In C++, the "less than or equal to" operator (<=) is used to compare two values, returning true if the left operand is less than or equal to the right operand.
Here’s a code snippet demonstrating its usage:
#include <iostream>
int main() {
int a = 5;
int b = 10;
if (a <= b) {
std::cout << "a is less than or equal to b" << std::endl;
} else {
std::cout << "a is greater than b" << std::endl;
}
return 0;
}
Understanding Comparison Operators
What are Comparison Operators?
In programming, comparison operators play a crucial role in determining relationships between two values. They allow you to compare variables and expressions, facilitating decision-making in your code. In C++, common comparison operators include:
- `<` (Less Than)
- `>` (Greater Than)
- `<=` (Less Than or Equal To)
- `>=` (Greater Than or Equal To)
- `==` (Equal To)
- `!=` (Not Equal To)
Symbols and Their Meaning
Each of these operators has a specific function that dictates how two values relate. Among these operators, the less than or equal to operator (`<=`) is particularly important for establishing inclusive boundaries in comparisons.

The Less Than or Equal To Operator (`<=`)
Definition and Syntax
The less than or equal to operator (`<=`) checks whether the left-hand operand is less than or equal to the right-hand operand. The syntax for using the `<=` operator in a C++ expression is straightforward:
expression1 <= expression2
This operation will return a boolean value: `true` if the condition is satisfied and `false` otherwise.
Context of Use
The `<=` operator is commonly used in various contexts, such as conditional statements and loop constructs. Understanding how to utilize this operator effectively allows you to write clear and efficient code.

Practical Examples
Basic Example
Here’s a simple example demonstrating the use of the `<=` operator in a conditional statement:
#include <iostream>
int main() {
int a = 5;
int b = 10;
if (a <= b) {
std::cout << "a is less than or equal to b." << std::endl;
}
return 0;
}
Explanation: In this example, the program checks if `a` is less than or equal to `b`. Since `5` is indeed less than `10`, the output will be:
a is less than or equal to b.
Comparing Different Data Types
Integer Comparisons
Let’s take a look at another example where we compare two integer values:
int num1 = 7;
int num2 = 7;
if (num1 <= num2) {
std::cout << "num1 is less than or equal to num2." << std::endl;
}
In this case, since both values are equal, the condition `num1 <= num2` evaluates to `true`, leading to the output:
num1 is less than or equal to num2.
Floating-Point Comparisons
The `<=` operator can also be used with floating-point numbers. However, there are certain nuances to be aware of when dealing with precision:
double x = 3.14;
double y = 3.14;
if (x <= y) {
std::cout << "x is less than or equal to y." << std::endl;
}
Discussion on Precision Issues: Unlike integers, floating-point comparisons can lead to misleading results due to precision errors. So, while `x` and `y` are initialized to the same value, any minor inaccuracies in floating-point representation can affect the outcome. It’s important to be mindful of this when comparing floating-point numbers.
Using `<=` in Loops
While Loop Example
The less than or equal operator (`<=`) is frequently employed in loops. Here’s an example using a `while` loop:
int count = 0;
while (count <= 5) {
std::cout << count << std::endl;
count++;
}
Explanation of Execution: The loop continues to execute as long as `count` is less than or equal to `5`. As a result, this loop will print:
0
1
2
3
4
5
For Loop Example
Similarly, you can use the `<=` operator in a `for` loop:
for (int i = 0; i <= 5; i++) {
std::cout << i << " ";
}
This code will produce the output:
0 1 2 3 4 5
By using the `<=` operator, you ensure the loop executes for the fifth iteration, demonstrating its utility thus far.

Practical Applications
Real-World Scenarios
The `<=` operator is indispensable in many real-world programming applications. For instance:
- User Input Validation: You might want to ensure that user-provided values fall within a specific range. Using the `<=` operator helps facilitate this control.
- Game Development: In games, you often check if a player's score is less than or equal to a target score to determine the next steps.
Performance Considerations
While using comparison operators like `<=`, it's essential to think about performance, particularly when handling large datasets or complex algorithms. Clear and concise logic improves code readability, making it easier to maintain and optimize in the future.
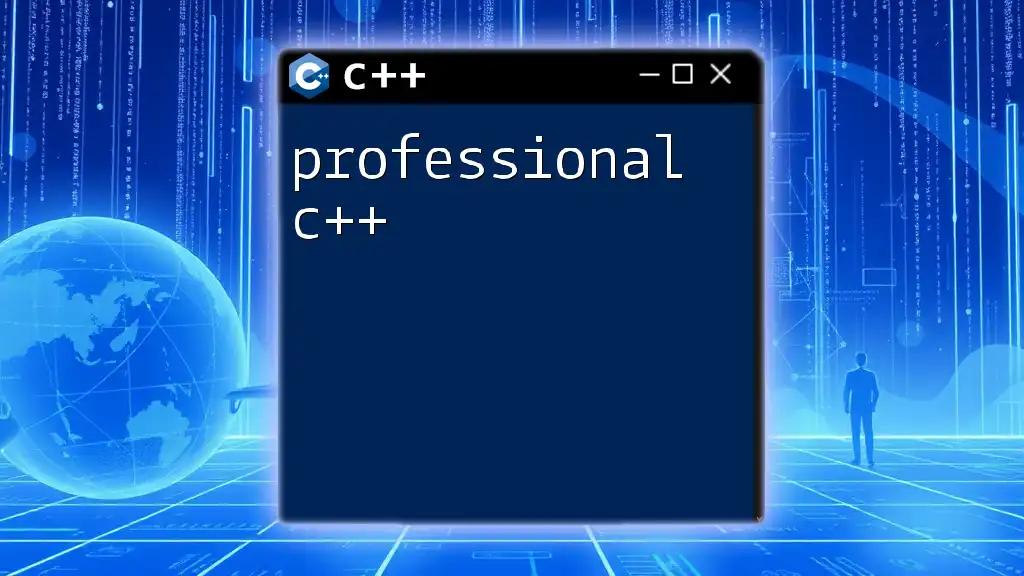
Debugging Common Issues
Common Mistakes
While using the `<=` operator, developers often fall into the trap of off-by-one errors. For instance, forgetting to adjust the boundary conditions of a loop can lead to unintended repetitions or missed iterations.
Troubleshooting Tips
To troubleshoot issues arising from incorrect use of the `<=` operator:
- Use Print Debugging: Insert print statements to observe variable values at different points in your code.
- Boundary Testing: Create test cases that target the boundary values to ensure your conditions work as expected.
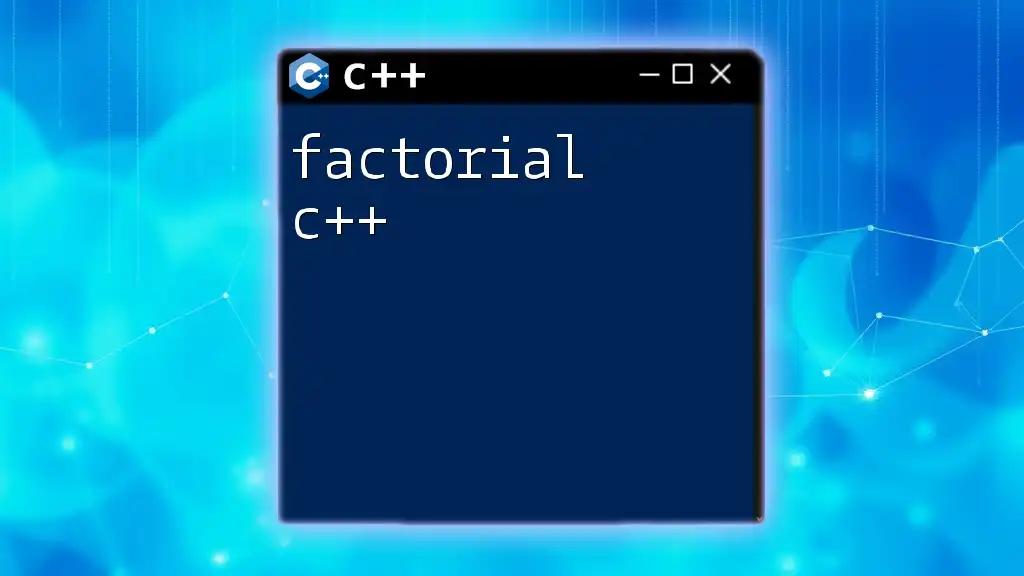
Conclusion
Understanding how to effectively use the less than or equal to operator (`<=`) in C++ can greatly enhance your programming capabilities. Whether in conditions, comparisons, or loops, this operator is integral to creating clear and efficient code. By practicing and implementing these concepts in various coding scenarios, you can master this essential aspect of C++.