To check if two strings are equal in C++, you can use the `==` operator to compare them directly. Here's a simple code snippet:
#include <iostream>
#include <string>
int main() {
std::string str1 = "hello";
std::string str2 = "hello";
if (str1 == str2) {
std::cout << "Strings are equal." << std::endl;
} else {
std::cout << "Strings are not equal." << std::endl;
}
return 0;
}
Understanding Strings in C++
What are Strings in C++?
In C++, strings are essential data types used to represent sequences of characters. There are two main types:
- C-style strings: Character arrays terminated by a null character (`'\0'`). For example: `char str[] = "hello";`.
- C++ `std::string`: An object of the `std::string` class, part of the Standard Library. This is more powerful and user-friendly, allowing easy manipulation of string characters.
Why Compare Strings?
Comparing strings is a fundamental operation in many programming tasks, including:
- Validating user inputs: Ensuring that passwords or identifiers meet criteria.
- Implementing search functionality: Determining if a search term is present in a collection of strings.
- Performing logic operations: Making decisions based on string content.
When comparing strings, it is crucial to consider the specific requirements of your application, such as case sensitivity or character encoding.
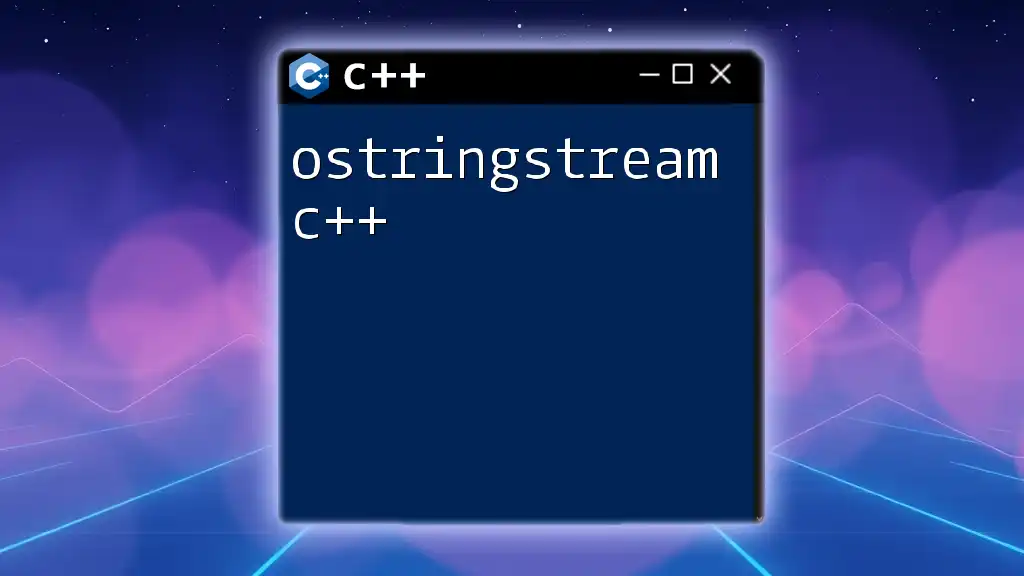
How to Compare Two Strings in C++
Using the `==` Operator
One of the simplest methods to check if two strings are equal in C++ is by using the `==` operator. This operator compares the actual content of two `std::string` objects.
Example Code
#include <iostream>
#include <string>
int main() {
std::string str1 = "hello";
std::string str2 = "hello";
if (str1 == str2) {
std::cout << "Strings are equal!" << std::endl;
} else {
std::cout << "Strings are not equal!" << std::endl;
}
return 0;
}
Explanation
In the above example, the `==` operator compares `str1` and `str2`. Since both contain the same sequence of characters, the output will be "Strings are equal!". This method is straightforward and, in most cases, recommended for its clarity and simplicity.
Using `std::string::compare()`
Another method for string comparison is utilizing the `compare()` member function of the `std::string` class. This method provides more flexibility and control:
- Return Values:
- `0` means the strings are equal.
- A positive value indicates that the calling string is lexicographically greater.
- A negative value indicates that the calling string is lexicographically lesser.
Example Code
#include <iostream>
#include <string>
int main() {
std::string str1 = "hello";
std::string str2 = "world";
if (str1.compare(str2) == 0) {
std::cout << "Strings are equal!" << std::endl;
} else {
std::cout << "Strings are not equal!" << std::endl;
}
return 0;
}
Explanation
Here, `str1.compare(str2)` returns a value that is not zero because `"hello"` is lexicographically less than `"world"`. Thus, the output will be "Strings are not equal!". The `compare()` function is particularly useful when you want to determine string order or need more detailed comparisons.
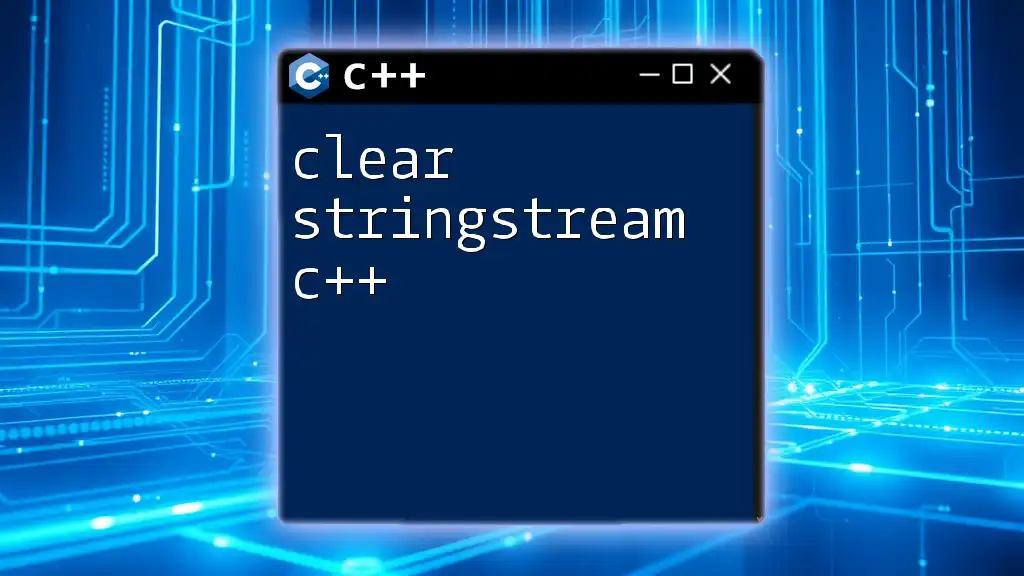
How to Check if Two Strings Are Equal Case-Insensitive
Using `std::transform` and `std::equal`
In many cases, you might want to compare two strings without considering case sensitivity. You can accomplish this by converting both strings to the same case (either lower or upper) before comparison.
Example Code
#include <iostream>
#include <string>
#include <algorithm>
bool caseInsensitiveEqual(const std::string& str1, const std::string& str2) {
std::string lowerStr1 = str1;
std::string lowerStr2 = str2;
std::transform(lowerStr1.begin(), lowerStr1.end(), lowerStr1.begin(), ::tolower);
std::transform(lowerStr2.begin(), lowerStr2.end(), lowerStr2.begin(), ::tolower);
return lowerStr1 == lowerStr2;
}
int main() {
std::string str1 = "HELLO";
std::string str2 = "hello";
if (caseInsensitiveEqual(str1, str2)) {
std::cout << "Strings are equal (case-insensitive)!" << std::endl;
} else {
std::cout << "Strings are not equal!" << std::endl;
}
return 0;
}
Explanation
In this example, `caseInsensitiveEqual` function transforms both `str1` and `str2` to lowercase using `std::transform`. The strings are then compared. As a result, the output will be "Strings are equal (case-insensitive)!", demonstrating successful case-insensitive comparison.
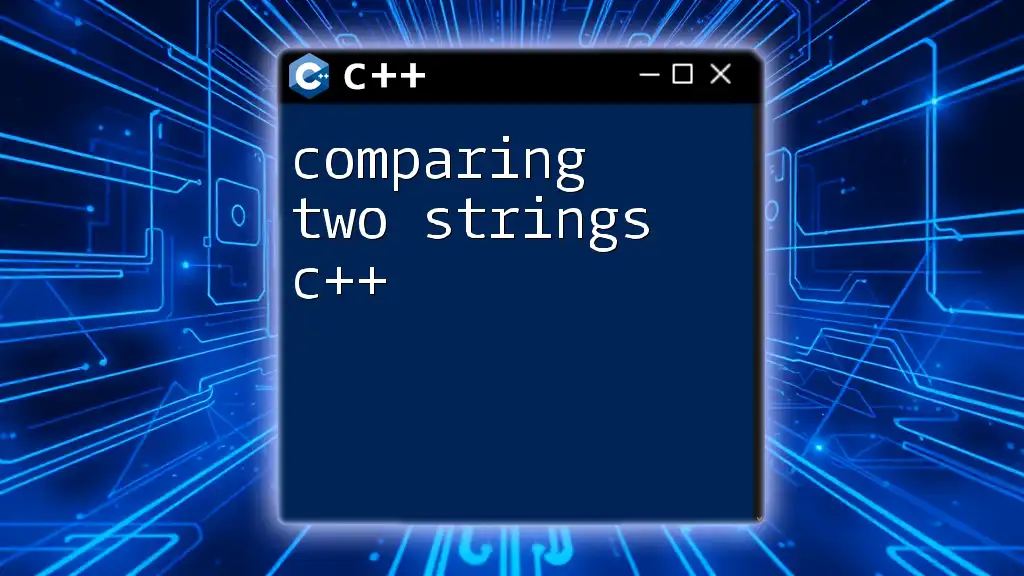
Performance Considerations in String Comparison
Time Complexity in Comparing Strings
The performance of string comparisons can vary based on the lengths of the strings and the methods used. Generally, comparing two strings has a time complexity of O(n), where n is the length of the shortest string.
Best Practices
To enhance performance:
- Short-circuiting: If comparing the lengths of two strings first and they differ, you can avoid a full comparison, thereby reducing operational time.
- Early exits: Implement conditions to break out of loops during comparisons as soon as a difference is found.
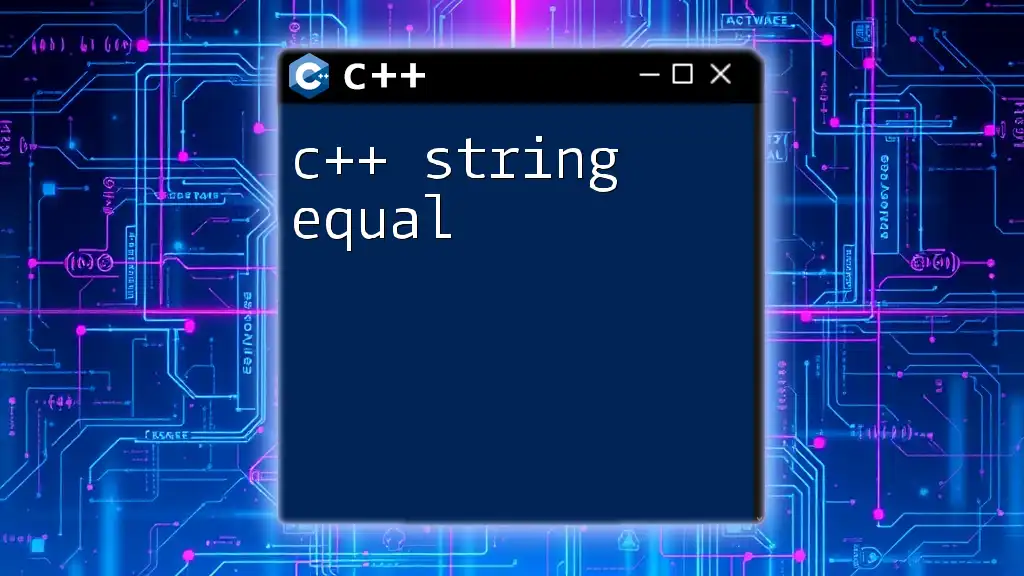
Common Errors in String Comparison
Understanding `nullptr` and Empty Strings
When comparing strings, it’s critical to account for potential pitfalls, like comparing with null pointers or empty strings.
- Attempting to compare a `nullptr` with a `std::string` will lead to undefined behavior.
- Always ensure strings are initialized before comparison to avoid crashes or misleading outputs.
Example of Pitfall
std::string str1;
std::string str2 = "test";
if (str1 == str2) {
// Potentially misleading if str1 is empty
}
Always validate string inputs before performing comparisons to ensure reliable behavior.
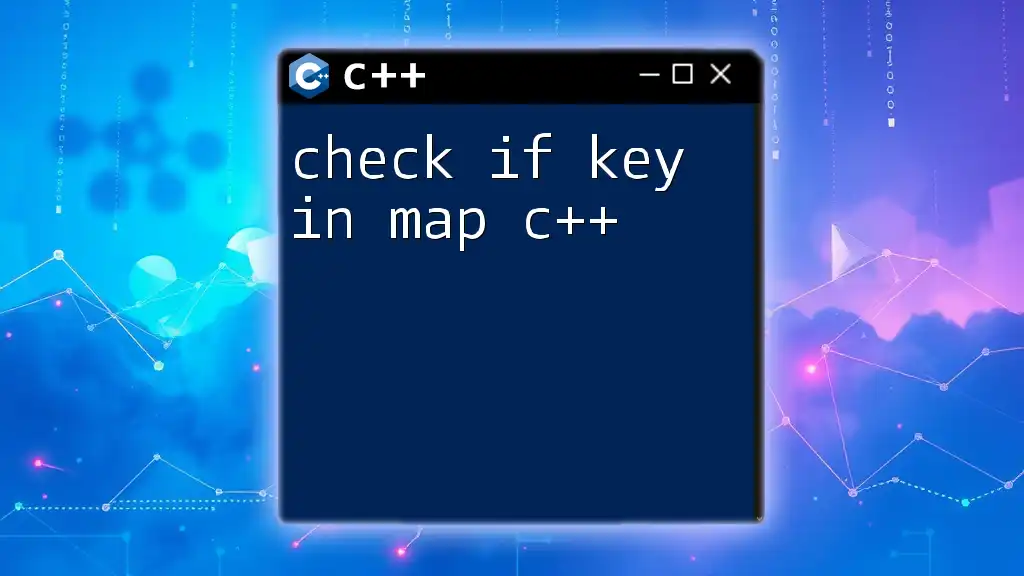
Conclusion
In C++, checking if two strings are equal can be accomplished with a few straightforward techniques. Utilizing the `==` operator or the `compare()` method provides flexibility and clarity. Case-insensitive comparisons can be performed efficiently, and understanding time complexity can lead to performance optimizations. By avoiding common errors and following best practices, you can ensure accurate and efficient string comparisons in your C++ applications.
Additional Resources
For further reading, consult the C++ Standard Library documentation for detailed explanations of `std::string` and related functions, or explore C++ string manipulation tutorials to expand your understanding of handling strings in various contexts.
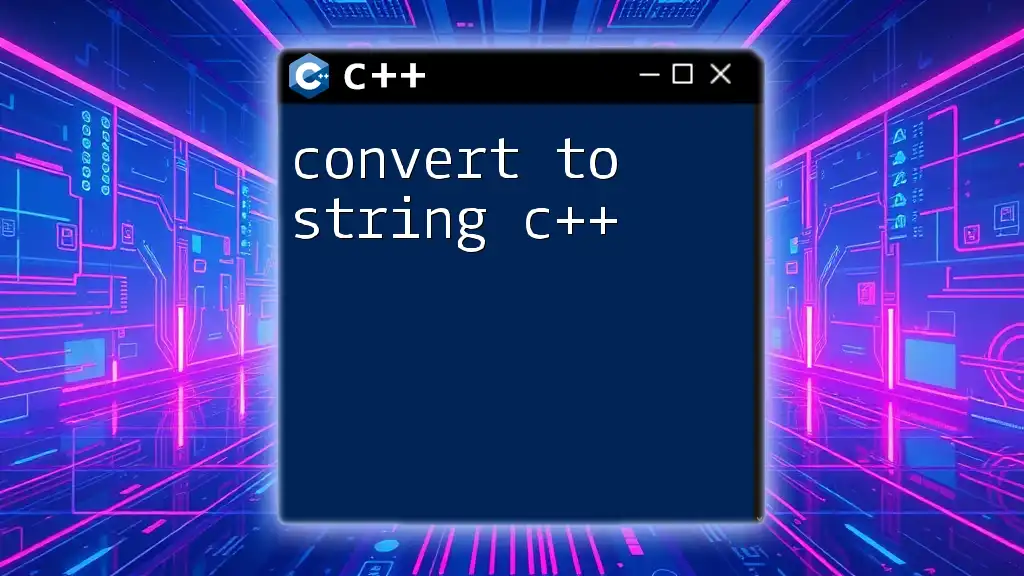
FAQs about String Comparisons in C++
What is the difference between `==` and `compare()`?
The `==` operator checks for equality between two `std::string` objects, while `compare()` offers a more detailed comparison that indicates their lexicographical order.
When should I use case-insensitive comparison?
Use case-insensitive comparison when the exact case of characters does not matter, such as in user inputs, usernames, or settings.
Can I compare C-style strings using `std::string` operators?
No, if comparing C-style strings (`char*`), you should use functions like `strcmp()` from the `<cstring>` library. Directly applying `==` on C-style strings leads to comparison of memory addresses, not the actual string content.