The "scope" in C++ refers to the region within a program where a variable or function is accessible and can be used, determined by where it is defined.
Here’s an example illustrating local and global scope:
#include <iostream>
int globalVar = 10; // Global scope
void display() {
int localVar = 5; // Local scope
std::cout << "Local variable: " << localVar << std::endl;
std::cout << "Global variable: " << globalVar << std::endl;
}
int main() {
display();
// std::cout << localVar; // This will cause an error because localVar is out of scope
return 0;
}
What is Scope?
In programming, scope refers to the area in a program where a particular variable is accessible. Understanding scope is crucial for writing effective and manageable code. In C++, scope determines the visibility and lifetime of variables, and it plays a significant role in preventing naming conflicts and unintended variable access.
Types of Scope
C++ defines several types of scope that impact how variables can be accessed. The main types of scope include:
- Local Scope: Variables declared within a function or a block are local to that function/block.
- Global Scope: Variables declared outside of any function can be accessed from any part of the program.
- Namespace Scope: Namespaces allow for the organization of code and prevention of naming collisions.
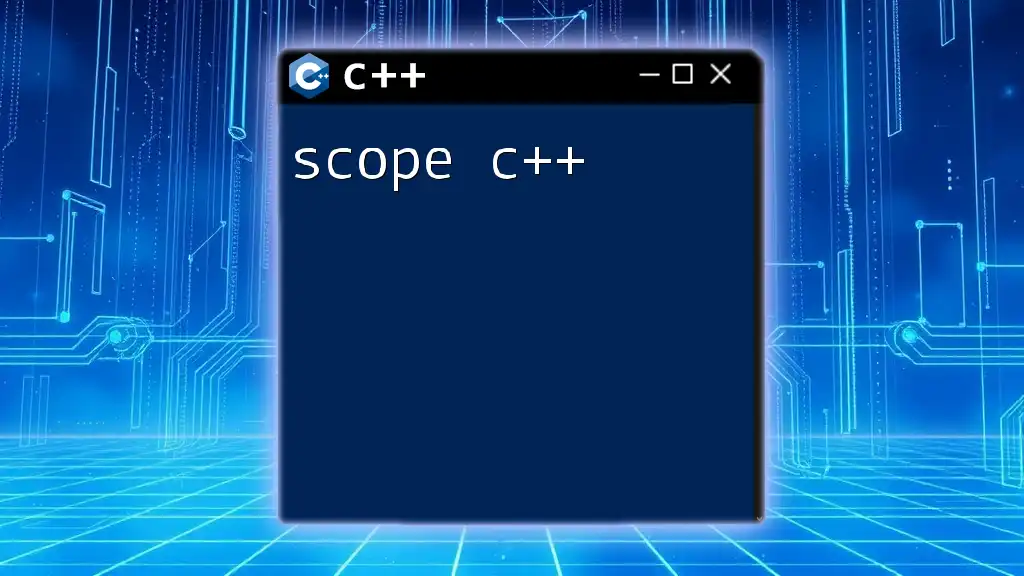
Local Scope
Understanding Local Variables
Local variables are defined within a function or a specific block of code. Their lifetime is limited to the duration of that function/block, meaning once the function exits, the local variables are destroyed. For example:
void localScopeExample() {
int x = 10; // Local variable
cout << x; // Accessible here
}
// cout << x; // Error: 'x' is not accessible here
In this snippet, `x` can only be accessed within `localScopeExample()`. Attempting to access it outside results in an error, showcasing the essence of local scope.
Block Scope
C++ utilizes block scope to limit variable access even further. A block is defined by curly braces `{}` in constructs like if statements and loops. For instance:
if (true) {
int y = 20; // Block-scoped variable
cout << y; // Accessible here
}
// cout << y; // Error: 'y' is not accessible here
Here, `y` is confined to the block defined by the if statement, emphasizing how block scope functions independently.
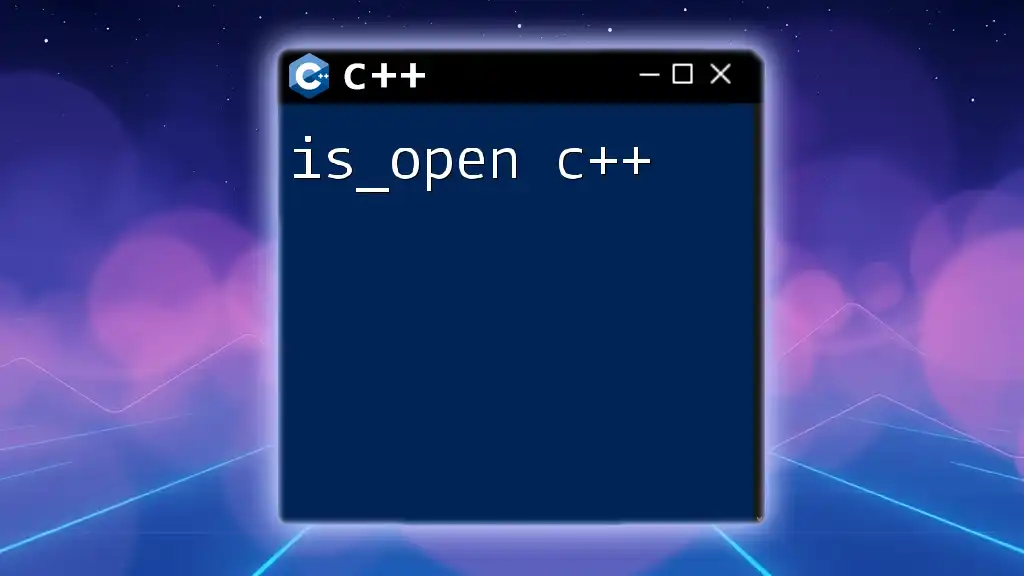
Global Scope
Understanding Global Variables
Variables defined outside any function are considered global variables. They are accessible from any part of the code after their declaration, making them a powerful yet dangerous tool. For example:
int z = 30; // Global variable
void globalScopeExample() {
cout << z; // Accessible here
}
In this case, `z` is accessible both within `globalScopeExample` and any function that follows its declaration.
Pros and Cons of Using Global Variables
While global variables can simplify access across multiple functions, they come with significant drawbacks. Benefits include easy accessibility, but drawbacks consist of the potential for unintended side effects if modified by various functions, often leading to maintenance nightmares. Therefore, caution should be exercised when employing global variables.
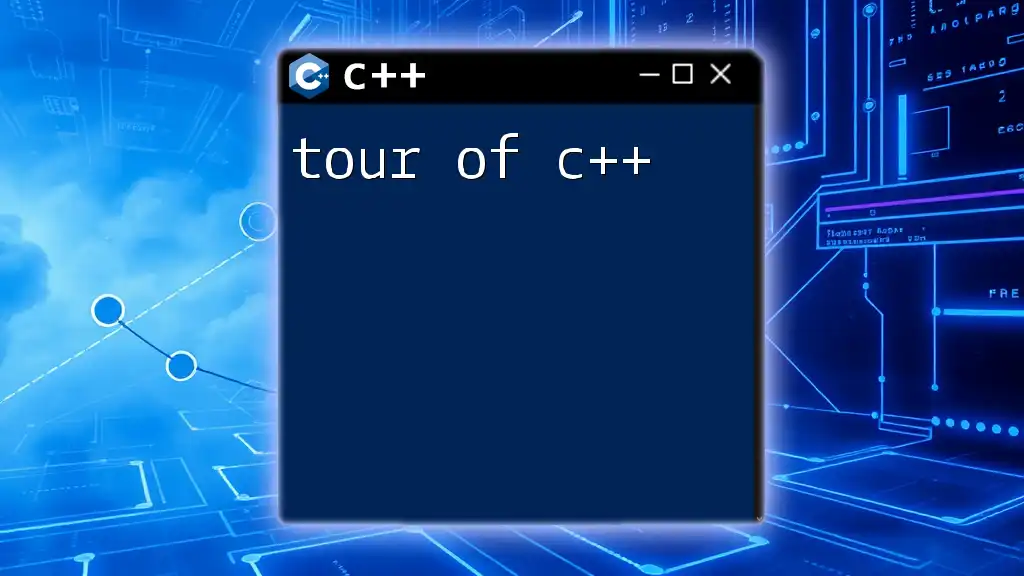
Namespace Scope
What are Namespaces?
Namespaces are a feature in C++ that help organize code and prevent naming conflicts. By grouping related identifiers, you can avoid the risk of having multiple items with the same name. For instance:
namespace MyNamespace {
int var = 40;
}
void namespaceScopeExample() {
cout << MyNamespace::var; // Accessing variable from the namespace
}
Here, `var` is encapsulated within `MyNamespace`. To access it, you use the `MyNamespace::` prefix, demonstrating how namespaces help maintain clarity and prevent conflict.
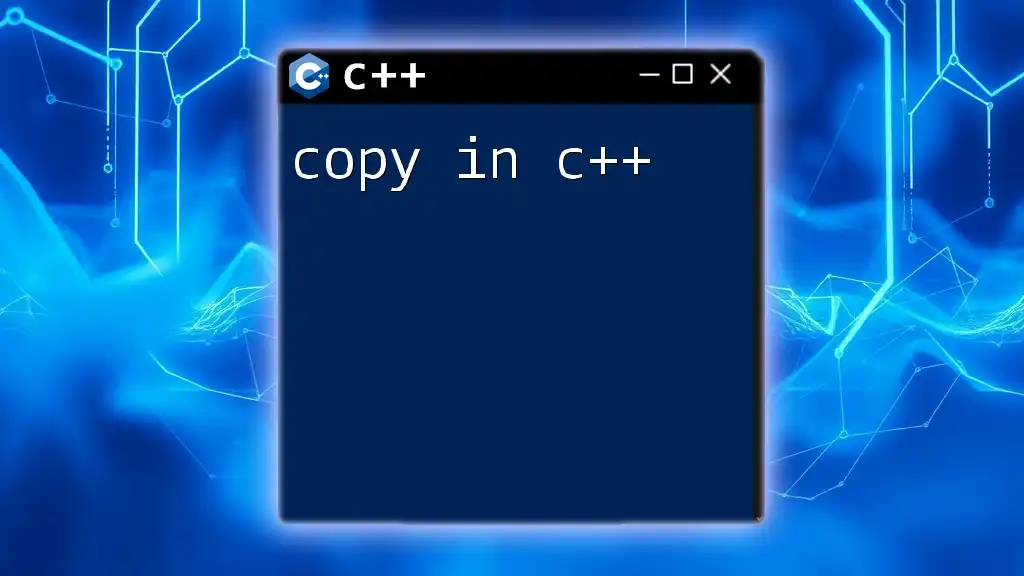
Function Scope
Understanding Function Parameters
When variables are passed to functions as parameters, their accessibility is also limited to the scope of the function. These parameters exist only during the function's execution:
void functionScopeExample(int a) {
cout << a; // Accessible within the function
}
// cout << a; // Error: 'a' is not accessible here
In this example, `a` can be accessed only inside `functionScopeExample`. Outside this function, it is not recognized, thus reinforcing the concept of function scope.
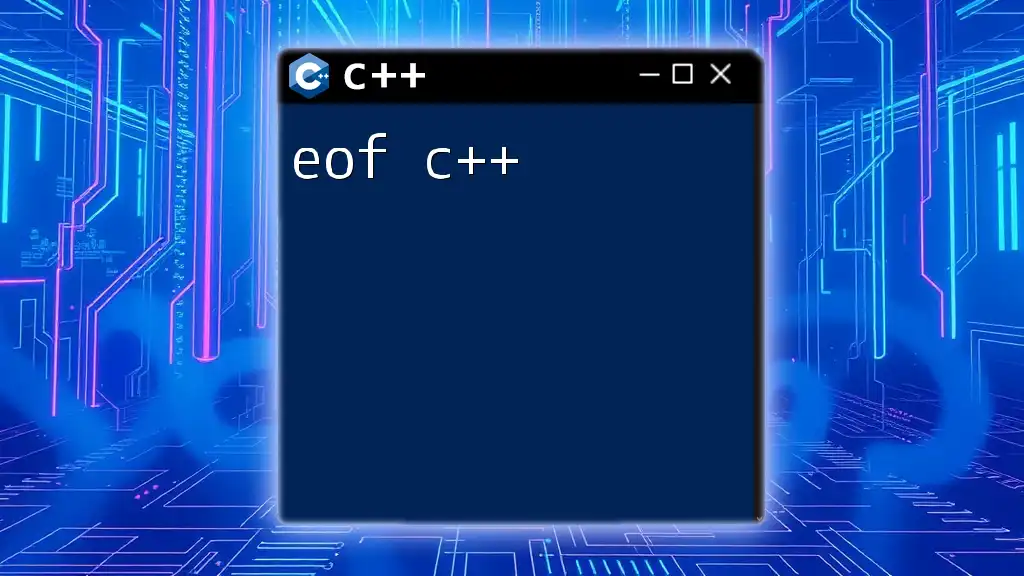
Class Scope
Member Variables and Functions
In object-oriented programming with C++, class members (variables and functions) can have different accessibility levels defined by access specifiers like `public`, `private`, and `protected`. Here’s how this works:
class MyClass {
public:
int memberVar;
void memberFunction() {
cout << memberVar; // Accessible here
}
};
In this case, `memberVar` exists within the scope of `MyClass`, and you can access it from member functions. However, outside of `MyClass`, you cannot access `memberVar` directly unless you create an object of `MyClass`.
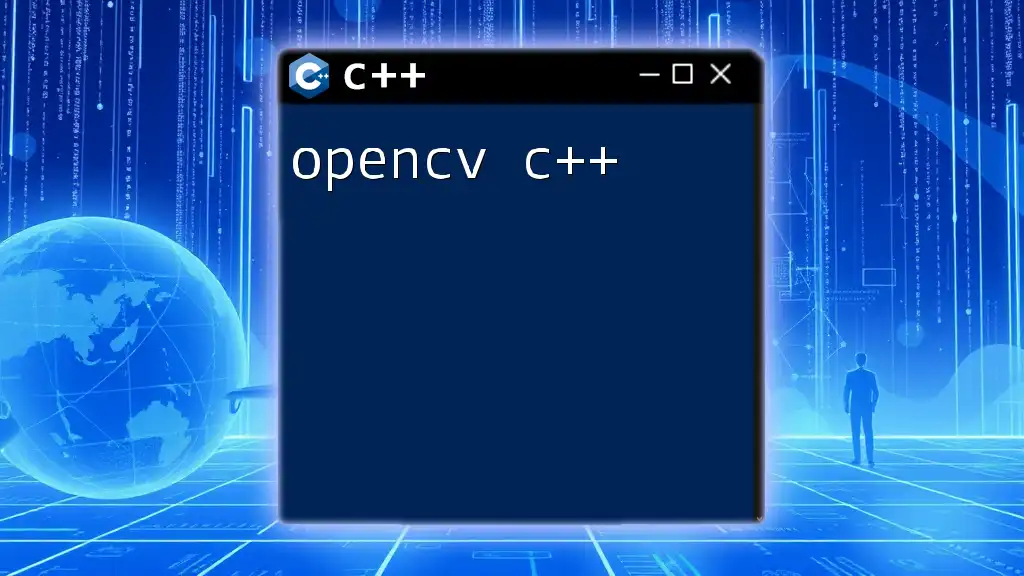
Static Scope
What is Static Storage Duration?
The `static` keyword in C++ provides a way to maintain the value of a variable between function calls. When declared as `static`, the variable retains its value even after the function exits. For example:
void staticScopeExample() {
static int counter = 0; // Retains value between function calls
counter++;
cout << counter;
}
If you call `staticScopeExample()` multiple times, `counter` will continue to increment rather than reset, illustrating how `static` changes the visibility and lifetime of a variable.
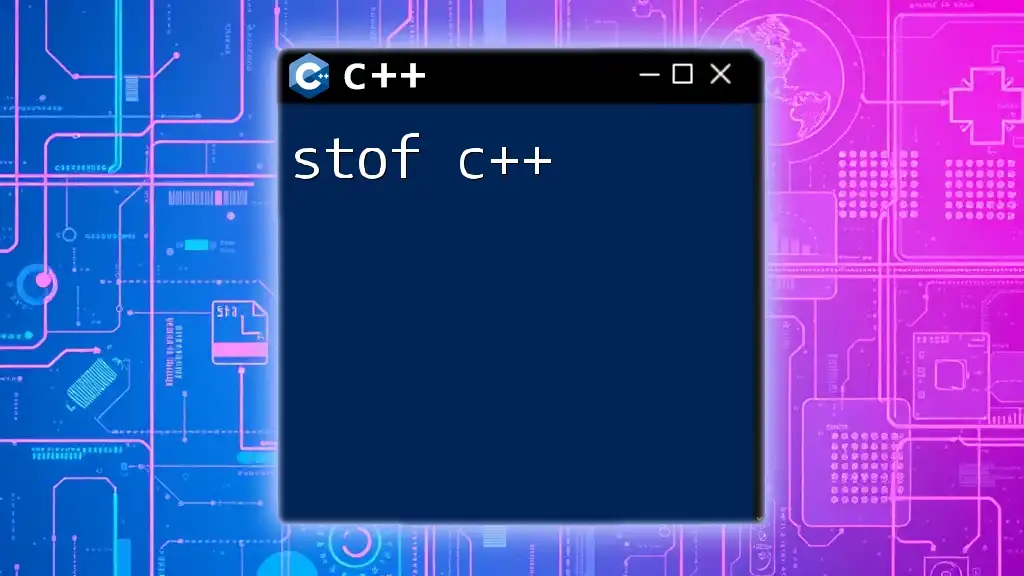
Scope Resolution Operator
Purpose of the Scope Resolution Operator (`::`)
The scope resolution operator helps clarify which variable or function you are referring to, particularly in cases of naming conflicts. It allows access to global variables or class members without ambiguity:
int globalVar = 50;
void scopeResolutionExample() {
int globalVar = 20;
cout << ::globalVar; // Accessing the global variable
}
In this scenario, `::globalVar` explicitly accesses the global variable despite the presence of a local variable with the same name, emphasizing the importance of clarity when dealing with scope.
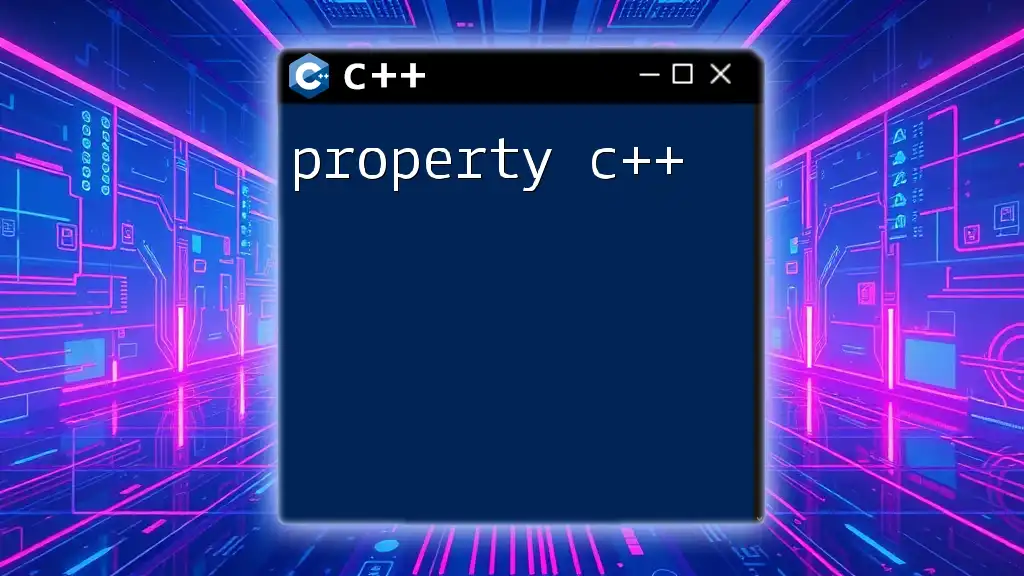
Conclusion
Understanding the scope of C++ is vital for effective programming. From local and global scopes to function parameters and class members, each type of scope plays a significant role in managing code visibility and lifetime. Mastering these concepts not only aids in writing cleaner code but also promotes better programming practices that can prevent errors and enhance maintainability. Make sure to experiment with these concepts to solidify your understanding, as they lay the foundation for proficient C++ programming.
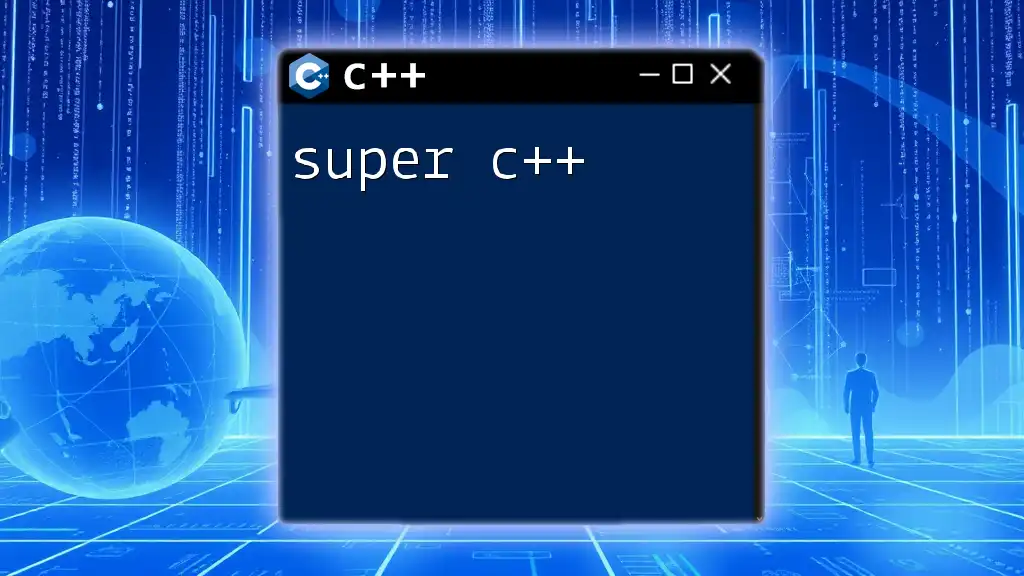
Additional Resources
Delve deeper into the scope of C++ through recommended books, online courses, coding challenges, and community forums!