Global constants in C++ are variables that are declared outside of any function or class, making them accessible throughout the entire program while ensuring their values cannot be modified.
Here's a code snippet demonstrating the use of a global constant:
#include <iostream>
const int GLOBAL_CONSTANT = 100;
int main() {
std::cout << "The value of the global constant is: " << GLOBAL_CONSTANT << std::endl;
return 0;
}
Understanding `const` in C++
What is `const`?
In C++, the `const` keyword is used to define constants, which are variables whose values cannot be changed after they are defined. This provides a layer of assurance that critical values remain unchanged throughout the execution of the program. When a variable is declared as `const`, the compiler will enforce this immutability, raising an error if any attempt is made to modify its value.
Local vs. Global `const` Variables
The difference between local constants and global constants is fundamental to understanding scope and usability in C++. A local constant is declared within a function or a block and can only be accessed from that scope. Conversely, a global constant is declared outside of all functions, making it accessible from any function within the same file. This broad accessibility makes global constants particularly useful for values that are shared across multiple functions or even multiple files.
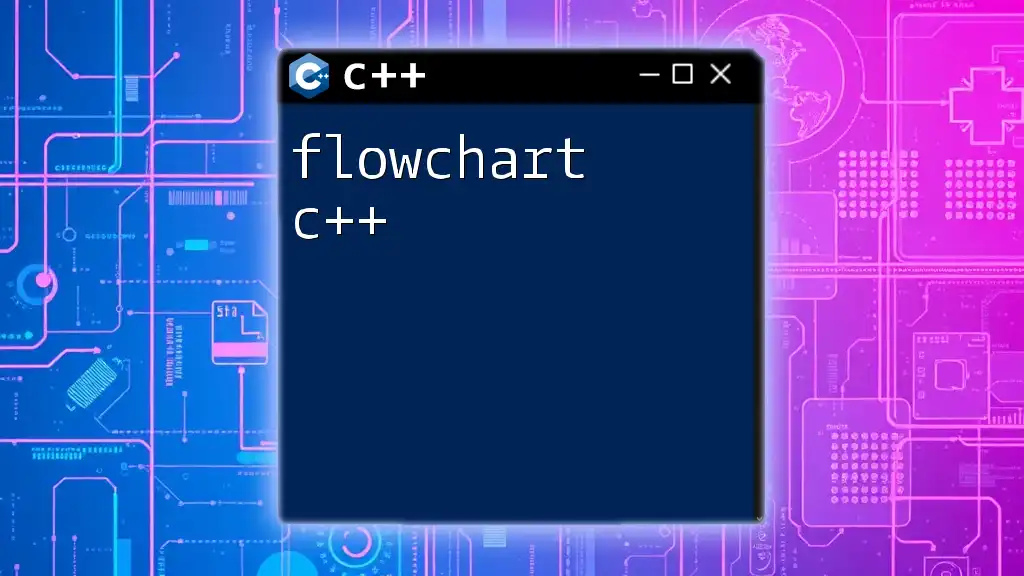
Declaring Global Constants in C++
Defining Global Constants
Global constants are declared using the `const` keyword, specifying the desired data type and assigning a value at the same time. The syntax is straightforward:
const int MAX_SIZE = 100;
In the above example, `MAX_SIZE` is a global constant of type `int` with a value of `100`. This constant can now be used throughout the program.
Case Study: Global Constants in Action
Consider a simple program that demonstrates the use of global constants. Here, we create a constant that holds the maximum number of students allowed in a classroom:
#include <iostream>
using namespace std;
const int MAX_STUDENTS = 30;
void displayMaxStudents() {
cout << "The maximum number of students in a classroom is: " << MAX_STUDENTS << endl;
}
int main() {
displayMaxStudents();
return 0;
}
In this example, `MAX_STUDENTS` serves as a reference point that can be easily modified if the requirements change, without having to track down multiple instances in your code.
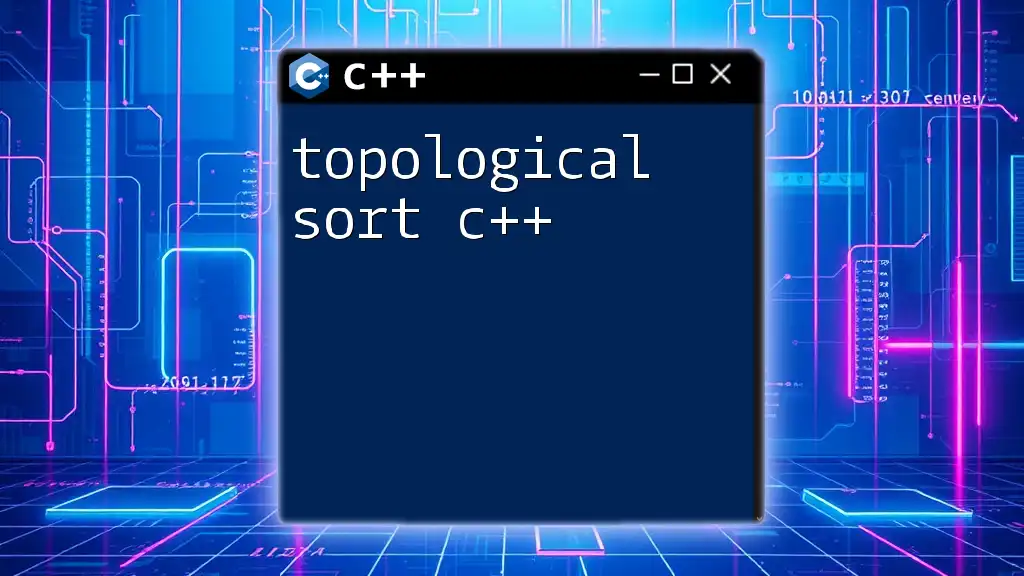
Benefits of Using Global Constants
Code Clarity and Maintenance
One of the primary advantages of using global constants is the enhanced readability they bring to your code. When someone reads your code, global constants provide clear references to meaningful values and intent. For example, instead of seeing a hardcoded number like `100`, your code might use `MAX_SIZE`, which immediately conveys its purpose and meaning.
Consistent Behavior Across the Codebase
Another key benefit of global constants is the consistency they ensure across your program. If a constant needs to be updated, changing its value in one location—where it is defined—automatically updates all references throughout your code. This minimizes errors and prevents discrepancies where some functions might use outdated values.
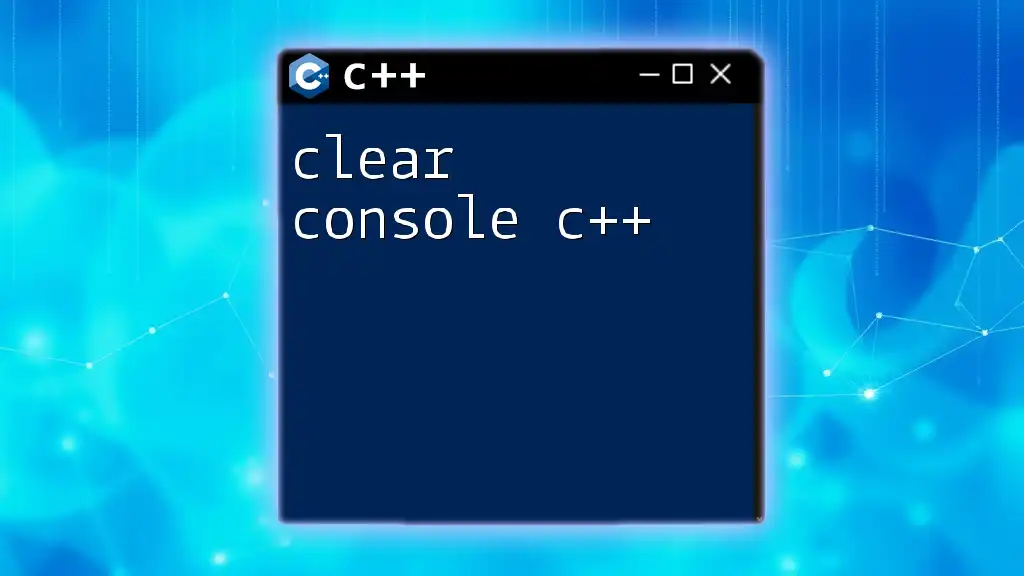
Best Practices for Global Constants
Naming Conventions
When defining global constants, adopting consistent naming conventions is crucial. A common practice is to write their names in uppercase letters, using underscores to separate words. This distinguishes constants from regular variables, facilitating easier recognition. For example:
const int MAX_CONNECTIONS = 10;
Avoiding Overuse
While global constants can simplify your code, it’s essential to avoid overuse. Relying too heavily on global constants can lead to cluttered code and make debugging more complex. Only use global constants when they genuinely serve a purpose across multiple functions.
Group Related Constants
Organizing constants into structures or classes can improve code management significantly. By grouping related constants, you not only reduce global namespace pollution but also enhance logical structure within your codebase.
struct Settings {
static const int MAX_USERS = 100;
static const float PI;
};
const float Settings::PI = 3.14159;
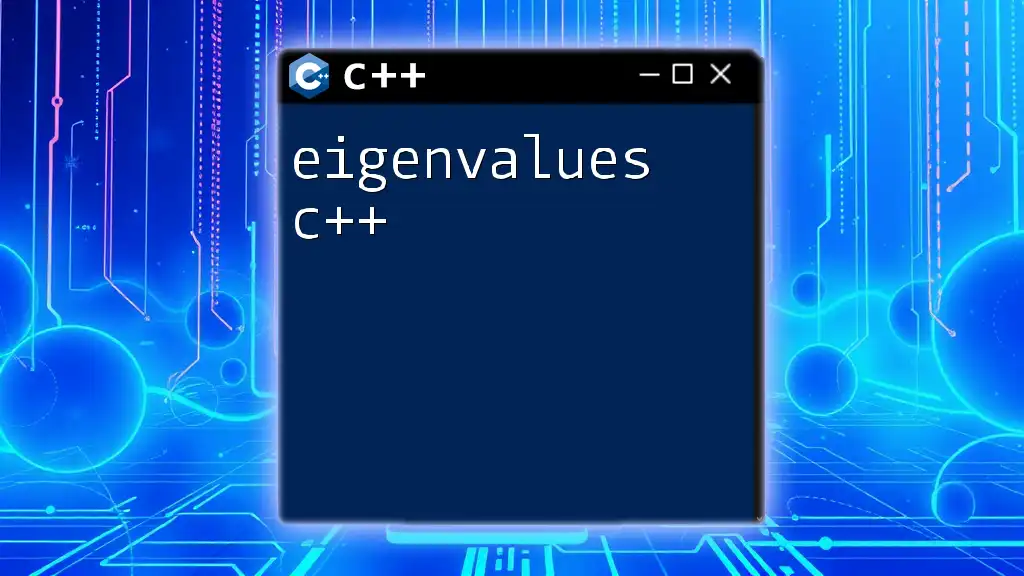
Scope and Lifetime of Global Constants
Understanding Scope
The scope of a variable refers to its visibility within the program. Global constants are visible across all functions in the file where they are defined. If you attempt to access a global constant from another file, it must be declared with the `extern` keyword in the file where you want to use it.
Lifetime Considerations
The lifetime of global constants extends for the entire duration of the program's execution. They are initialized before the program starts running and remain in memory until the program terminates. This persistent nature of global constants makes them suitable for values that never change throughout the lifetime of the application.
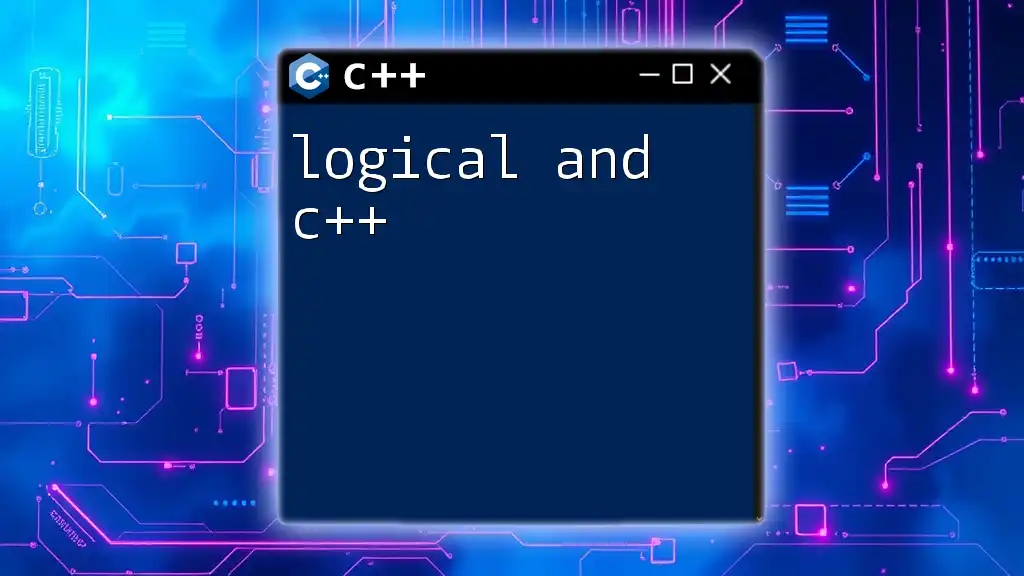
Example Implementation
Let’s create a more complete example that employs global constants to calculate the area of a circle. This example illustrates how a global constant can simplify calculations and enhance program clarity.
#include <iostream>
using namespace std;
const float PI = 3.14159;
const int MAX_RADIUS = 100;
float calculateArea(float radius) {
if (radius > MAX_RADIUS) {
cout << "Radius exceeds maximum allowed value." << endl;
return 0;
}
return PI * radius * radius;
}
int main() {
float radius;
cout << "Enter radius (max " << MAX_RADIUS << "): ";
cin >> radius;
float area = calculateArea(radius);
if (area > 0) {
cout << "Area of the circle: " << area << endl;
}
return 0;
}
In this implementation, `PI` and `MAX_RADIUS` are used as global constants, promoting clarity and maintaining consistency for the calculation of the circle's area.
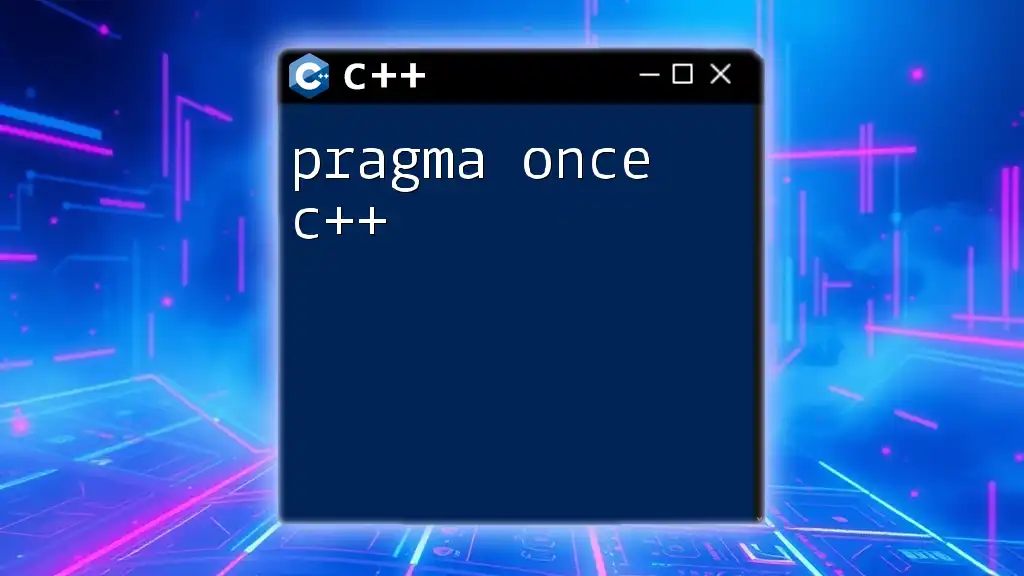
Common Mistakes with Global Constants
Relying Too Much on Globals
One common mistake when using global constants is the over-reliance on them. While they can simplify code, high dependence can make code difficult to test and reduce modularity. It’s crucial to balance the use of global constants with the need for encapsulation within classes and functions.
Not Re-using Constants Effectively
Another pitfall is failing to utilize defined constants properly, such as hardcoding values instead of referencing global constants. This practice undermines the benefits of having constants and leads to errors that can be difficult to diagnose later. Always strive to replace magic numbers with meaningful const names.
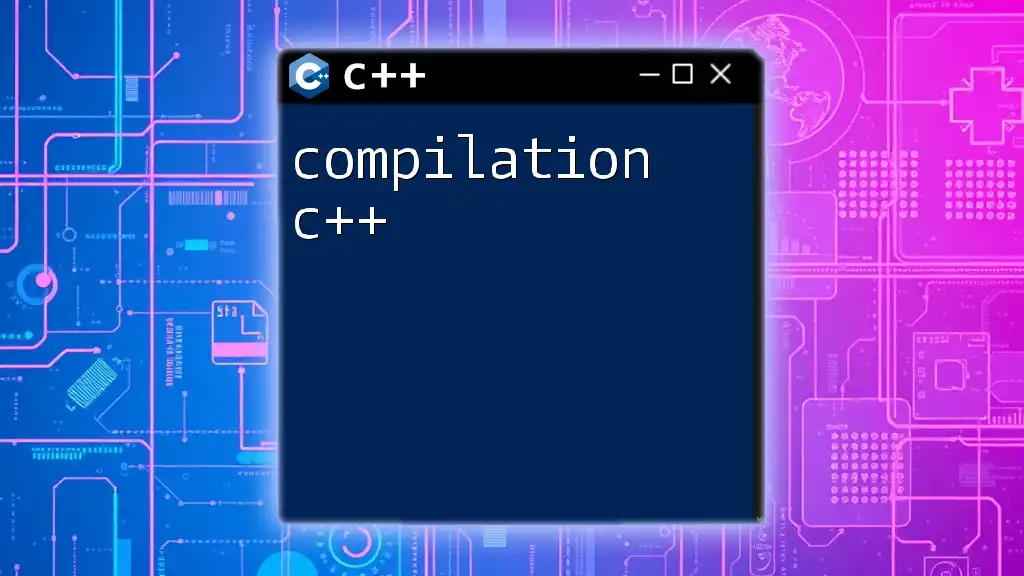
Further Learning Resources
To deepen your understanding of global constants in C++, consider the following resources:
-
Recommended Books and Online Courses: Many books cover advanced C++ concepts and best practices, which can provide more context and examples on global constants and their best use.
-
Community Support and Forums: Online platforms such as Stack Overflow or dedicated C++ forums can be invaluable for sharing knowledge, troubleshooting issues, and learning from experienced developers.
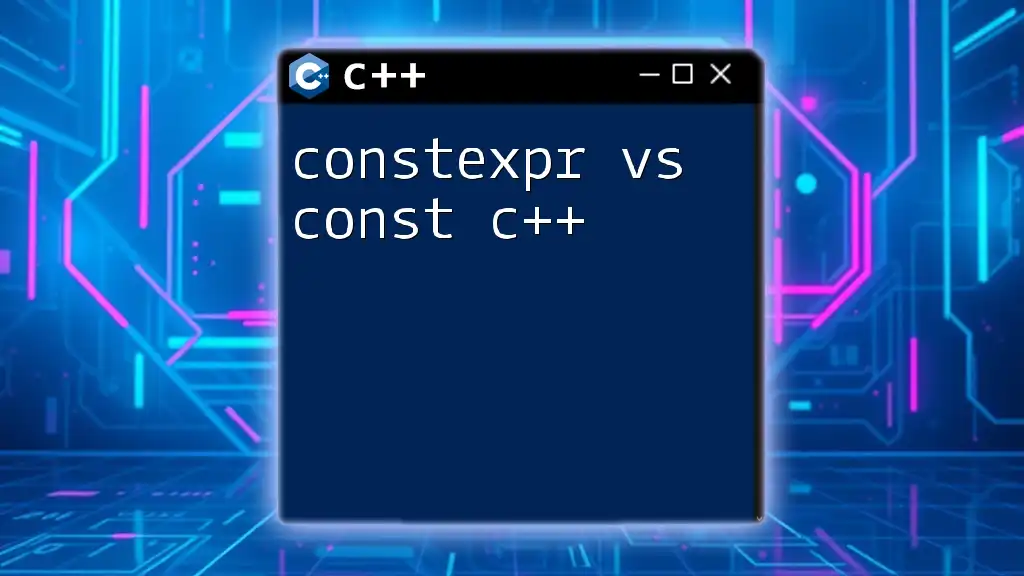
Conclusion
In summary, global constants are an essential feature in C++ programming, providing clarity, consistency, and maintainability. By leveraging global constants appropriately, you can significantly improve the quality of your code and streamline the development process. As you practice, challenge yourself to implement global constants in your projects, which will foster a deeper understanding of their advantages and best practices in C++.