To convert a string to lowercase in C++, you can use the `std::transform` function along with `std::tolower` from the `<algorithm>` and `<cctype>` libraries.
#include <algorithm>
#include <cctype>
#include <string>
std::string toLowerCase(const std::string& str) {
std::string lowerStr = str;
std::transform(lowerStr.begin(), lowerStr.end(), lowerStr.begin(), ::tolower);
return lowerStr;
}
Understanding Strings in C++
What is a String in C++?
In C++, a string is a sequence of characters that can represent text. The standard library provides the `std::string` class to facilitate string manipulation. Unlike character arrays, `std::string` handles memory management automatically, allowing for easier concatenation, comparison, and modification.
Why Convert to Lowercase?
Converting a string to lowercase has several practical applications in programming. One common use case is when performing case-insensitive comparisons between strings. For instance, in user login systems, checking usernames and passwords without considering their case improves user experience. Additionally, data normalization often requires strings to be standardized to a single case, such as lower case, before database storage or analysis.
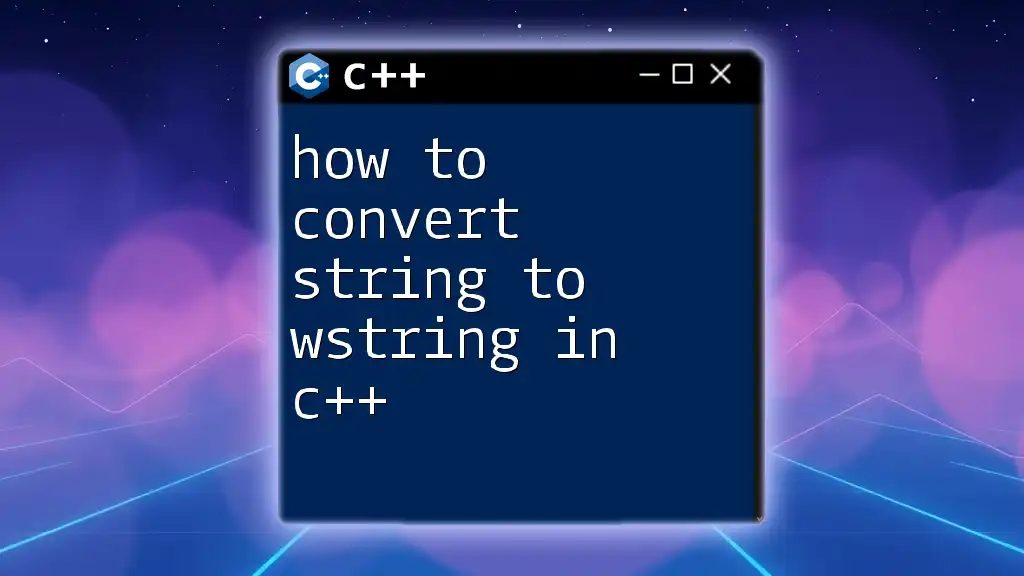
Methods to Make String Lowercase in C++
Using the `std::transform` Function
Overview of `std::transform`
The `std::transform` function, found in the `<algorithm>` header, is a powerful tool in C++ for applying a transformation to a range of elements. It can efficiently convert each character in a string to lowercase using the `::tolower` function.
Code Example
#include <iostream>
#include <string>
#include <algorithm>
int main() {
std::string str = "Hello World!";
std::transform(str.begin(), str.end(), str.begin(), ::tolower);
std::cout << str << std::endl; // Output: hello world!
return 0;
}
In this example, `std::transform` iterates over each character in the string `str`, applying the `tolower` function to it. The resultant lowercase string replaces the original `str`.
Using a Loop and the `tolower` Function
Manual Approach for Converting String to Lowercase
If you prefer an explicit approach, you can manually traverse the string and convert each character to lowercase using the `tolower` function from the `<cctype>` library.
Code Example
#include <iostream>
#include <string>
#include <cctype>
int main() {
std::string str = "Hello World!";
for (char& c : str) {
c = std::tolower(c);
}
std::cout << str << std::endl; // Output: hello world!
return 0;
}
In this example, a range-based for loop iterates over each character represented by the reference `c`. The `tolower` function is called for each character, thereby modifying the original string directly.
Utilizing C++11's `std::string::transform`
Advantages of Built-in Functions
Leveraging built-in functions like `std::transform` not only simplifies your code but also enhances performance. These functions are optimized and often more efficient than a manual implementation.
Code Example
#include <iostream>
#include <string>
#include <algorithm>
int main() {
std::string str = "Hello World!";
std::string lowerStr = str;
std::transform(lowerStr.begin(), lowerStr.end(), lowerStr.begin(), [](unsigned char c) { return std::tolower(c); });
std::cout << lowerStr << std::endl; // Output: hello world!
return 0;
}
In this code, a lambda function is passed to `std::transform`, ensuring that the `tolower` function is applied correctly to each character. This approach is beneficial as it ensures that the transformation is adaptable and concise.
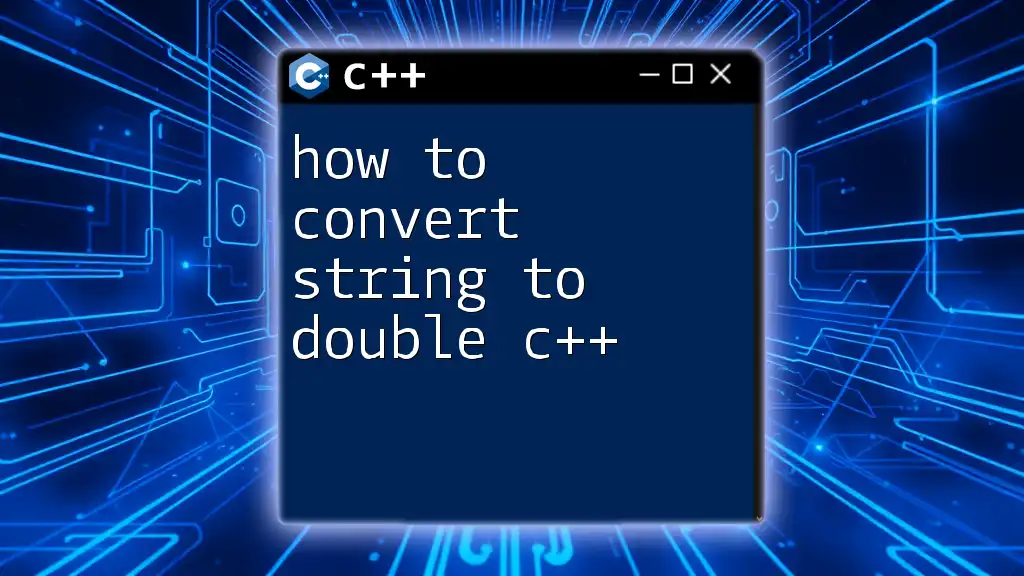
Best Practices for Handling Strings in C++
Efficient String Manipulation
When dealing with string conversions, be mindful of performance, especially in loops or larger datasets. Using references (like `char& c` rather than `char c`) minimizes unnecessary copying, improving efficiency. Additionally, consider using `std::string`'s capacity and resize features to manage memory effectively.
Common Mistakes to Avoid
Some common pitfalls include failing to account for special characters or digits, which do not require conversion. Always ensure your string is not empty before attempting conversions, as this may lead to undefined behavior or exceptions.
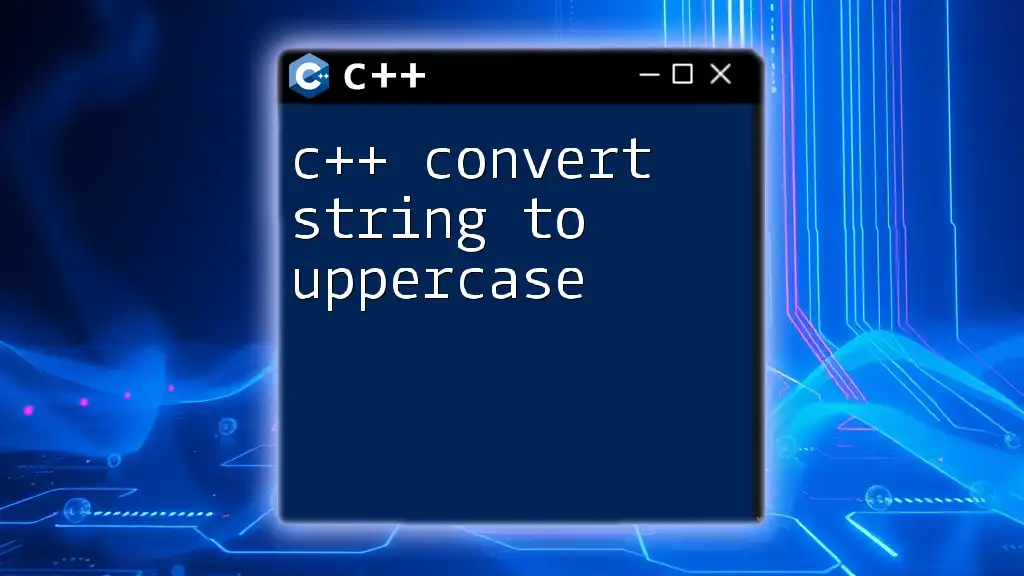
Conclusion
In this guide on how to convert a string to lowercase in C++, we've explored various methods to achieve this, from using `std::transform` to manual loops with `tolower`. Each approach offers unique advantages, and it's crucial to choose based on your application's specific needs. Practicing these techniques will enhance your programming skills and facilitate effective string manipulation in your projects.
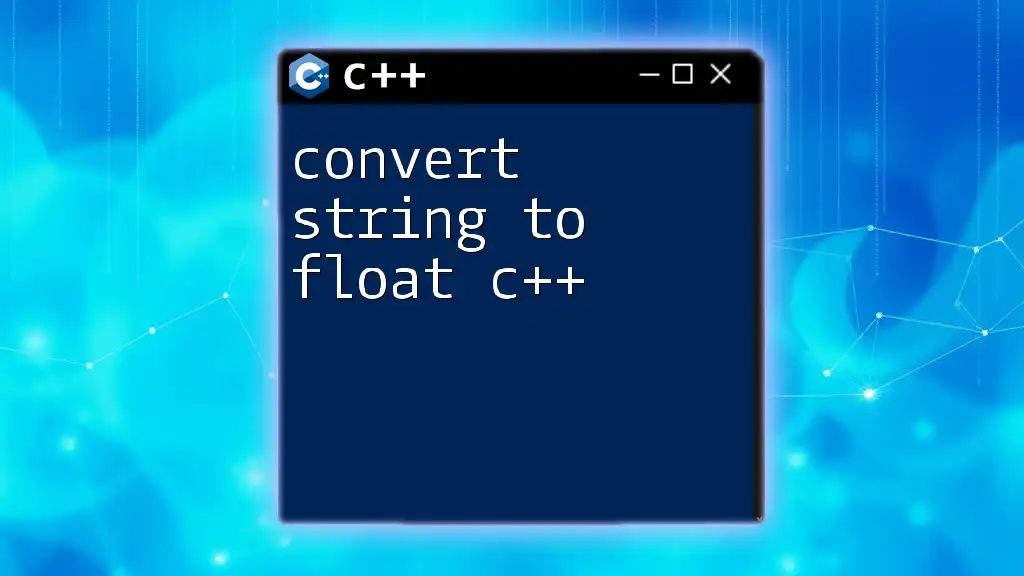
Additional Resources
For further exploration of string manipulation in C++, consider checking out official documentation from the C++ standard library and additional tutorials focusing on string comparisons, regex, and other related topics. Engaging with community forums and discussions can also offer valuable insights and tips from experienced developers.
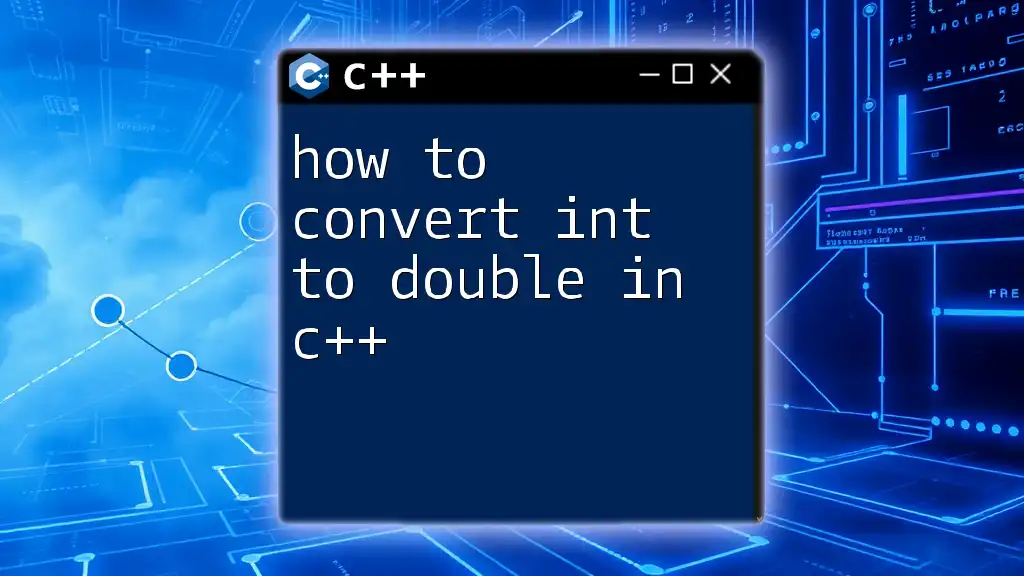
Call to Action
We invite you to share your experiences with string manipulation in C++. What challenges have you faced while converting strings? Comment below with your thoughts, and feel free to share this article with fellow programmers looking to enhance their C++ skills!