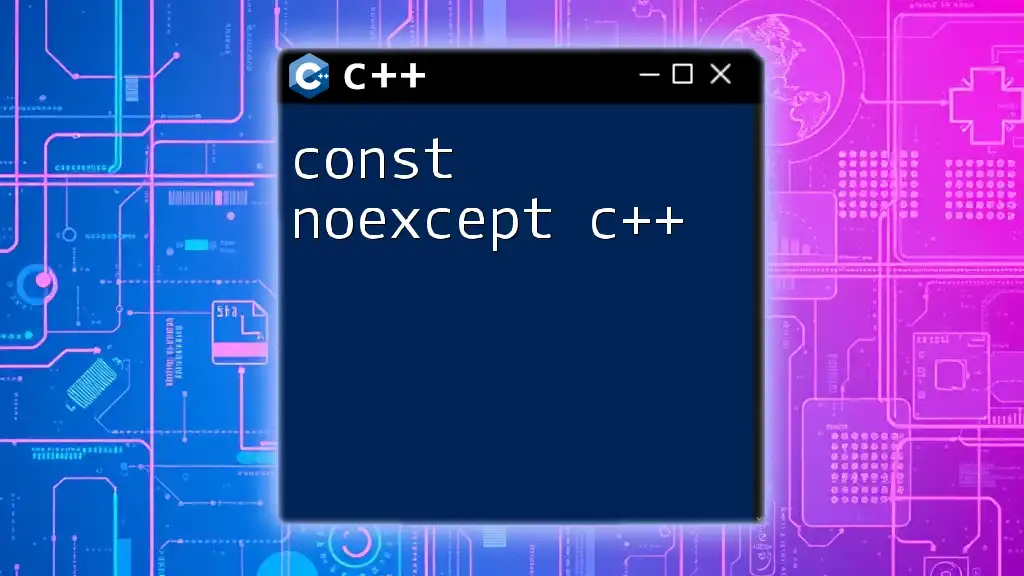
Conclusion
Mastering OOP concepts in C++ is essential for anyone looking to develop efficient, maintainable, and scalable software. Understanding these principles opens up opportunities to leverage the power of C++ and engage in more complex projects. Practice implementing these concepts in your own projects, and experience how they simplify complex problems in software development.
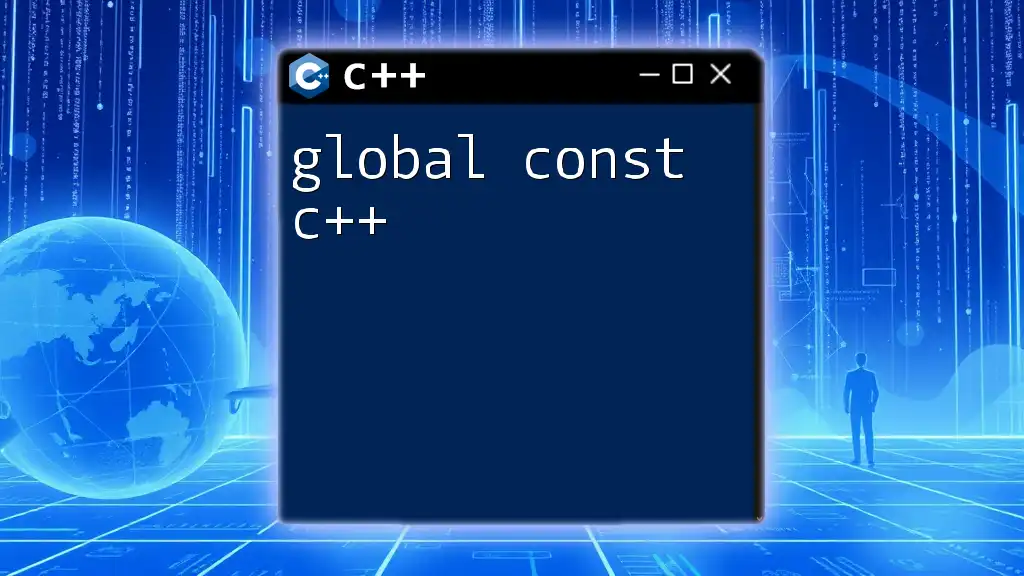
Additional Resources
To further your understanding and expertise, consider exploring recommended books, online courses, and communities focused on C++ and OOP principles. Engaging with these resources will deepen your knowledge and skills.
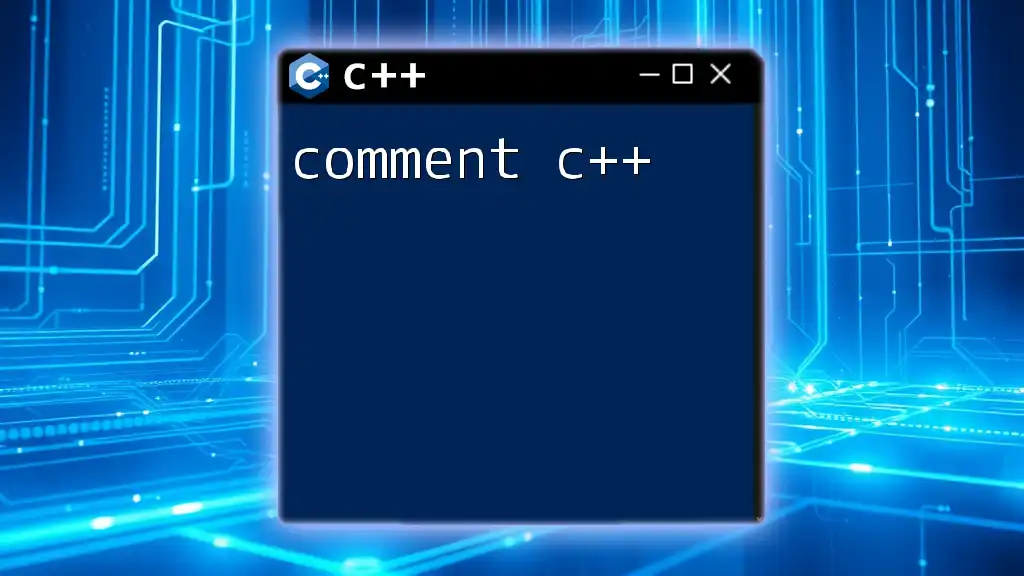
Call to Action
If you're eager to dive deeper into the world of C++ and learn more about OOP concepts, connect with our company for comprehensive training and hands-on experience! Expand your understanding of how to utilize these principles effectively in your programming journey.