In C++, `constexpr` is used to define a constant expression that can be evaluated at compile time, allowing for the use of strings in a context where their values need to be known during compilation.
#include <iostream>
constexpr const char* greet() {
return "Hello, constexpr!";
}
int main() {
std::cout << greet() << std::endl; // Output: Hello, constexpr!
return 0;
}
Understanding `constexpr`
In C++, `constexpr` is a specifier that allows the evaluation of functions and variables at compile time. This feature is particularly useful for optimization purposes, as it can significantly enhance performance by conducting computations before the program even runs. These compile-time evaluations allow developers to leverage the power of the C++ compiler in a way that reduces runtime overhead.
Differences between `constexpr` and `const`
While both `const` and `constexpr` are used to define constants, they serve different purposes. A `const` variable can only be initialized with a value at runtime, whereas `constexpr` variables must have their values known at compile time. For instance, any expression assigned to a `constexpr` variable should be evaluable by the compiler.
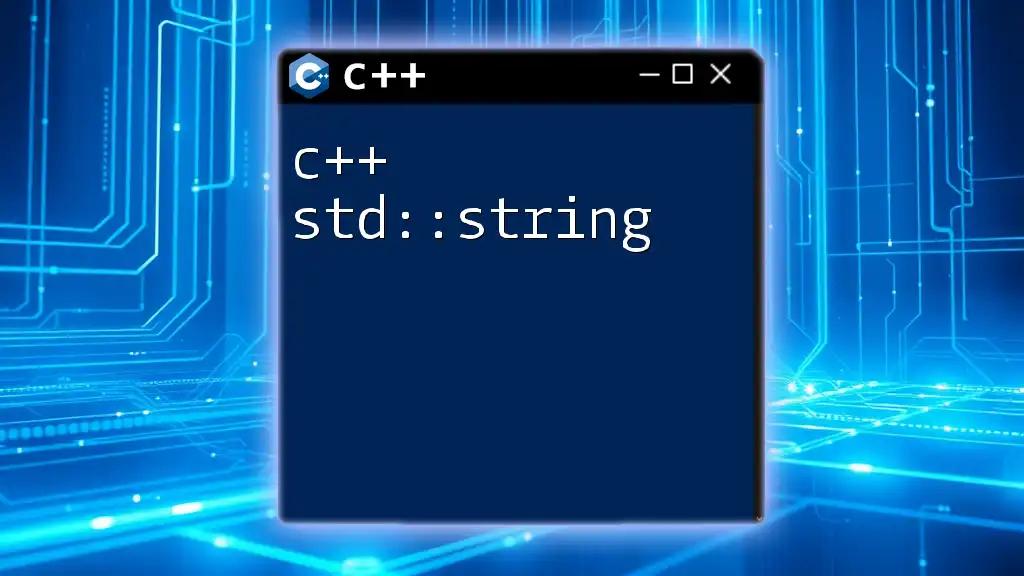
What is `constexpr` String?
A `constexpr` string is essentially a string literal that can be determined at compile time. Unlike regular strings, which are evaluated at runtime, `constexpr` strings can be used in contexts that require compile-time constants, such as template parameters and function arguments.
Key characteristics of `constexpr` strings
- Immutable: The contents of a `constexpr` string cannot be modified.
- Compile-time constructs: Operations utilizing `constexpr` strings are evaluated during compilation, leading to better performance.
Benefits of Using `constexpr` Strings
The primary advantage of `constexpr` strings lies in their compile-time optimizations. They enhance memory efficiency since they can be resolved during compilation, reducing allocation overhead during execution. Additionally, they improve the readability and maintainability of code, as developers can specify values that are meant to be constant, aiding in clearer documentation of program structure.
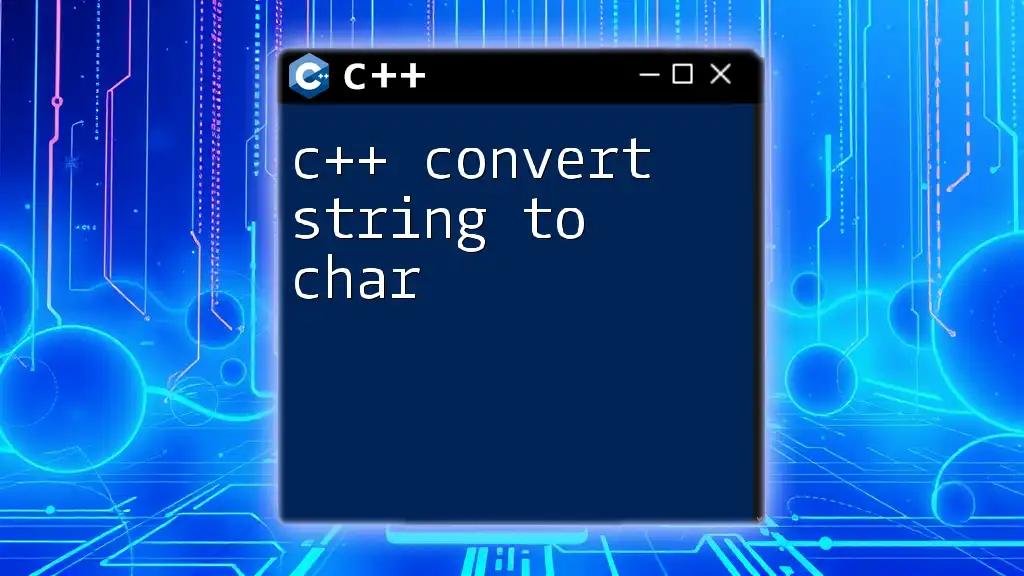
How to Create `constexpr` Strings
Basic Syntax for `constexpr` Strings
To create a `constexpr` string, one can simply use a string literal assigned to a `constexpr` variable. Here’s an example of basic declaration:
constexpr const char* myString = "Hello, constexpr!";
In this case, the string "Hello, constexpr!" will be known at compile time, marking it as a fixed value throughout the program.
Creating `constexpr` String Containers
Using `std::array` for Strings
`std::array` can be employed to define `constexpr` strings where the size must be known at compile time. Consider the following code snippet:
#include <array>
constexpr std::array<char, 13> myArray = {'H', 'e', 'l', 'l', 'o', ',', ' ', 'c', 'o', 'n', 's', 't', 'r'};
In this example, `myArray` is a compile-time constant array of characters, effectively creating a string-like structure. Each element of the array is available during compilation, making it possible to leverage these characters in a compile-time context.
Creating Custom `constexpr` String Class
In scenarios where a more flexible or feature-rich implementation is desired, creating a custom string class can be advantageous. A simple `constexpr` string class might resemble the following:
template<std::size_t N>
class ConstexprString {
public:
constexpr ConstexprString(const char(&arr)[N]) {
for (std::size_t i = 0; i < N; ++i) {
value[i] = arr[i];
}
}
constexpr const char* data() const { return value; }
private:
char value[N];
};
This class wraps a character array and provides compile-time initialization, enabling the use of `ConstexprString` in a manner similar to built-in string types.
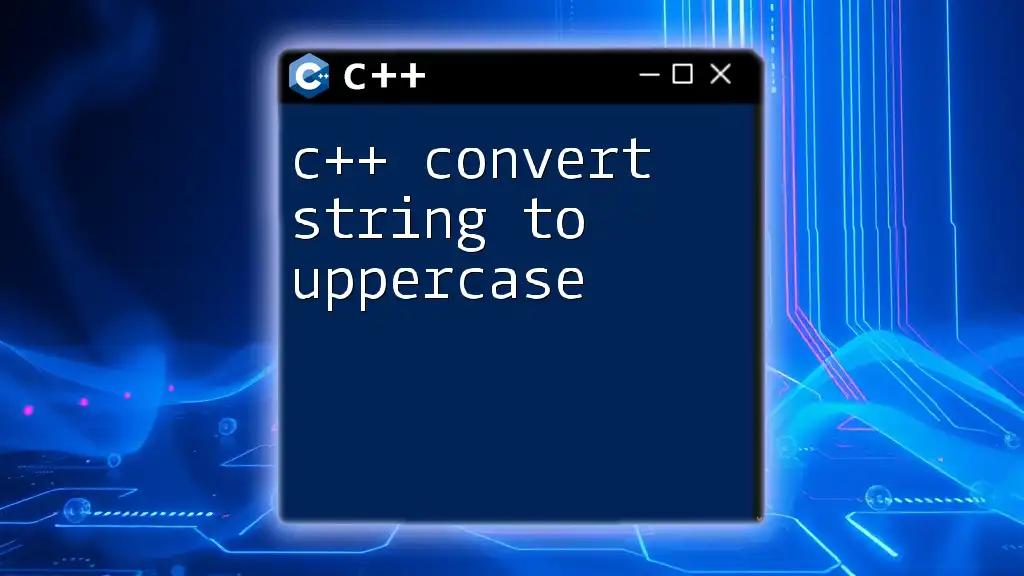
Manipulating `constexpr` Strings
Concatenation of `constexpr` Strings
String concatenation is a common operation, but it can be tricky with `constexpr` strings. Here's an incorrect example:
constexpr const char* str1 = "Hello, ";
constexpr const char* str2 = "World!";
constexpr const char* combined = str1 + str2; // Incorrect usage
The problem arises because the C++ language does not inherently support compile-time concatenation of string literals through pointer arithmetic. Instead, we can define a `constexpr` function to handle concatenation during compile time.
Common String Operations with `constexpr`
Finding Length of a `constexpr` String
One frequent requirement is determining the length of a `constexpr` string. We can achieve this with a recursive function, as shown below:
constexpr size_t constexpr_strlen(const char* str) {
return *str ? 1 + constexpr_strlen(str + 1) : 0;
}
This function recursively computes the length of the string until it encounters the null terminator.
String Comparison at Compile Time
When working with `constexpr` strings, it’s often necessary to compare them. The following example demonstrates how to compare two `constexpr` strings:
constexpr bool constexpr_compare(const char* str1, const char* str2) {
return (*str1 == *str2 && (*str1 == '\0' || constexpr_compare(str1 + 1, str2 + 1)));
}
This function checks whether two strings are equal by recursively comparing their characters.

Use Cases for `constexpr` Strings
Application in Template Programming
One powerful application of `constexpr` strings is within template programming. Templates often require compile-time constants, and `constexpr` strings fit perfectly into this paradigm, enabling complex behaviors based on string contents. This can include generating functions and type traits that require string manipulations at compile time.
Using `constexpr` Strings in a Real-World Scenario
Consider a case in which we define a logging system. The log messages can be `constexpr` strings, enabling assurance that the log format is consistent and determined at compile time:
constexpr const char* logMessage = "Error occurred in module %s";
Here, the log message is readily available during compilation, ensuring that any logging system built around it functions without run-time overhead.

Best Practices and Limitations
Best Practices for Optimizing `constexpr` Strings
To maximize the utility of `constexpr` strings, adhere to the following best practices:
- Prefer `std::array` for fixed-size strings: This ensures bounds checking and easy manipulation.
- Leverage recursive functions for operations: As demonstrated, recursion aids in compiling string operations safely.
- Keep strings immutable: Avoid unnecessary modifications to preserve `constexpr` integrity.
Limitations and Considerations of `constexpr` Strings
Despite their benefits, it’s important to be aware of limitations. `constexpr` strings must be known at compile time, which implies that they cannot dynamically change or hold variable values post-execution. This makes them less suitable for scenarios demanding dynamic content representation. It’s crucial to evaluate the context in which you wish to use `constexpr` strings to determine if a different approach may yield better results.
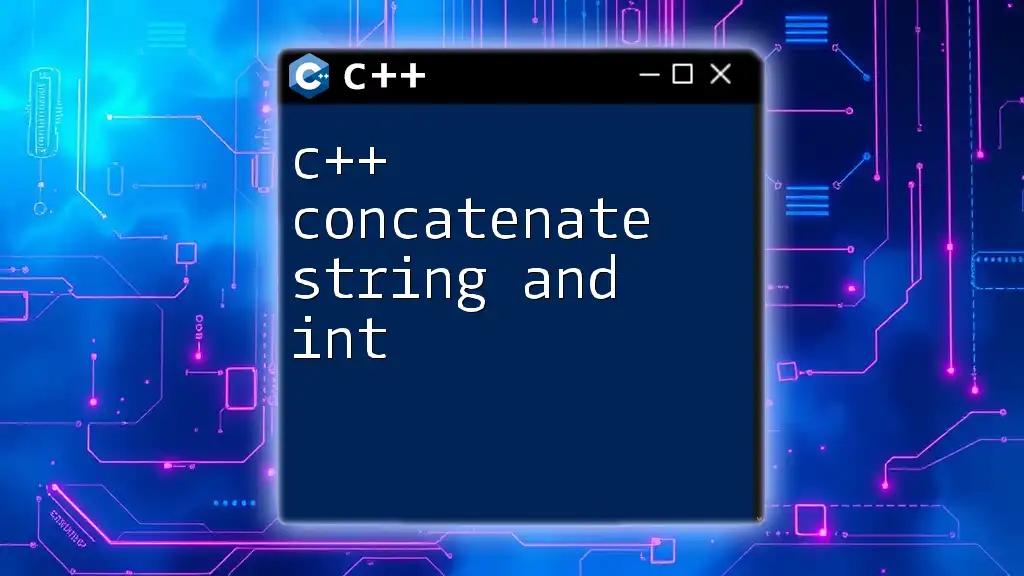
Conclusion
In summary, `constexpr` strings in C++ offer a powerful means of enhancing performance through compile-time evaluation. Their immutability and efficiency facilitate cleaner, more maintainable code, enabling developers to take full advantage of modern C++ features. By understanding their creation, manipulation, and practical applications, you can integrate `constexpr` strings effectively into your programming toolkit. Continue exploring and practicing these concepts to become more proficient in using `constexpr` strings in your C++ projects.