To convert a `char` to a `string` in C++, you can use the `std::string` constructor, as shown in the following code snippet:
char myChar = 'A';
std::string myString(1, myChar); // Convert char to string
Understanding Char and String in C++
What is a Char in C++?
In C++, a char data type represents a single character. It occupies 1 byte of memory and can be used to store letters, digits, and special symbols. Each character is enclosed in single quotes, like `'A'`, `'1'`, or `'#'`.
For instance:
char c = 'A';
This statement declares a character variable `c` and initializes it with the value `A`.
What is a String in C++?
A string in C++ is typically represented using the `std::string` class, which is part of the C++ Standard Library. Unlike a character, which is a single byte, a string can hold a sequence of characters and dynamically manage memory. Strings are usually enclosed in double quotes, such as `"Hello, World!"`.
The `std::string` class provides numerous functionalities for string manipulation, such as concatenation, comparison, and searching, which makes it more versatile than character arrays.
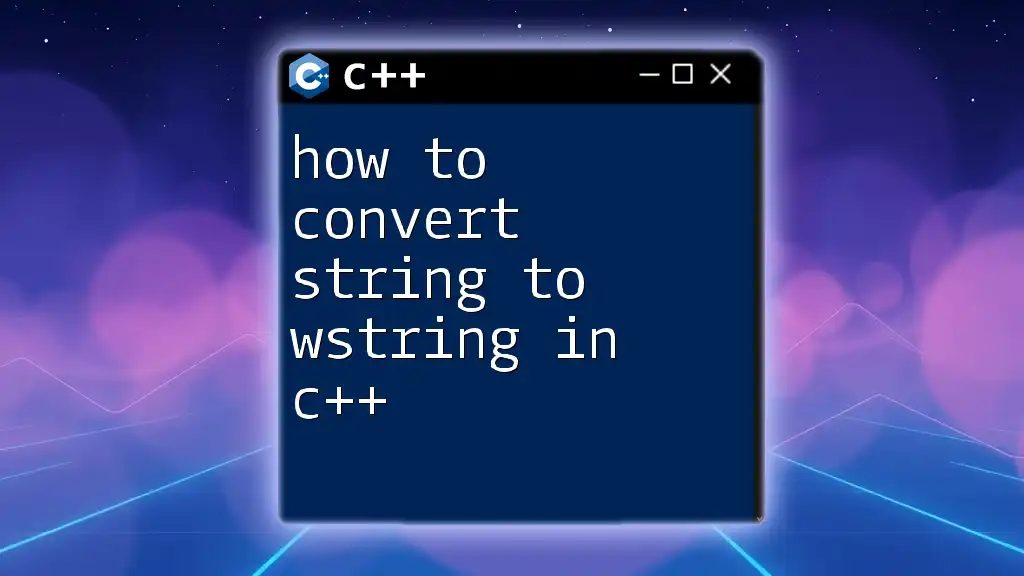
Why Convert Char to String in C++?
Understanding how to convert char to string in C++ is crucial in various situations, such as:
- When concatenating characters with other strings.
- Formatting output where string representations are required.
- Ensuring compatibility with functions or methods expecting string inputs.
The ability to convert a `char` to a `std::string` can provide better readability and improved performance in string handling.
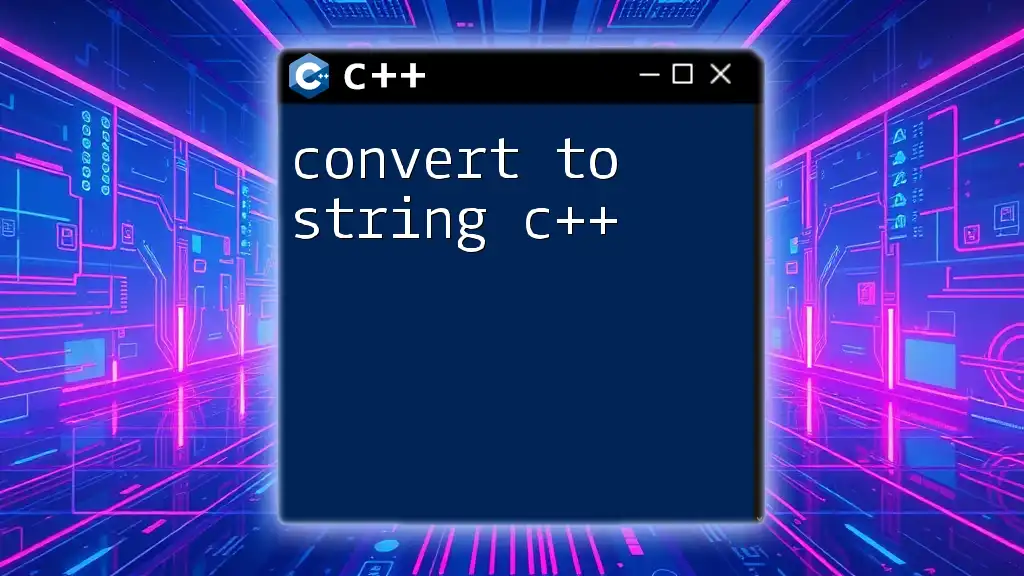
Methods to Convert Char to String in C++
Using the String Constructor
One straightforward method for converting a char to a string is to utilize the `std::string` constructor that accepts two arguments: the count of characters and the character itself.
Consider the following code snippet:
char c = 'A';
std::string str(1, c); // Convert char to string
In this example, the `std::string` constructor creates a new string containing one occurrence of the character `c`. This method is concise and efficient.
Using String Assignment
Another simple approach is to assign a char directly to a string variable. This method allows for a clear and understandable conversion.
Here's a code example:
char c = 'B';
std::string str;
str = c; // Convert char to string
In this code, the character `B` is directly assigned to the string variable `str`. The implicit conversion from `char` to `std::string` occurs seamlessly.
Using the `std::to_string` Function
While primarily designed for numeric types, you can creatively use `std::to_string` in conjunction with string manipulation techniques.
For example, consider this code:
char c = 'C';
std::string str = std::string(1, c); // Alternative method using to_string indirectly
The above snippet demonstrates another use of the `std::string` constructor to provide an alternative to directly creating a string from a char. While this method may add some complexity, it's a valid solution.
Using String Concatenation
One can also convert a `char` to `std::string` by concatenating it with an empty string. This method is simple and effective.
Here's how you might do it:
char c = 'D';
std::string str = "" + std::string(1, c); // Concatenation method
In this example, concatenating an empty string with the one-character string created from `c` effectively converts `char` to `std::string`.
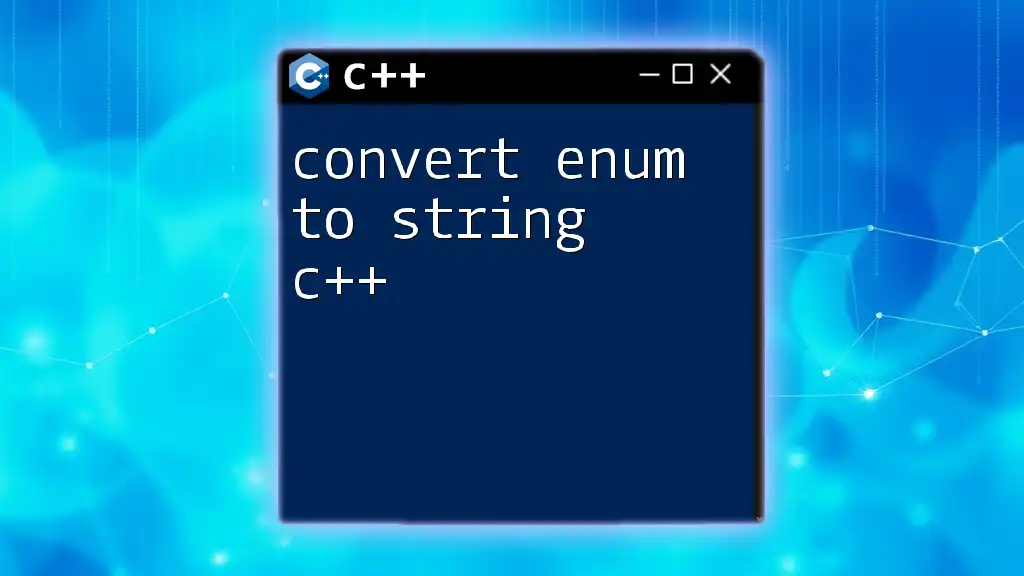
Best Practices for Char to String Conversion
Avoiding Common Pitfalls
When converting `char` to `string`, it is crucial to be mindful of issues like null termination in C-style strings. Unlike `std::string`, a character array may have undefined behavior if accessed improperly, particularly when manipulating large strings or dealing with special characters. Always ensure that string lengths and boundaries are handled carefully.
When to Use Each Method
Choosing the appropriate conversion method can depend on specific requirements:
- String Constructor: Best for clarity and performance when dealing with a directly known character.
- String Assignment: Ideal for simpler code, especially if you already have a char variable ready.
- Concatenation: Effective when you want to combine multiple characters or strings dynamically.
- Indirect Use of std::to_string: Mostly for numeric conversions; not the preferred method for characters.
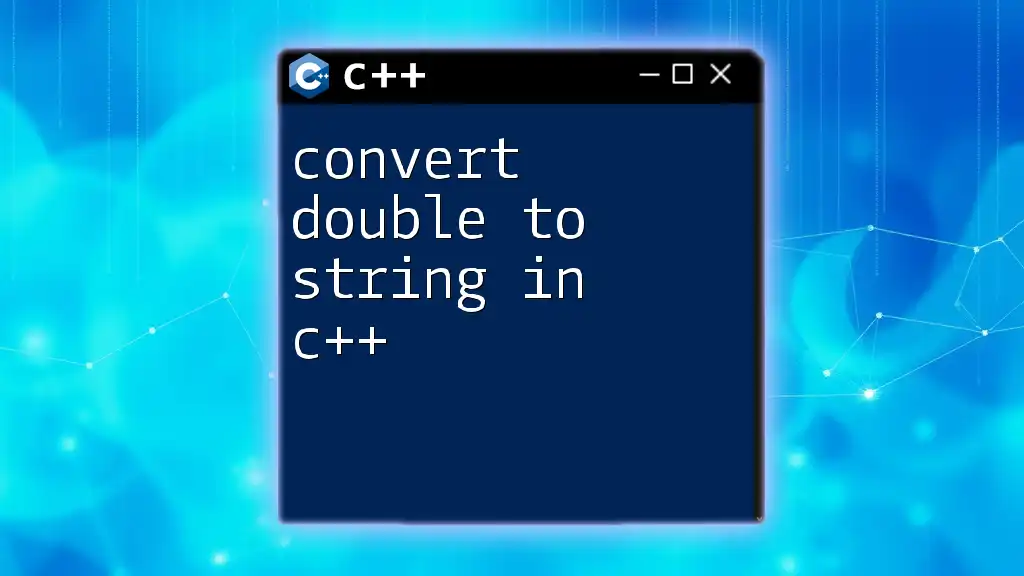
Conclusion
In summary, knowing how to convert char to string in C++ is an essential skill for anyone working with character data types. The four primary methods demonstrated—using the string constructor, string assignment, the `std::to_string` function, and concatenation—all provide viable solutions for this conversion.
Experimenting with each method in your C++ coding projects will deepen your understanding and help you find the most suitable way to handle character and string data effectively.