The `execvp` function in C++ is used to execute a file, replacing the current process with a new process specified by the file name and arguments, and is part of the exec family of functions in POSIX-compliant systems.
Here’s a simple code snippet demonstrating the use of `execvp`:
#include <iostream>
#include <unistd.h>
int main() {
char *args[] = {"ls", "-l", nullptr}; // Command and arguments
execvp(args[0], args); // Replace the current process with the new one
perror("execvp failed"); // If execvp returns, an error occurred
return 1;
}
Understanding `execvp`
Definition and Purpose
The `execvp` function is an essential part of the Unix/Linux process management toolkit. It belongs to a family of functions that replace the current process image with a new process image. Executing `execvp` enables a program to run another program altogether, utilizing the specified file and arguments. Unlike some other functions, `execvp` does not create a new process; rather, it replaces the existing one with the new one. This makes it a powerful command for scripting and automation scenarios in C++.
Syntax of `execvp`
The syntax for using `execvp` in C++ is straightforward:
int execvp(const char *file, char *const argv[]);
- `file`: This parameter takes in the name of the executable that you wish to run. It can either be an absolute path or a relative path.
- `argv`: This is a pointer to an array of character pointers that represent the argument list. The first element of this array is generally the name of the command being executed, followed by any additional arguments needed for that command.
Parameters Explained
- The `file` parameter essentially serves as the command you want to execute.
- The `argv` array needs to be terminated with a `nullptr`, signaling the end of the arguments.
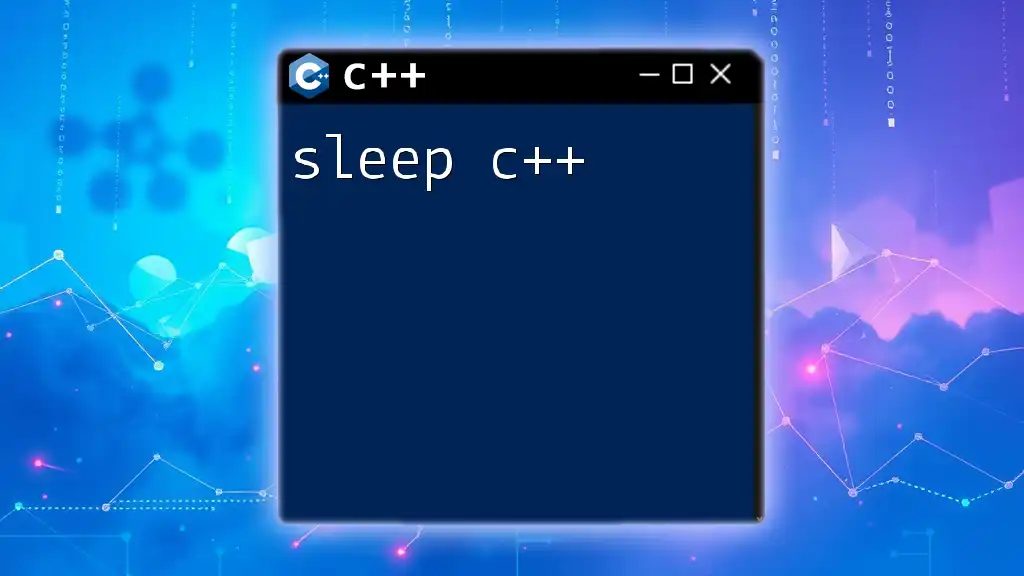
How `execvp` Works
Process Replacement
When a program calls `execvp`, it effectively replaces its own binary code with that of the new command specified by `file`. This results in the current process being terminated (unless `execvp` fails), and a new process being initiated. The new executable starts with the same process ID, which can be crucial for resource management within an application.
Environment and Path Searching
One notable feature of `execvp` is its ability to search for the executable file in the directories specified by the `PATH` environment variable. If the `file` is not a full path, `execvp` will look through each directory listed in `PATH` until it finds the executable to run. This behavior allows for running commands without needing the user to specify the full path every time.
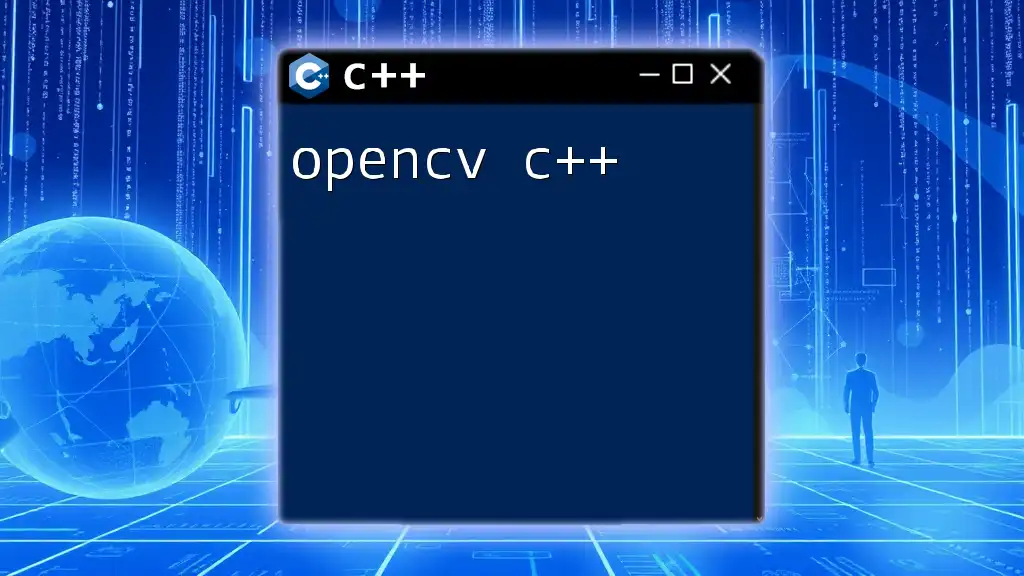
Error Handling in `execvp`
Common Error Scenarios
Errors can occur for several reasons when utilizing `execvp`. Some common scenarios include:
- Executable not found: If the specified `file` does not exist in the searched directories or is incorrectly named, the command will fail.
- Incorrect argument structure: The `argv` array must be correctly formatted to ensure the command receives the appropriate input.
Using `errno`
In case of an error, the system sets the `errno` variable to reflect the specific issue. Understanding and handling `errno` is essential for effective debugging and user notifications. Common values you might encounter in relation to `execvp` include:
- `ENOENT`: The specified file or command could not be found.
- `EACCES`: The file found exists but is not executable.
Example of Error Handling
#include <iostream>
#include <unistd.h>
#include <cstring>
int main() {
const char *file = "non_existent_program";
char *const argv[] = {const_cast<char *>(file), nullptr};
if (execvp(file, argv) == -1) {
std::cerr << "Error: " << strerror(errno) << std::endl;
}
return 0;
}
In the example above, if `execvp` fails, an error message will be displayed, providing insights about what went wrong.
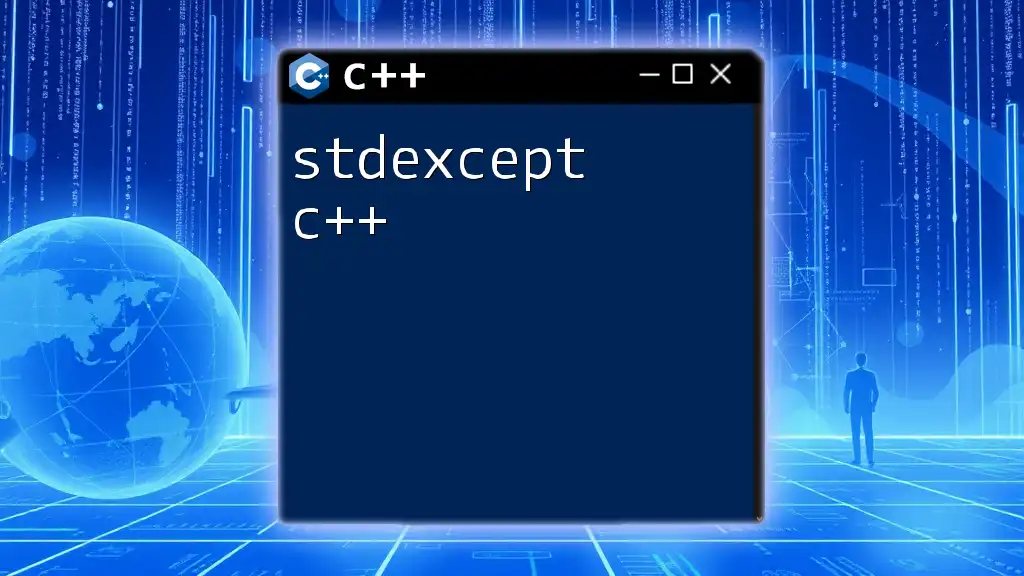
Practical Examples of Using `execvp`
Basic Example with `ls`
One of the simplest ways to demonstrate `execvp` is by running a common system command like `ls`. This command lists the contents of the directory.
#include <iostream>
#include <unistd.h>
int main() {
const char *file = "ls";
char *const argv[] = {const_cast<char *>(file), "-l", nullptr};
execvp(file, argv);
return 0; // This will not execute if execvp is successful
}
In this code snippet, the `ls -l` command is executed, and if it is successful, the process is replaced, meaning any code that follows would not execute.
Example with Custom Executable
To showcase `execvp` further, consider an example where a user-defined executable is called. Here's how you can implement this:
#include <iostream>
#include <unistd.h>
int main() {
const char *file = "./my_program"; // Assume my_program is compiled and exists
char *const argv[] = {const_cast<char *>(file), "arg1", "arg2", nullptr};
execvp(file, argv);
std::cerr << "Failed to execute the command." << std::endl; // Error handling
return 0;
}
This example will execute `my_program`, passing `arg1` and `arg2` as arguments. If `execvp` fails for any reason, the error message will guide developers toward resolving the issue.
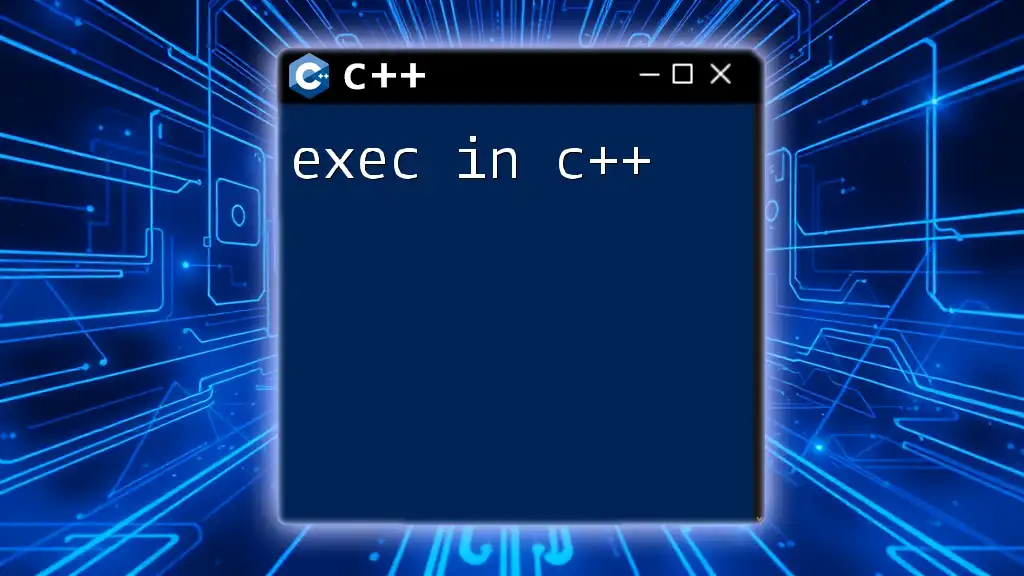
Use Cases for `execvp`
Scripting and Automation
The usage of `execvp` extends beyond simple examples. Its ability to run processes efficiently makes it invaluable in scripting and automation tasks. Whether managing system processes or automating repetitive command-line actions, `execvp` streamlines execution, enabling more robust scripts.
Building Shells
Another significant use case for `execvp` involves building command-line interfaces. Custom shells often utilize `execvp` to execute user-input commands. This empowers developers to create a fully functional shell environment that can interpret and run various commands, greatly enhancing the command-line experience.
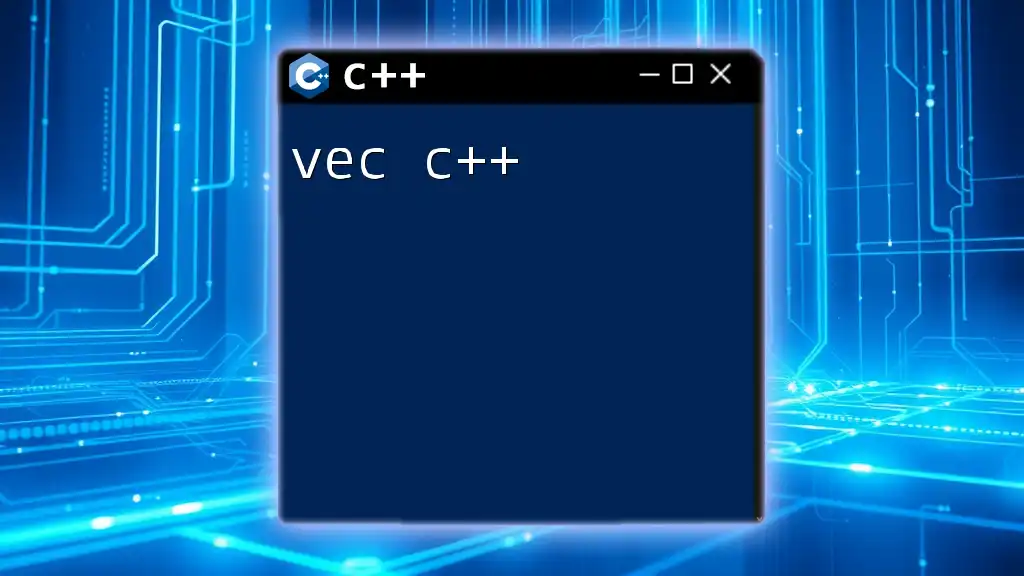
Conclusion
In summary, `execvp` is a powerful function in C++ that allows for the execution of commands and scripts by replacing the current process image with a new one. Understanding its syntax, parameters, and error handling is crucial for any developer working in systems programming. Whether for simple tasks or more complex applications, mastering `execvp` opens the door to efficient process management and execution in Unix/Linux environments.
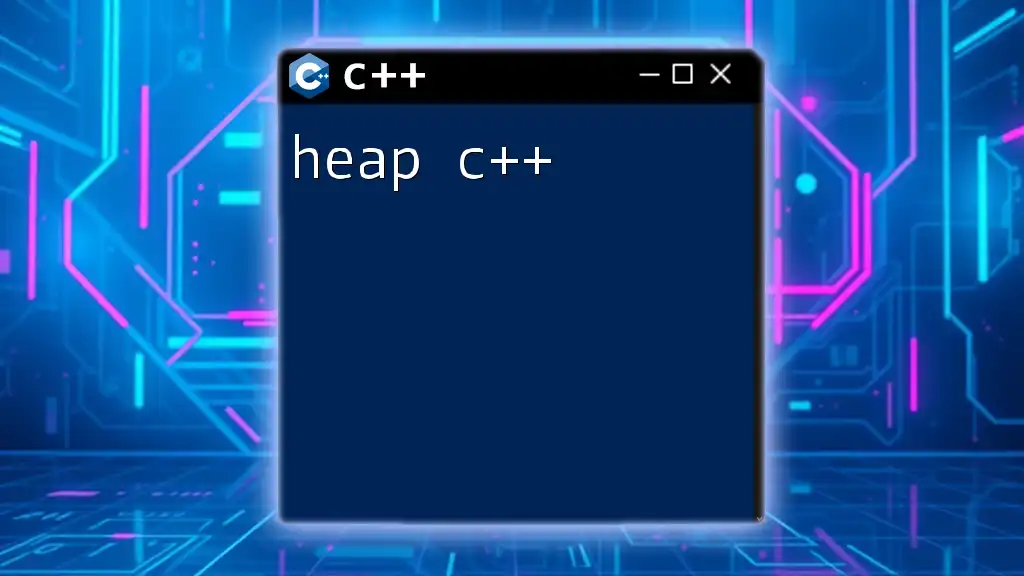
Additional Resources
For those keen on diving deeper into the topic, consider consulting:
- Official documentation for `execvp`
- Recommended books on Unix/Linux system programming
- Online tutorials focusing on process management in C++
Exploring these resources will solidify your understanding and proficiency with this essential function.