To check if a string is empty in C++, you can use the `empty()` method, which returns `true` if the string has no characters.
#include <iostream>
#include <string>
int main() {
std::string myString;
if (myString.empty()) {
std::cout << "The string is empty." << std::endl;
}
return 0;
}
Understanding Strings in C++
What is a String in C++?
In C++, a string is defined as a sequence of characters that can include letters, digits, symbols, and whitespace characters. The standard way to work with strings in C++ is by using the `std::string` class provided in the `<string>` header file. This class offers numerous functionalities that simplify string manipulation, making it a powerful tool for developers.
Why Check if a String is Empty?
Checking if a string is empty is a common yet crucial operation in programming. Some scenarios where string emptiness matters include:
- User Input Validation: When collecting input from users, an empty string may indicate that they skipped a required field.
- File Handling: When reading from a file, an empty string might mean that the file is empty or that the end of the file has been reached.
- Conditional Logic: An empty string might influence the flow of a program, triggering specific actions based on whether or not a string contains text.
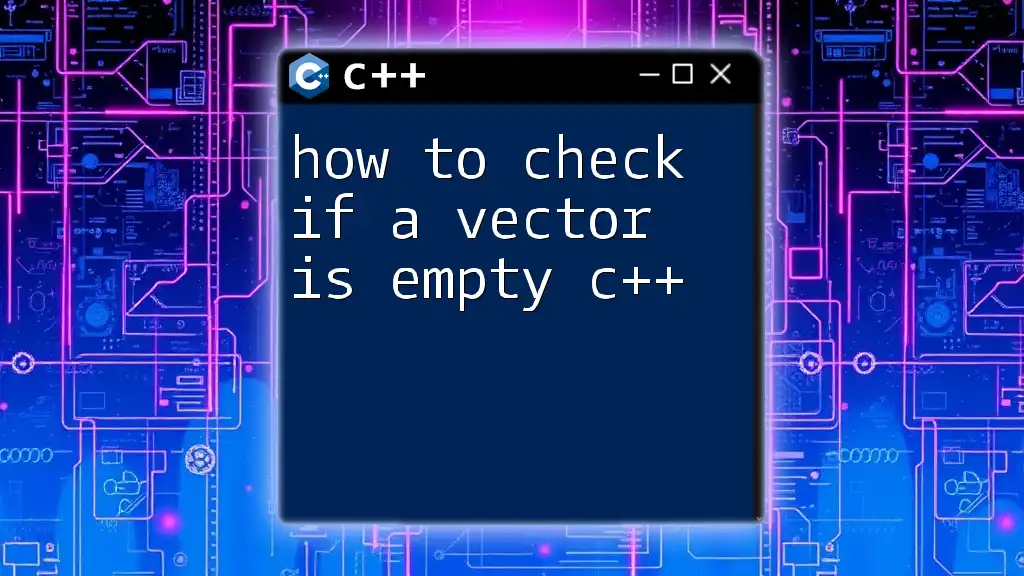
Methods to Check if a String is Empty in C++
Using `std::string::empty()`
The simplest and most intuitive way to check if a string is empty in C++ is to use the `empty()` method. This method returns a boolean value indicating whether the string has any characters or not.
Here’s an example:
#include <iostream>
#include <string>
int main() {
std::string str;
if (str.empty()) {
std::cout << "The string is empty." << std::endl;
} else {
std::cout << "The string is not empty." << std::endl;
}
return 0;
}
Advantages of using `empty()`:
- It provides clear and concise syntax.
- It explicitly indicates the intention of checking for emptiness, enhancing code readability.
Using `std::string::size()` or `std::string::length()`
Another method to check if a string is empty is by using the `size()` or `length()` methods, which return the number of characters in the string. If the result is `0`, the string is considered empty.
For example:
#include <iostream>
#include <string>
int main() {
std::string str;
if (str.size() == 0) {
std::cout << "The string is empty." << std::endl;
} else {
std::cout << "The string is not empty." << std::endl;
}
return 0;
}
You can also use `length()` in place of `size()` as follows:
#include <iostream>
#include <string>
int main() {
std::string str;
if (str.length() == 0) {
std::cout << "The string is empty." << std::endl;
} else {
std::cout << "The string is not empty." << std::endl;
}
return 0;
}
Comparison of `size()` and `length()`:
- Both methods are functionally equivalent; you can choose either based on personal preference or specific coding standards.
- Using `size()` or `length()` can be less readable than using `empty()` because it implies more of a numerical comparison instead of a straightforward check.
Checking Against an Empty String Literal
Another approach is to check if the string is equivalent to an empty string literal (`""`). This method directly compares the string's contents with an explicit empty string.
Here’s an example:
#include <iostream>
#include <string>
int main() {
std::string str;
if (str == "") {
std::cout << "The string is empty." << std::endl;
} else {
std::cout << "The string is not empty." << std::endl;
}
return 0;
}
Discussion on implicit conversions and readability:
- While this method works, it can be less clear than the `empty()` method. The explicit nature of `empty()` signals a precise condition check, making your intent immediately obvious to other programmers reviewing your code.
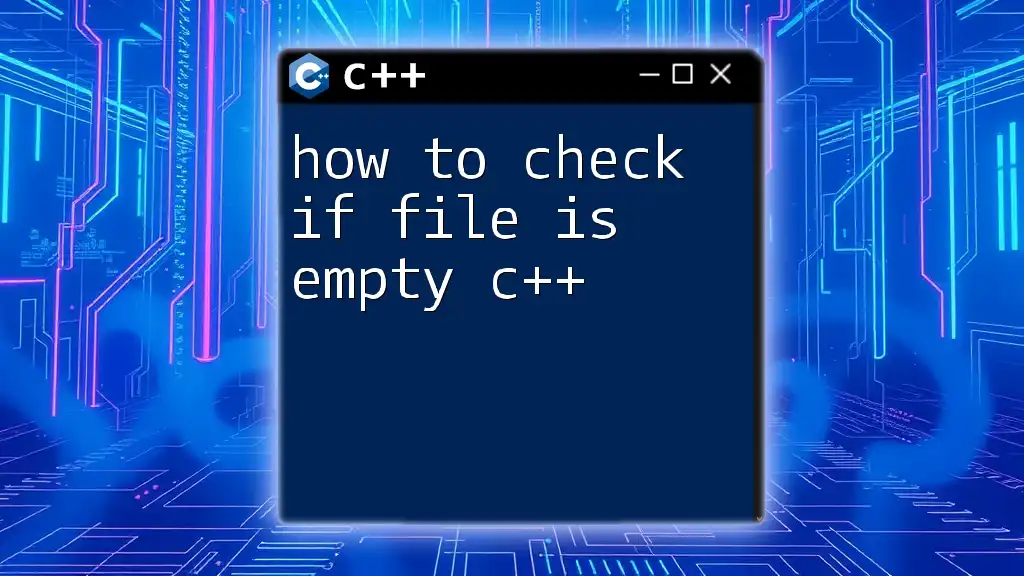
Performance Considerations
Efficiency of Different Methods
When considering performance, here's a quick overview:
- The `empty()` method is optimized for checking emptiness and does not involve any substring or size calculations, making it potentially more efficient than the other approaches.
- While `size()` and `length()` provide the number of characters, they internally perform similar checks and may involve additional overhead compared to a simple boolean return of `empty()`.
In general, if you need to check if a string is empty, prefer using `empty()` for readability and performance, especially in performance-critical applications.
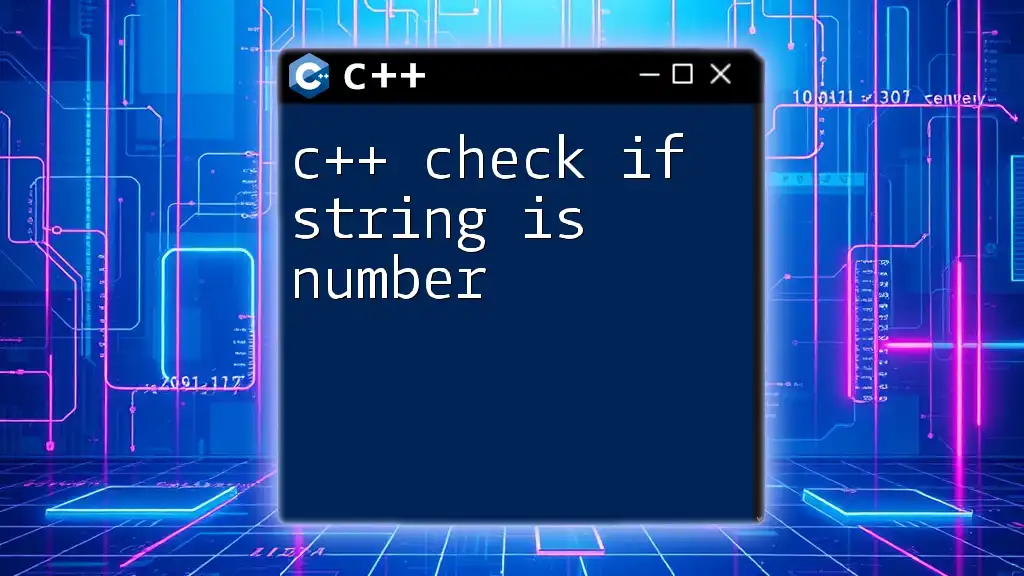
Common Mistakes to Avoid
Misunderstanding String Initialization
Developers might mistakenly think that an uninitialized string is empty. However, this is not always the case. An uninitialized string may lead to undefined behavior. To avoid this, always initialize your strings:
std::string str; // An empty string
std::string uninitialized; // Undefined behavior if used before assigning
When you initialize a string, it starts as empty, which allows you to safely check for emptiness without concerns about undefined behavior.
Confusing Pointers and Arrays
A common mistake involves the difference between C-style strings and C++ `std::string`. C-style strings are arrays of characters, often terminated by a null character (`'\0'`). Here's an example:
char cStr[50] = ""; // An empty C-style string
if (cStr[0] == '\0') {
std::cout << "The C-style string is empty." << std::endl;
}
In contrast, the `std::string` class manages its data internally, providing higher-level functionality and automatic memory management. Understanding these differences is crucial when deciding how to handle strings correctly in your programs.
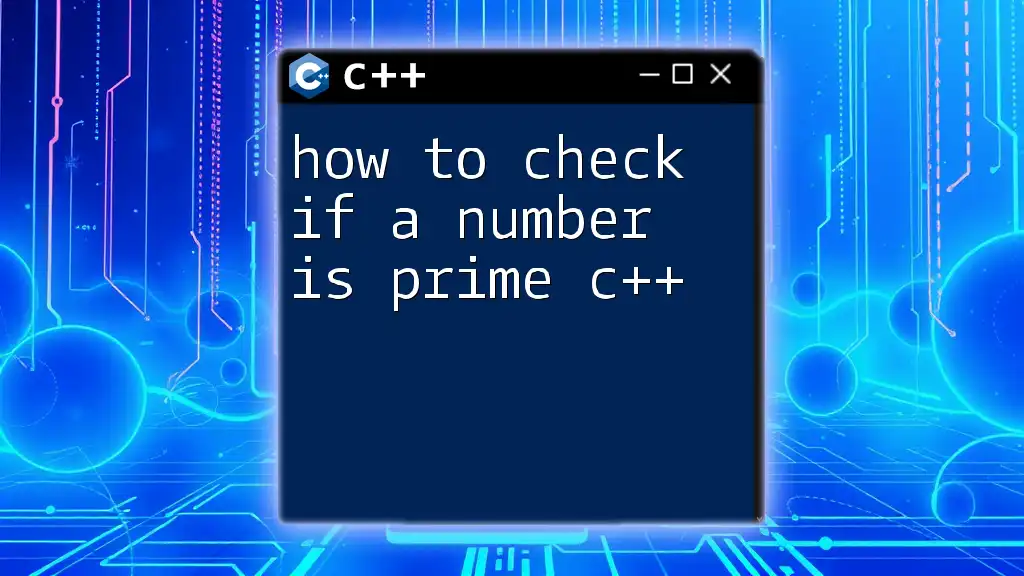
Conclusion
In conclusion, understanding how to check if a string is empty in C++ is fundamental for effective string manipulation. You can utilize methods like `std::string::empty()`, `std::string::size()`, or comparing with an empty string literal. Each method has its advantages and nuances, but overall, the `empty()` method is often the most recommended due to its simplicity and efficiency.
Mastering string operations, including checking for emptiness, will greatly enhance your coding abilities in C++. Start practicing with these techniques in your projects to become more proficient in handling strings!
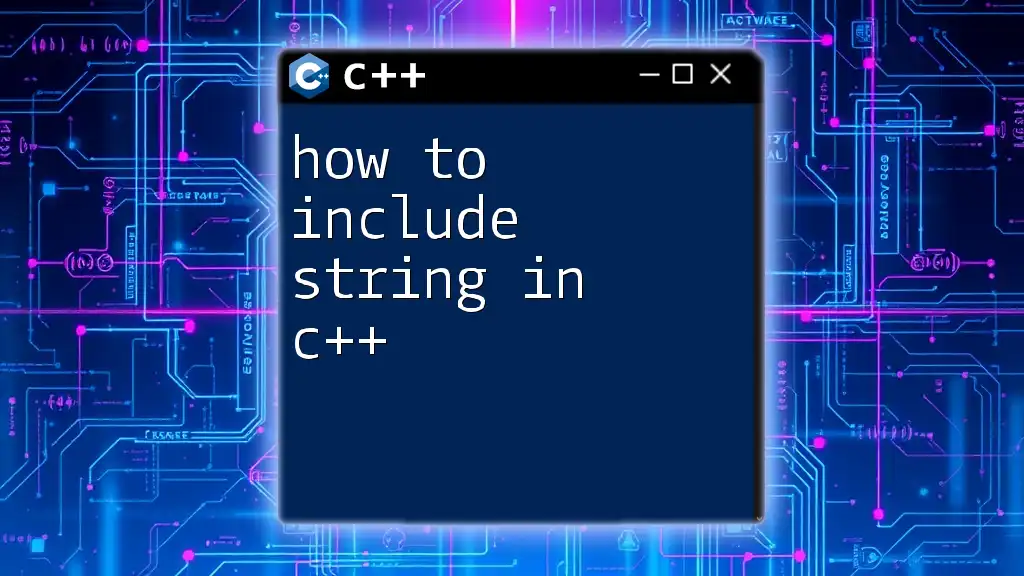
Additional Resources
For further learning, consider exploring:
- Recommended books on C++ programming and standard libraries.
- Online courses and tutorials focused on C++ string manipulation and data types.
- Coding challenge platforms that specifically focus on string operations to sharpen your skills.
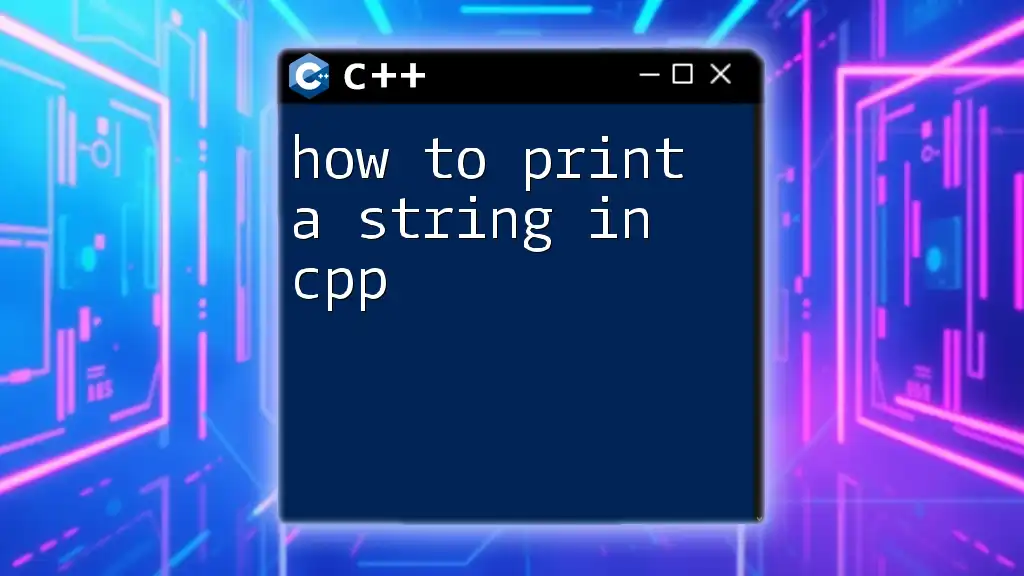
Call to Action
Don’t hesitate to try out different string operations in your own applications. Feel free to subscribe for more C++ programming tips and tutorials to expand your knowledge further!