In C++, you can convert a string to uppercase using the `std::transform` function along with the `toupper` function from the `<algorithm>` and `<cctype>` libraries.
Here’s a code snippet demonstrating this:
#include <iostream>
#include <string>
#include <algorithm>
#include <cctype>
int main() {
std::string str = "hello world";
std::transform(str.begin(), str.end(), str.begin(), [](unsigned char c){ return std::toupper(c); });
std::cout << str << std::endl; // Outputs: HELLO WORLD
return 0;
}
Understanding C++ String Types
What is a C++ String?
In C++, a string is an object of the `std::string` class, which is a part of the Standard Library. This class provides a flexible way to handle sequences of characters and offers many useful operations for string manipulation. Unlike C-style strings, which are simply arrays of characters ending with a null terminator (`'\0'`), `std::string` manages memory automatically, allowing for dynamic resizing and easier manipulation.
Different Types of Strings in C++
When working in C++, it is essential to understand the distinction between different string types:
-
C-Style Strings: These are simple character arrays and do not encapsulate many of the features or conveniences provided by `std::string`. C-strings are prone to manipulation errors, such as buffer overflows, due to the lack of bounds checking.
-
std::string: This modern approach to strings offers various functionalities, including:
- Dynamic sizing
- Built-in functions for searching, modifying, and comparing strings
- Ease of use with concatenation and formatted output
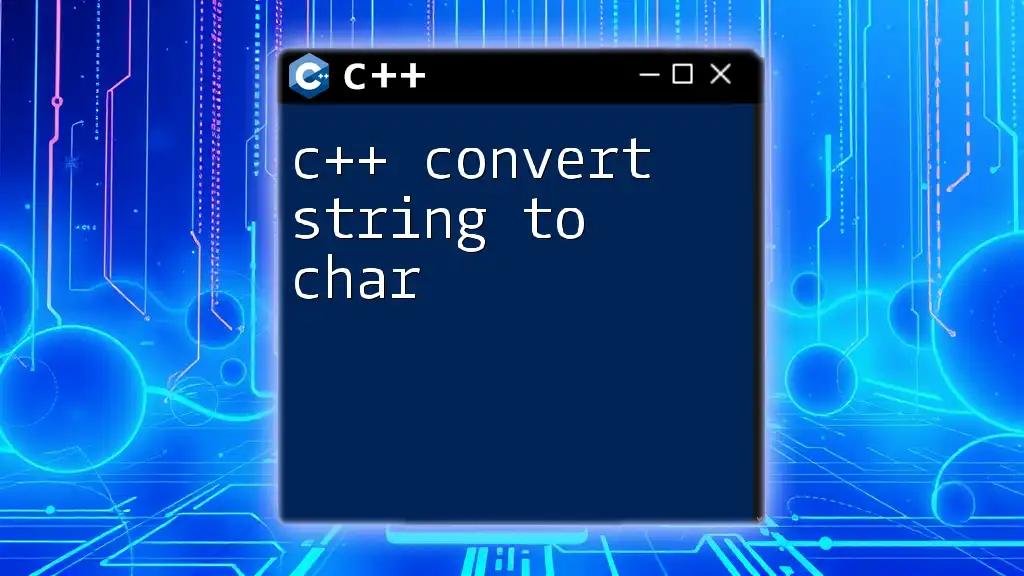
The Importance of String Case Conversion
String case conversion is more than just a stylistic choice; it serves practical purposes in programming. For instance, many applications require consistent input formatting, particularly when evaluating user inputs or dealing with database queries. Here are some contexts where converting a string to uppercase is essential:
- Data Normalization: This involves converting strings to a common case to ensure uniformity, thereby improving the accuracy of comparisons.
- User Interfaces: Presenting outputs in uppercase can enhance readability and make the data more visually appealing.

C++ Functions for String Uppercase Conversion
Using the `toupper` Function
The `toupper` function is a fundamental part of the `<cctype>` header and is used primarily to convert a single character to its uppercase counterpart. Its syntax is straightforward:
int toupper(int ch);
Example of using the `toupper` function to convert characters in a string to uppercase:
#include <iostream>
#include <string>
#include <cctype>
std::string convertToUpper(std::string str) {
for (char &c : str) {
c = toupper(c);
}
return str;
}
// Example usage
std::string myString = "Hello, World!";
std::cout << convertToUpper(myString) << std::endl; // Output: HELLO, WORLD!
In this example, we iterate through each character of the input string, applying the `toupper` function.
Converting a C++ String to Uppercase
Using a Loop
You can convert a C++ string to uppercase using a simple loop, as seen in the previous example. The loop's straightforward nature makes it an excellent choice for beginners wanting to grasp string manipulation basics.
Using `std::transform`
For a more compact and efficient solution, leveraging the `std::transform` function from the `<algorithm>` header is highly effective. This method is preferred for its clarity and conciseness.
Here’s how you can implement it:
#include <algorithm>
#include <iterator>
std::string convertToUpper(std::string str) {
std::transform(str.begin(), str.end(), str.begin(), ::toupper);
return str;
}
// Example usage
std::cout << convertToUpper("hello world") << std::endl; // Output: HELLO WORLD
With `std::transform`, you provide a range defined by the string’s begin and end iterators, applying `toupper` to each character in the specified range. This approach is cleaner and takes advantage of the power of STL algorithms.
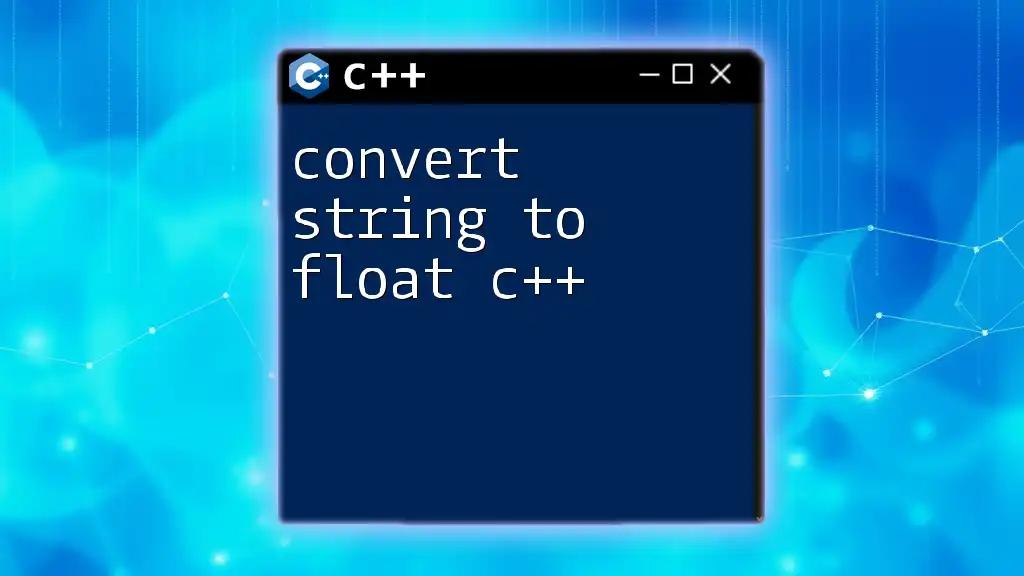
Additional Techniques for String to Uppercase Conversion
Using Custom Functions
When developing more complex applications, you might want to implement tailored functions for uppercase conversion. Customization is important for specific scenarios, such as logging or conditionally converting strings.
Here’s a simple example of a function that logs the conversion process:
void convertAndLog(const std::string &input) {
std::string upperString = convertToUpper(input);
std::cout << "Converted String: " << upperString << std::endl;
}
Using Lambda Functions
For a more modern approach, consider using lambda functions. They allow for concise, inline function definitions without the need for named functions.
Here’s an example of using a lambda to convert a string:
auto convertToUpperLambda = [](std::string str) {
std::transform(str.begin(), str.end(), str.begin(), ::toupper);
return str;
};
Lambda functions can enhance the readability of your code while providing the same functionality as traditional functions.
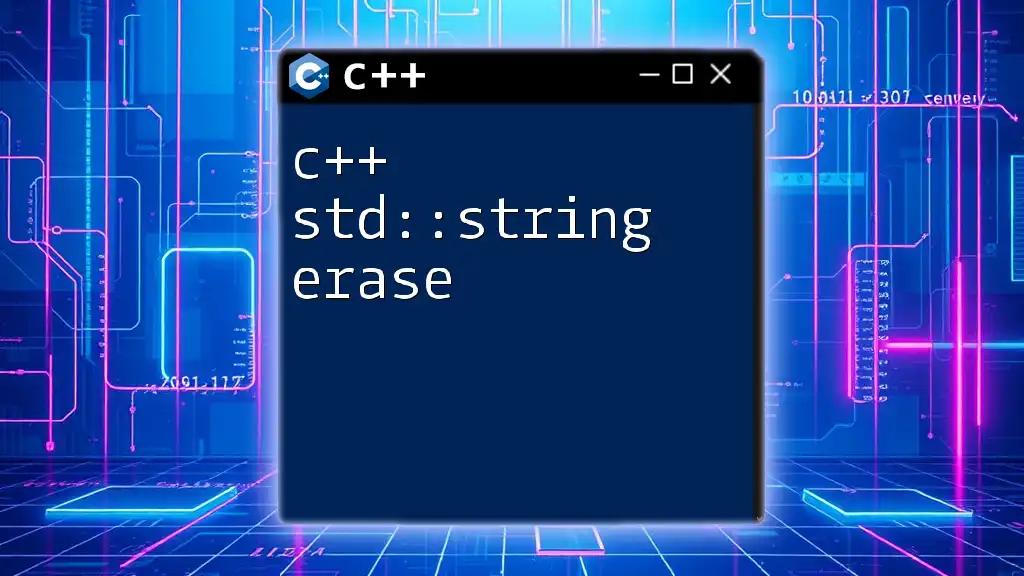
Performance Considerations
While manipulating strings, especially large datasets, performance can become a concern. Here are some key points to consider:
- String Resizing: Frequent resizing of strings can lead to performance bottlenecks. Using `std::string` avoids this issue but be mindful of how often you modify strings.
- Algorithm Efficiency: Using `std::transform` is often more efficient than manual loops. Always prefer STL functions whenever possible for better performance and readability.
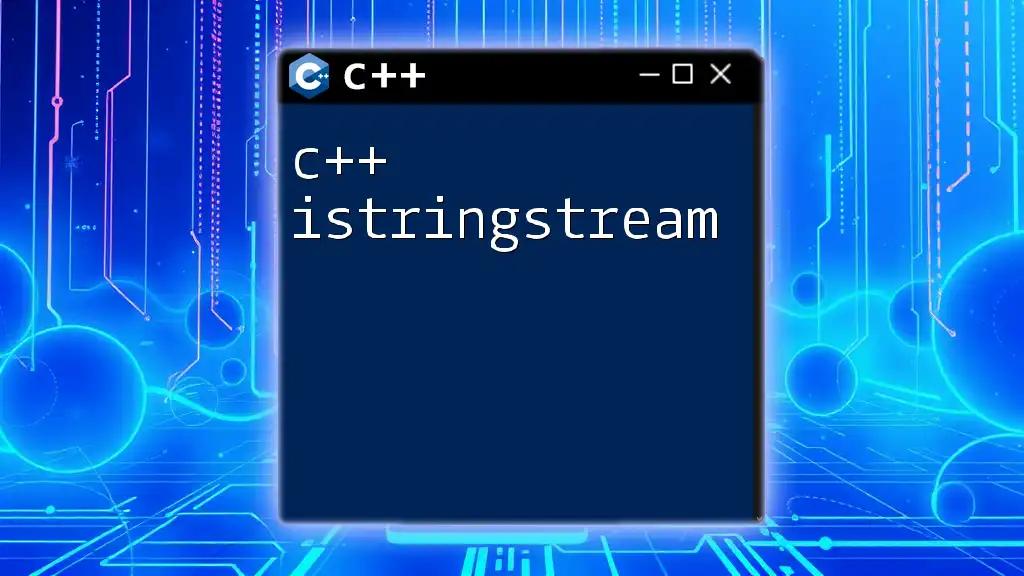
Real-World Applications
Understanding how to convert strings to uppercase in C++ has practical applications:
- Data Validation: When handling user inputs, converting inputs to uppercase can ensure consistent comparisons without case sensitivity issues.
- Database Queries: Normalizing string inputs before querying databases improves data integrity and accuracy.
- User Interfaces: Outputting data in uppercase can elevate the overall user experience, making applications more intuitive.
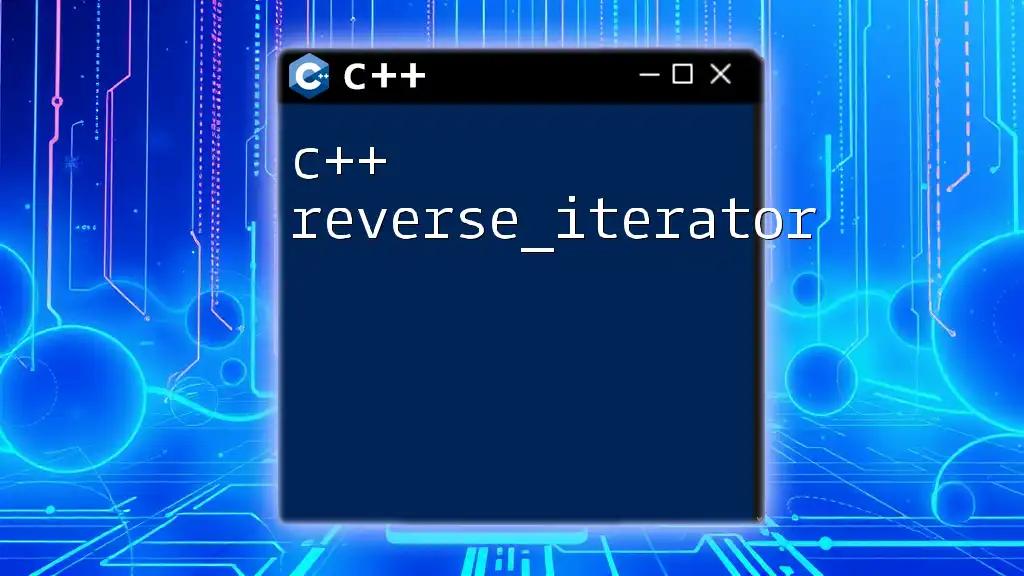
Conclusion
Converting strings to uppercase in C++ is an essential skill that enhances your ability to manage and manipulate text data effectively. By mastering various techniques, such as using `toupper`, loops, `std::transform`, and lambda functions, you can efficiently ensure consistency and readability in your applications.
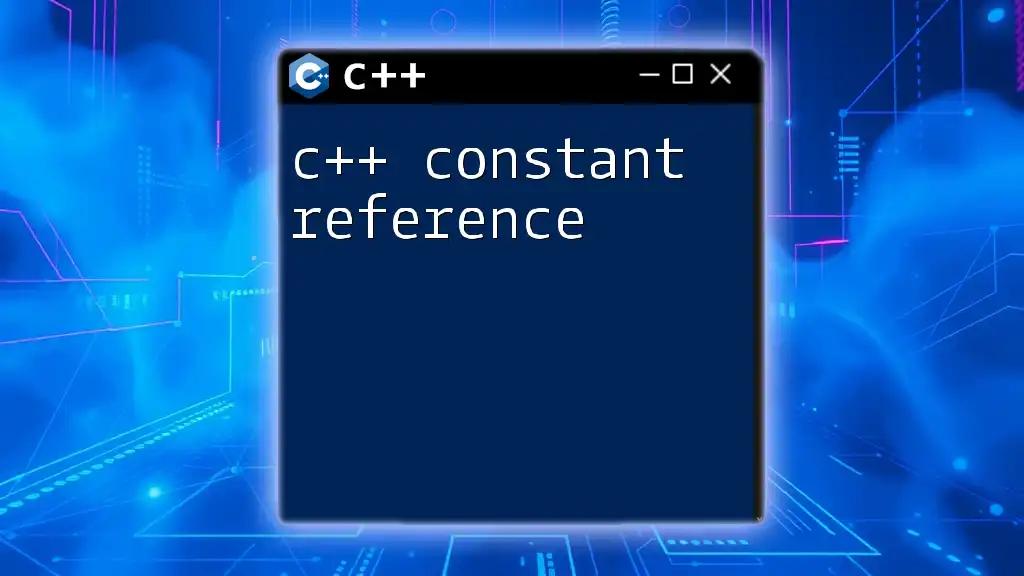
Further Learning Resources
For those interested in expanding their knowledge of string manipulation in C++, consider exploring the following resources:
- Books focusing on C++ programming, such as "C++ Primer" and "Effective C++".
- Online courses and tutorials on C++ Standard Library and string manipulation techniques.
- Official C++ documentation for `std::string` and associated functions.

Examples and Exercises
As a great way to solidify your understanding, try implementing your own string conversion functions. Challenge yourself to create a case-insensitive comparison function that utilizes the concepts discussed.
By practicing these methods, you'll become confident in applying string manipulation techniques across your C++ projects.