OpenCV is a powerful library in C++ that simplifies computer vision tasks, allowing developers to easily process images and videos with just a few lines of code.
Here’s a simple example of loading and displaying an image using OpenCV in C++:
#include <opencv2/opencv.hpp>
int main() {
cv::Mat image = cv::imread("path_to_image.jpg");
if (image.empty()) {
std::cout << "Could not open or find the image." << std::endl;
return -1;
}
cv::imshow("Display window", image);
cv::waitKey(0);
return 0;
}
Introduction to OpenCV C++
OpenCV, or Open Source Computer Vision Library, is a powerful toolkit that significantly simplifies the tasks involved in the field of computer vision. Originally developed for the Intel Integrator, it has now become a go-to resource for developers, researchers, and enthusiasts wanting to work with real-time image processing. OpenCV offers a comprehensive suite of features and functions that enable quick and efficient handling of image and video data.
Getting Started with OpenCV in C++
To begin your journey with OpenCV C++, it’s crucial to ensure that you have the right setup. Familiarity with C++ is a prerequisite, as OpenCV primarily utilizes this programming language. Below are the key steps to get you started:
-
Installation: You can install OpenCV by downloading a pre-built version from the official OpenCV website or by building it from source. The latter allows for more customization based on your system's requirements.
-
Setting Up Your IDE: Popular IDEs for C++ development such as Visual Studio, Code::Blocks, or CLion can easily integrate OpenCV. Be sure to link the OpenCV libraries correctly in your project settings to ensure seamless access to its functionalities.

Basics of Image Processing
Understanding Images in OpenCV
Images in OpenCV are represented using the Mat class, which is a powerful data structure designed to store images in various formats. Once your OpenCV development environment is set up, you can easily read an image file with the following code:
cv::Mat image = cv::imread("path/to/image.jpg");
This command reads an image from the specified file path and stores it in a Mat object. To see the image displayed, utilize the following:
cv::imshow("Display window", image);
cv::waitKey(0);
This code opens a window depicting the image you just loaded, allowing interaction until any key is pressed.

Core Functions in OpenCV
Basic Image Operations
Working with images usually involves various operations. Here are some fundamental functions provided by OpenCV:
-
Resizing Images: Adjusting the dimensions of an image can be done effortlessly. For instance:
cv::resize(image, resized_image, cv::Size(new_width, new_height));
This snippet will produce a resized version of the original image according to the specified new width and height.
-
Cropping Images: Cropping helps in isolating significant portions of images for further processing or analysis. Use the following template:
cv::Rect region_of_interest(x, y, width, height); cv::Mat cropped_image = image(region_of_interest);
Here, you define a rectangular region specified by its top-left corner (x, y) and its dimensions.
-
Color Space Conversions: OpenCV facilitates seamless conversion between different color spaces. For example, converting an image from BGR (default format for OpenCV) to Grayscale can be achieved with:
cv::Mat gray_image; cv::cvtColor(image, gray_image, cv::COLOR_BGR2GRAY);
This command generates a grayscale representation, helping in reducing complexity for further image processing tasks.
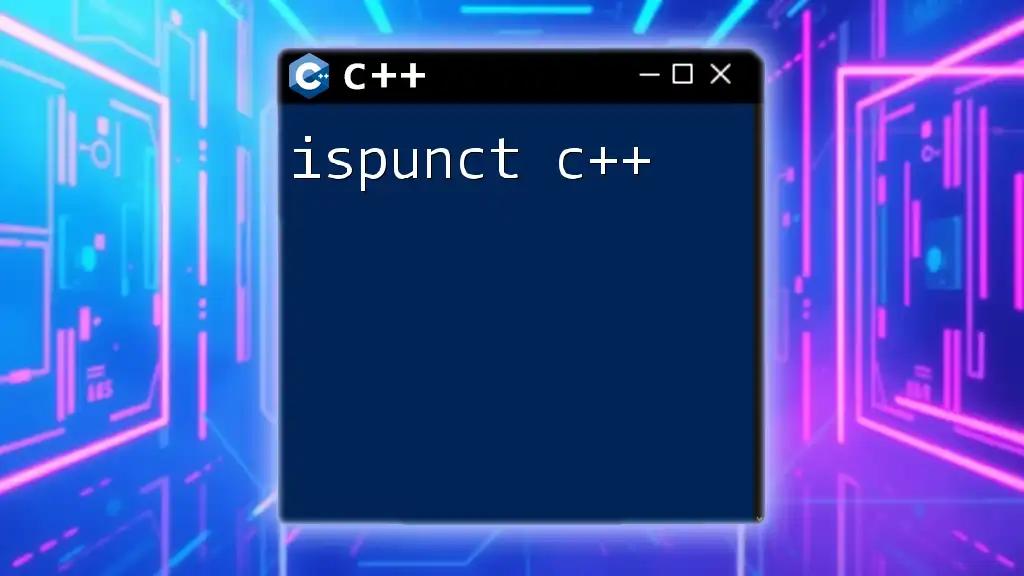
Advanced Image Processing Techniques
Filtering and Smoothing
Filter applications play a crucial role in improving the quality of images by reducing noise and blurriness.
-
Gaussian Blur is commonly employed for this purpose:
cv::GaussianBlur(image, blurred_image, cv::Size(5, 5), 0);
-
Median Filtering and Bilateral Filtering are other techniques presented by OpenCV to preserve edges and reduce noise.
Edge Detection
Detecting edges is fundamental to identifying objects within images. The Canny Edge Detection method is one of the most effective algorithms available, and can be implemented as follows:
cv::Canny(image, edges, threshold1, threshold2);
Choosing appropriate thresholds is key to achieving optimal results, as they dictate which edges are defined as significant.

Working with Videos
Reading and Writing Videos
OpenCV simplifies video processing by providing robust functions for both reading and writing video files.
To open a video, use:
cv::VideoCapture cap("path/to/video.mp4");
This line initializes the video capture object, enabling the user to process each frame accordingly. When finished, you can write video frames using:
cv::VideoWriter writer("output_video.avi", cv::VideoWriter::fourcc('M','J','P','G'), fps, frame_size);
Ensure to replace `fps` and `frame_size` with appropriate values.
Processing Video Frames
Processing video frames involves handling each frame individually. You can achieve this with a loop that continuously reads frames and applies necessary image processing techniques. This kind of iterative analysis can add dynamic capabilities to your applications.
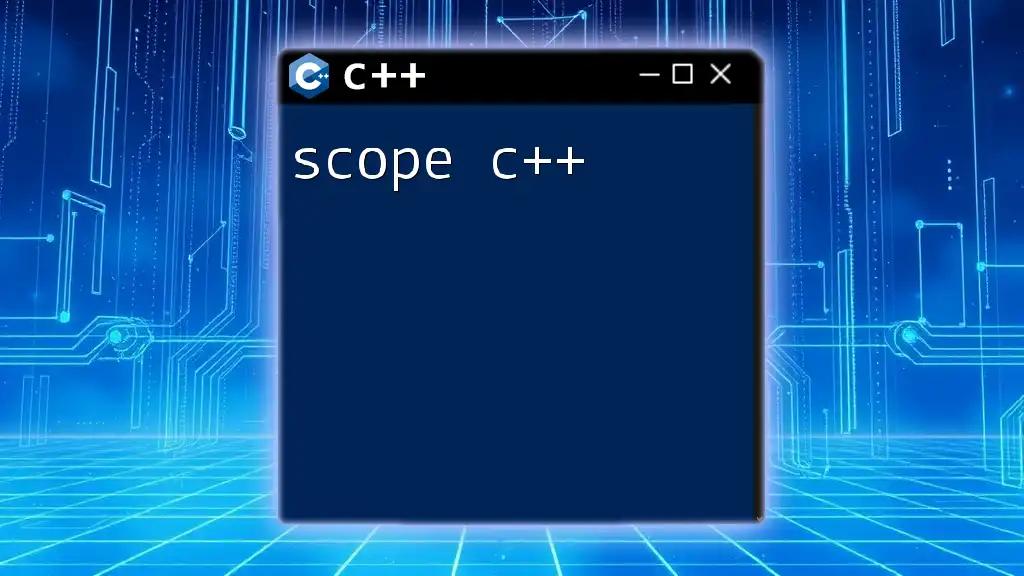
Object Detection and Recognition
Introduction to Object Detection
OpenCV provides substantial support for detecting various objects, making it a versatile tool in numerous applications, from security systems to augmented reality.
Using Pre-trained Models
Integrating Haar Cascades is a popular method for face detection. You can utilize the following code snippet to initialize and use the classifier:
cv::CascadeClassifier face_cascade("path/to/haarcascade_frontalface_default.xml");
This classifier can detect faces within images, and when combined with the video processing techniques mentioned above, real-time face detection can be achieved.
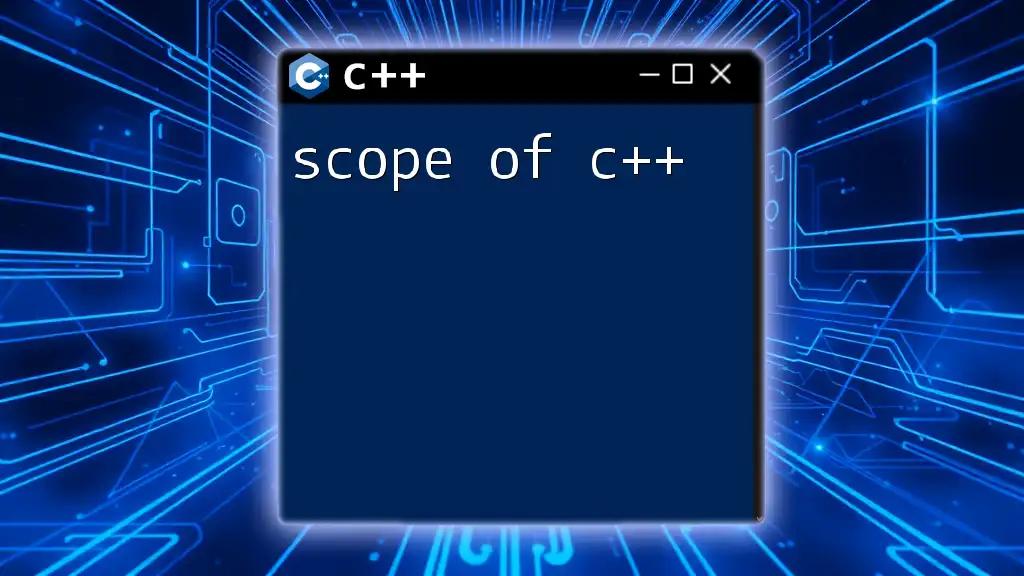
Machine Learning in OpenCV
Overview of Machine Learning in OpenCV
OpenCV has integrated machine learning capabilities to solve various image recognition challenges. It provides various algorithms that can be employed for classification and clustering tasks.
Using k-Nearest Neighbors (k-NN) for Classification
One of the simplest algorithms provided by OpenCV is k-NN. To utilize it efficiently, ensure that your dataset is correctly prepared and model parameters are configured.
// Sample code for k-NN
This code snippet aids in building a simple k-NN classifier based on the pre-defined parameters and data.
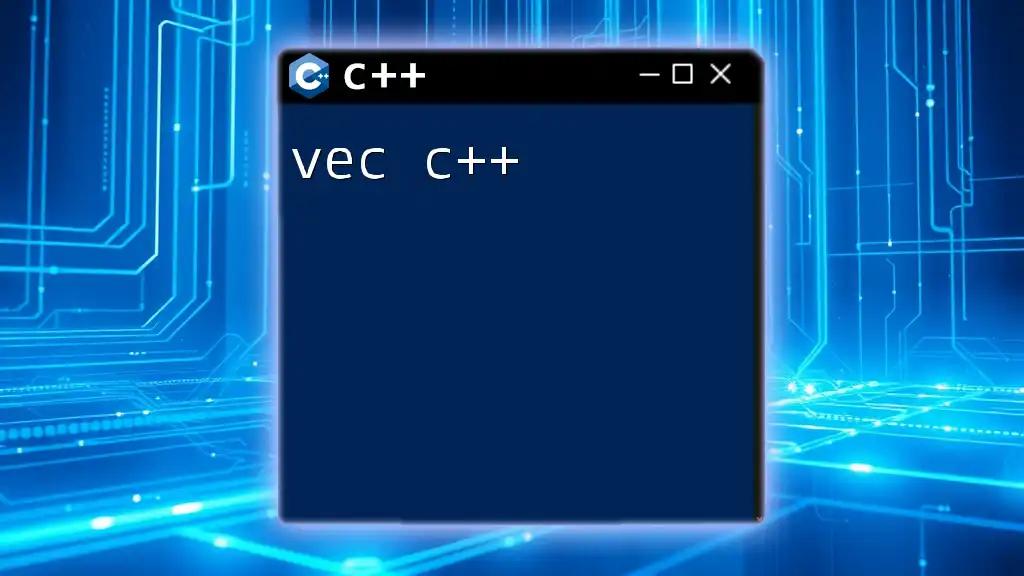
Best Practices and Tips
When developing with OpenCV C++, it’s important to follow best practices for optimal performance. Ensure that you:
- Optimize your code for memory management, especially when working with large images or video files.
- Avoid common pitfalls such as accessing uninitialized variables or failing to release resources.
- Regularly consult the OpenCV documentation for updates in methods and functionalities.
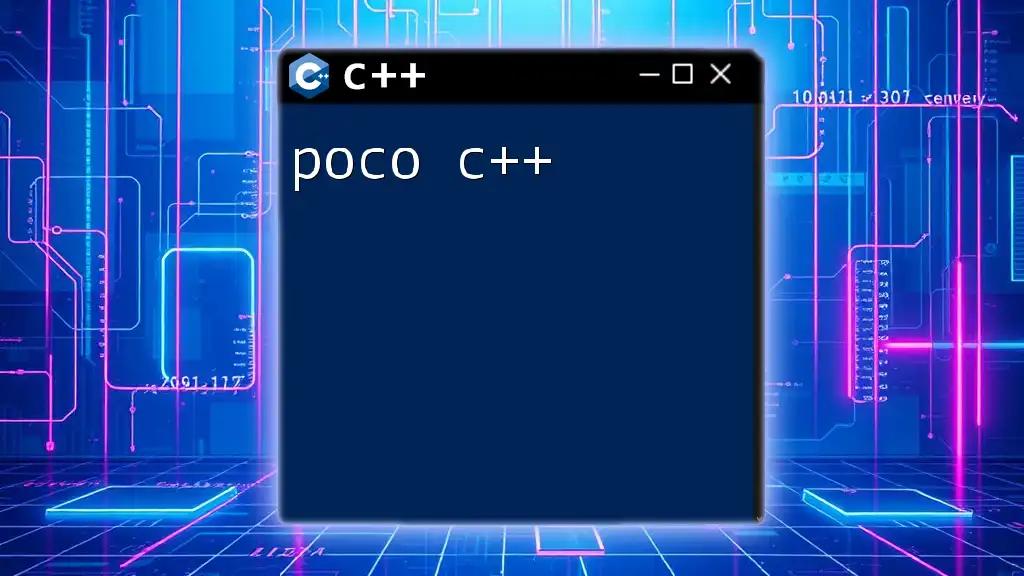
Conclusion
By understanding these fundamental concepts and utilizing the rich functionalities of OpenCV C++, you can elevate your projects to new heights in the realm of image and video processing. Exploring further into the library will empower you to experiment and develop innovative solutions in computer vision, ensuring a promising trajectory in your programming journey.
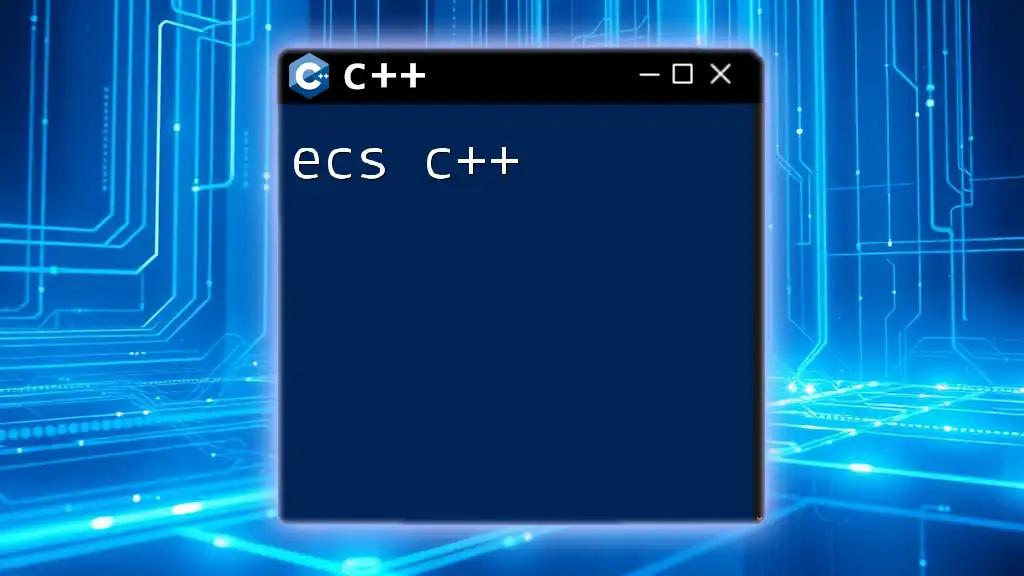
Additional Resources
To deepen your knowledge, dive into the OpenCV [official documentation](https://docs.opencv.org/) for comprehensive guides on various functionalities, explore recommended books and tutorials, and engage with community forums to enhance your learning experience.