OpenSSL is a powerful library in C++ for implementing cryptography and secure communication, allowing developers to encrypt, decrypt, and manage SSL/TLS connections efficiently.
Here's an example of using OpenSSL in C++ to generate an SHA256 hash of a string:
#include <iostream>
#include <openssl/sha.h>
int main() {
std::string input = "Hello, OpenSSL!";
unsigned char hash[SHA256_DIGEST_LENGTH];
SHA256(reinterpret_cast<const unsigned char*>(input.c_str()), input.size(), hash);
// Print hash in hexadecimal format
for(int i = 0; i < SHA256_DIGEST_LENGTH; i++) {
printf("%02x", hash[i]);
}
std::cout << std::endl;
return 0;
}
What is OpenSSL?
OpenSSL is a widely-used cryptographic library that enables secure transactions over networks. It provides robust tools for implementing cryptographic protocols such as SSL (Secure Sockets Layer) and TLS (Transport Layer Security). In the context of openssl c++, it allows developers to integrate secure communication features directly into their C++ applications.
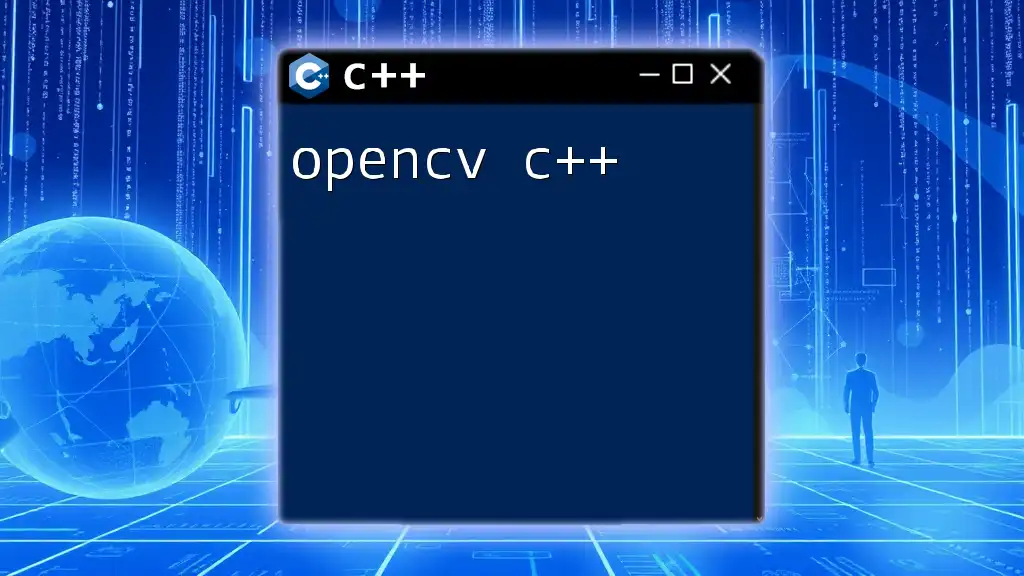
Why Use OpenSSL with C++?
Integrating OpenSSL with your C++ applications enhances security through proper encryption, ensuring data integrity and confidentiality. Common use cases include:
- HTTPS connections that secure web traffic.
- Data encryption to protect sensitive information during storage and transit.
- Digital signatures to verify the authenticity of messages or programs, thereby ensuring trusted communications.
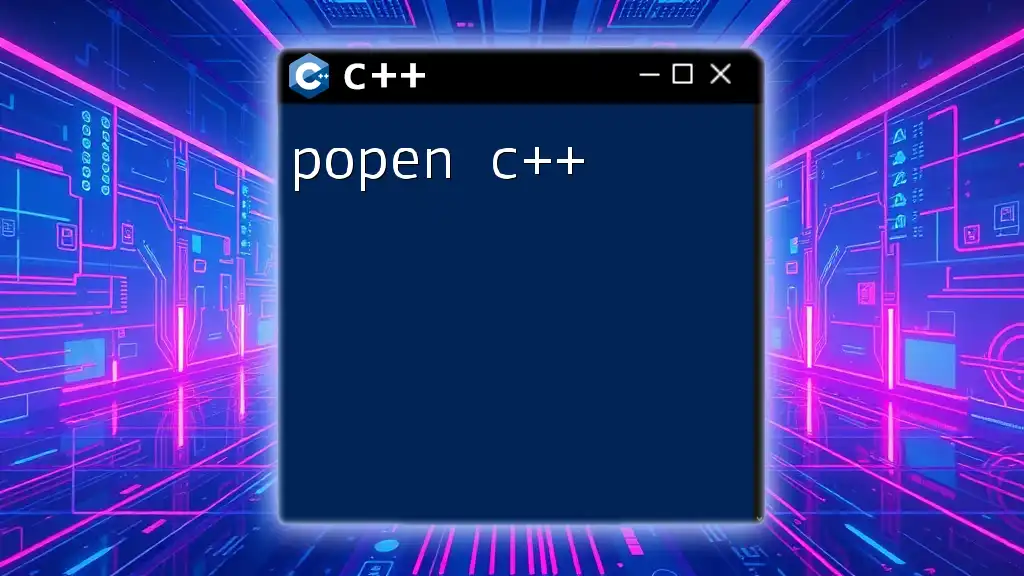
Setting Up OpenSSL for C++
Installing OpenSSL
Before diving into coding, you must first install OpenSSL on your operating system. For different platforms, the process may vary slightly:
- On Windows, precompiled binaries are available, or you can compile from source using MinGW.
- For macOS, you can use Homebrew:
brew install openssl
- On Linux (Debian-based systems), install OpenSSL via:
sudo apt-get install libssl-dev
Configuring Your C++ Project with OpenSSL
After installation, you’ll need to incorporate OpenSSL into your C++ project. If you’re using CMake, for example, you would include OpenSSL like so:
find_package(OpenSSL REQUIRED)
target_link_libraries(your_target OpenSSL::SSL OpenSSL::Crypto)
This ensures that your project can locate and link against the OpenSSL libraries effectively.
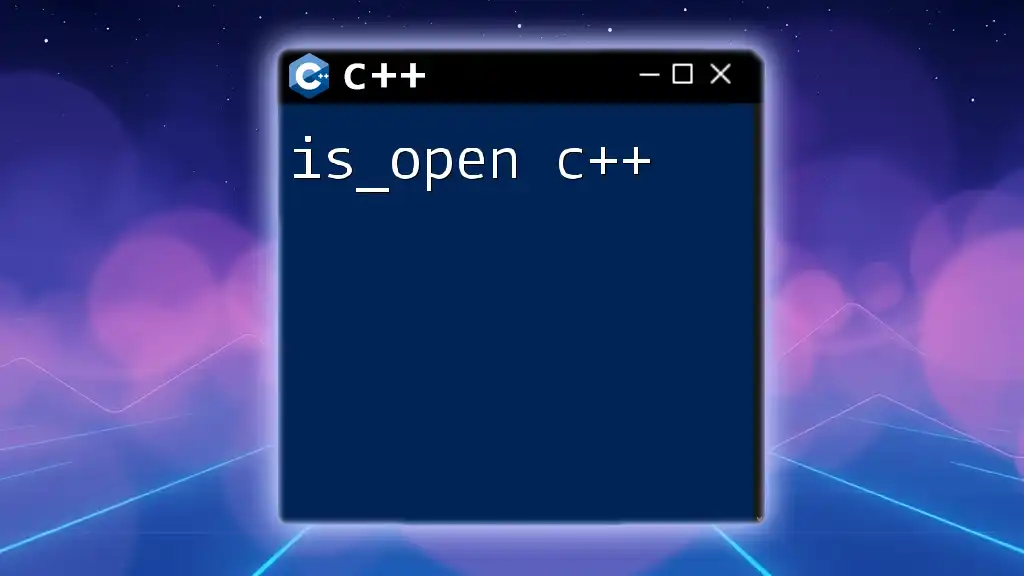
Fundamental Concepts of OpenSSL
Key Cryptography
Understanding the difference between symmetric and asymmetric encryption is crucial for implementing security properly.
- Symmetric Encryption uses the same key for both encryption and decryption, making it fast and efficient for large datasets but requiring secure key distribution.
- Asymmetric Encryption employs a pair of keys (public and private) which offers secure key distribution but at the cost of speed.
Understanding Cryptographic Hash Functions
A cryptographic hash function takes an input and produces a fixed-size string of characters. The output is unique to each unique input, making it ideal for data integrity checks. OpenSSL supports numerous hash functions; two common types are SHA-256 and MD5.
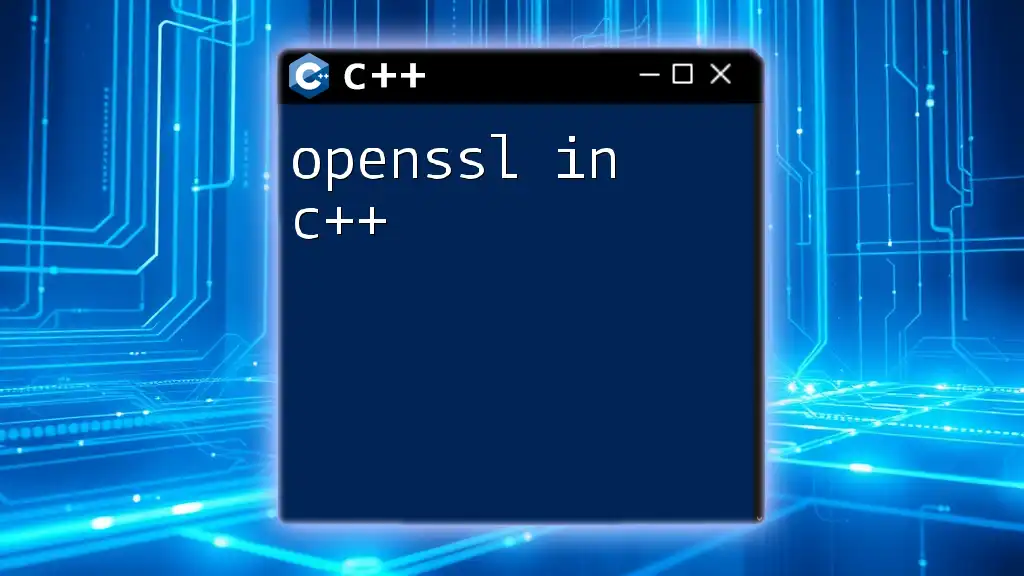
Basic OpenSSL Commands in C++
Creating and Managing Keys
Generating a random key is essential for symmetric encryption. Here’s how to do it with OpenSSL in C++:
#include <openssl/rand.h>
unsigned char key[32]; // AES-256
RAND_bytes(key, sizeof(key));
Encrypting and Decrypting Data
Encrypting data requires you to set up an AES key and apply it to your plain text:
#include <openssl/aes.h>
AES_KEY encryptKey;
AES_set_encrypt_key(key, 256, &encryptKey);
unsigned char input[16]; // Plain text of 16 bytes
unsigned char ciphertext[16];
AES_encrypt(input, ciphertext, &encryptKey);
The decryption process is just as crucial:
AES_KEY decryptKey;
AES_set_decrypt_key(key, 256, &decryptKey);
unsigned char output[16];
AES_decrypt(ciphertext, output, &decryptKey);
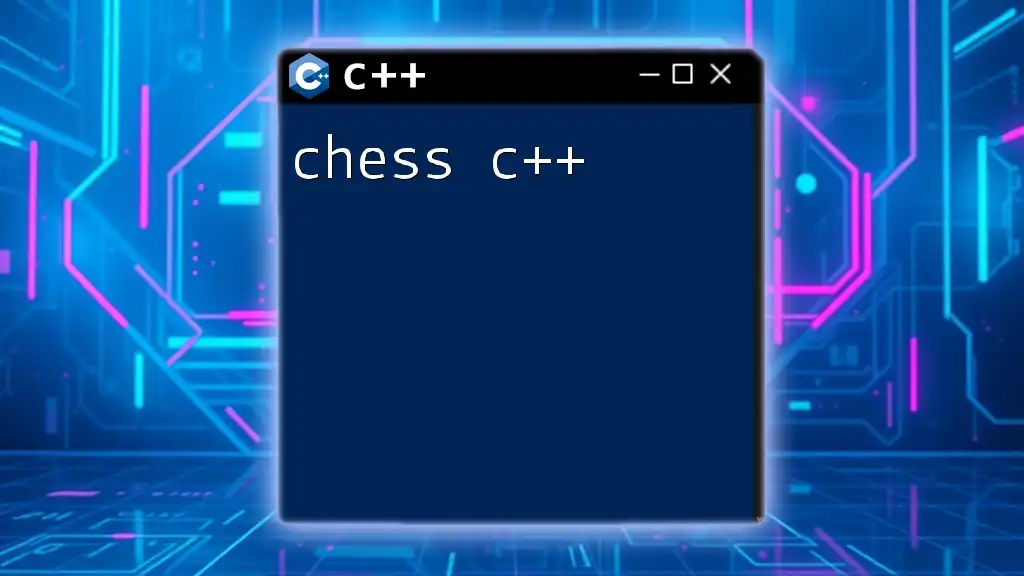
Handling SSL/TLS in C++
Creating SSL Context
The SSL_CTX structure holds data necessary for establishing SSL connections. Create one using:
SSL_CTX *ctx = SSL_CTX_new(TLS_method());
This method is a flexible way to work with various TLS protocols.
Establishing SSL Connections
Creating an SSL connection involves binding it to a socket file descriptor:
SSL *ssl = SSL_new(ctx);
SSL_set_fd(ssl, socket_fd);
SSL_connect(ssl);
This sets up the secure communication channel.
Sending and Receiving Data Securely
Once the SSL connection is established, you can securely exchange data:
const char *message = "Hello, secure world!";
SSL_write(ssl, message, strlen(message));
char buffer[1024];
SSL_read(ssl, buffer, sizeof(buffer));
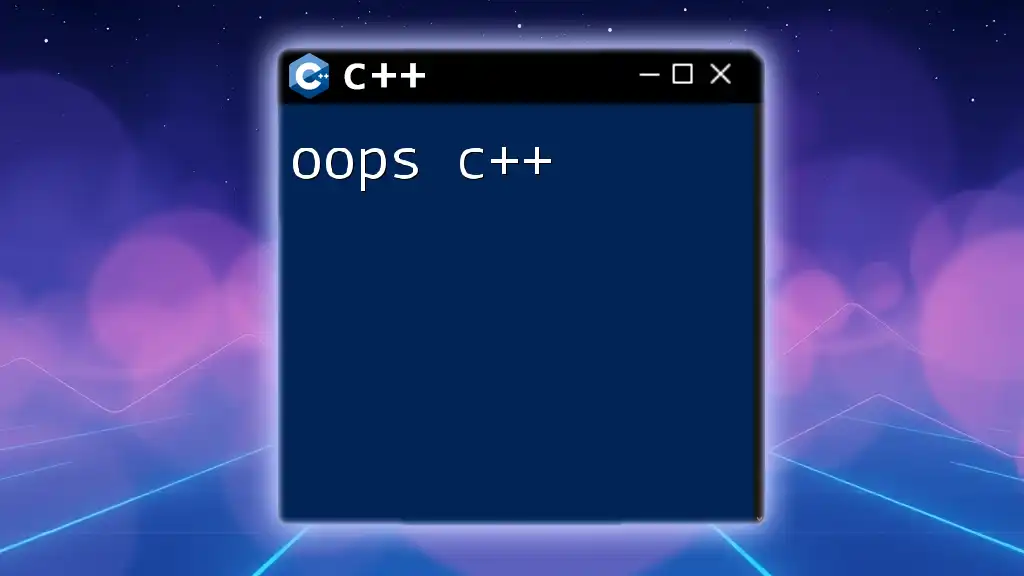
Error Handling and Debugging
Common OpenSSL Errors
Working with OpenSSL may lead to various errors. Familiarizing yourself with common codes can save a lot of debugging time.
Using ERR_get_error for Debugging
Integrating proper error handling is essential for robust applications. You can retrieve detailed error information using:
unsigned long err = ERR_get_error();
if(err) {
printf("Error: %s\n", ERR_error_string(err, NULL));
}
This snippet captures and prints any error that occurs while using OpenSSL functions.
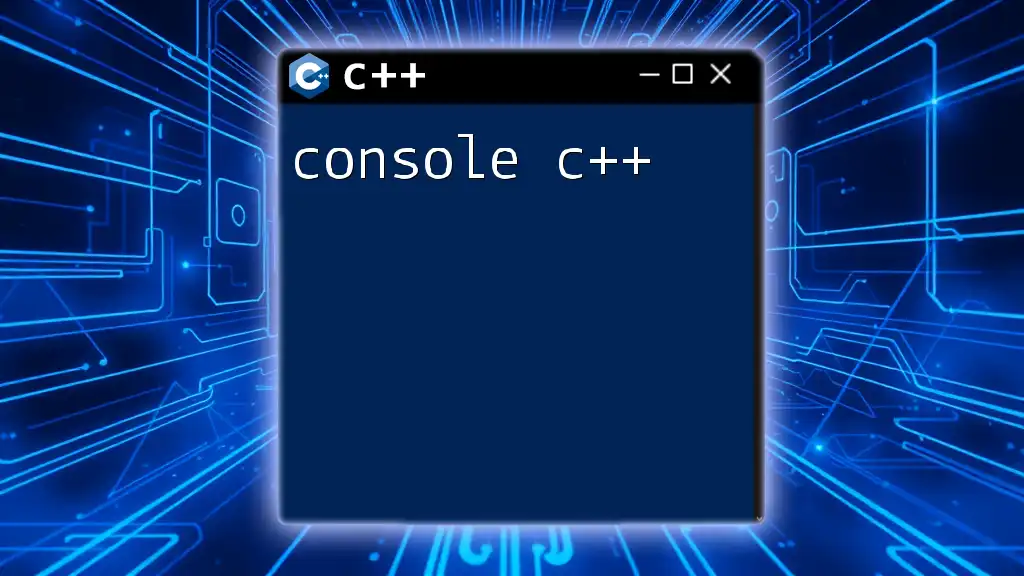
Advanced OpenSSL Features
Certificate Management
Understanding and managing X.509 certificates is crucial for establishing trusted connections. OpenSSL provides tools for generating self-signed certificates.
Implementing Custom Encryption Algorithms
While OpenSSL comes packed with numerous algorithms, you can extend it by introducing your custom cipher. This is an advanced topic that allows complete flexibility for specialized requirements.
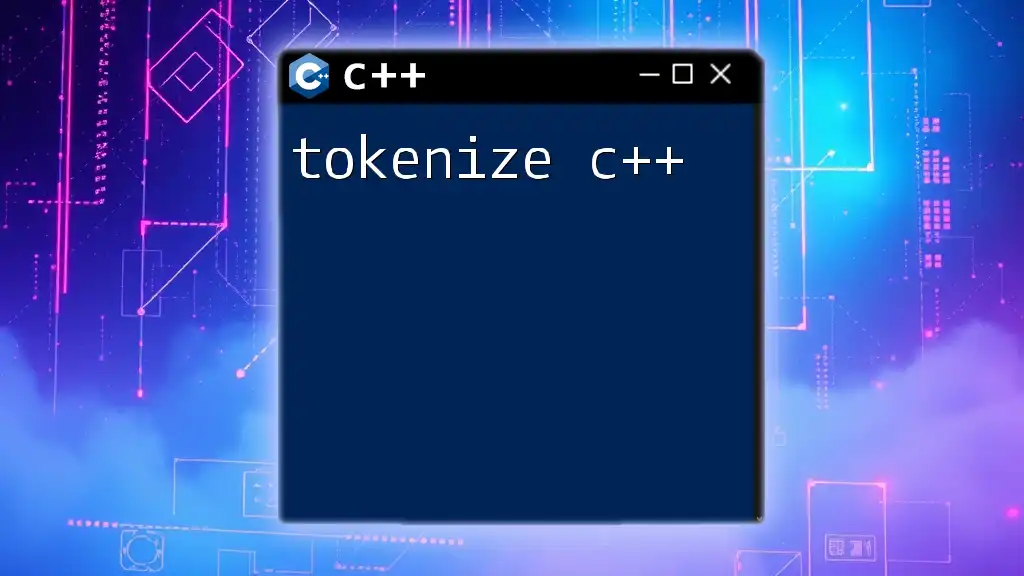
Best Practices for Using OpenSSL in C++
Security Recommendations
To maximize security, keep OpenSSL updated regularly, and implement strong algorithms while adhering to proper key management practices.
Performance Optimization Tips
For high-performance applications, profile your OpenSSL usage and apply optimizations as necessary. Analyze bottlenecks and ensure you are using non-blocking I/O for SSL operations to maintain responsiveness.
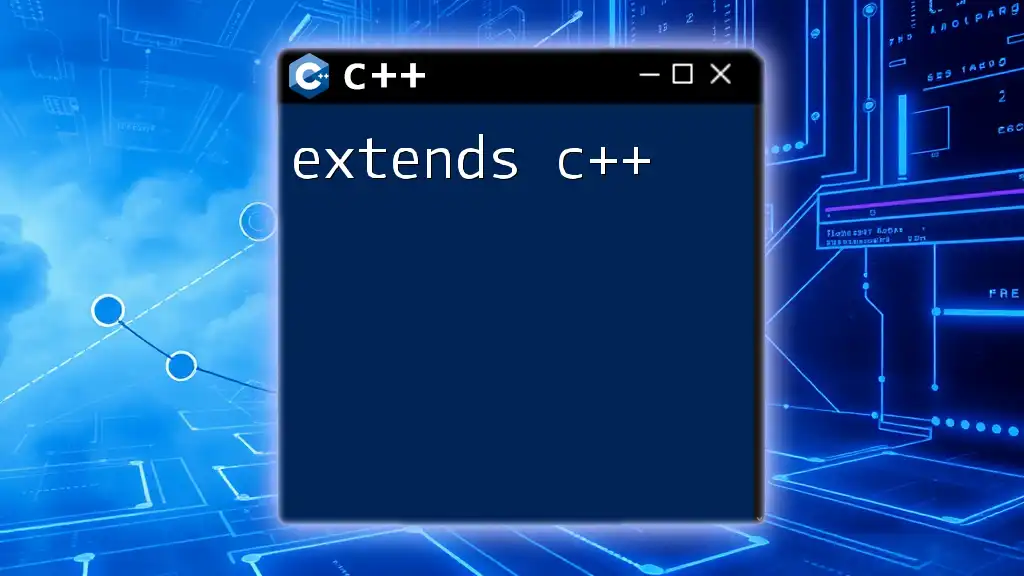
Conclusion
Understanding openssl c++ is vital for developing secure applications. This guide has covered installation, basic commands for encryption, SSL/TLS handling, error management, and advanced topics. Remember to keep learning and explore the vast resources available to harness the full power of OpenSSL in your C++ projects.
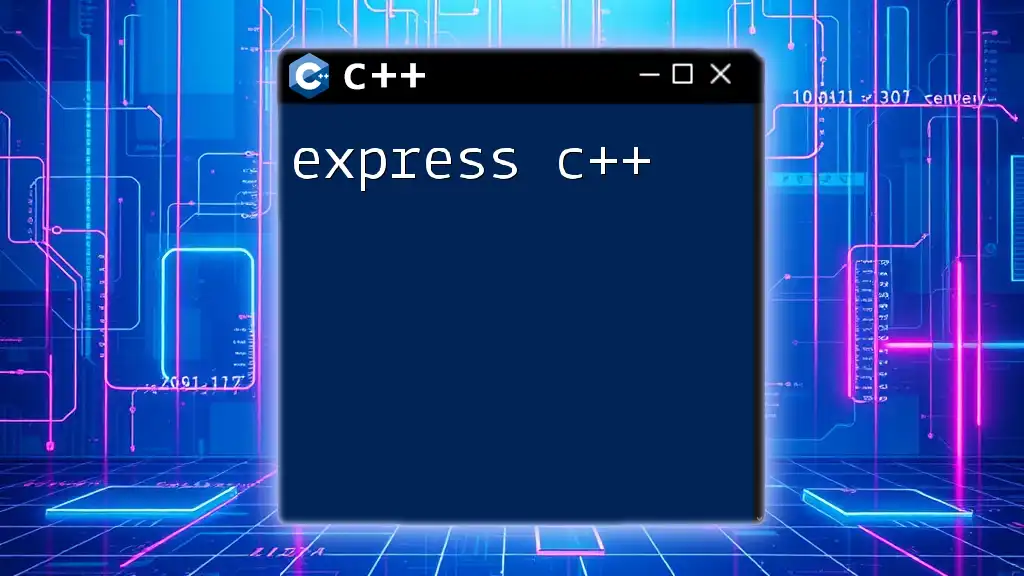
References
To deepen your knowledge further, consult the OpenSSL documentation, engage with community forums, and consider diving into tutorials that focus on specific use cases within the OpenSSL library.