In C++, `constexpr` declares that a variable's value is constant and can be evaluated at compile-time, while `const` simply means the variable's value cannot be changed after initialization, regardless of when it is evaluated.
constexpr int squared(int x) { return x * x; }
const int value = squared(5);
Understanding const in C++
What is const?
`const` is a keyword in C++ that is used to define constants. When a variable is declared `const`, its value cannot be modified after initialization. This immutability is crucial in programming as it ensures that certain values remain constant, thereby preventing accidental changes that could lead to bugs.
Example of const Usage
Here’s a straightforward example demonstrating how to use `const`:
const int MAX_SIZE = 100;
In this example, `MAX_SIZE` is defined as a constant integer. Attempts to modify `MAX_SIZE` later in the code will result in a compilation error.
Key Characteristics of const
-
Usage Flexibility: `const` can be applied to various data types, including `int`, `float`, pointers, and user-defined types.
-
Mutability Control: While `const` itself prevents modification of the variable it qualifies, it's important to note that it does not guarantee immutability of objects pointed to by pointers if the pointer itself is not declared as `const`.
const int* ptr = &MAX_SIZE; // Pointer to const int
Here, `ptr` points to a `const` integer, meaning the integer it points to cannot be changed through `ptr`. However, if `ptr` was a regular pointer, you could modify the value of `MAX_SIZE`.
- Run-time Evaluation: Values declared with `const` can be determined at run-time, providing flexibility in certain scenarios where dynamic computation is required.
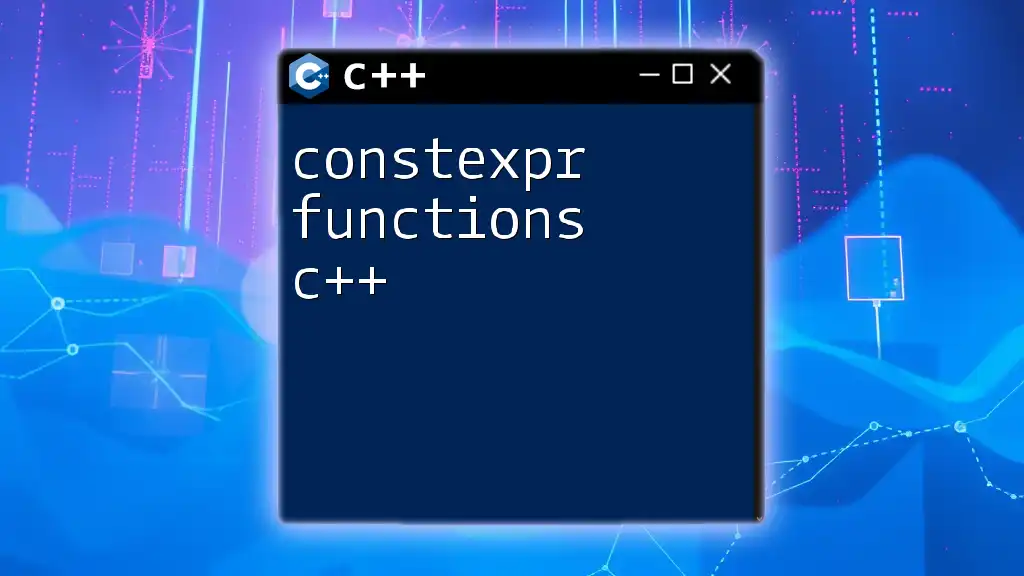
Understanding constexpr in C++
What is constexpr?
Introduced in C++11, `constexpr` is a specifier used to declare that it is possible for a function or variable to be evaluated at compile time. This provides an additional layer of performance optimization, as it allows certain computations to be resolved before the program runs.
Example of constexpr Usage
A simple example of a `constexpr` function is as follows:
constexpr int square(int x) {
return x * x;
}
The `square` function can be evaluated at compile time, allowing the compiler to optimize calls to `square` with constant arguments.
Key Characteristics of constexpr
-
Compile-time Evaluation: The key feature of `constexpr` is its requirement for compile-time evaluation. This makes it suitable for use in constant expressions.
-
Limitations: While `constexpr` allows for powerful optimizations, it comes with restrictions. For example, `constexpr` functions must contain a return statement and cannot have any side effects.
constexpr int getFive() { return 5; }
-
Complexity Handling: With C++20, `constexpr` has gained even more capabilities, allowing `constexpr` functions to contain more complex operations, including dynamic memory allocation.
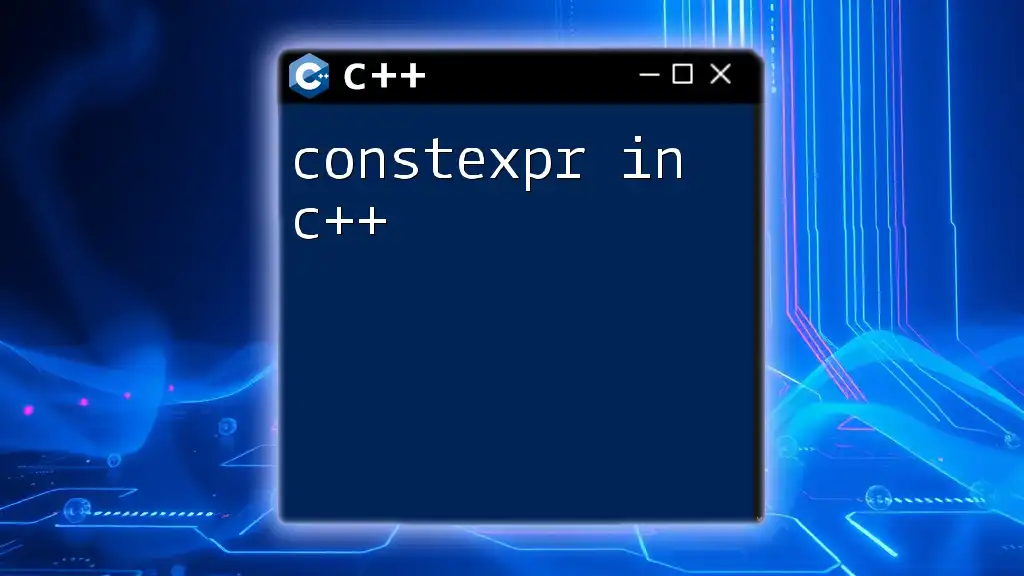
Comparing const and constexpr
Fundamental Differences
- Storage Duration: `const` variables are typically stored in memory (static or automatic), while `constexpr` variables are often evaluated at compile time, possibly stored directly in code either inlined or optimized.
- Type of Computation: `const` can be evaluated at run-time, allowing for dynamic values, whereas `constexpr` supports compile-time constant expressions exclusively.
When to Use Each
-
You should prefer using `const` when you have values that might need to be assigned at run-time. This is especially relevant for constants dependent on external input or conditions.
-
In contrast, use `constexpr` when you want to ensure that your value is a compile-time constant, especially for scenarios involving templates, constant expressions, or optimization in performance-critical areas.
Performance Implications
Using `constexpr` can lead to performance benefits due to its compile-time evaluation feature. For example, when configuring arrays or initializing data structures that depend on constant values, `constexpr` can significantly reduce run-time overhead.
constexpr int arraySize = 10;
int myArray[arraySize]; // Fully resolved at compile-time
By employing `constexpr`, the array size is determined at compile time, leading to optimized code and in some scenarios, better memory management.
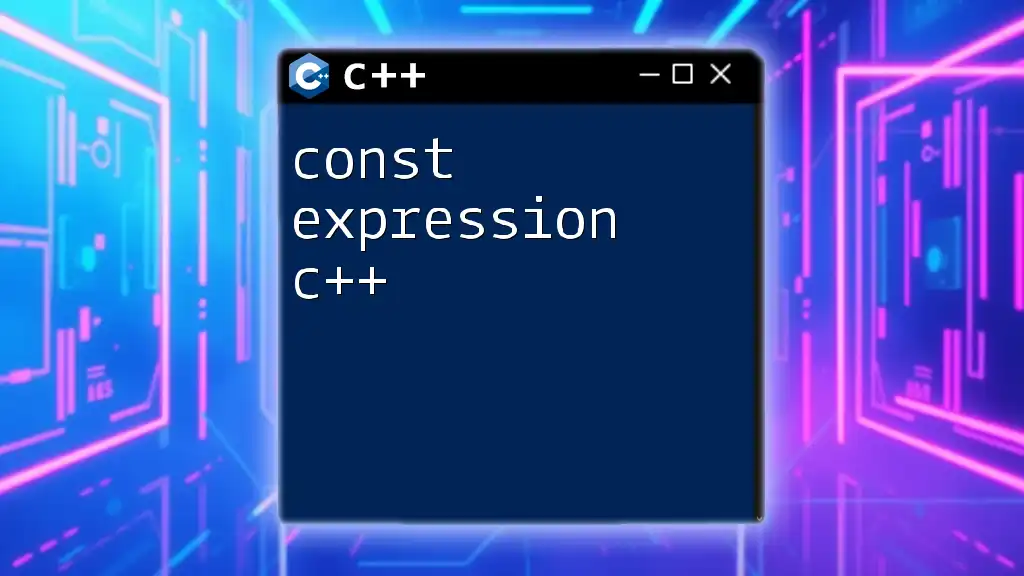
Practical Examples
Using const in Classes
When working with classes, `const` can be utilized to protect member functions from modifying the object:
class MyClass {
public:
void Display() const {
std::cout << "Displaying data." << std::endl;
} // This method cannot modify member variables
};
Here, the `Display` function is declared as `const`, indicating that it does not alter the state of the instance of `MyClass`.
Using constexpr in Classes
In C++, `constexpr` can be particularly useful for building classes where constructors and member functions compute values at compile time:
class Rectangle {
public:
constexpr Rectangle(int w, int h) : width(w), height(h) {}
constexpr int area() const { return width * height; }
private:
int width, height;
};
In this example, both the constructor and `area` function are defined as `constexpr`, allowing computations to occur at compile time, which can enhance efficiency.
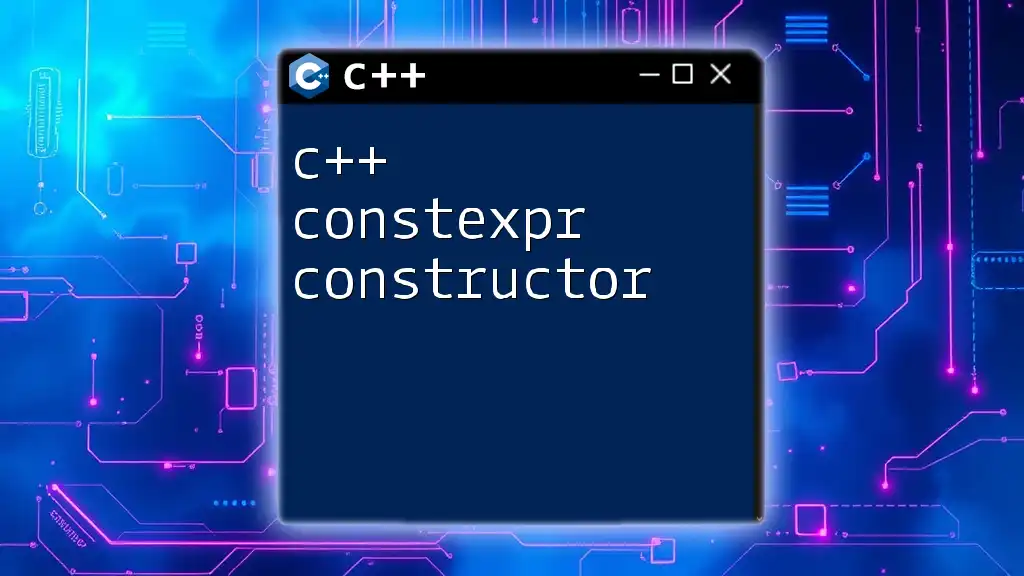
Best Practices
When to Prefer const
It's advisable to use `const` for variables that do not need to change throughout their lifespan. This improves code clarity and safety. Use `const` for:
- Constants used for configuration settings.
- Function parameters that should not be modified.
When to Prefer constexpr
Choose `constexpr` when performance matters, and compile-time evaluation can play a crucial role. Use `constexpr` for:
- Functions that will be heavily utilized and benefit from compile-time resolution.
- Values that will be utilized in constant expressions, especially with templates.
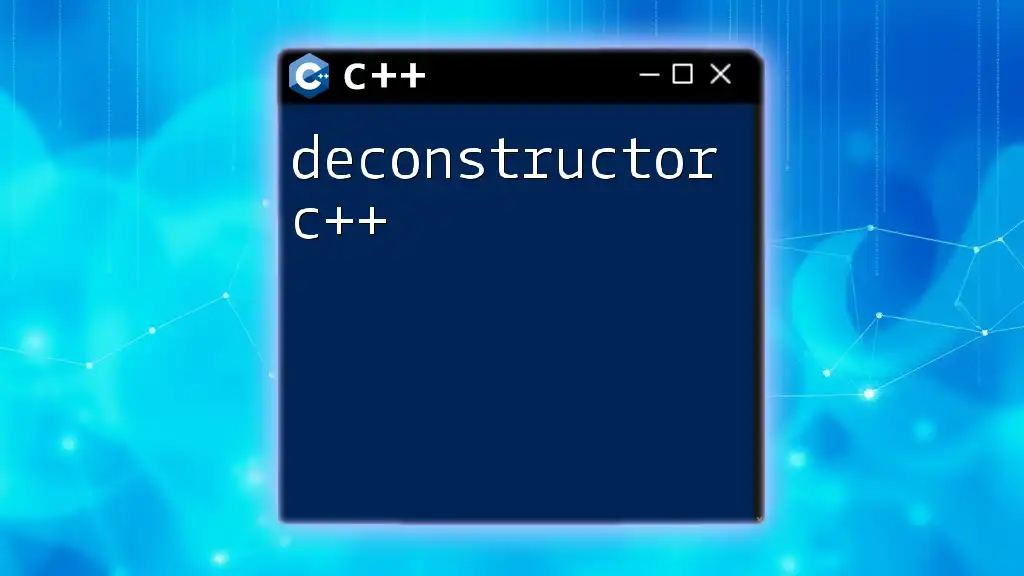
Conclusion
Understanding the distinctions and appropriate uses of `constexpr` versus `const` is fundamental for C++ programmers. While `const` offers flexibility for managing values, `constexpr` provides the ability to enhance performance through compile-time evaluations. Mastery of these concepts will empower developers to write more efficient and robust C++ code.
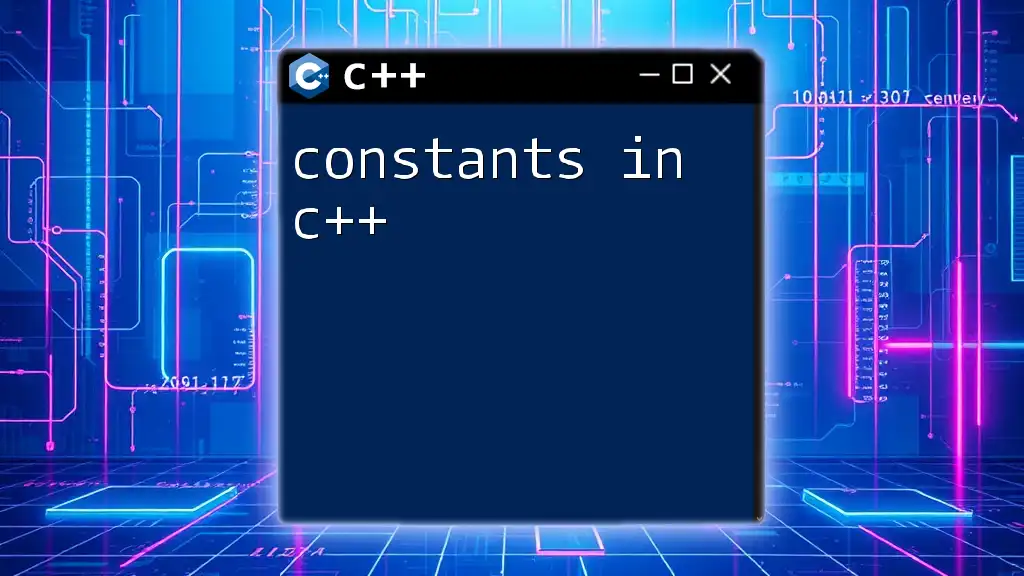
Further Reading and Resources
For deeper insights, consider exploring the C++ standards, engaging in advanced C++ programming books, or accessing tutorials tailored to improving your understanding of these constant keyword utilizations in C++.