To convert a string to a double in C++, you can use the `std::stod` function from the `<string>` library, which parses the string and returns the corresponding double value.
#include <iostream>
#include <string>
int main() {
std::string str = "3.14";
double value = std::stod(str);
std::cout << "The double value is: " << value << std::endl;
return 0;
}
Understanding the Basics of Data Types
What is a String in C++?
In C++, a string is a sequence of characters used to represent text. Strings are incredibly versatile and can be declared using the `std::string` class from the Standard Library.
For example:
#include <string>
std::string name = "John Doe";
std::string greeting = "Hello, " + name + "!";
Common operations on strings include concatenation, length calculation, and searching for substrings, making them essential for handling textual data effectively.
What is a Double in C++?
A double in C++ is a data type used for representing floating-point numbers with double precision. This provides a greater range and accuracy compared to the `float` type. A double can hold large values and is especially useful in scientific calculations and financial applications where precision is crucial.
double pi = 3.141592653589793;
double e = 2.718281828459045;
The distinction between `float` and `double` lies primarily in their precision and range, with `double` allowing for a more extensive set of decimal values.
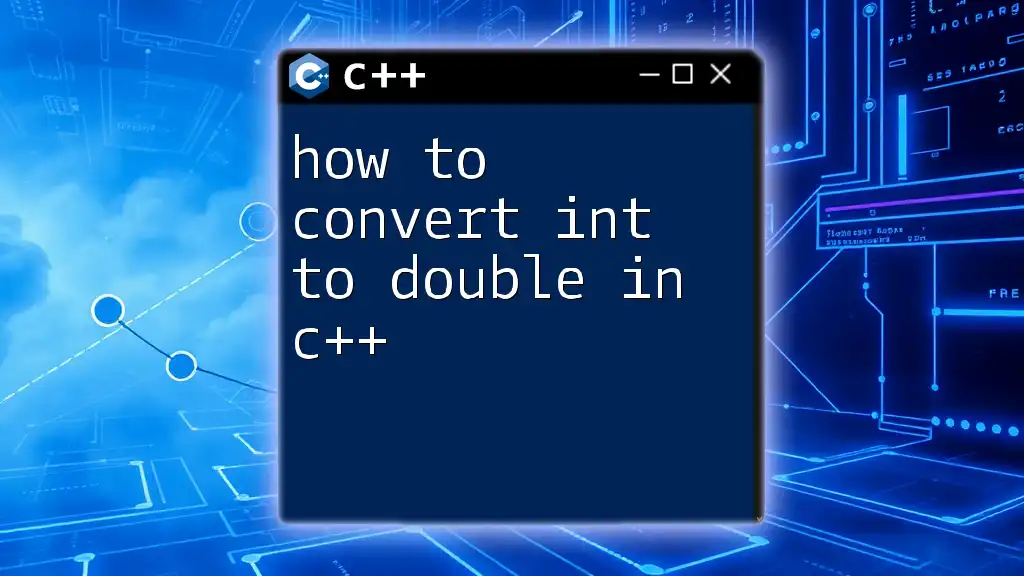
Conversion Fundamentals
Why Convert String to Double in C++?
Converting a string to a double is often necessary when dealing with user inputs or data parsed from files and when the program requires numerical computations.
For example, if a user inputs data in text format like "3.14", you may need to convert this input for mathematical calculations where a double representation is expected.
C++ String to Double Conversion Methods Overview
C++ provides several ways to convert a string to a double. Each method has its advantages and limitations:
- `std::stod`: A straightforward and modern approach that handles exceptions well.
- `stringstream`: An older method that provides flexibility and is useful for complex parsing tasks.
- C-style functions (`atof`): Quick and simple but lack robustness and safety.
- Custom parsing functions: Adaptable for specific needs but requires more code.
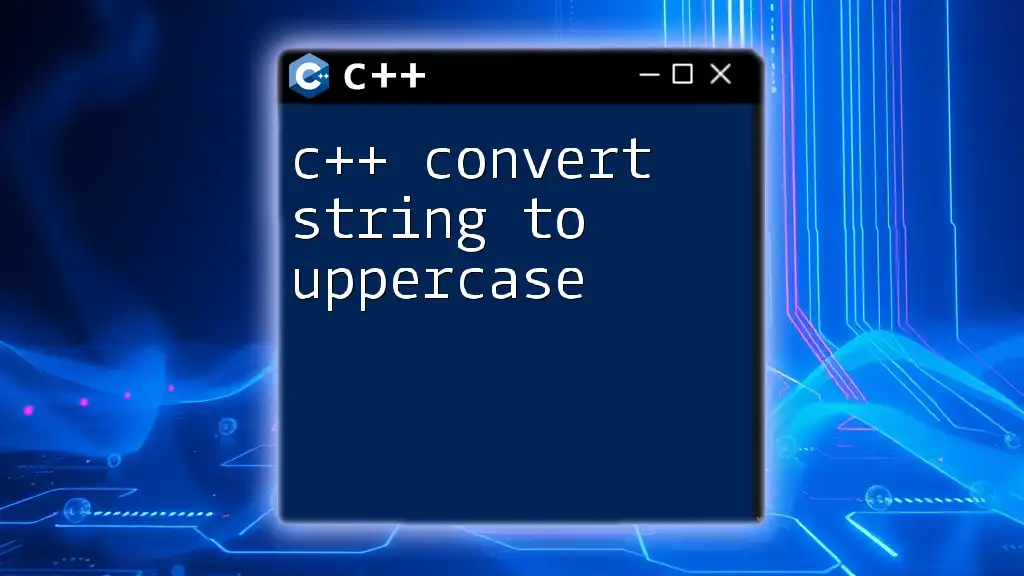
Using `std::stod` for String to Double Conversion
Introduction to `std::stod`
The function `std::stod` is a part of the Standard Library and is specifically designed to convert a string to a double. Its syntax is straightforward:
double std::stod(const std::string& str, std::size_t* pos = 0);
Example of Using `std::stod`
Here's how you can use `std::stod` to convert a string to a double:
#include <iostream>
#include <string>
int main() {
std::string str = "3.14159";
try {
double num = std::stod(str);
std::cout << "Converted string to double: " << num << std::endl;
} catch (const std::invalid_argument& e) {
std::cerr << "Invalid argument: " << e.what() << std::endl;
} catch (const std::out_of_range& e) {
std::cerr << "Out of range: " << e.what() << std::endl;
}
return 0;
}
In this code, we convert the string "3.14159" to a double. We use a `try-catch` block to expertly handle any potential conversion errors, such as passing an invalid string.
Limitations of `std::stod`
While `std::stod` is convenient, it may not be suitable in all cases. It can throw exceptions that need to be handled, making it slightly complex for scenarios where performance is crucial or when error handling may not be feasible.
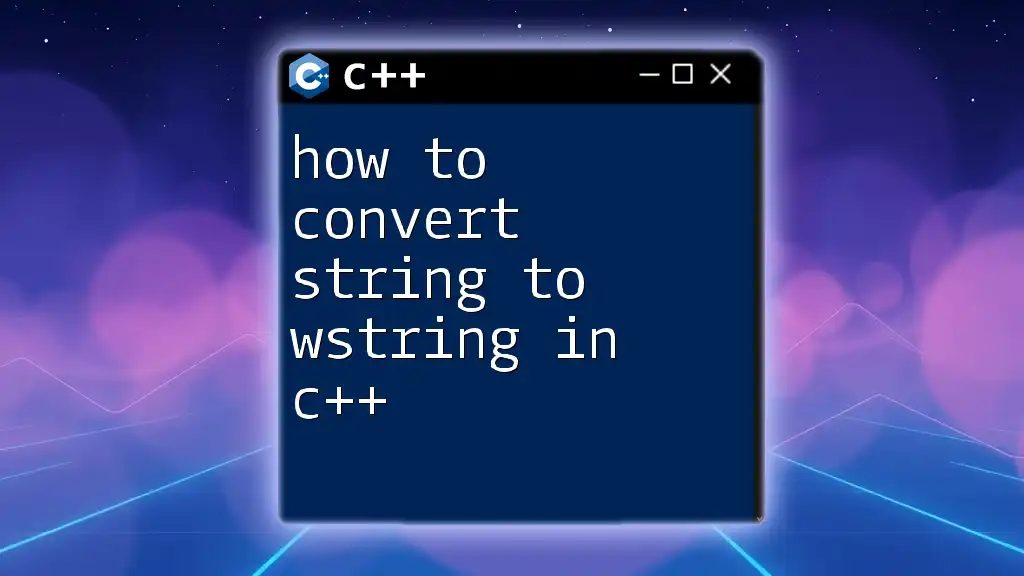
Alternative Method: Using `stringstream`
Introduction to `stringstream`
The `stringstream` class allows for versatile input/output operations on strings, making it a useful tool for converting strings to various data types, including double.
Example of Using `stringstream`
Here's an example of using `stringstream` to convert a string to a double:
#include <iostream>
#include <string>
#include <sstream>
int main() {
std::string str = "2.71828";
std::stringstream ss(str);
double num;
ss >> num;
if (ss.fail()) {
std::cerr << "Conversion failed: input string may be invalid." << std::endl;
} else {
std::cout << "Converted string to double using stringstream: " << num << std::endl;
}
return 0;
}
In this snippet, we read from the `stringstream` and check if the operation succeeded by using `ss.fail()`. The `stringstream` method excels in cases where conversion formats may vary or additional processing is needed.
Error Handling with `stringstream`
To handle errors effectively with `stringstream`, it’s crucial to check whether the stream state is valid after the conversion. This can save you from potential crashes and ensure reliability in your applications.
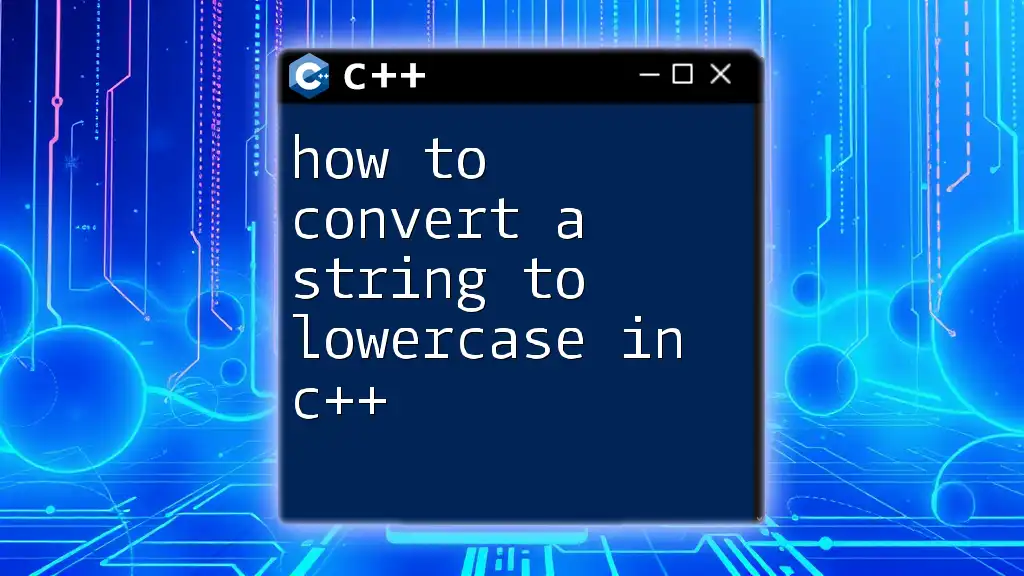
Other Methods for String to Double Conversion
Using C-style Casting
In simple scenarios, you might consider using `atof`, which is a C-style function for converting strings to doubles:
#include <iostream>
#include <cstdlib>
int main() {
const char* str = "1.41421";
double num = atof(str);
std::cout << "Converted string to double using atof: " << num << std::endl;
return 0;
}
While `atof` is quick and easy to use, it lacks built-in error handling. Thus, if the conversion fails, it will return 0. Therefore, using `atof` can lead to mistakes if 0 is a valid output from your string.
Using Custom Parsing Functions
If the built-in methods don't meet your requirements, you can create a custom string-to-double parser that accommodates specific formats or validation:
#include <iostream>
#include <string>
double customParseDouble(const std::string& str) {
// Simplified parsing logic
// This example can be refined for production code to enhance robustness
try {
return std::stod(str);
} catch (...) {
std::cerr << "Invalid string for conversion: " << str << std::endl;
return 0.0; // Return a default value or handle as needed
}
}
int main() {
std::string numberStr = "0.57721";
std::cout << "Custom parsed double: " << customParseDouble(numberStr) << std::endl;
return 0;
}
This custom parser incorporates error handling, making it more resilient to input variations while allowing for enhancements according to your needs.
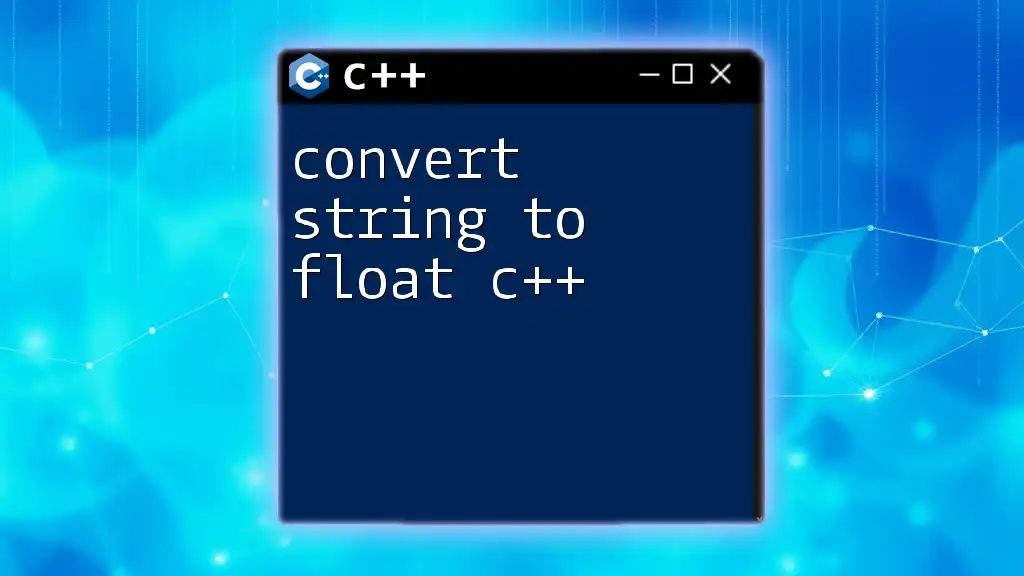
Best Practices for String to Double Conversion
Input Validation
Before attempting any string to double conversion, it is crucial to input validate the strings. If the string contains non-numeric characters, skips, or is empty, avoid conversion to prevent runtime errors. Examples of validation checks include:
- Checking if the string is empty.
- Ensuring all characters are digits or valid decimal points.
- Stripping out unwanted whitespaces.
Exception Handling
When using methods like `std::stod`, always implement exception handling using `try-catch` blocks. This allows your program to respond gracefully to conversion failures without crashing unexpectedly.
Performance Considerations
For high-performance applications, consider the method that best fits your needs. While `std::stod` offers safety, `stringstream` can be beneficial for parsing complex strings. Balancing safety and performance is key to writing efficient C++ code.
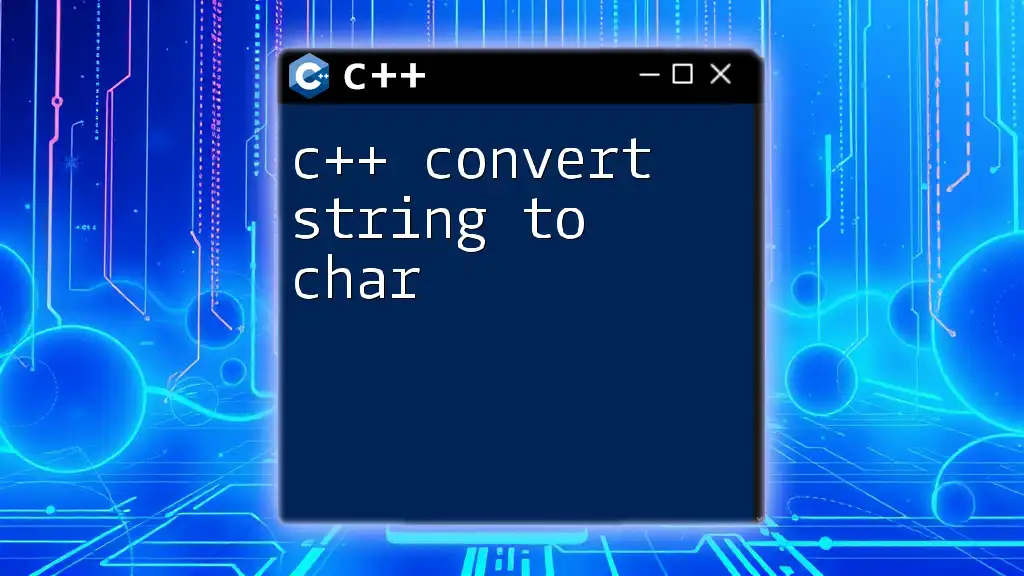
Conclusion
By understanding various methods for how to convert string to double in C++, ranging from `std::stod` to `stringstream`, you can choose the method that best fits your application needs. Each method has its strengths and ideal use cases, making it essential to evaluate them in the context of your particular problem.
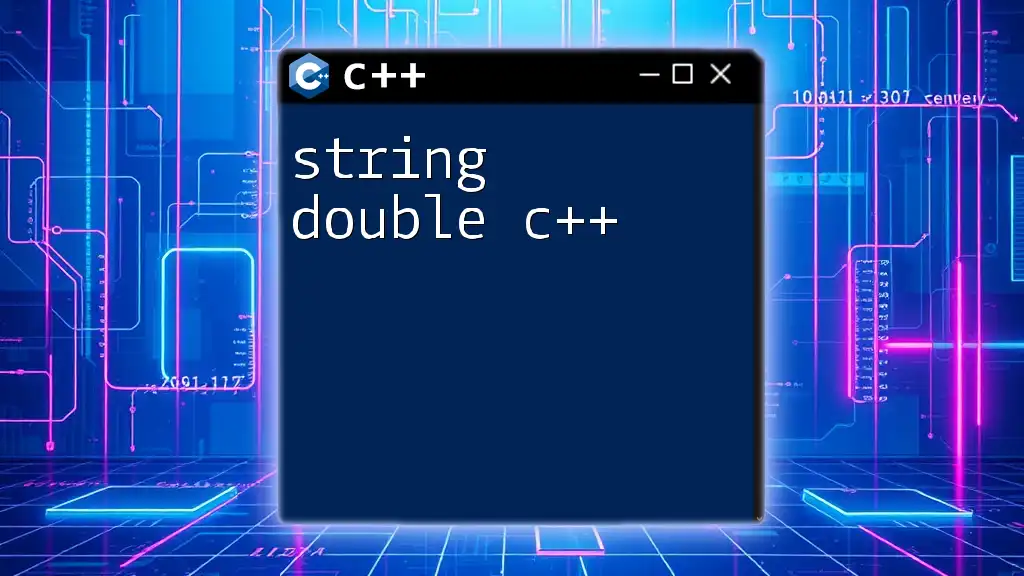
Additional Resources
To further enhance your C++ skills and deepen your knowledge of data conversions, explore recommended books, online courses, and documentation. Engaging with community forums and discussions can also provide invaluable insights and practical experiences shared by fellow programmers.