In C++, the "less than or equal to" operator (`<=`) is used to compare two values and returns `true` if the left value is less than or equal to the right value; otherwise, it returns `false`.
Here’s a code snippet demonstrating its use:
#include <iostream>
int main() {
int a = 5;
int b = 10;
if (a <= b) {
std::cout << "a is less than or equal to b" << std::endl;
} else {
std::cout << "a is greater than b" << std::endl;
}
return 0;
}
Understanding Comparison Operators in C++
Comparison operators are fundamental components of C++ programming that allow developers to evaluate relationships between values. They help in making decisions based on conditions, which is essential for controlling the flow of a program.
Among these operators, the less than or equal to operator (`<=`) plays a crucial role in comparisons, enabling programmers to check not only if one value is less than another but also if the two values are equal.
The Less Than or Equal To Operator
The less than or equal to operator is represented as `<=`. This operator returns `true` when the left operand is either less than or equal to the right operand. Its simple yet powerful functionality is pivotal in various programming scenarios, including conditional statements and loop controls.

Syntax of the Less Than or Equal To Operator
The syntax for using the less than or equal to operator is straightforward. It follows this basic structure:
a <= b
Here, `a` and `b` are the operands being compared. If `a` is less than or equal to `b`, the expression evaluates to `true`; otherwise, it evaluates to `false`.
Code Snippet Example
To illustrate the syntax, consider the following code:
#include <iostream>
using namespace std;
int main() {
int a = 5;
int b = 10;
cout << (a <= b); // Outputs 1 (true)
return 0;
}
In this example, the expression `(a <= b)` evaluates to `true`, producing the output `1`. This demonstrates how the operator functions in a basic scenario.

Practical Usage of Less Than or Equal To in C++
The versatility of the less than or equal to operator shines through in practical programming scenarios, particularly in control structures like `if-else` statements and loops.
Control Structures Using <=
If-Else Statements
The operator can be seamlessly integrated into `if-else` conditions to execute different blocks of code based on specific criteria. For instance:
#include <iostream>
using namespace std;
int main() {
int score;
cout << "Enter your score: ";
cin >> score;
if (score <= 50) {
cout << "You need to improve." << endl;
} else {
cout << "Well done!" << endl;
}
return 0;
}
In this code, the program evaluates the `score` input by the user and provides feedback based on whether the score is less than or equal to 50.
Loops Using <=
The operator is also incredibly valuable in controlling loop execution. For instance, using `<=` in a `for` loop can define how long the loop will run:
#include <iostream>
using namespace std;
int main() {
for (int i = 1; i <= 5; i++) {
cout << i << " ";
}
return 0;
}
This example prints the numbers from 1 to 5. The condition `i <= 5` ensures that the loop continues executing as long as `i` is less than or equal to 5.
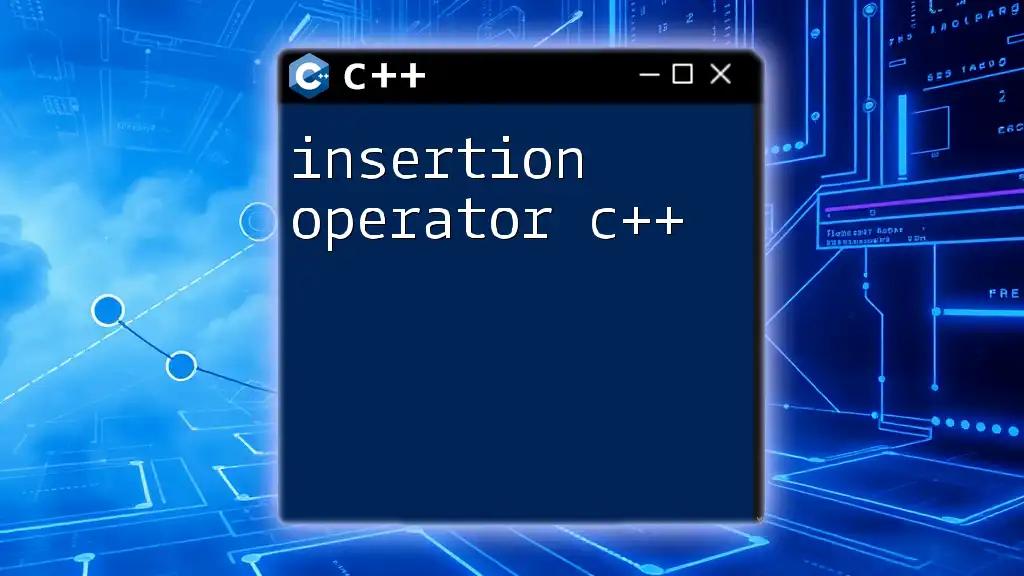
Common Scenarios Where C++ Less Than or Equal To is Used
In Sorting Algorithms
In sorting algorithms, comparison operators play a vital role in determining the order of elements. Using `<=` can help implement effective sorting strategies, such as bubble sort or selection sort, by comparing neighboring elements and arranging them accordingly.
In Conditional Statements
The less than or equal to operator is frequently used in conditional statements where decisions must be made based on variable values. For example, you might check if an input value is valid before processing it.
In Validating User Input
Often, input validation involves ensuring that a user's input meets specific criteria. The use of `<=` allows programmers to enforce restrictions effectively, ensuring the program operates within the expected parameters.
Example Scenario
Here’s an example demonstrating user input validation:
#include <iostream>
using namespace std;
int main() {
int age;
cout << "Enter your age: ";
cin >> age;
if (age <= 18) {
cout << "You are a minor." << endl;
} else {
cout << "You are an adult." << endl;
}
return 0;
}
In this code, it checks whether the provided age is less than or equal to 18 to categorize the user appropriately.

Best Practices for Using Less Than or Equal To in C++
When coding with the less than or equal to operator, it is essential to adopt best practices to avoid common pitfalls.
Avoiding Common Mistakes
A frequent mistake is confusing `<=` with `<`. The difference is subtle but significant—using `<` means the value cannot be equal, while `<=` allows for equality. Ensuring this distinction can prevent critical logical errors.
Naming Conventions in Conditions
It’s crucial to use clear and descriptive variable names when incorporating `<=` in conditions. For example, naming a variable `userAge` instead of just `age` in the previous examples enhances clarity and readability.

Conclusion
The less than or equal to in C++ operator is not just a simple comparison tool; it is a powerful element that drives logic and flows in programming. Understanding its syntax and practical applications equips programmers with the ability to build robust and effective C++ applications. By practicing its use and applying best practices, developers can enhance their coding efficiency and accuracy.
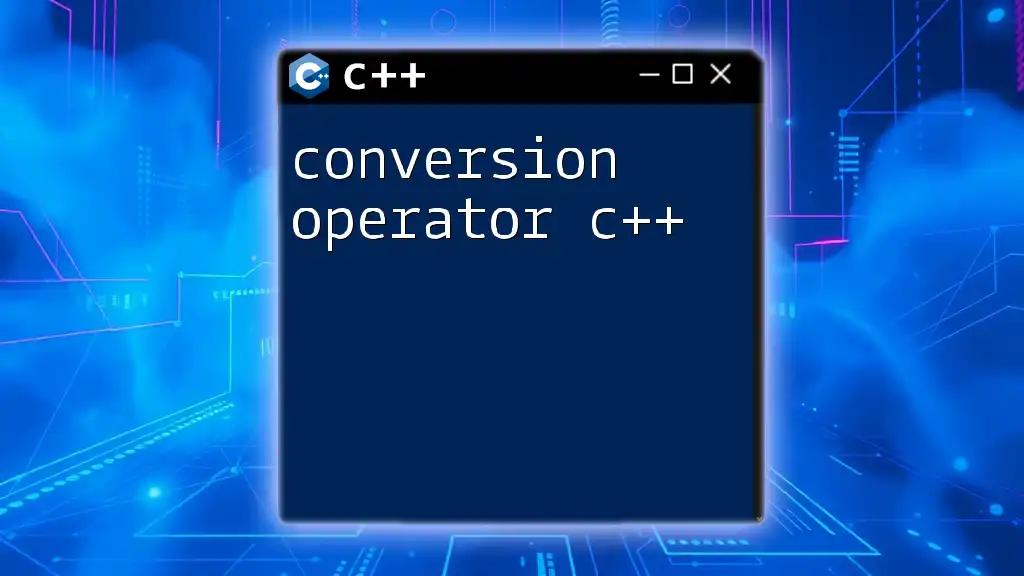
FAQs
What is the difference between `<` and `<=`?
The `<` operator checks if the left operand is strictly less than the right operand, whereas the `<=` operator checks if it is either less than or equal to the right operand. This distinction is crucial when determining conditions in your code.
When should I use less than or equal to inside loops?
Using `<=` in loops is common when you need to include the upper limit in your iteration count. For example, if you're printing numbers from 1 to N, using `i <= N` allows N to be included in the output.
Can I use `<=` with floating-point numbers?
Yes, the less than or equal to operator can be used with floating-point numbers, but be cautious due to the precision issues associated with floating-point arithmetic. Always consider the possible rounding errors when performing comparisons with floating-point values.
This comprehensive guide to the less than or equal to operator in C++ serves as a valuable resource for both novice and seasoned programmers, enhancing their understanding and implementation of comparison operations in C++.