In C++, an HTTP request can be made using libraries like cURL to easily communicate with web servers; below is a simple example demonstrating how to perform a GET request.
#include <curl/curl.h>
#include <iostream>
int main() {
CURL *curl;
CURLcode res;
curl_global_init(CURL_GLOBAL_DEFAULT);
curl = curl_easy_init();
if(curl) {
curl_easy_setopt(curl, CURLOPT_URL, "http://example.com");
res = curl_easy_perform(curl);
curl_easy_cleanup(curl);
}
curl_global_cleanup();
return 0;
}
Understanding HTTP Requests
What is an HTTP Request?
HTTP, or Hypertext Transfer Protocol, is the foundation of data communication on the World Wide Web. An HTTP request is a message sent by a client to a server. This request asks for resources, data, or actions that the server can perform. Understanding the different types of HTTP requests is essential when learning how to effectively manage data between a client and a server.
The most common types of HTTP requests include:
- GET: Retrieves data from a specified resource.
- POST: Sends data to the server to create or update a resource.
- PUT: Updates a resource.
- DELETE: Removes a specified resource.
The Structure of an HTTP Request
An HTTP request comprises several components that define what the client wants to do with the resource. The structure includes:
- Request Line: Specifies the method (GET, POST, etc.), the URL, and the HTTP version.
- Headers: Provide additional information (like content type, authorization, etc.) to the server.
- Body: (If applicable) Contains data being sent to the server, usually in POST and PUT requests.
Here’s an example of a basic HTTP GET request:
GET /index.html HTTP/1.1
Host: www.example.com
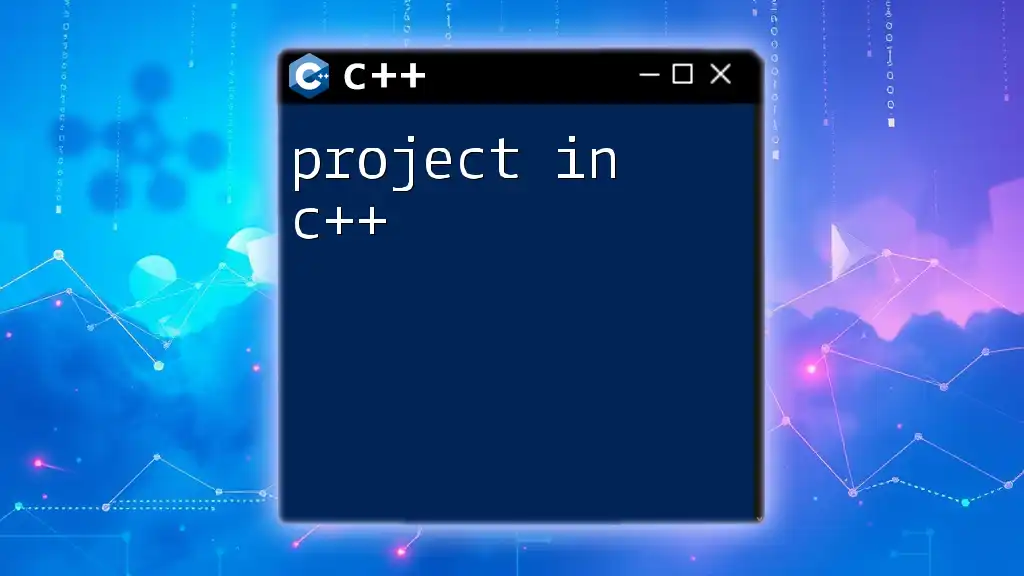
Setting Up the C++ Environment for HTTP Requests
Required Libraries
To manage HTTP requests in C++, various libraries can be utilized. Two prominent libraries are:
- cURL: A powerful and widely-used library for transferring data with URLs. It supports many protocols including HTTP, HTTPS, FTP, etc.
- Boost.Beast: A newer library that builds upon Boost.Asio and provides more modern C++ features, offering robust capabilities for making HTTP requests.
Comparison of Libraries
When choosing a library for making HTTP requests in C++, consider the following:
- cURL: Excellent for its ease of use and broad support for protocols.
- Boost.Beast: Better suited for projects requiring asynchronous operations or detailed control over the request process.
Installing the Libraries
To begin using these libraries, you will first need to install them on your development environment.
Installing cURL
For Windows users:
- Download the cURL installer from [cURL's official website](https://curl.se/download.html).
- Follow the installation instructions.
For Linux users: You can install cURL using your package manager:
sudo apt-get install libcurl4-openssl-dev
For macOS users: You can use Homebrew:
brew install curl
Installing Boost.Beast
To install Boost libraries, including Boost.Beast, follow these steps based on your environment:
- For Windows, you can download the binaries or use vcpkg to install Boost.
- On Linux and macOS, you can install using:
sudo apt-get install libboost-all-dev
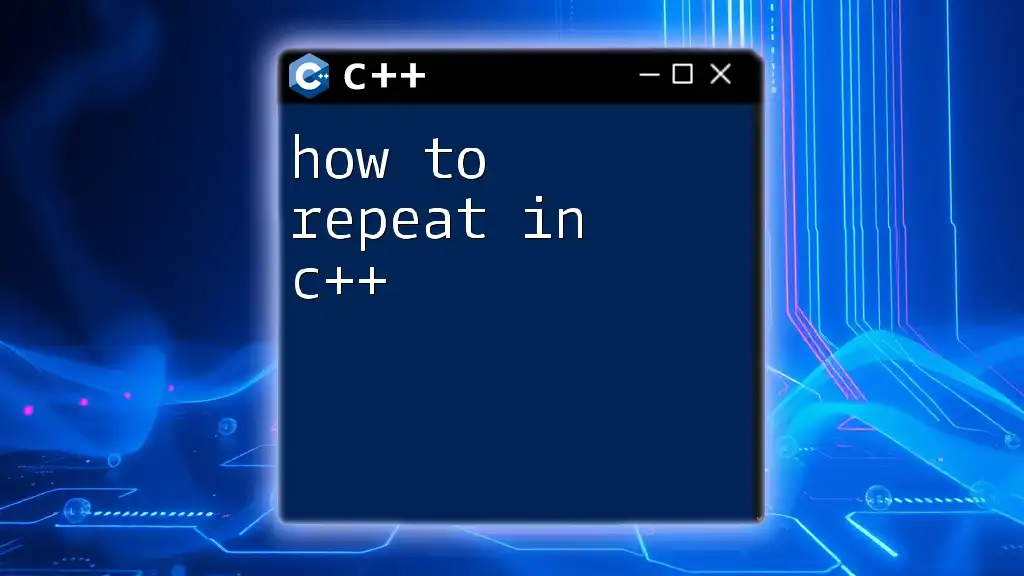
Making Your First HTTP Request in C++
Using cURL to Make HTTP Requests
Simple GET Request
Making your first HTTP request in C++ with cURL is straightforward. Here’s a simple example demonstrating how to perform a GET request.
#include <iostream>
#include <curl/curl.h>
int main() {
CURL *curl;
CURLcode res;
curl_global_init(CURL_GLOBAL_ALL);
curl = curl_easy_init();
if(curl) {
curl_easy_setopt(curl, CURLOPT_URL, "http://example.com");
res = curl_easy_perform(curl);
// Check for errors
if(res != CURLE_OK) {
std::cerr << "curl_easy_perform() failed: " << curl_easy_strerror(res) << std::endl;
}
// Cleanup
curl_easy_cleanup(curl);
}
curl_global_cleanup();
return 0;
}
In the code above:
- We initialize cURL with `curl_global_init`, allowing it to work across threads.
- We create a cURL easy handle with `curl_easy_init`.
- We set the URL for the request using `curl_easy_setopt`.
- We perform the request with `curl_easy_perform`, and finally, we clean up resources.
This example fetches the HTML content from "http://example.com". Upon successful execution, you should see the output of the HTTP request in your console.
Sending a POST Request
To send data to the server with a POST request, you can modify the above code as follows:
curl_easy_setopt(curl, CURLOPT_POST, 1L);
curl_easy_setopt(curl, CURLOPT_POSTFIELDS, "name=example&project=cURL");
This extra configuration sets the request method to POST and sends URL-encoded form data containing key-value pairs.
Using Boost.Beast for HTTP Requests
Setting Up the Boost Environment
Ensure you have configured your project to use Boost libraries. Include necessary header files for both Asio and Beast.
GET Request Example
Here’s an example of a GET request using Boost.Beast:
#include <boost/beast/core.hpp>
#include <boost/beast/http.hpp>
#include <boost/asio.hpp>
namespace beast = boost::beast;
namespace http = beast::http;
namespace net = boost::asio;
int main() {
net::io_context io_context;
// Create a resolver, and then a socket
tcp::resolver resolver{io_context};
beast::tcp_stream stream{io_context};
// Resolve the domain name
auto const results = resolver.resolve("example.com", "80");
// Connect to the server
net::connect(stream.socket(), results.begin(), results.end());
// Set up the request
http::request<http::string_body> req{http::verb::get, "/", 11};
req.set(http::field::host, "example.com");
req.set(http::field::user_agent, BOOST_BEAST_VERSION_STRING);
// Send the request
http::write(stream, req);
// Prepare a buffer to hold the response
beast::flat_buffer buffer;
// Create a response object
http::response<http::string_body> res;
// Receive the response
http::read(stream, buffer, res);
std::cout << res << std::endl;
// Close the stream
beast::error_code ec;
stream.socket().shutdown(tcp::socket::shutdown_both, ec);
return 0;
}
In the code example, the following occurs:
- We create a `tcp::resolver` to resolve the server address.
- We connect to the server and prepare an HTTP GET request.
- We send the request and await the response, which is printed to the console.
Sending Data with a POST Request
When sending data with Boost.Beast, you can create a JSON payload as follows:
http::request<http::string_body> req{http::verb::post, "/submit", 11};
req.set(http::field::content_type, "application/json");
req.body() = R"({"name":"example", "project":"Boost.Beast"})";
req.prepare_payload();
// Send the request
http::write(stream, req);
Here, we set the content type to `application/json`, assign the JSON string to the request body, and then send the request to the server.
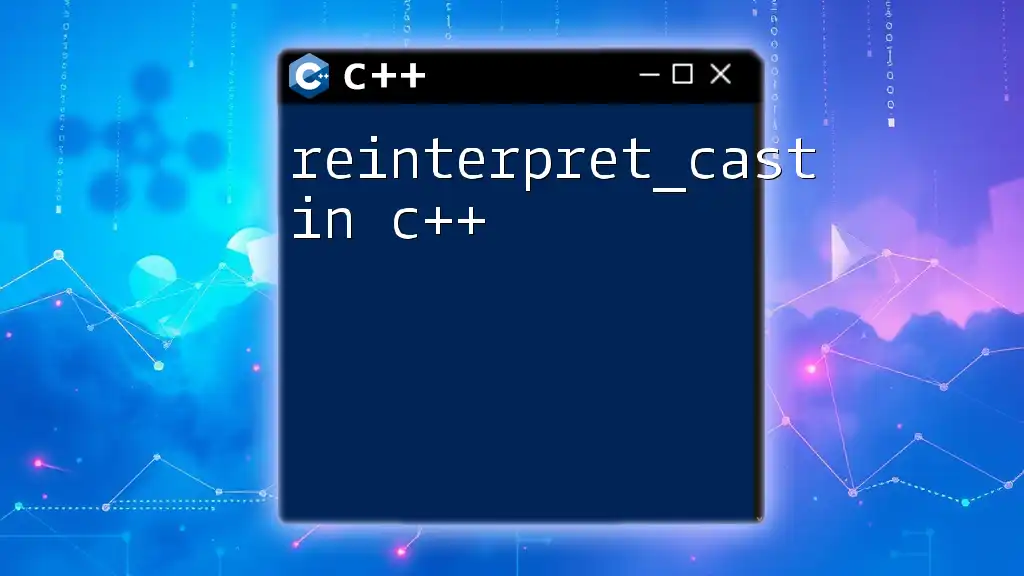
Handling Responses
Reading Response Data
Upon receiving a response, it's essential to process the data. As demonstrated in the Boost.Beast example, you can extract the response body using the `body()` method. This allows you to manipulate or display the data as needed.
Error Handling
Error handling is crucial for robust applications. Common errors include connection timeouts, unreachable servers, or incorrect request formats. Always check for and handle errors in your code gracefully. For instance, in cURL, you check the return value of `curl_easy_perform()` to ensure that the operation succeeded.
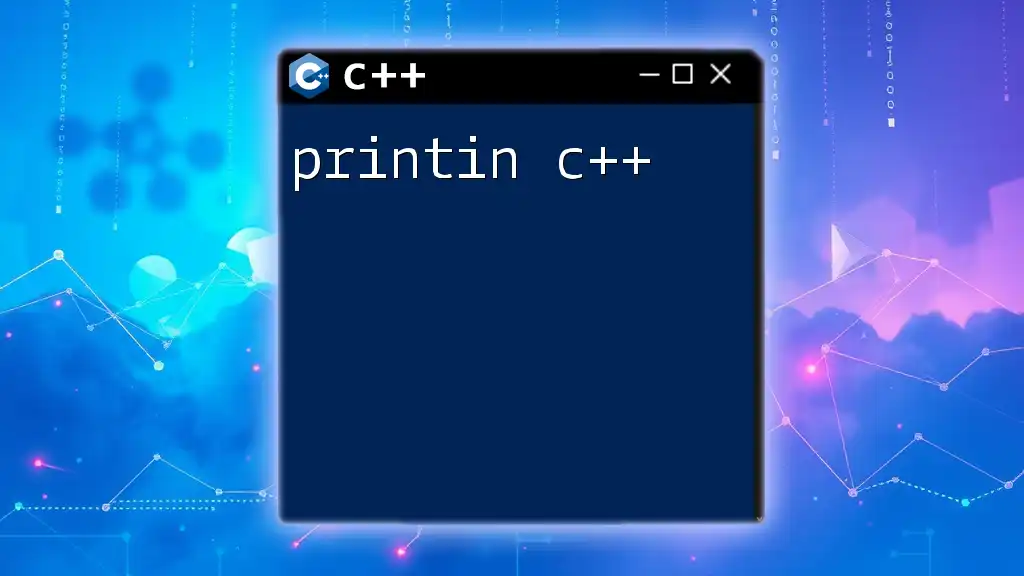
Conclusion
Making an HTTP request in C++ opens up numerous possibilities in web development and networking applications. Both cURL and Boost.Beast provide powerful tools to handle such requests straightforwardly.
As you practice and experiment with these libraries, you can dynamically interact with web services, retrieve data, and send information securely. This hands-on experience will deepen your understanding of client-server communication in the context of modern programming.
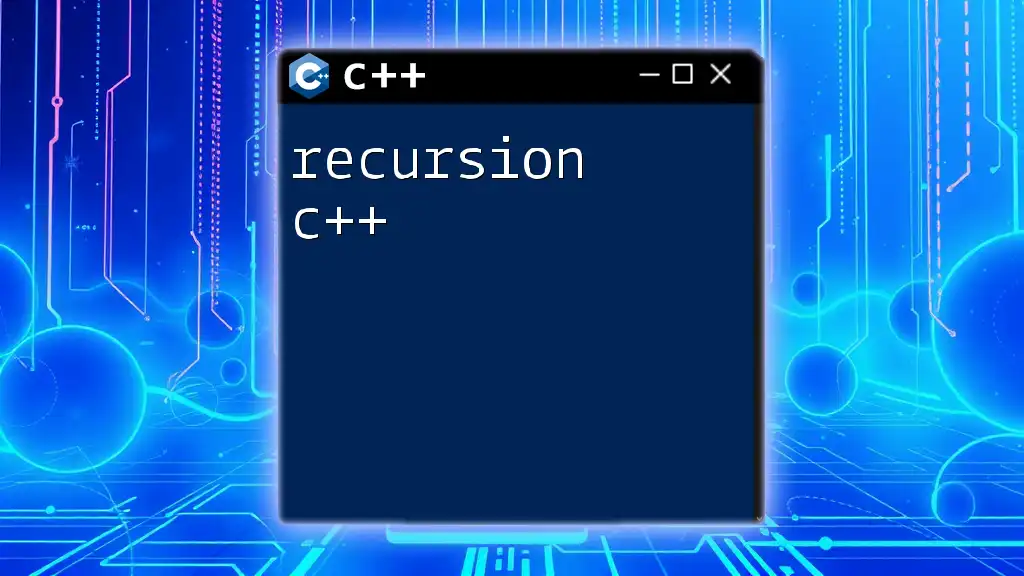
Additional Resources
For further exploration, consider visiting the official documentation for cURL [here](https://curl.se/libcurl/) and Boost.Beast [here](https://www.boost.org/doc/libs/release/doc/html/boost/beast.html). These resources offer in-depth insights and examples, enabling you to become proficient in managing HTTP requests in your C++ projects.
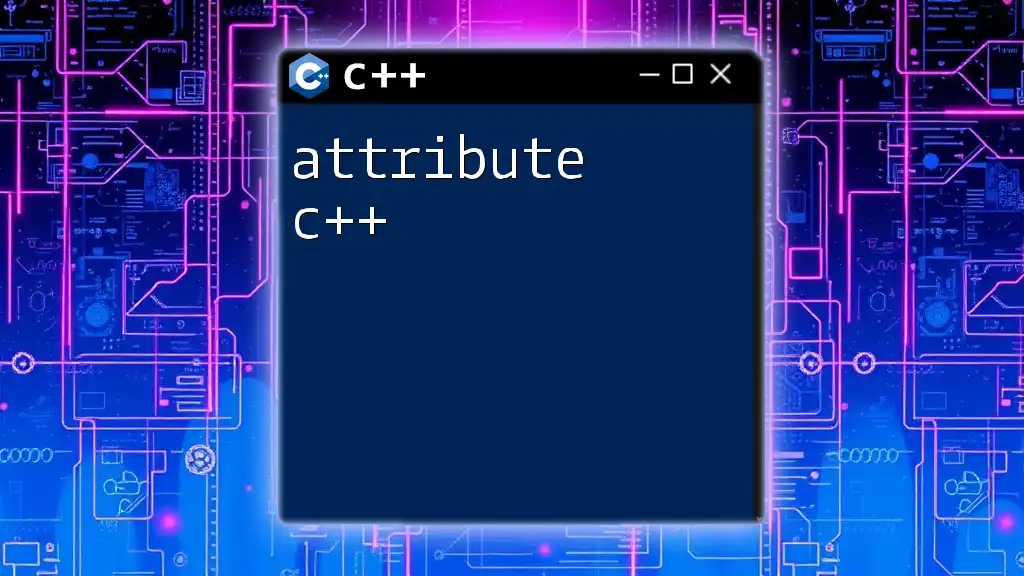
Call to Action
Now that you’ve learned the fundamentals of making HTTP requests in C++, it’s time to take action. Try implementing your own requests using the examples provided. Feel free to share your experiences or any questions you might have in the comments!